The primary goal of this chapter is to clearly explain how blocks are mined in a blockchain. Indirectly, this explains how new currency comes into being, as well as how disparate people forming a network can all reach consensus (agree on the state of the blockchain). And so, you’ll leave this chapter with a practical understanding of Proof of Work—the protocol which achieves this.
We’ll also see that due to the peer-to-peer, decentralized nature of peer-to-peer networks, there is never one single blockchain at any given time. There are many valid chains at once, but over time, peers reach consensus on what the authoritative chain is.
In the previous chapter, we implemented the methods in our blockchain class. We also learnt how blocks are “chained” together using hashes and implemented methods to create and add new blocks. It’s crucial that you have a firm grasp of hashing as this chapter is where the real magic begins—we’ll be clarifying the concept of “mining” or, more formally, creating proofs of work.
Interacting with the blockchain class using iPython
Going forward, you’ll need to be proactive in playing with your blockchain class to gain an intuitive understanding of the upcoming concepts.
Introduction to proof of work
In forming Bitcoin, Satoshi Nakamoto’s genius was not in creating, but rather in the amalgamation of preexisting breakthroughs by cryptographers concerned with the erosion of privacy. These breakthroughs span disparate fields and topics, such as addressing the problem of centralization and trust by leveraging peer-to-peer networks originally used to share files over the Internet (torrents); or more specifically using technology invented to combat email spam to solve the problem of “minting” money.
But Bitcoin’s biggest breakthrough was the leveraging of proofs of work to form consensus among peers on a network who don’t know each other, and more importantly, don’t trust each other. In simpler words: in a decentralized network where everyone has an equal say, we need a way of agreeing on things; things like whether a transaction is fraudulent or if a blockchain is valid. Now, we may be jumping the gun here (we’ll clarify most of these concepts soon), but it’s important to zoom out and see where the different parts of the puzzle fit.
Proof of work or, more formally, a proof-of-work (POW) algorithm describes the method in which new blocks are added to a blockchain. Bitcoin and Ethereum (at present) both use proof-of-work algorithms to add new blocks to their blockchains. Proof-of-work algorithms sound pretty complicated, but in practice they’re actually very simple. To get the cogs turning before we dive in, I’d like to pose a problem to you: How do you prove to someone that you did work?
Think about it for a minute or two. Let’s say that you’re digging holes in your backyard and you find a gold nugget. Is that gold nugget proof that effort was done to obtain it? I’d argue that it’s proof that someone did some work. Let’s switch gears here for a second—how about proof that you ran a marathon? You could probably strap a camera to your head and record the whole race, step by step. That would be sufficient, wouldn’t it? Maybe, but the footage could’ve been modified, or someone else may have been wearing the camera. Perhaps we’re grabbing at straws here, but I’m trying to draw your attention to just how difficult it is to prove that work was done without doubt (or trust), especially if you’re behind a computer, which is pretty handy at faking things.
In lay terms, proof-of-work algorithms achieve just this—they allow you to prove that your computer did some work. More technically, proof-of-work algorithms are consensus mechanisms—if you can prove that work was done, then you can show your proof to someone else who can easily verify that it is true. There are many variants of these algorithms, but we’re going to focus on a simple example to sink this in.
We’ve already explained that POW is the algorithm for mining new blocks. The actual method of the proof of work algorithm is simple—to discover a number which satisfies a small mathematical problem: the number must be difficult to find but easy to verify, computationally speaking, by anyone on the network. This is the core idea behind proof of work: difficult to do, but easy to verify. We’ll look at a very simple example to help this sink in.
A trivial example of Proof of Work
In Bitcoin, the proof-of-work algorithm has a name; it is called Hashcash . And it’s not too different from the preceding basic example. It’s the algorithm that miners race to solve in order to create a new block. In general, the difficulty is determined by the number of zeros at the beginning of a string; the miners are then rewarded for their solution by receiving a set number of Bitcoins—in a transaction; and the network is able to easily verify their solution, as we did earlier.
An analogy: Jigsaw puzzles

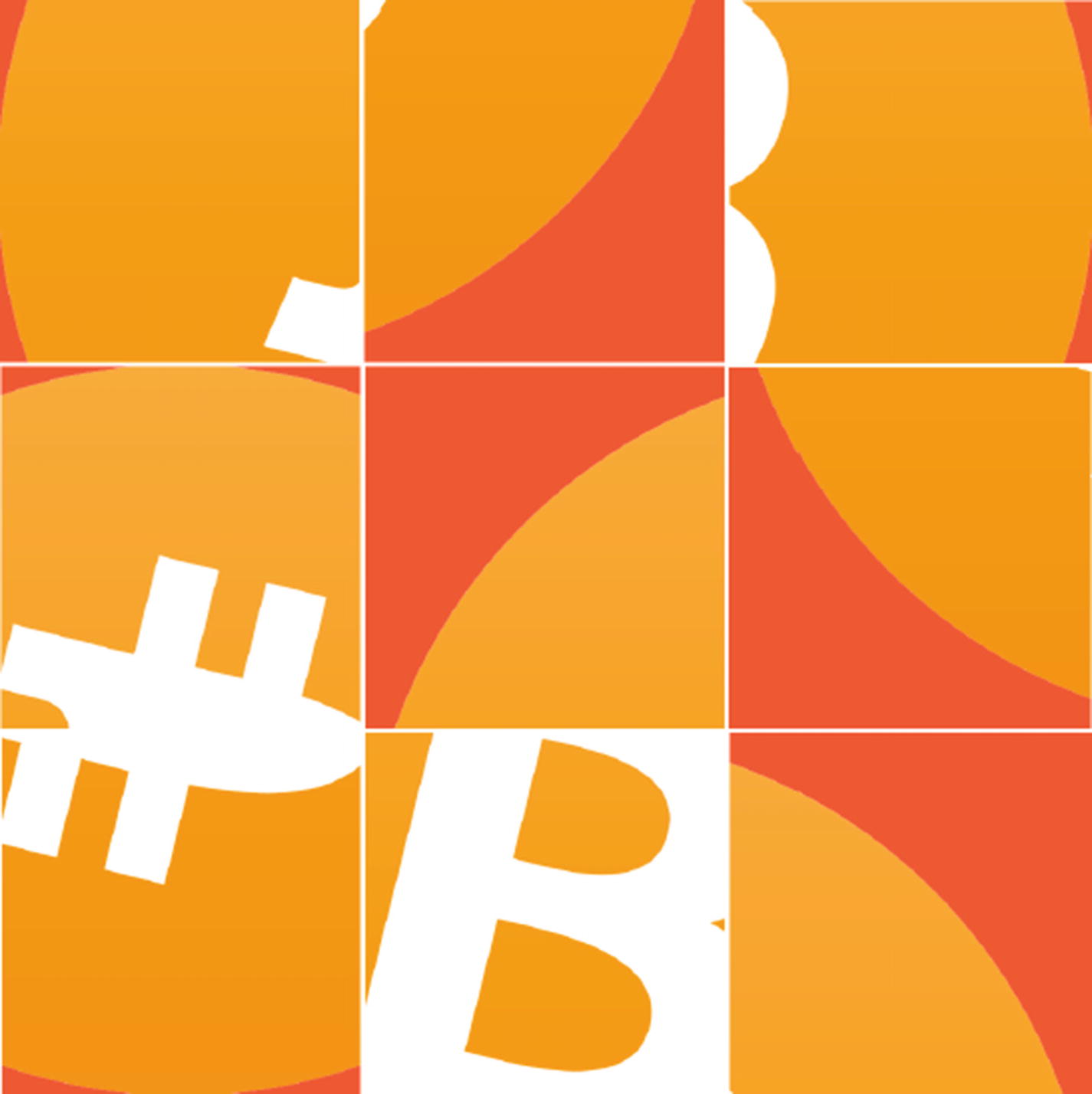

And you could now show this image to someone, and they would know beyond a doubt that work was done.
Implementing Proof of Work
Let’s implement a similar algorithm for our blockchain. Our rule will be similar to the preceding example:
Find a number p that when hashed with the previous block’s solution, a hash with four leading 0s is produced.
We’ve also added a new field to our block structure called nonce, which means a nonsense string. Think of a nonce as a once-off random number, which will be used as an important source of randomness for our blocks. For these nonces, we’ll generate a random 64-bit hex number using Python’s random module:
- 1.
We create a new block (which contains a random nonce).
- 2.
Then hash the block to see if it’s valid.
- 3.
If it’s valid, then we return it; else we repeat from 1. forever.
Delete the print statement inside the new_block() method, or you’ll land up with a lot of unwanted output in your console when mining.
On line 33, inside the new_block() method, delete the line self.chain.append(block) which adds the new block to the chain (we don’t want unvalidated blocks) being added.
To adjust the difficulty of our mining, we could modify the number of leading zeros. But four is sufficient. You’ll find out that the addition of a single leading zero makes an exponential difference to the time required to find a solution. In Bitcoin, the difficulty is adjusted every 2016 blocks by consensus on the network. The difficulty is made harder to stave off the inevitable acceleration of hardware.
Monetary Supply
Now you have a fully functioning blockchain class that implements mining to create new blocks. This is extremely close to how mining happens in production blockchains like Bitcoin. It’s the mining algorithm which generates new Bitcoin: a miner, when completing the mining of a block inserts a transaction from nobody to himself with an amount of Bitcoin that halves every 210,000 blocks. This is how new Bitcoins come into existence.