This chapter provides an introduction to classic cryptography. It explains the symmetric class of ciphers, whereby the encryption key is the same for both operations—encryption and decryption. Such ciphers are Caesar, Vigenère, Hill, and others. The main goals of this chapter are to give you a clear understanding of the main symmetric cryptographic mechanisms and to provide you with proper implementations in MATALB. These ciphers are not secure and so are not used anymore, but they are good for understanding the main concepts involved in cryptography.
Symmetric Cryptography
Generally speaking, classic cryptography ciphers/algorithms are known as symmetric systems. This is because both participants involved in the communication process use the same cryptographic key. Another important aspect, which is vital for the communication (see Figure 6-1), is based on the fact that once you find the encryption key (Enck), the decryption key (Deck) can be found/computed very quickly by computing the inverse function; see [1] and [2].
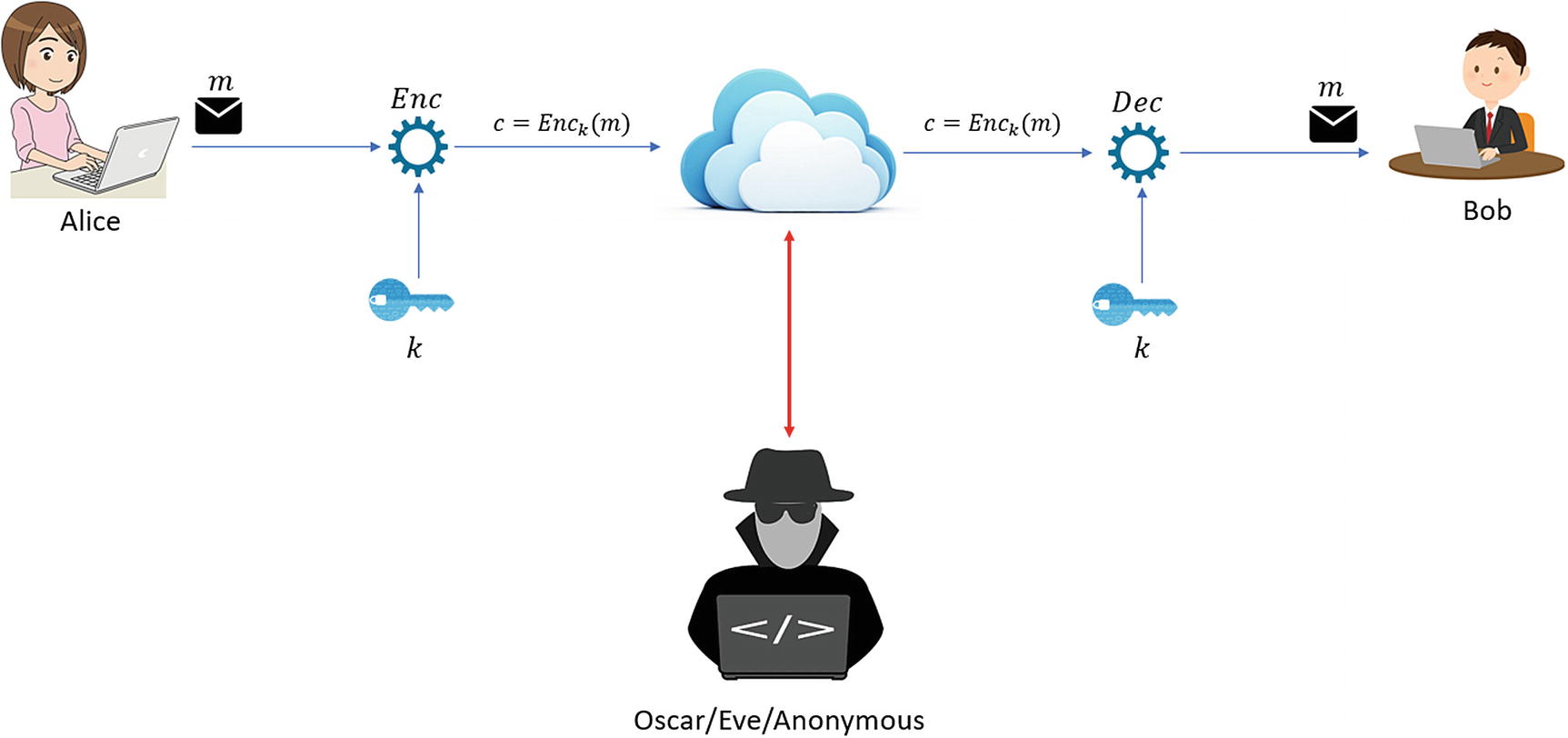
Overview of a practical scenario for symmetric encryption1
Figure 6-1 shows a general scenario that illustrates the communication process in classic cryptography (if it is used in a real communication process). The same key is used by Alice and Bob to encrypt and decrypt the message. If a malicious user gains access to the key, the entire communication process can easily be corrupted.
Classic Ciphers
This section covers the basic implementations in MATLAB of the Caesar, Vigenère, and Hill classic cryptography ciphers.
These implementations are straightforward yet provide the necessary elements for future implementations.
The goal of this section is to provide you with some basic concepts in implementing cryptography algorithms/ciphers, especially using MATLAB. This section can serve as a quick review of the main concepts. As mentioned, these kinds of algorithms/ciphers are not used anymore in real environments, but studying them can help improve your programming skills, in this case, specifically for MATLAB.
The Caesar Cipher
The Caesar Cipher represents one of the simplest and best-known ciphers. It’s a substitution cipher, whereby each letter from the plaintext is shifted a certain number of positions (characters) in the alphabet. As an example, if we shift the alphabet two spaces, the letter “A” is replaced with “C,” the letter “B” is replaced with “D,” and so on. As you will see, the Caesar Cipher is used by other classical ciphers, such as the Vigenère Cipher. The main steps of the Caesar Cipher are included as a step in the Vigenère Cipher. Another cipher that uses the Caesar encryption process is known as ROT13, which uses 13 as the offset.
Example
When we want to send a message from a person to another, the same cryptographic key is used by both partners engaged in this communication process. In the Caesar Cipher, the key is the number of characters used to shift the alphabet.
Caesar Encryption Procedure
As you can see in Example 6-1, the encryption process is very simple, as each plaintext character is shifted in the alphabet by three letters.
Encryption/Decryption Operations
If a different key is used (except k = 3), the decryption will be totally different.
Mathematical Background


Cryptanalysis
It is very easy to crack the Caesar Cipher with the help of automated tools [10]. There are only 25 possible keys representing the alphabet (0-25). Brute-force decryption methods can crack these types of encrypted messages in milliseconds.
Determine the statistics in the unencrypted text.
Compute the probability that the ciphertext is the same, using the distribution.
Implementing the Caesar Cipher
Listing 6-1 shows an implementation of the Caesar Cipher. A similar implementation can be found in [7] by Professor Alexander Stanoyevitch. Because the steps of the encryption are quite straightforward and standard, the only different or extra steps that can be added are represented by validating the inputs.
Let’s quickly review the implementation of the Caesar Cipher in Listing 6-1. As you can see, the main elements provided by the user are represented by the plaintext that we want to encrypt (the clear_text variable in Line 5) and the cryptographic key (the key in Line 8). It is very important to determine the alphabet structure (see Line 2, the alphabet variable) before proceeding with the encoding (encryption) phase.
As you can see in Line 10, we are testing if the key is situated between 0 and 26 and in Line 11 there is a modulo computation in Z26.
Implementation of the Caesar Cipher
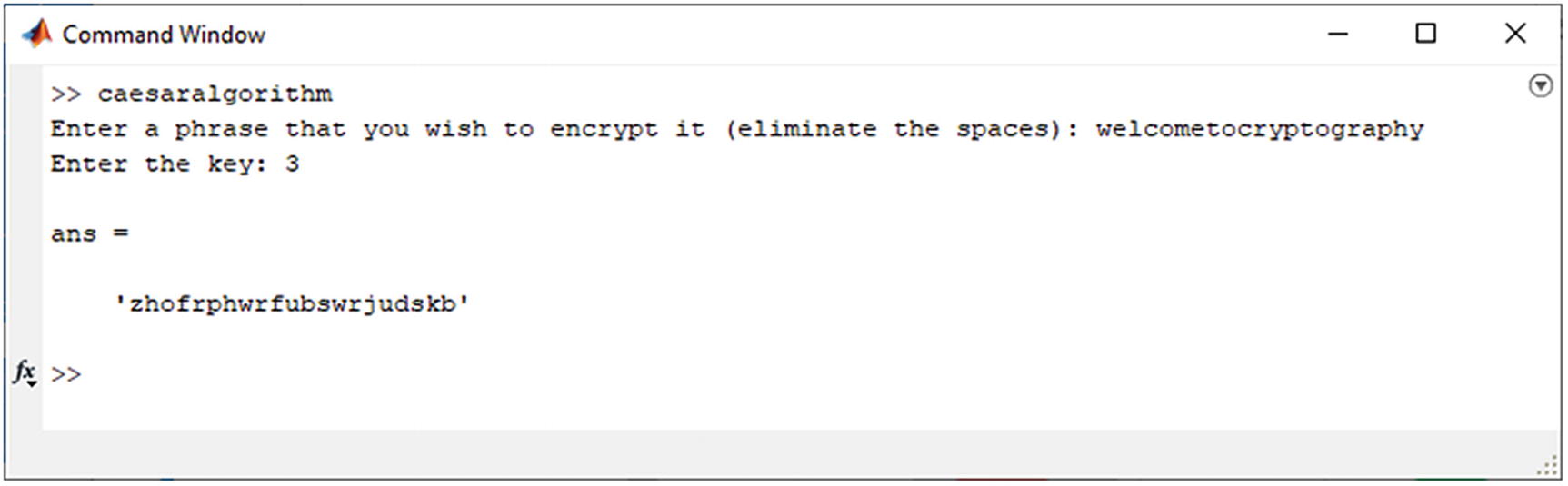
Encryption output of the Caesar Cipher
The Vigenère Cipher
The Vigenère Cipher is a polyalphabetic substitution cipher. The cipher was considered unbreakable for 300 years, and studies of it are still being published in different journals and conference proceedings.
The first successful attack on the Vigenère Cipher was published in 1863 by Friedrich Kasiski, even though Charles Babbage had designed a successful attack some years before. Another important aspect that is worth mentioning is the fact that Gilbert Vernam worked on the cipher in the early 1900s, proposing and designing what we know now as OTP (One Time Pad). His work had a great impact on current authentication systems.
Example
As an example, we use WELCOME TO THE WORLD OF CRYPTOGRAPHY as the text that we want to encrypt. The cryptographic key is APRESS.
Tabula Recta
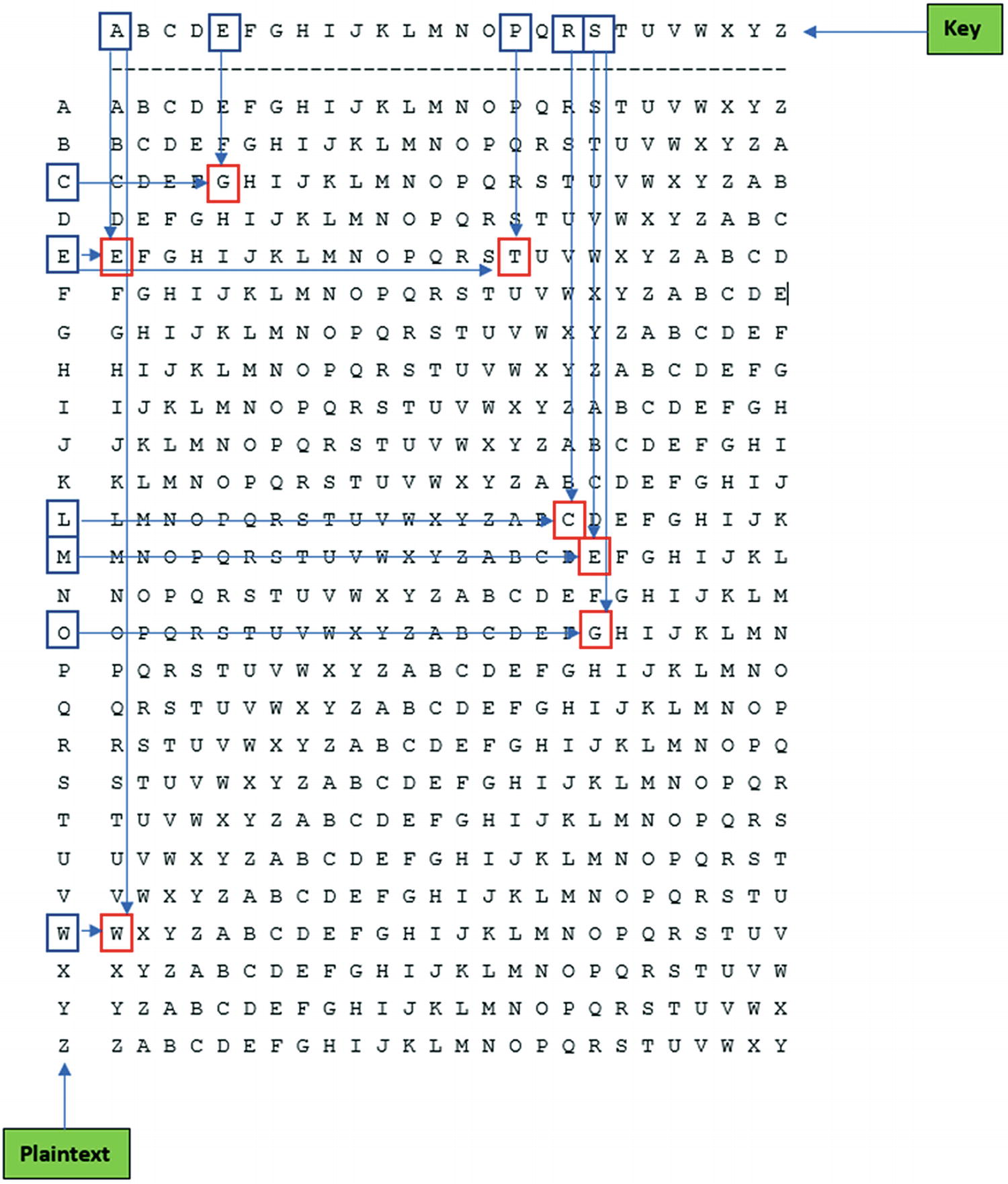
The encryption process
As an example, we took the first word of the plaintext (WELCOME) and encrypted it using the method described. For the rest of the plaintext, the procedure is the same. The result of the encryption operation is WTCGGEE, as you can see in Figure 6-3.
Mathematical Background
Notations
Notation | Definition |
---|---|
P | The space in the plaintext |
C | The space in the ciphertext |
K | The space in the keys |
c1, c2, …, cm | The characters that will be encrypted from the plaintext |
h1, h2, …, hm | The encrypted values of the characters |
k | The cryptographic key |
enck | The encryption function |
deck | The decryption function |


where the entire set of operations is computed in Z26.
Suppose in the following example, K = (7, 3). Let’s compute 7−1 mod 26 = 15. The encryption function is enck(c) = 7x + 3, and the decryption function is deck(h) = 15(h − 3) = 15h − 19.

Implementing the Vigenère Cipher
Providing an implementation of the Vigenère Cipher is easier than for the Caesar Cipher. Another implementation provided by Professor Alexander Stanoyevitch is shown in [7]. Comparing it with the version provided in Listing 6-2, there are a couple of similarities at first sight. This is due to the main steps of the cipher and the unique way of implementing them, not only in MATLAB but also in other programming languages; see [3], [4], and [6].
Implementation of the Vigenère Cipher – Encryption Function
Shift Encryption Function
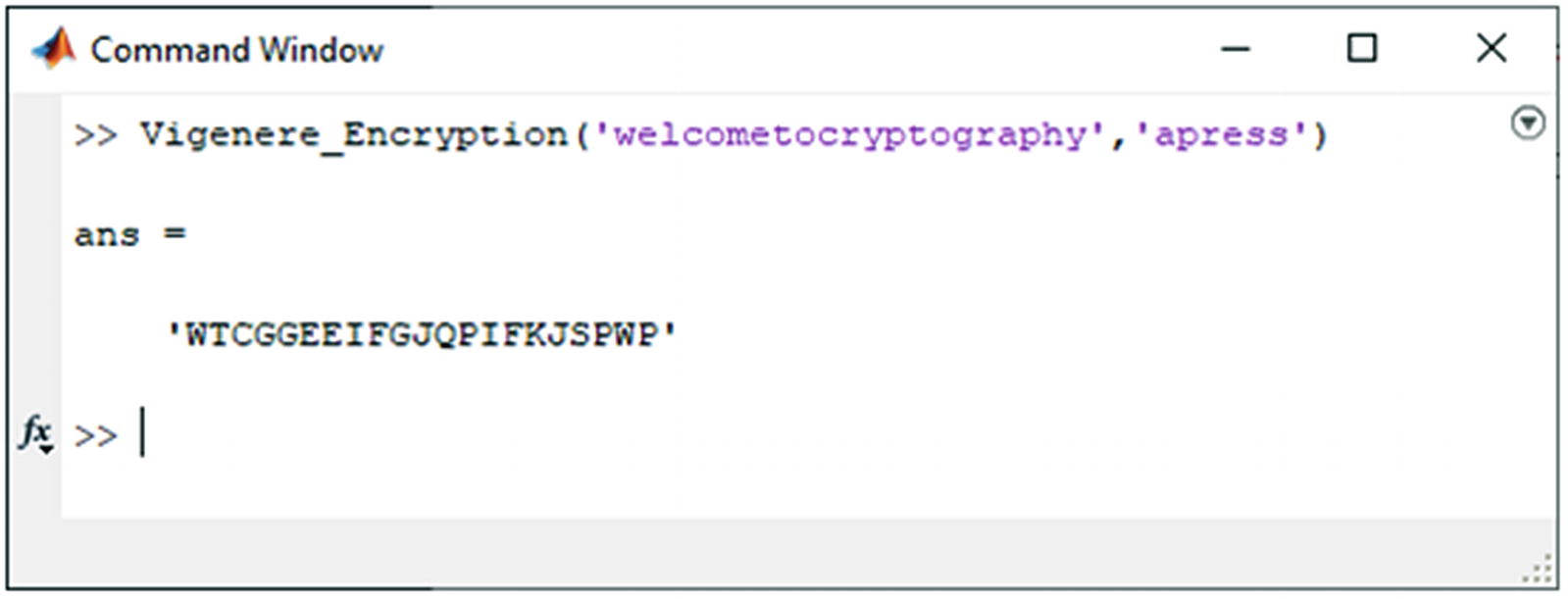
Encryption result of the Vigenère Cipher
Implementation of the Vigenère Cipher – Decryption Function
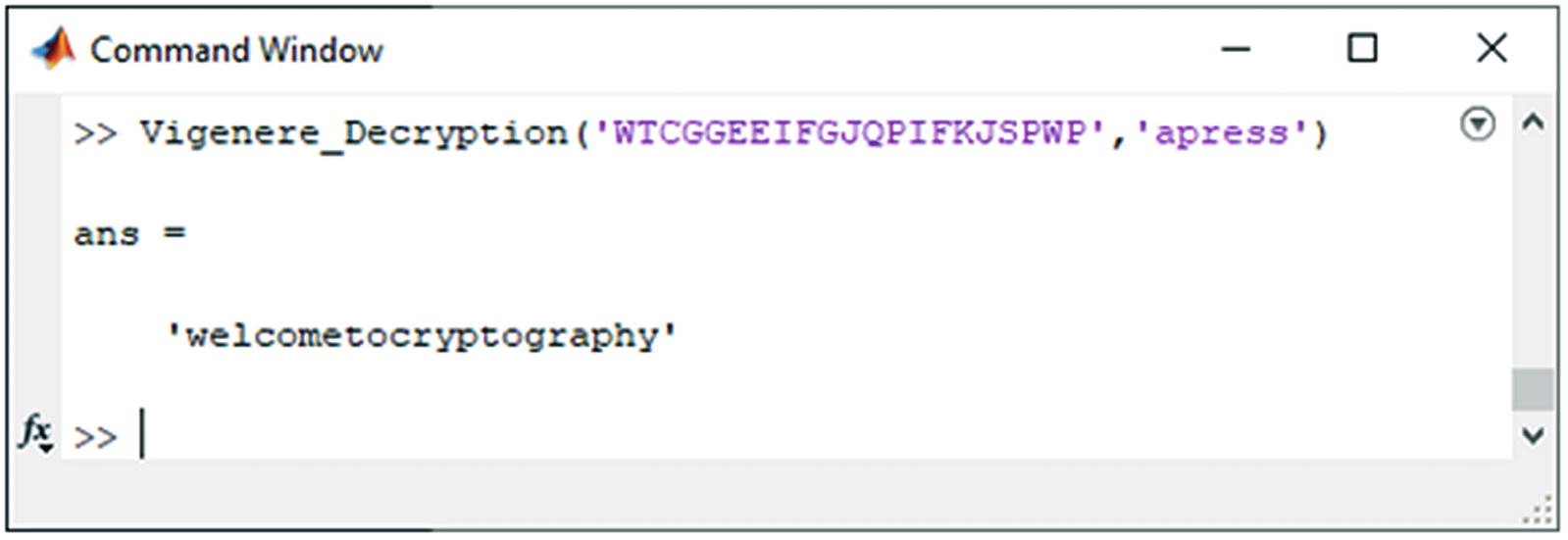
Vigenère Cipher decryption result
The Hill Cipher
The Hill Cipher was designed in 1929 by Lester S. Hill as a polygraphic substitution cipher based on linear algebra. The main essence of the Hill Cipher is that it uses matrices and matrix multiplication to mix up the plaintext.
The system proposed by Hill was designed to use a series of gear wheels and chains. Unfortunately, the proposed device was never sold.
Example
![$$ left[2 6 8 12 14 1 3 19 9
ight] $$](https://imgdetail.ebookreading.net/202111/01/9781484273340/9781484273340__9781484273340__files__images__500692_1_En_6_Chapter__500692_1_En_6_Chapter_TeX_Equf.png)
This example has a size of 3 × 3. It can be any size, as long as the matrix is a square. As an example, let’s suppose that you want to encode the message WELCOME TO CRYPTOGRAPHY. To encrypt the message, you need to divide the plaintext into pieces that are three characters/letters long. Let’s consider the first three characters/letters from the message (plaintext), WEL, and write them as an array with numeric values that correspond to the letters: W = 22, E = 4, and L = 11.
![$$ left[2 6 8 12 14 1 3 19 9
ight]left[22 4 11
ight]=left[156 331 241
ight]left(mathit{operatorname{mod}} 26
ight)=left[0 19 7
ight]= ATH $$](https://imgdetail.ebookreading.net/202111/01/9781484273340/9781484273340__9781484273340__files__images__500692_1_En_6_Chapter__500692_1_En_6_Chapter_TeX_Equg.png)
So, the encoding of WEL is ATH. This process is done for all blocks of letters from the plaintext. In some documentation, the plaintext is padded with extra letters to make it a whole number of blocks.
![$$ {K}^{-1}=left[0 19 7
ight]left(mathit{operatorname{mod}} 26
ight)=left[22 4 11
ight]= WEL $$](https://imgdetail.ebookreading.net/202111/01/9781484273340/9781484273340__9781484273340__files__images__500692_1_En_6_Chapter__500692_1_En_6_Chapter_TeX_Equh.png)
Cryptanalysis
The Hill Cipher is very vulnerable to a known plaintext attack. Once you know the plaintext and the encrypted version of it, the key can be easily recovered. This is possible because everything is linear.
If you are dealing with a 2 × 2 case of the Hill Cipher, you can launch the attack by computing the frequencies of all the digraphs occurring within the encrypted version of the plaintext. It is very important to understand and to have some familiar knowledge of standard English digraphs, such as “th” and “he”. For more information about the cryptanalysis process, resource [8] is recommended, especially for professionals working with old manuscripts, documents, letters, etc.
Implementing the Hill Cipher
Implementing the Hill Cipher in different programming languages (e.g., C#, Java, or C++), as shown in [3] and [4], can be a real adventure due to the multiple steps that need to be achieved. When we are doing the implementation in MATLAB, things are straightforward, as shown in Listing 6-5. A similar version is provided by Professor Alexander Stanoyevitch in [7].
Remember that the matrix given as the second parameter in the call must be inverse modulo Z26.
Let’s continue with a quick overview of the source code provided in Listing 6-5. We will start the analysis with Line 2, in which we compute the size of the matrix provided by the user as input (see Figure 6-6). In Line 3, we make sure that all the characters from the plaintext are lowercase letters. Once we have the entire array of characters, in Line 4 we compute the length. In Line 5, we compute a padded value (append_array_length) based on the modulo operation between the length of the array (plaintext) and the size of the square matrix (n).
The Hill Cipher Encryption Function

Encryption output of the Hill Cipher
Conclusion
This chapter discussed symmetric cryptography as a main entry point into practical cryptography, providing basic information so you can acquire the basic programming skills you need in MATLAB.
For this purpose, we focused on the Caesar, Vigenère, and Hill Ciphers, as they are well-known ciphers in classic cryptography and are used often during the learning process.
References
- [1]
Atanasiu, Adrian. Securitatea Informației – Volumul 1: Criptografie. InfoData, 2007. ISBN: 978-973-1803-16-6.
- [2]
Menezes, A. J., et al. Handbook of Applied Cryptography. CRC Press, 1997.
- [3]
Mihailescu, Marius Iulian, and Stefania Loredana Nita. Pro Cryptography and Cryptanalysis: Creating Advanced Ciphers with C# and .NET. Apress, 2021. DOI.org (Crossref), doi:10.1007/978-1-4842-6367-9.
- [4]
Mihailescu, Marius Iulian, and Stefania Loredana Nita. Pro Cryptography and Cryptanalysis with C++20: Creating and Programming Advanced Ciphers. Apress, 2021. DOI.org (Crossref), doi:10.1007/978-1-4842-6586-4.
- [5]
Paar, Christof, and Jan Pelzl. Understanding Cryptography: A Textbook for Students and Practitioners. Springer Berlin Heidelberg, 2010. DOI.org (Crossref), doi:10.1007/978-3-642-04101-3.
- [6]
Schneier, Bruce. Applied Cryptography: Protocols, Ciphers, and Source Code in C. 20th anniversary edition, Wiley, 2015.
- [7]
Stanoyevitch, Alexander. Introduction to Cryptography with Mathematical Foundations and Computer Implementations. CRC Press, 2011. ISBN: 979-8651423514.
- [8]
Practical Cryptography (website). Hill Cipher - http://practicalcryptography.com/ciphers/classical-era/hill/.
- [9]
Practical Cryptography (website). Quadgram Statistics as a Fitness Measure - http://practicalcryptography.com/cryptanalysis/text-characterisation/quadgrams/.
- [10]
Practical Cryptography (website). Cryptanalysis of Caesar Cipher - http://practicalcryptography.com/cryptanalysis/stochastic-searching/cryptanalysis-caesar-cipher/.
- [11]
Practical Cryptograhy (website). Caesar Cipher - http://practicalcryptography.com/ciphers/caesar-cipher/.
- [12]
Practical Cryptography (website). Ciphers - http://practicalcryptography.com/ciphers/.
- [13]
Cipher Types (website). https://www.cryptogram.org/resource-area/cipher-types/.