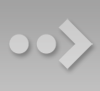
almost anything will do, following a few simple rules will generally make all your pro-
gramming easier. Here we will discuss only a few language-independent rules, and point
you to other books in the Suggested Readings section at the end of this chapter.
n
The most important rule is to be consistent—especially in your choice of names, capi-
talization, and programming conventions. If you are programming alone, the particular
choice of conventions is not important as long as you are consistent. You should also
try to follow the established conventions of the programming language you are using,
even if it would not otherwise be your choice. This will ensure that you do not introduce
two conventions. For example, it is established practice in Java to start class names with
uppercase letters, and variable names with lowercase letters. If your name has more than
one word, use capitalization to signal the word boundaries. This results in names such
as
FileClass and fileVariable. In C, the convention is to use lowercase almost exclu-
sively and to separate with an underscore. Thus, when we program in C, we follow the
C conventions. The choice of words for common operations is also dictated by conven-
tion. For example, printing, displaying, showing, or echoing a variable are some of the
terminologies meaning similar actions. Language conventions also provide hints as to
default names for variables, preference for shorter or longer names, and other issues.
Try to be as consistent as possible in your choice, and follow the conventions for your
language.
n
Choose names carefully. In addition to being consistent in naming, try to make sure
names for functions and variables are descriptive. If the names are too cumbersome
or if a good name cannot be easily found, that is usually a sign that there may be a
problem in the design. A good rule of thumb is to choose long, descriptive names
for things that will have global scope such as classes and public methods. Use short
names for local references, which are used in a very limited scope such as local vari-
ables, private names, and so on.
n
Test before using a function or method. Make sure that it works. That way if there are
any errors, you know that they are in the module you are currently writing. Careful unit
testing, with test cases written before or after the unit, will help you gain confidence in
using that unit.
n
Know thy standard library. In most modern programming languages, the standard
library will implement many common functions, usually including sorting and collec-
tions of data, database access, utilities for web development, networking, and much
more. Don’t reinvent or reimplement the wheel. Using the standard libraries will save
extra work, make the code more understandable, and usually run faster with fewer
errors, because the standard libraries are well debugged and optimized. Keep in mind
that many exercises in introductory programming classes involve solving classic prob-
lems and implementing well-known data structures and algorithms. Although they are
a valuable learning exercise, that does not mean you should use your own implemen-
tations in real life. For our sample programming problem, Java has a sorting routine
that is robust and fast. Using it instead of writing your own would save time and effort
and produce a better implementation. We will still implement our own for the sake of
illustration but will also provide the implementation using the Java sorting routine.
91998_CH01_Tsui.indd 9 1/10/13 6:19:01 AM