JBehave is a Java BDD framework used for writing acceptance tests that are able to be executed and automated. The steps used in stories are bound to Java code through several annotations provided by the framework:
- First of all, add JBehave to Gradle dependencies:
dependencies { testCompile 'org.jbehave:jbehave-core:3.9.5' }
- Let's go through a few example steps:
@Given("I go to Wikipedia homepage") public void goToWikiPage() { open("http://en.wikipedia.org/wiki/Main_Page"); }
- This is the Given type of step. It represents a precondition that needs to be fulfilled for some actions to be performed successfully. In this particular case, it will open a Wikipedia page. Now that we have our precondition specified, it is time to define some actions:
@When("I enter the value $value on a field named $fieldName")
public void enterValueOnFieldByName(String value, String fieldName) { $(By.name(fieldName)).setValue(value); } @When("I click the button $buttonName") public void clickButonByName(String buttonName){ $(By.name(buttonName)).click(); }
- As you can see, actions are defined with the When annotation. In our case, we can use those steps to set some value to a field or click on a specific button. Once actions are performed, we can deal with validations. Note
that steps can be more flexible by introducing parameters:
@Then("the page title contains $title") public void pageTitleIs(String title) { assertThat(title(), containsString(title)); }
Validations are declared using the Then annotation. In this example, we are validating the page title as expected.
These steps can be found in the WebSteps class in the https://bitbucket.org/vfarcic/tdd-java-ch02-example-web repository.
Once we have defined our steps, it is time to use them. The following story combines those steps in order to validate a desired behavior:
Scenario: TDD search on wikipedia
It starts with naming the scenario. The name should be as concise as possible, but enough to identify the user case unequivocally; it is for informative purposes only:
Given I go to Wikipedia homepage When I enter the value Test-driven development on a field named search When I click the button go Then the page title contains Test-driven development
As you can see, we are using the same steps text that we defined earlier. The code related to those steps will be executed in a sequential order. If any of them are halted, the execution is halted and the scenario itself is considered failed.
Even though we defined our steps ahead of stories, it can be done the other way around with a story being defined first and the steps following. In that case, the status of a scenario would be pending, meaning that the required steps are missing.
This story can be found in the wikipediaSearch.story file in the https://bitbucket.org/vfarcic/tdd-java-ch02-example-web repository.
To run this story, execute the following:
$> gradle testJBehave
While the story is running, we can see that actions are taking place in the browser. Once it is finished, a report with the results of an execution is generated. It can be found in build/reports/jbehave:
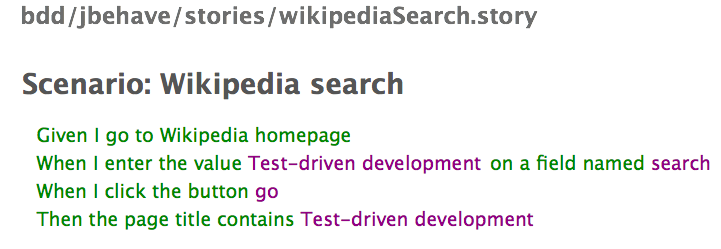
For brevity, we excluded the build.gradle code to run JBehave stories. The completed source code can be found in the https://bitbucket.org/vfarcic/tdd-java-ch02-example-web repository.
For further information on JBehave and its benefits, visit http://jbehave.org/.