Delphi Pascal or, simply, Delphi is the most popular version of Object Pascal which, in turn, is an extension of the classic Pascal programming language (Cantu, 2016). This chapter introduces the basic concepts of the language.
Delphi As a Programming Language
Delphi is a general-purpose programming language. As a Pascal descendent, it draws its strong typing and syntactical characteristics from the original Pascal language developed by Niklaus Wirth in the early 1970s, but it, loosely, relates to the ISO standard Pascal (i.e., it is not a superset). Over the past decades, Delphi has evolved, and now it has features that makes it a modern programming language capable of building professional software in multiple platforms.
Syntax
If you look at Delphi source code, you will notice that it is dominated by words rather than symbols. Code appears inside a begin...end block rather than inside symbols like curly brackets ({..}) as in other languages.
Typically, code flows from top to bottom and from left to right. This implies that variables, objects, constants, and other elements need first to be declared before they are used (with the exception of forward declaration of classes).
Delphi is case insensitive, meaning that coding elements like variables, objects, methods, and the like can be declared in small or capital letters or in a combination of both. For example, the following declarations are all valid in Delphi: delphiBook, delphi_Book, DelphiBook, DELPHIBOOK, delphiBOOK. There are naming rules which prohibit the use of specific characters (e.g., an identifier cannot start with a number, etc.), but the limitations are very few, and, practically, when you code in Delphi, it is not common to come across them.
A notable difference with other languages is the operator to assign values to variables. In Delphi, a colon followed by the equal sign (:=) is used for this purpose, and the simple equal sign (=) is used to test equality in expressions.
Lastly, a convention that survived from the classic Pascal is the way the end of code line is declared in Delphi. Most of the lines of code end with a semicolon (;) with the exception of keywords (e.g., begin...end, if...then, while...do, etc.) and the last keyword in a code file. Every code file ends with the keyword end followed by a period (end.)
Programming Paradigms
Delphi is a fully developed object-oriented programming (OOP) language but does not force any specific development paradigm. You are free to use the OOP approach, but if, for some reasons, you prefer to use pure procedural programming, Delphi can fully support you. In fact, a huge part of the native libraries in Delphi come as procedures rather than embedded in objects and classes. This stands for Windows API calls, but, as the language is moving to cross-platform code, more libraries come in classes and records.
Compilation to Native Code
The final artifact of compilation of Delphi code is binary files with native code. In computing, this means that the final files represent machine code instead of an intermediate form like the one you find in virtual machine bytecode other languages produce. As a result, the executables run directly on top of the operating system without any translation layers between the executables and the underlying APIs of the operating systems.
Visual Applications
Delphi provides two out-of-the-box frameworks to support the development of visual applications: the Visual Component Library (VCL) and, starting from Delphi XE2, the FireMonkey (FMX) framework . VCL is used for Windows applications only, and FMX provides cross-platform components to build graphical user interfaces. Apart from VCL and FMX, there are third-party frameworks and libraries available to enrich the development of visual applications.
One Code Base for Multiple Platforms
One of the most distinguished characteristics of modern Delphi is the ability to produce binaries for multiple platforms from the same code base. At the time of writing, there are very few development tools in the market that truly support this. This means that, as a developer, you write code without any considerations as to which platform it will compile to, and Delphi takes the task to produce the appropriate executables or libraries for the platform of your choice. Currently, Delphi supports the following platforms: Windows 32-bit, Windows 64-bit, macOS 32-bit, macOS 64-bit, Android 32-bit, Android 64-bit, iOS, iOS 32-bit, iOS 64-bit, iOS Simulator, and Linux 64-bit. It is worth mentioning that although you can create applications for all the preceding platforms, the development is done on Windows only; that is, the compilers are Windows programs themselves.
Although you can write cross-platform code without considering the details of the target platform, it is almost inevitable that your code, at some stage, will need to take advantage of the specificities of the target operating system. For that matter, Delphi allows you to fine-tune your code base using compiler directives and attributes.
Anatomy of a Delphi Program
A typical Delphi program can generate a number of different files depending on the nature of the program and the target platform.
Project Files
A program in Delphi has one source code file saved under the name of the application and with the .dpr extension. The code starts with the program keyword followed by the name of the application, and it has one main block of code enclosed in the begin..end keywords. The last end keyword is followed by a period (end.), and this signifies the end of the code file. Any text that appears after this generates a warning, but it is ignored by the compiler.
Delphi also generates a file with the .dproj extension. This file holds vital information about the cross-platform configurations, and it can also be used when the compilation of code is streamlined to MSBUILD.
There are a number of other support files with different extensions (e.g., .local, .deployproj) you may find, but they are not vital for the correct compilation of a Delphi program, or the compiler can regenerate them automatically.
Units
You can, very easily, create one big file and store all your code in it (with the exception of visual elements like forms and frames). Delphi will not complain and will compile your code correctly. However, this does not sound something that scales up easily when you write complex software. Instead, common practice suggests to organize your code in smaller separate files or modules as they are known in software engineering.
Delphi is a modular language and provides support to modules via unit files. In Pascal world, the term unit is used instead of module. The term module still exists in Delphi, and it refers to a special component (TDataModule) which sits in its own separate unit file. A unit is a separate code file, it has the .pas extension, and it is linked back to the project and is compiled to a binary file with the extension .dcu. DCUs are more important than the source code files because the compiler is able to use a .dcu file without the need to locate and access the corresponding .pas file. The downside is that DCU files are tightly linked to the version of the compiler that was used to create them. There were some exceptions in the past, but this is the general rule.
The uses clause can appear either in the interface or the implementation part of a unit.
Forms and Frames
A form in Delphi is a representation of the typical window you see in visual applications. If you want to add a label or an edit field in the window, you add them in a form, and, when the code is executed, you see a window with the components.
Delphi creates two files for each form: a typical .pas file which contains all the declarations and any custom code you want to add to alter the behavior of the form and a .dfm (in VCL) or .fmx (in FireMonkey) file which holds information about the components in a form. A valid form needs both files.
Frames are very similar to forms with the difference that they do not represent stand-alone windows and they do not have system menus and icons. A frame can be embedded in forms or in other frames to build more complex and reusable user interfaces. In terms of files, frames use the same file structure as forms.
Delphi As Integrated Development Environment (IDE)
It is very possible to use a simple text editor to write Delphi code and then compile it using the compiler. This is the typical workflow of writing software in other programming languages.
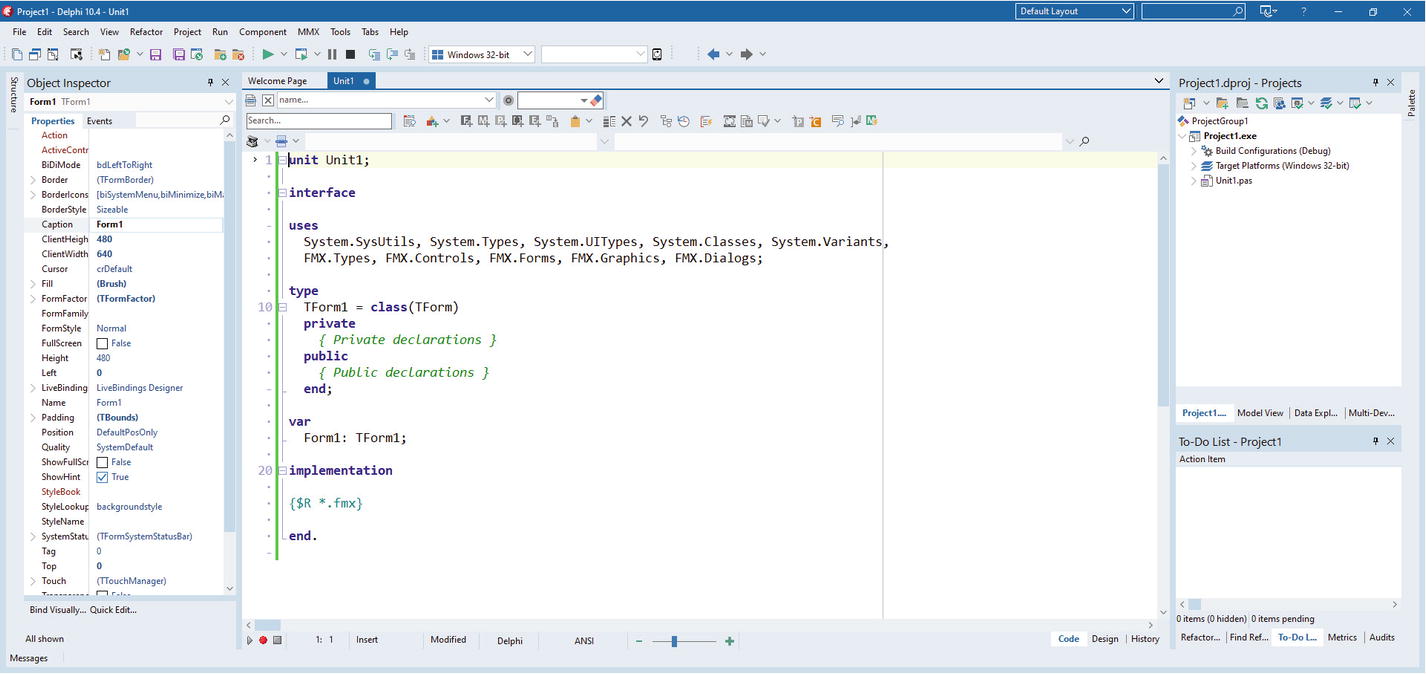
The Code View of Delphi IDE (Delphi 10.4 Sydney)

The Form Designer in RAD Studio (Delphi 10.4 Sydney)
The Delphi IDE is one of the most feature-rich development environments that exist in the market. If you would like to learn more, please read the official documentation for the latest release of the IDE (Embarcadero, n.d.) or download and install either the community edition or the trial version from the product’s home page (Embarcadero, 2020).
A Simple Application (Console)
The simplest application you can create is a console application. This type of application does not have any graphical environment. It provides a text-only interface (Windows Console, macOS Terminal, etc.), and the interaction with the user is done via the keyboard and the display screen.
- 1.
Open Delphi IDE.
- 2.
Select the File ➤ New ➤ Console Application – Delphi menu item.
This will create a simple console application with the minimum code to support the development of a console application.
- 3.
Save the project under the name Cheers.
- 4.We just want to print a simple message in the console. Go to the Code Editor by clicking on the tab at the bottom of the main part of the screen and add the following lines (in bold):program Cheers;{$APPTYPE CONSOLE}{$R *.res}usesSystem.SysUtils;begintry{ TODO -oUser -cConsole Main : Insert code here }Writeln('Hey Delphi, Cheers!');Writeln('Press Enter');Readln;excepton E: Exception doWriteln(E.ClassName, ': ', E.Message);end;end.
- 5.
Then either go to Run ➤ Run menu item, press F9 or use the relevant button in the toolbar. This will compile and execute the code, and you will be able to see the output in a console window (Figure 1-3). Press Enter to close it and return to the IDE.
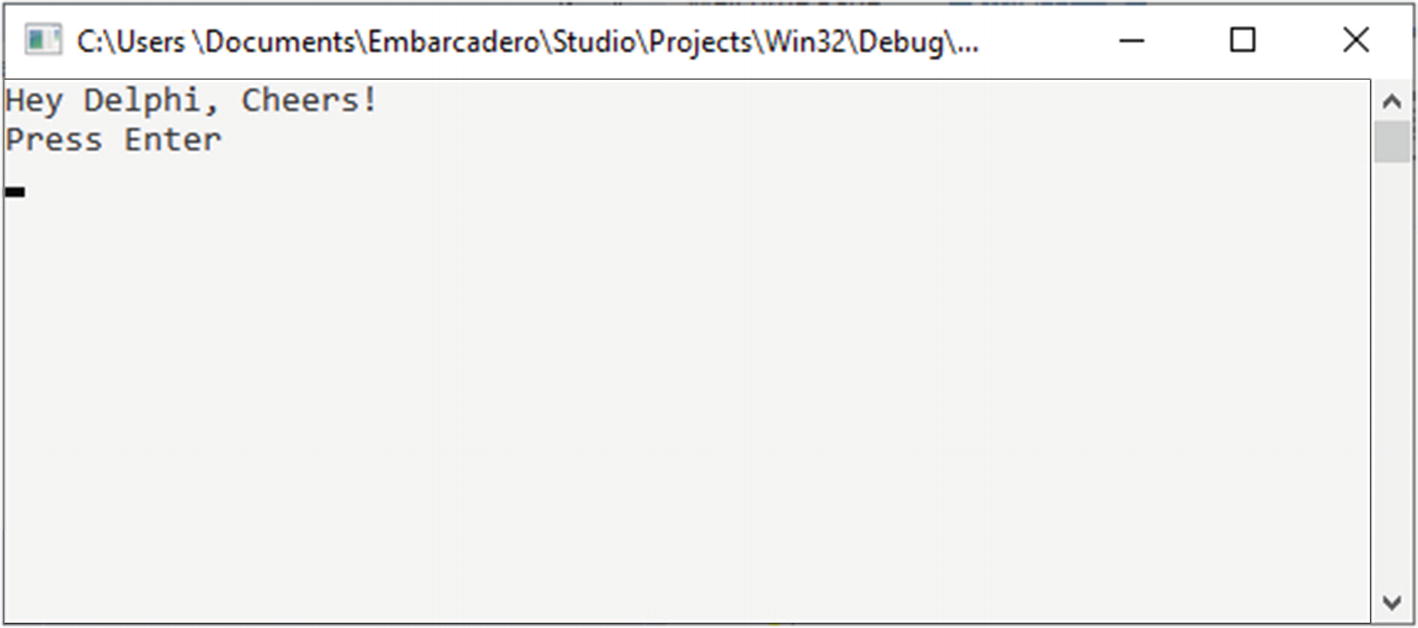
Simple Output to Console
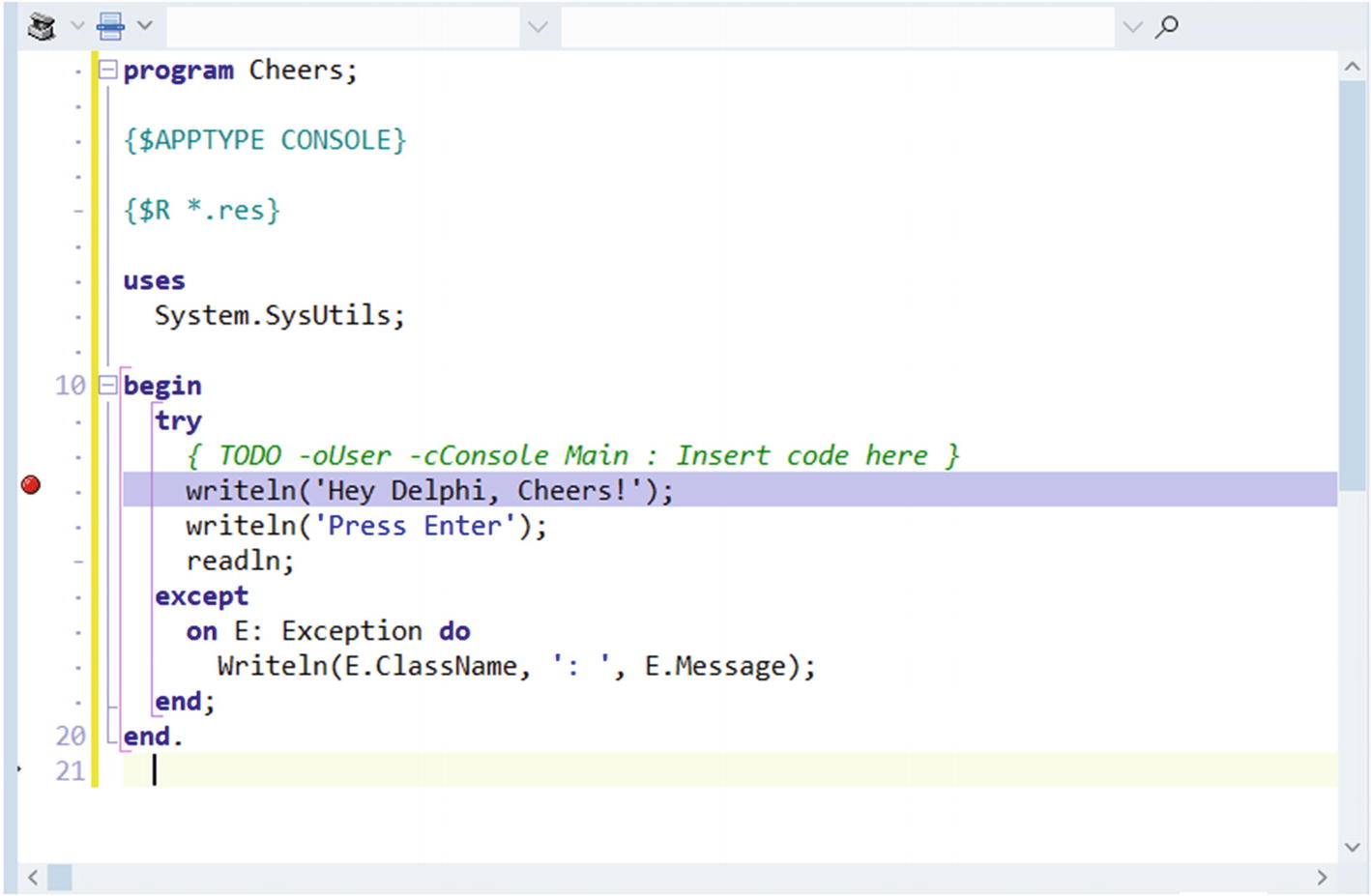
Breakpoints in the Delphi IDE
Run again the project by pressing F9. This time the execution will stop at the line with the breakpoint, and you will be able to step through the code gradually by using the debugger buttons in the toolbar.
A Simple Application (Graphical)
- 1.
Select File ➤ New ➤ Windows VCL Application – Delphi or Multi-Device Application – Delphi from the main menu.
The VCL, obviously, uses the VCL framework, and the Multi-Device Application uses FireMonkey (FMX).
- 2.
If you select Multi-Device Application, you will be offered a list of different types of FMX applications (templates). Just select Blank Application.
- 3.
Now the IDE will open the form designer.
- 4.Use the Palette panel (usually located on the right-hand side of the screen), find the TButton component (Figure 1-5), and drag and drop it to the form. Alternatively, you can click once the TButton and then click again somewhere in the form. This will add a button (Figure 1-6).Figure 1-5
Selecting TButton from the Palette
- 5.Double-click the button. The designer will change to the code editor and will add some code. Then, add the following code:procedure TForm1.Button1Click(Sender: TObject);beginShowMessage('Hey Delphi, Cheers!');end;

Form Designer in Delphi IDE (Delphi 10.4 Sydney)
- 6.
Run the application and click the button. You are greeted with a message (Figure 1-7).
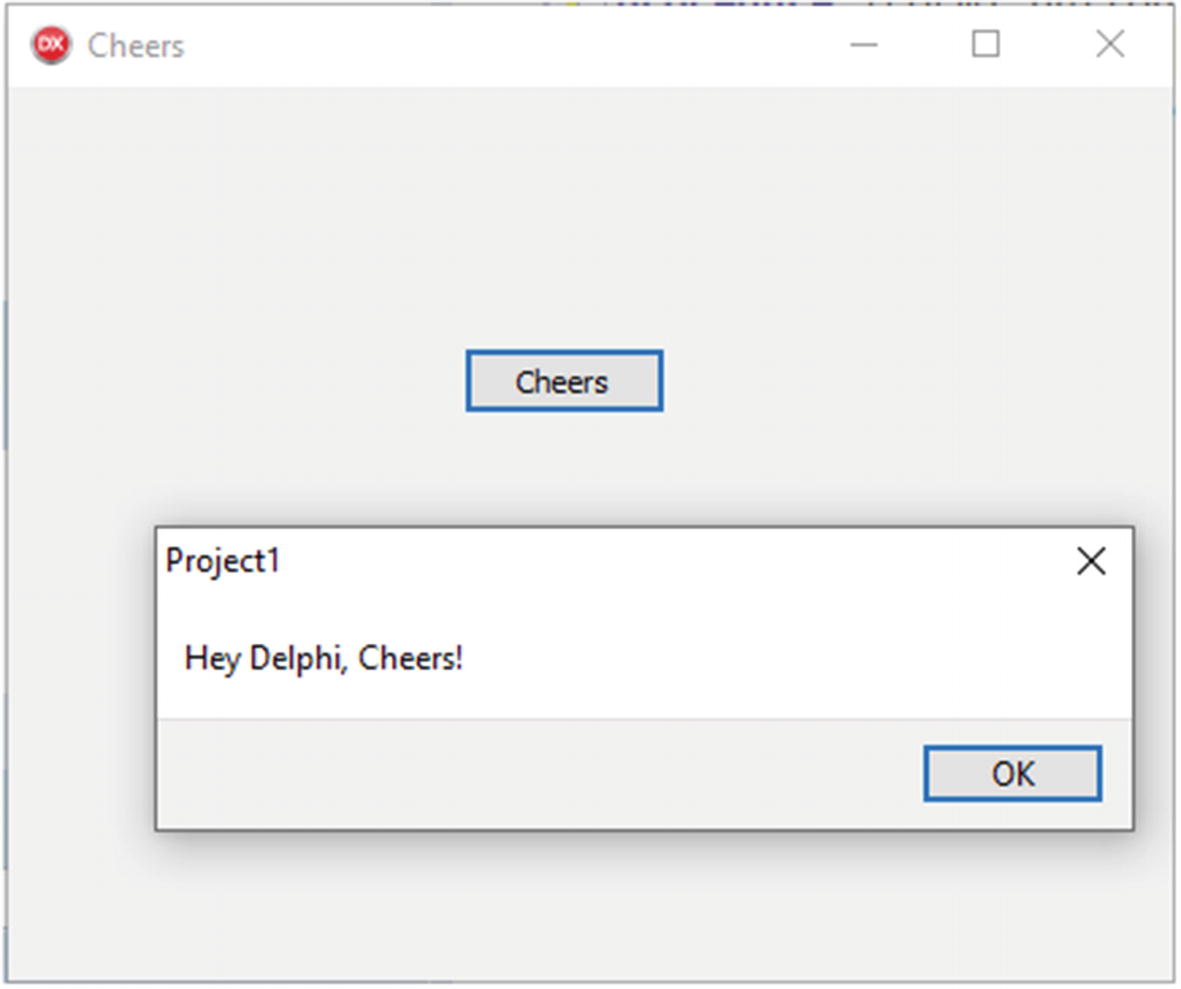
VCL Application in Delphi IDE (Delphi 10.4 Sydney)
Alternative IDEs
As mentioned earlier, Delphi development is done in Delphi IDE, which comes together with the compilers. The only other alternative to write pure Delphi code is to use Visual Studio Code with the OmniPascal extension (OmniPascal, 2020). OmniPascal adds to Visual Studio Code the capability to understand Delphi syntax and then to compile, debug, and run Delphi programs. The only downside is that it does not offer a form designer, which means that the Delphi IDE remains the only way to develop graphical applications in Delphi.
If we open the scope of the IDE and look at the domain of Object Pascal more broadly, there is another IDE worth mentioning. The Free Pascal community offers Lazarus (Lazarus, 2020) which is a cross-platform open source IDE. Lazarus is highly compatible with Delphi, but it is primarily made to support Free Pascal—another flavor of Object Pascal.
Delphi Style Guide
Delphi allows coders to use any naming conventions (with some exceptions as mentioned earlier) they feel work best for them and make their code readable especially when teams of developers are involved.
As it happens in every programming language, over the years, specific approaches to naming and other syntactical elements have emerged, and they are now commonly used among Delphi developers. For a complete guide, check this post (Calvert, n.d.). There are also some commonly found approaches to naming variables which are summarized in this piece (Riley, 2019).
Summary
In this chapter, we started with a very basic introduction of Delphi as a programming language. Then, we touched upon RAD Studio, the integrated environment that is, almost exclusively, used to develop Delphi software. In the next chapter, we review the basic elements of the language.
References
Calvert, C., n.d.. Object Pascal Style Guide. [Online] Available at: http://edn.embarcadero.com/article/10280#2.0 [Accessed 27 04 2020].
Cantu, M., 2016. Object Pascal Handbook. s.l.:s.n.
Embarcadero, 2020. RAD Studio Product Page. [Online] Available at: www.embarcadero.com/products/rad-studio [Accessed 08 04 2020].
Embarcadero, n.d. RAD Studio Rio. [Online] Available at: http://docwiki.embarcadero.com/RADStudio/Rio/en/Main_Page [Accessed 08 04 2020].
Lazarus, 2020. Lazarus. [Online] Available at: www.lazarus-ide.org/ [Accessed 08 04 2020].
OmniPascal, 2020. OmniPascal. [Online] Available at: www.omnipascal.com/ [Accessed 08 04 2020].
Riley, M., 2019. What is the “A” prefix I see used on parameters?. [Online] Available at: https://capecodgunny.blogspot.com/2019/03/delphi-tip-of-day-what-is-a-prefix-i.html [Accessed 27 04 2020].