This chapter offers a quick overview of how PHP works and gives you the basic rules. It’s aimed primarily at readers who have no previous experience of PHP or coding. Even if you’ve worked with PHP before, check the main headings to see what this chapter contains and brush up your knowledge on any aspects that you’re a bit hazy about.
Understanding how PHP is structured
Embedding PHP in a web page
Storing data in variables and arrays
Getting PHP to make decisions
Looping through repetitive tasks
Using functions for preset tasks
Displaying PHP output
Understanding PHP error messages
PHP: The Big Picture
At first glance, PHP code can look quite intimidating, but once you understand the basics, you’ll discover that the structure is remarkably simple. If you have worked with any other computer language, such as JavaScript or jQuery, you’ll find they have a lot in common.
The correct filename extension, usually .php
PHP tags surrounding each block of PHP code (the closing PHP tag is optional if the file contains only PHP code)
Variables to act as placeholders for unknown or changing values
Arrays to hold multiple values
Conditional statements to make decisions
Loops to perform repetitive tasks
Functions or objects to perform preset tasks
Let’s take a quick look at each of these in turn, starting with the filename and the opening and closing tags.
Telling the server to process PHP
Give every page a PHP filename extension; the default is .php. Use a different extension only if you are specifically told to do so by your hosting company.
Identify all PHP code with PHP tags.
You may come across <? as an alternative short version of the opening tag. However, <? isn’t enabled on all servers. Stick with <?php, which is guaranteed to work.
When a file contains only PHP code, it’s strongly recommended to omit the closing PHP tag. This avoids potential problems when working with include files (see Chapter 5).
Note
To save space, most examples in this book omit the PHP tags. You must always use them when writing your own scripts or embedding PHP into a web page.
Embedding PHP in a Web Page
PHP is an embedded language . This means that you can insert blocks of PHP code inside ordinary web pages. When somebody visits your site and requests a PHP page, the server sends it to the PHP engine, which reads the page from top to bottom looking for PHP tags. HTML and JavaScript pass through untouched, but whenever the PHP engine encounters a <?php tag, it starts processing your code and continues until it reaches the closing ?> tag (or the end of the script if nothing follows the PHP code). If the PHP produces any output, it’s inserted at that point.
Tip
A page can have multiple PHP code blocks, but they cannot be nested inside each other.
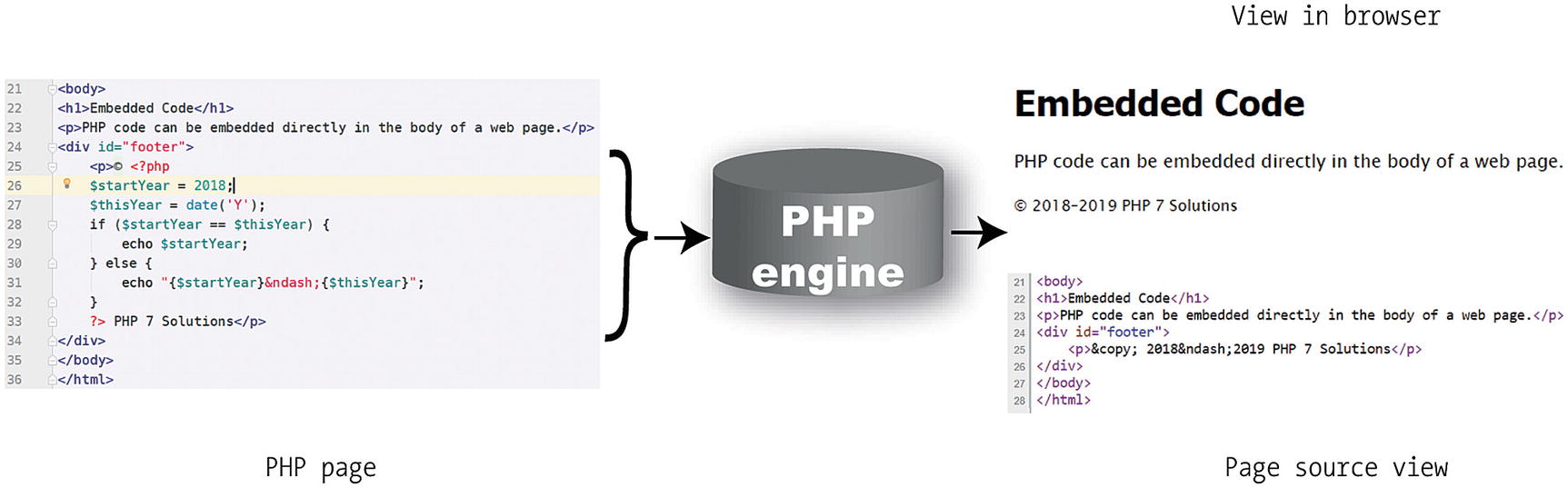
The PHP code remains on the server; only the output is sent to the browser
Tip
PHP doesn’t always produce direct output for the browser. It may, for instance, check the contents of form input before sending an email message or inserting information into a database. Therefore, some code blocks are placed above or below the main HTML code, or in external files. Code that produces direct output, however, goes where you want the output to be displayed.
Storing PHP in an External File
As well as embedding PHP in HTML, it’s common practice to store frequently used code in separate files. When a file contains only PHP code, the opening <?php tag is mandatory, but the closing ?> tag is optional. In fact, the recommended practice is to leave out the closing PHP tag. However, you must use the closing ?> tag if the external file contains HTML after the PHP code.
Using Variables to Represent Changing Values
The code in Figure 3-1 probably looks like a very long-winded way to display a range of years. But the PHP solution saves you time in the long run. Instead of your needing to update the copyright statement every year, the PHP code does it automatically. You write the code once and forget it. What’s more, as you’ll see in Chapter 5, if you store the code in an external file, any changes to the external file are reflected on every page of your site.
This ability to display the year automatically relies on two key aspects of PHP: variables and functions . As the name suggests, functions do things; they perform preset tasks, such as getting the current date and converting it into human-readable form. I’ll cover functions a little later, so let’s work on variables first. The script in Figure 3-1 contains two variables: $startYear and $thisYear.
Tip
A variable is simply a name that you give to something that may change or that you don’t know in advance. Variables in PHP always begin with $ (a dollar sign).
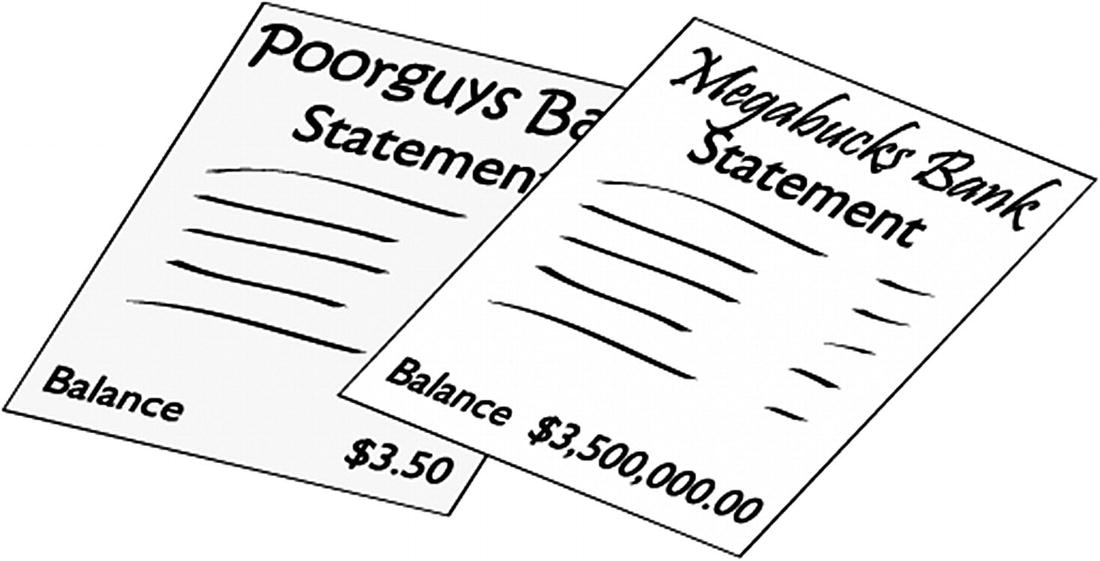
The balance on your bank statement is an everyday example of a variable—the name stays the same, even though the value may change from day to day
Simple.
Naming variables
Variables always begin with a dollar sign ($).
Valid characters are letters, numbers, and the underscore.
The first character after the dollar sign must be a letter or the underscore(_).
No spaces or punctuation marks are allowed, except for the underscore.
Variable names are case-sensitive: $startYear and $startyear are not the same.
When naming variables, choose something that tells you what it’s for. The variables you’ve seen so far—$startYear, $thisYear, $name, and $balance—are good examples. It’s a good idea to capitalize the first letter of the second or subsequent words when combining them (sometimes called camel case). Alternatively, you can use an underscore ($start_year, $this_year, etc.).
Tip
Accented characters commonly used in Western European languages are valid in variables. For example, $prénom and $förnamn are acceptable. In practice, you can also use other alphabets, such as Cyrillic, and nonalphabetic scripts, such as Japanese kanji, in variable names in PHP 7; but at the time of this writing, such use is undocumented, so I recommend sticking to the preceding rules.
Don’t try to save time by using really short variables. Using $sy, $ty, $n, and $b instead of the more descriptive ones makes code harder to understand—and that makes it hard to write. More important, it makes errors more difficult to spot. As always, there are exceptions to a rule. By convention, $i, $j, and $k are frequently used to keep count of the number of times a loop has run, and $e and $t are used in error checking. You’ll see examples of these later in this chapter.
Caution
Although you have considerable freedom in the choice of variable names, you can’t use $this, because it has a special meaning in PHP object-oriented programming. It’s also advisable to avoid using any of the keywords listed at https://secure.php.net/manual/en/reserved.php .
Assigning values to variables
User input through online forms
A database
An external source, such as a news feed or XML file
The result of a calculation
Direct inclusion in the PHP code
The variable goes on the left of the equal sign, and the value goes on the right. Because it assigns a value, the equal sign is called the assignment operator.
Caution
Familiarity with the equal sign from childhood makes it difficult to get out of the habit of thinking that it means “is equal to.” However, PHP uses two equal signs (==) to signify equality. This is a major cause of beginner mistakes, and it sometimes catches more experienced developers, too. The difference between = and == is covered in more detail later in this chapter.
Ending commands with a semicolon
As with all rules, there is an exception: you can omit the semicolon after the last statement in a code block. However, don’t do it except when using the short echo tag as described later in this chapter. Unlike JavaScript, PHP won’t assume there should be a semicolon at the end of a line if you leave it out. This has a nice side effect: you can spread long statements over several lines for ease of reading. PHP, like HTML, ignores whitespace in code. Instead, it relies on semicolons to indicate where one command ends and the next one begins.
Tip
A missing semicolon will bring your script to a grinding halt.
Commenting scripts
To insert a reminder of what the script does
To insert a placeholder for code to be added later
To disable a section of code temporarily
When a script is fresh in your mind, it may seem unnecessary to insert anything that isn’t going to be processed. However, if you need to revise the script several months later, you’ll find comments much easier to read than trying to follow the code on its own. Comments are also vital when you’re working in a team. They help your colleagues understand what the code is intended to do.
During testing, it’s often useful to prevent a line of code, or even a whole section, from running. PHP ignores anything marked as a comment, so this is a useful way of turning on and off code.
There are three ways of adding comments: two for single-line comments and one for comments that stretch over several lines.
Single-line comments
Comments aren’t PHP statements, so they don’t end with a semicolon. But don’t forget the semicolon at the end of a PHP statement that’s on the same line as a comment.
Multiline comments
Multiline comments are particularly useful when testing or troubleshooting, as they can be used to disable long sections of script without the need to delete them.
Tip
Good comments and well-chosen variable names make code easier to understand and maintain.
Using arrays to Store Multiple Values
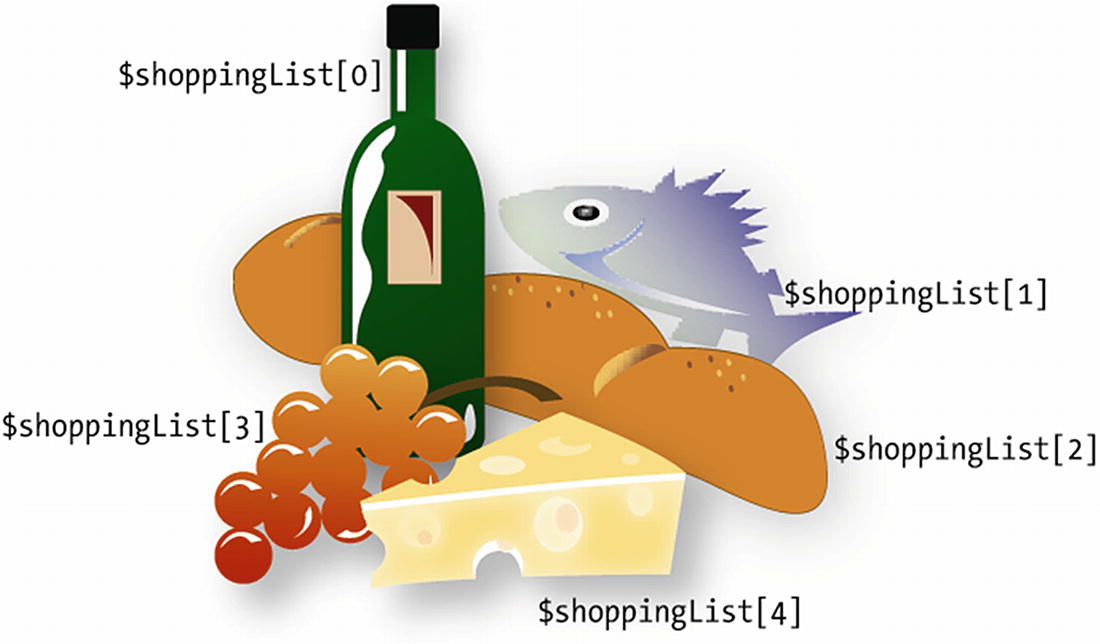
Arrays are variables that store multiple items, just like a shopping list
Individual items—or array elements —are identified by means of a number in square brackets immediately following the variable name. PHP assigns the number automatically, but it’s important to note that the numbering always begins at 0. So the first item in the array, wine in our example, is referred to as $shoppingList[0], not $shoppingList[1]. And although there are five items, the last one (cheese) is $shoppingList[4]. The number is referred to as the array key or index, and this type of array is called an indexed array .
This type of array is called an associative array . Note that the array key is enclosed in quotes (single or double, it doesn’t matter).
Arrays are an important and useful part of PHP. You’ll use them a lot, starting with Chapter 5, when you’ll store details of images in an array to display a random image on a web page. Arrays are also used extensively with databases as you fetch the results of a search in a series of arrays.
Note
You can learn the various ways of creating arrays in Chapter 4.
PHP’s built-in Superglobal Arrays
PHP has several built-in arrays that are automatically populated with useful information. They are called superglobal arrays, and they normally begin with a dollar sign followed by an underscore. The only exception is $GLOBALS, which contains references to all variables in the global scope of the script (see “Variable scope—functions as black boxes” in Chapter 4 for a description of scope).
Two superglobals that you will see frequently are $_POST and $_GET. They contain information passed from forms through the Hypertext Transfer Protocol (HTTP) post and get methods, respectively. The superglobals are all associative arrays, and the keys of $_POST and $_GET are automatically derived from the names of form elements or variables in a query string at the end of a URL.

You can retrieve the values of user input through the $_POST array, which is created automatically when a form is submitted using the post method
You’ll work with the $_POST array in Chapter 6 when you send the content of an online feedback form by email to your inbox. Other superglobal arrays that you’ll use in this book are $_SERVER, to get information from the web server in Chapters 5, 14, and 15, $_FILES to upload files to your web site in Chapter 8, and $_SESSION, to create a simple login system in Chapters 11 and 19.
Caution
Don’t forget that variable names in PHP are case-sensitive. All superglobal array names are written in uppercase. $_Post or $_Get, for example, won’t work.
Understanding When to Use Quotes
Numbers: No quotes
Text: Requires quotes
As a general principle, it doesn’t matter whether you use single or double quotes around text or a string, as text is called in PHP and other computer languages. The situation is a bit more complex, as explained in Chapter 4, because there’s a subtle difference in the way single and double quotes are treated by the PHP engine.
Note
The word “string” is borrowed from computer and mathematical science, where it means a sequence of simple objects—in this case, the characters in text.
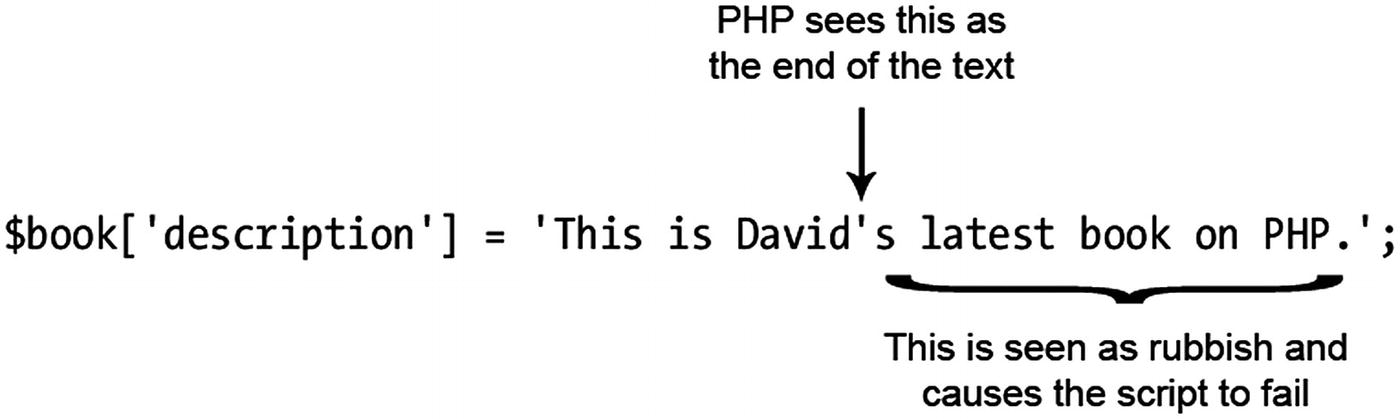
An apostrophe inside a single-quoted string confuses the PHP engine
Use double quotes if the text includes any apostrophes.
Precede apostrophes with a backslash (this is known as escaping).
Single quotes and apostrophes are fine inside a double-quoted string.
Double quotes are fine inside a single-quoted string.
Anything else must be escaped with a backslash.
Tip
Use single quotes most of the time and reserve double quotes for situations where they have a special meaning, as described in Chapter 4.
Special Cases: True, False, and null
Although text should be enclosed in quotes, three keywords—true, false, and null—should never be unless you want to treat them as strings. The first two mean what you expect; null means “nonexistent value.”
Note
True and false are known as Boolean values . They’re named after nineteenth-century mathematician George Boole whose system of logical operations became the basis of much modern-day computing.
This does exactly the opposite of what you might expect: it makes $OK true! Why? Because the quotes around false turn it into a string, and PHP treats strings as true. (There’s a more detailed explanation in “The truth according to PHP” in Chapter 4.)
Don’t enclose them in quotes.
They are case-insensitive.
Making decisions
Decisions, decisions, decisions… Life is full of decisions. So is PHP. They enable it to change the output according to circumstances. Decision-making in PHP uses conditional statements. The most common of these uses if and closely follows the structure of normal language. In real life, you may be faced with the following decision (admittedly not very often in Britain): if the weather’s hot, I’ll go to the beach.
Tip
Conditional statements are control structures and are not followed by a semicolon. The curly braces keep together one or more individual statements that are intended to be executed as a group.
The code inside the curly braces is executed only if the condition is true. If it’s false, PHP ignores everything between the braces and moves on to the next section of code. How PHP determines whether a condition is true or false is described in the following section.
You can use as many elseif clauses in a conditional statement as you like. Only the first condition that equates to true will be executed; all others will be ignored, even if they’re also true. This means you need to build conditional statements in the order of priority that you want them to be evaluated. It’s strictly a first-come, first-served hierarchy.
Note
Although elseif is normally written as one word, you can use else if as separate words.
Making comparisons
Conditional statements are interested in only one thing: whether the condition being tested equates to true. If it’s not true, it must be false. There’s no room for half measures or maybes. Conditions often depend on the comparison of two values. Is this bigger than that? Are they both the same? And so on.
Caution
Don’t use a single equal sign in the first line ($status = 'administrator'). Doing so opens the admin area of your web site to everyone. Why? Because this automatically sets the value of $status to administrator; it doesn’t compare the two values. To compare values, you must use two equal signs. It’s a common mistake, but one with potentially disastrous consequences.
Note
Chapter 4 describes how to test for multiple conditions simultaneously.
Using indenting and whitespace for clarity
Indenting code helps to keep statements in logical groups, making it easier to understand the flow of the script. PHP ignores any whitespace inside code, so you can adopt any style you like. Just be consistent so that you can spot anything that looks out of place.
The style isn’t important. What matters is that your code is consistent and easy to read.
Using loops for Repetitive Tasks
Loops are huge timesavers because they perform the same task over and over again, yet involve very little code. They’re frequently used with arrays and database results. You can step through each item one at a time looking for matches or performing a specific task. Loops are particularly powerful in combination with conditional statements, allowing you to perform operations selectively on a large amount of data in a single sweep. Loops are best understood by working with them in a real situation. Details of all looping structures, together with examples, are in Chapter 4.
Using functions for Preset Tasks
Functions do things … lots of things, mind-bogglingly so in PHP. A typical PHP setup gives you access to several thousand built-in functions. You’ll only ever need to use a handful, but it’s reassuring to know that PHP is a full-featured language.
Note
Chapter 16 covers in depth how PHP handles dates and time.
It doesn’t take a genius to work out that this sends an email to the address stored in the first argument, with the subject line stored in the second argument, and the message stored in the third one. You’ll see how this function works in Chapter 6.
Tip
You’ll often come across the term “parameter” in place of “argument.” Technically speaking, parameter refers to a variable used in the function definition, while argument refers to an actual value passed to the function. In practice, both terms tend to be used interchangeably.
As if all the built-in functions weren’t enough, PHP lets you build your own custom functions, as described in the next chapter. Even if you don’t relish the idea of creating your own functions, throughout this book you’ll use some that I have made. You use them the same way as built-in functions.
Displaying PHP output
There’s not much point in all this wizardry going on behind the scenes unless you can display the results in your web page. There are two ways of doing this in PHP: using echo or print. There are some subtle differences between the two, but they are so subtle you can regard echo and print as identical. I prefer echo for the simple reason that it’s one fewer letter to type.
When using echo or print with a variable, the variable must contain only a single value. You cannot use them to display the contents of an array or of a database result. This is where loops are so useful: you use echo or print inside the loop to display each element individually. You’ll see plenty of examples of this in action throughout the rest of the book.
The parentheses make no difference. Unless you enjoy typing for the sake of it, leave them out.
Using the Short Echo Tag
Because it’s shorthand for echo, no other code can be in the same PHP block, but it’s particularly useful when embedding database results in a web page. It goes without saying that the value of the variable must be set in a previous PHP block before you can use this shortcut.
Tip
Because no other code can be in the same PHP block, it’s common practice to omit the semicolon before the closing PHP tag when using the short echo tag.
Joining Strings Together
As the comment in the final line of code indicates, when two strings are joined like this, PHP leaves no gap between them. Don’t be fooled into thinking that adding a space after the period will do the trick. It won’t. You can put as much space on either side of the period as you like; the result will always be the same, because PHP ignores whitespace in code. In fact, it’s recommended to leave a space on either side of the period for readability.
Tip
The period—or concatenation operator, to give it its correct name—can be difficult to spot among other code. Make sure the font size in your editor is large enough to see the difference between periods and commas.
Working with numbers
PHP can do a lot with numbers, from simple addition to complex math. The next chapter contains details of the arithmetic operators you can use with PHP. All you need to remember at this stage is that numbers must not contain any punctuation other than a decimal point. PHP will choke if you feed it numbers that contain commas (or anything else) as the thousands separator.
Understanding PHP error messages
Error messages are an unfortunate fact of life, so you need to understand what they’re trying to tell you. The following illustration shows a typical error message.

PHP error messages report the line where PHP discovered a problem. Most newcomers—quite naturally—assume that’s where to look for their mistake. Wrong…
Most of the time, PHP is telling you that something unexpected has happened. In other words, the mistake lies before that point. The preceding error message means that PHP discovered an echo command where there shouldn’t have been one. (Error messages always prefix PHP elements with T_, which stands for token.)
Instead of worrying what might be wrong with the echo command (probably nothing), start working backward, looking for anything missing, probably a semicolon or closing quote on a previous line.
Sometimes, the message reports the error on the last line of the script. That always means you have omitted a closing curly brace somewhere further up the page.
Fatal error: Any HTML output preceding the error will be displayed, but once the error is encountered—as the name suggests—everything else is killed stone dead. A fatal error is often caused by referring to a nonexistent file or function.
Parse error: This means there’s a mistake in your code syntax, such as mismatched quotes or a missing semicolon or closing brace. It stops the script in its tracks, and it doesn’t even allow any HTML output to be displayed.
Warning: A warning indicates a serious problem, such as a missing include file. (Include files are the subject of Chapter 5.) However, the error is usually not serious enough to prevent the rest of the script from being executed.
Deprecated: This warns you about features that are scheduled to be removed from a future version of PHP. If you see this type of error message, you should seriously consider updating your script, as it could suddenly stop working if your server is upgraded.
Strict: This type of error message warns you about using techniques that are not considered good practice.
Notice: This advises you about relatively minor issues, such as the use of a nondeclared variable. Although this type of error won’t stop your page from displaying (and you can turn off the display of notices), you should always try to eliminate them. Any error is a threat to your output.
Why is My Page Blank?
Many beginners are left scratching their heads when they load a PHP page into a browser and see absolutely nothing. There’s no error message, just a blank page. This happens when there’s a parse error—in other words, a mistake in the code—and the display_errors directive in php.ini is turned off.
If you followed the advice in the previous chapter, display_errors should be enabled in your local testing environment. However, most hosting companies turn off display_errors. This is good for security, but it can make it difficult to troubleshoot problems on your remote server. As well as parse errors, a missing include file often causes blank pages.
Put this code on the first line after the opening PHP tag, or in a separate PHP block at the top of the page if the PHP is lower down the page. When you upload the page and refresh the browser, you should see any error messages generated by PHP.
If you still see a blank page after adding this line of code, it means there’s an error in your syntax. Test the page locally with display_errors turned on to find out what’s causing the problem.
Caution
After correcting the error, remove the code that turns on the display of errors. If something else breaks in the script at a later stage, you don’t want to expose potential vulnerabilities on your live web site.
PHP Quick Checklist
Always give PHP pages the correct filename extension, normally .php.
Enclose PHP code between the correct tags: <?php and ?>.
Avoid the short form of the opening tag: <?. Using <?php is more reliable.
Omit the closing PHP tag in files that contain only PHP code.
PHP variables begin with $ followed by a letter or the underscore character.
Choose meaningful variable names and remember they’re case-sensitive.
Use comments to remind you what your script does.
Numbers don’t require quotes, but strings (text) do.
The decimal point is the only punctuation allowed in numbers.
You can use either single or double quotes around strings, but the outer pair must match.
Use a backslash to escape quotes of the same type inside a string.
To store related items together, use an array.
Use conditional statements, such as if and if . . . else, for decision-making.
Loops simplify repetitive tasks.
Functions perform preset tasks.
Display PHP output with echo or print.
With most error messages, work backward from the position indicated.
Keep smiling—and remember that PHP is not difficult.
The next chapter fills in essential details that you can refer to as you progress through this book.