In a cryptocurrency network such as Bitcoin or Helium, the fundamental unit of interaction is a transaction where value is transferred from one entity to another. In this type of network, the blockchain is an immutable, distributed ledger of all of the transactions that have transpired on the network. Value is transferred from one public address to another public address. We can examine and enumerate all of the transactions associated with a public address by scanning the entire blockchain for transactions that involve this address. In fact, if we scan the blockchain forward from the genesis block, then the last transaction involving this address also provides the cryptocurrency balance which is associated with this address.
The type of cryptocurrency blockchain that we have just described has no concept of a user account. The blockchain does not provide each transactor with a unique account wherein all of the account activity is recorded. Rather, the application only records transactions on the blockchain as they occur. Each transaction is comprised of one or more origin public addresses from which value is transferred to one or more destination public addresses. Thus, since an individual uses a public address to receive value and transfer away value, the transactions that involve an individual can be constructed by scanning the blockchain from the genesis block forward for transactions involving the public addresses that the person owns. This is precisely how a user’s wallet is constructed. It should be mentioned that it is considered bad practice to reuse public addresses since it is essentially a zero-cost endeavor to create them offline as well as online. Furthermore, using multiple addresses obscures identity to some extent.
One thing to notice about cryptocurrency networks like Bitcoin and Helium is that the contractual relations that transfer value reside outside the network. The network does not record contractual relations. This is in contrast to Ethereum, which can encode contractual terms on the network.
This chapter and the next two chapters examine cryptocurrency transactions. In this chapter, we are going to delve into cryptocurrency transactions, the validation of such transactions, and, in particular, how value is transferred securely from the hands of its rightful owner to an intended recipient. In the next chapter, we will examine merkle trees and how they can be used to validate the transactions in a block. In Chapter 11, we will write code for Helium transaction processing.
Public Address Construction
In a typical transaction, the receiver of value provides the transferor of value with a public address to which value is to be sent. Using cryptographic techniques, the receiver of value can prove that he or she generated the public address and is therefore the owner of the value that has been transferred to this address.
Henceforth and for the sake of brevity, I shall refer to the hexadecimal string-encoded output of a cryptographic hash function such as SHA-256 or RIPEMD-160 simply as a message digest or a hash.
- 1.
Generate a random private-public pair of ECC keys.1
- 2.
Generate the SHA-256 message digest of the public key.
- 3.
Generate the RIPEMD-160 message digest of the result in Step 2.
- 4.
Add a version byte, 0x1, in front of the RIPEMD-160 hash.
- 5.
Compute the SHA-256 message digest of the result in Step 4.
- 6.
Extract the last four bytes of the result in Step 5. This will be our error detection checksum.
- 7.
Concatenate the error checksum with the result of Step 4.
- 8.
Base58 encode the result in Step 7. The result is a public address.2
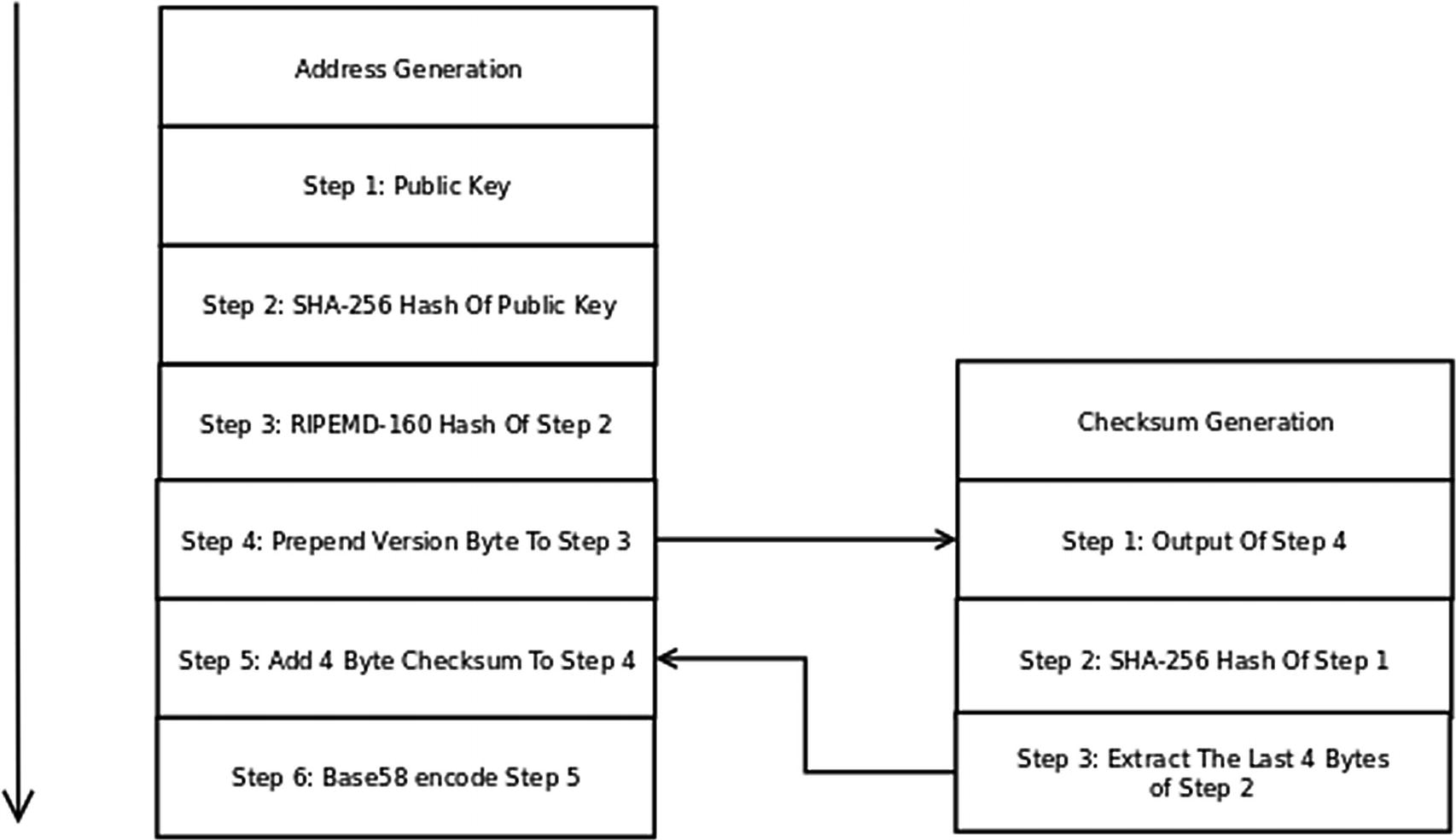
Public Address Generation
The version byte of Helium addresses will be fixed to the value in the hconfig module. The last four bytes prior to the Base58 encoding are a checksum that enables us to prove that the address is not corrupted.
- 1.
Base58 decode the public address.
- 2.
Strip the last four bytes from the result of the previous step. These four bytes are the error checksum. We will call this the extracted checksum. The remaining portion is the prepended version byte and the RIPEMD-160 hash. We will call this portion the RIPEMD hash.
- 3.
Take the SHA-256 hash of the RIPEMD hash and extract the last four bytes. This is our computed checksum.
- 4.
The public address is invalid if the computed checksum is not equal to the extracted checksum.
Note that due to the random generation of the public key and the cryptographic properties of hashes, a public key will always generate a corresponding unique public address, a fortiori, the address is generated by some unique private-public key pair.3
Canonical Transaction Structure
The text that is enclosed in angle brackets specifies the type of the value corresponding to the key.
The value of the version key describes the version of the application software that is used to process the transaction. For Helium, this is fixed at “1”.
The transactionid is a universally unique identifier for the transaction. We will use the secrets module in the Python standard library to create a random and secure cryptographic hexadecimal-encoded string that is 512 bits long.4
locktime is a non-negative integer. This value, if greater than zero, instructs a miner to refrain from processing the transaction until a specified amount of time has elapsed. Elapsed time is measured in seconds. A miner who caches the transaction for future processing does not include the transaction in a block until the requisite time period has transpired. locktime cannot have a value in excess of the MAX_LOCKTIME specified in the Helium configuration module.
The Transaction vin List
We are now in a position to discuss the vin list and unlocking previous transaction amounts. vin is an acronym for value in. vin is a list of transaction inputs that consume the outputs of previous transactions.
txid is the transactionid of a previous transaction, a portion of whose output is being consumed.
vout_index is an index into the previous transaction’s vout array. Each vout element of a previous transaction specifies a value that can be consumed (transferred). The total value consumed is a summation of values transferred by all of the vin elements. Take note that the entire output in the vout element of the previous transaction must be consumed.
The ScriptSig script enables the transfer of value by proving ownership of the value. It is the concatenation of a digital signature and a public key. The public key is the key that was used to generate the public address to which value was transferred in the previous transaction. We will look at this script in short order.
The Transaction vout List
vout is an acronym for value out. Recall that when a public address receives value pursuant to a transaction, then this value can be subsequently spent in another transaction by its owner. A transaction’s vout list specifies the values that are transferrable to one or more receivers of value. To reiterate, the vout list specifies transaction values that are available to be spent in subsequent transactions by the owners of such values.
value is the value of the cryptocurrency that is received by a specific public address. The string RIPEMD-160(SHA-160(Public Key)) is deconstructed from this public address;5 this deconstructed string is in ScriptPubKey. ScriptPubKey is a list of symbolic unlocking operations, to be discussed subsequently. The cryptocurrency value in a vout element can be spent by the entity who can unlock the ScriptPubKey script. The only entity that can unlock the script is the entity that possesses the private key pertaining to RIPEMD-160(SHA-160(Public Key)). I will show this subsequently.
There are two aspects of vout to notice. Firstly, only integer amounts can be transferred. In the Bitcoin realm, the smallest transferable unit is one satoshi, or a hundred millionth of a bitcoin. In Helium, the smallest transferable unit is a helium cent, which is also a hundred millionth of a helium. By handling only integer arithmetic, we avoid all of the messy complications involved in decimal and floating-point arithmetic. Secondly, observe that the sum of values over the entire vout list is the total value received by public addresses that have been provided by the recipients of the transaction. Ordinarily, this amount will be slightly less than the amount transferred into this transaction. The difference is the transaction fee.
Transaction Mechanics
In Newtonian mechanics, the fundamental unit of computation is a body which has mass. Bodies have well-defined properties, and there are physical laws that govern their behavior. Analogously, the fundamental unit of computation in a cryptocurrency network is the transaction. Without transactions, there is no blockchain. Transactions have a rigid and well-defined structure, as well as fixed rules that govern their behavior.
Let us go through all of the steps that are involved in a typical transaction. Suppose that Alice wants to transfer one helium to Smith. The task before Alice is to create a transaction object (the previous dictionary structure) that enables this transfer. This transaction object must (i) unlock some of the previous heliums that Alice owns and (ii) lock the output helium values so that only Smith can unlock and use them. Unlocking the heliums that Alice owns means that she has proven her ownership of these heliums, and hence, they can be transferred away by her. Once these heliums are unlocked, they become outputs that can be delivered to Smith. Alice locks these outputs so that only Smith can unlock them. This will prove that Smith owns these Heliums.
- 1.
Alice requests Smith to send her a public address to which she can send one bitcoin.
- 2.
Smith sends Alice a public address that he has created.
- 3.Alice creates a transaction id for her transaction. We suppose that this id is"d6b77f077ff700cd36df061ed75af353506f0cec6e267f0ce674eaa3b5d53217"
- 4.
When Alice received heliums in the previous transaction “618...9e1”, she had provided a public address to the transferor. Alice now gets the public key (say, pPubKey) with which this address was created and the private key (say, PrivKey) paired with this public key. Alice extracts the following value, which is available in the previous transaction’s output (vout element):
- 5.Using this information, Alice creates the vin element in the transaction’s vin list. This list contains exactly one element. The vout_index element is the index into the vout list of the previous transaction, and it identifies the output of the previous transaction that is being consumed (let us assume it is 0). The vin element is{"txid": "618a125ac95ba370187c574d174eb7d618f3ee35d374899ca0007a035ba0a9e1""vout_index": 0"ScriptSig": [Sig, PublicKey]}
- 6.
Alice next takes the public address provided by Smith and reverses the Base58 encoding and strips off the checksum. The result is
- 7.Alice’s vout element is{"value": 1,"ScriptPubKey": "<DUP> <HASH-160> MDHASH <EQ_VERIFY> <CHECK_SIG>"}
- 8.And Alice has now created the transaction object, which looks like this:{"version": "1""transactionid": "d6b77f077ff700cd36df061ed75af353506f0cec6e267f0ce674eaa3b5d53217""locktime": 0"vin": {"txid": "618a125ac95ba370187c574d174eb7d618f3ee35d374899ca0007a035ba0a9e1""vout_index": 0"ScriptSig": "Sig PublicKey"}"vout": {"value": 1,"ScriptPubKey": [<DUP> <HASH-160> MDHASH <EQ_VERIFY> <CHECK_SIG>]}}
- 9.
Having created the transaction object, Alice serializes it into a Base58 byte sequence and broadcasts it on the Helium peer-to-peer network. Miners who are listening on this network for transactions pick up the transaction and (may) include it in a block that they intend to mine.
Subsequently in this chapter, we will show how the ScriptSig script ensures that only Alice can unlock the previous transaction output of 1.08 heliums and how the ScriptPubKey script ensures that only Smith will be able to unlock the transaction output of one helium. A transaction is only deemed to be valid if both the ScriptSig and the ScriptPubKey succeed without error. Bitcoin and Helium both implement a simple stack processor to verify that these scripts execute successfully.
You will have noticed that we unlocked 1.08 heliums but we transferred only 1 helium to Smith. What has happened to the remainder of 0.08 heliums? This excess amount is called a transaction fee. It is an inducement to miners to include Alice’s transaction in a block that miners intend to mine. If a miner successfully mines a block that contains Alice’s transaction, then the miner can claim these 0.08 heliums.
The preceding logic can be applied, mutatis mutandis, to handle inputs from several previous transactions holding outputs that Alice owns (there will be more than one previous transaction vout list element), and the transaction can direct outputs to several helium addresses (the transaction will have more than one vout element).
The steps that I have described earlier would typically be carried out by Alice’s Helium wallet.
In a typical fiat currency transaction at a cash register, you tender some money and get some change back. Change is handled in the following manner in a cryptocurrency. Suppose that Alice has a previous transaction with 5.08 heliums in it and she intends to use these heliums in her transaction with Smith. Alice would create a public address and direct 4 heliums into this address. Thus, Smith would receive one helium to the address that he gave to Alice, Alice would get change of 4 heliums at the address that she has created, and the transaction fee would be 0.08 heliums. This aspect of a transaction would also be handled transparently by a Helium wallet.
The ScriptPubKey and ScriptSig Scripts
The ScriptPubKey script in a vout element of a transaction is used to lock the value specified in this element. Only the owner of the public address to which this value is transferred can unlock the value.
The ScriptSig script in a vin element of a transaction describes a value in a prior transaction that will be consumed in the present transaction. This value will be transferred to one or more public addresses. The consumed value can be identified completely from the transaction id of the previous transaction and the index of the vout element in this transaction. The ScriptSig script ensures that this value in the prior transaction can only be transferred by the entity that owns the value.
Notice that each vin element in a transaction references exactly one vout element in a previous transaction and such a vin element must consume the entirety of the value in this vout element.
The ScriptPubKey and ScriptSig scripts are both simple lists. They are processed by a simple stack language which I will now describe.
An element (list element) in these scripts is either an operator symbol or an operand. We will call an operator symbol an opcode. An operand is a value; for example, the integer 5 and the string “hello world” are both operands. Opcodes are symbolic operations that perform operations on operands. For example, the addition operator + is an opcode that operates on numbers. Each list element contains exactly one opcode or one operand. Opcodes are designated between angle brackets.
An execution stack is a list of opcodes and operands. For example, ScriptPubKey and ScriptSig are both execution stacks.
Each element of the vin and vout lists is either an opcode or an operand. Each list behaves like a stack, and hence, it permits only two operations on the stack, PUSH and POP. The POP operation pops an element from the head of a stack, and a PUSH operation pushes an element onto the head of a stack.
Finally, we also specify a result stack. An opcode on the execution stack will operate on values on the result stack, and the result of the operation may be be pushed back onto the result stack if the semantics of the opcode requires this. If the value on the execution stack is an operand, it is simply pushed onto the result stack.
Valid opcodes are DUP, HASH-160, PK_HASH, EQ-VERIFY, and CHECK-SIG. The meaning of these opcodes is described as follows. Valid operands must be strings.
This specification describes a simple stack language.
As stated previously, opcodes will be denoted by enclosing them in angle brackets. Operands, which denote values, will not be enclosed in angle brackets. The opcode that is to be executed will be indicated by an execution pointer (a fat arrow).
We are going to examine P2PKHASH (person to public key hash) scripts. These types of scripts transfer value from a single entity to another single entity. P2PKHASH scripts constitute 98% to 99% of all transactions in typical cryptocurrency networks.
ScriptSig Scripts

ScriptSig Structure
A ScriptSig script is composed of two operands. PUBKEY is the public key (hexadecimal string) that was used to create the public address that received the previous transaction output.
This value is also available in the vout element of the previous transaction.
ScriptPubKey Scripts
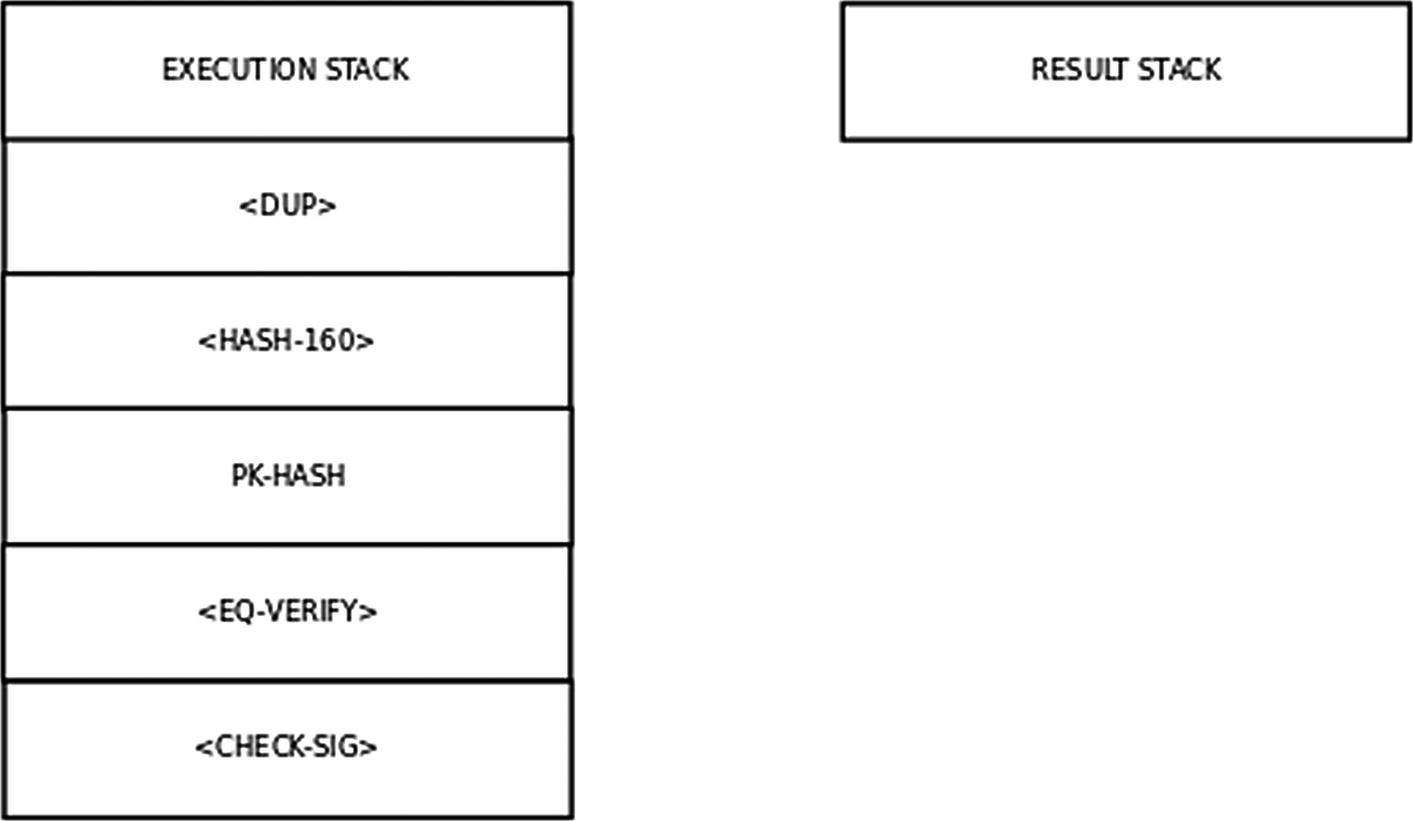
Execution Stack for a ScriptPubKey
This diagram also shows an empty result stack on the right side. This stack will hold operands which will be acted upon by the opcodes in the execution stack.
The meaning of the opcodes in the execution stack are as follows:
<DUP> means taking the value at the head of the result stack and pushing a copy of this value back onto the result stack.
and then pushing the message digest onto the result stack. PK-HASH is a string.
<EQUAL_VERIFY> means popping two elements from the result stack and verifying that they are equal.
<CHECK_SIG> means popping two elements from the result stack and verifying the signature.
ScriptSig and ScriptPubKey Scripts in Action
Let us see how the ScriptSig and ScriptPubKey scripts are used to validate a P2PKHASH transaction. Such a transaction is valid if both of these scripts execute without an error. A valid transaction unlocks previous transaction outputs that are used in the transaction. We will use our previous example where Alice transfers one helium coin to Smith.
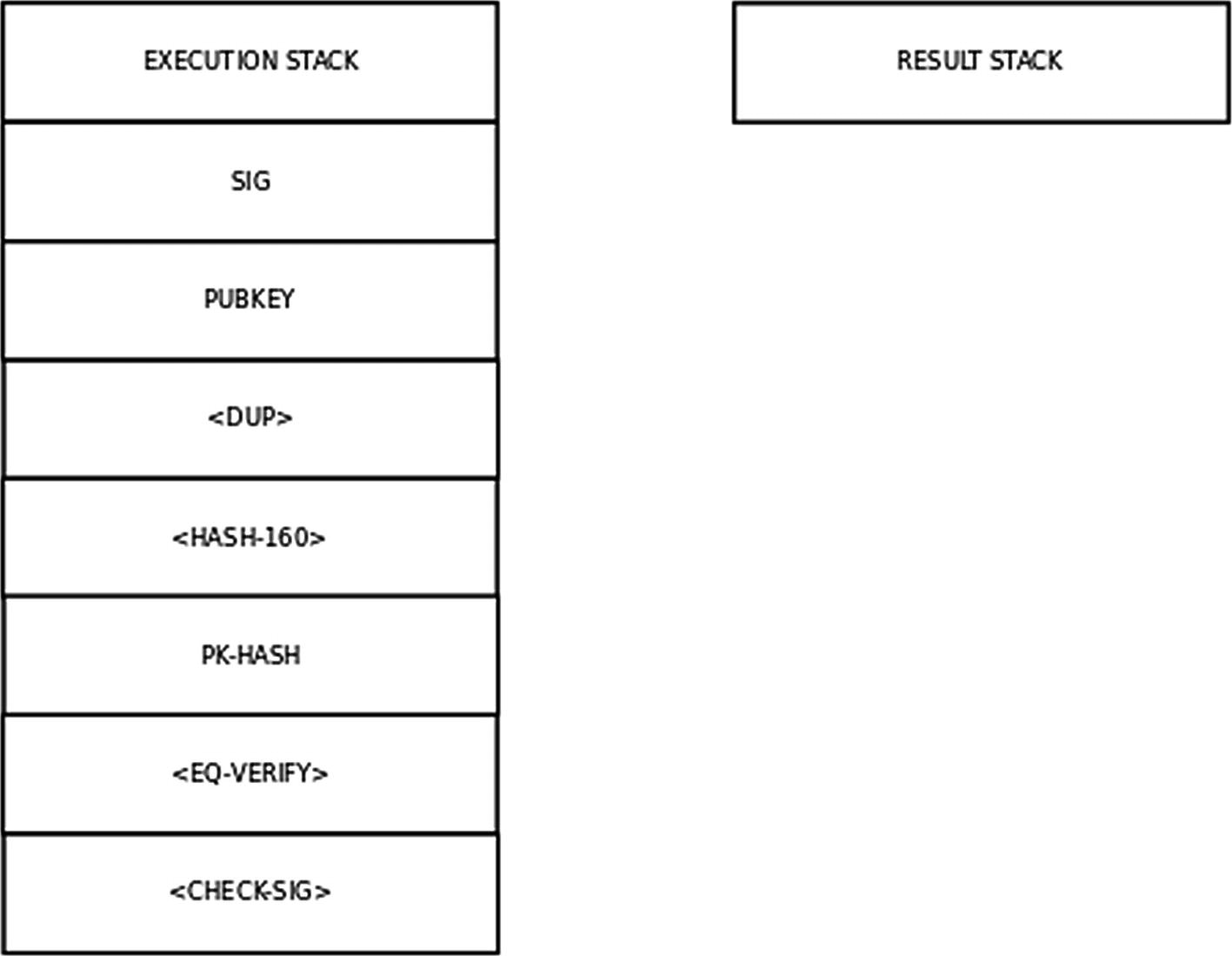
Execution Stack for ScriptSig and ScriptPubKey
We will now execute the opcodes on this stack. Any operands that are present in this stack will simply be pushed onto the result stack.
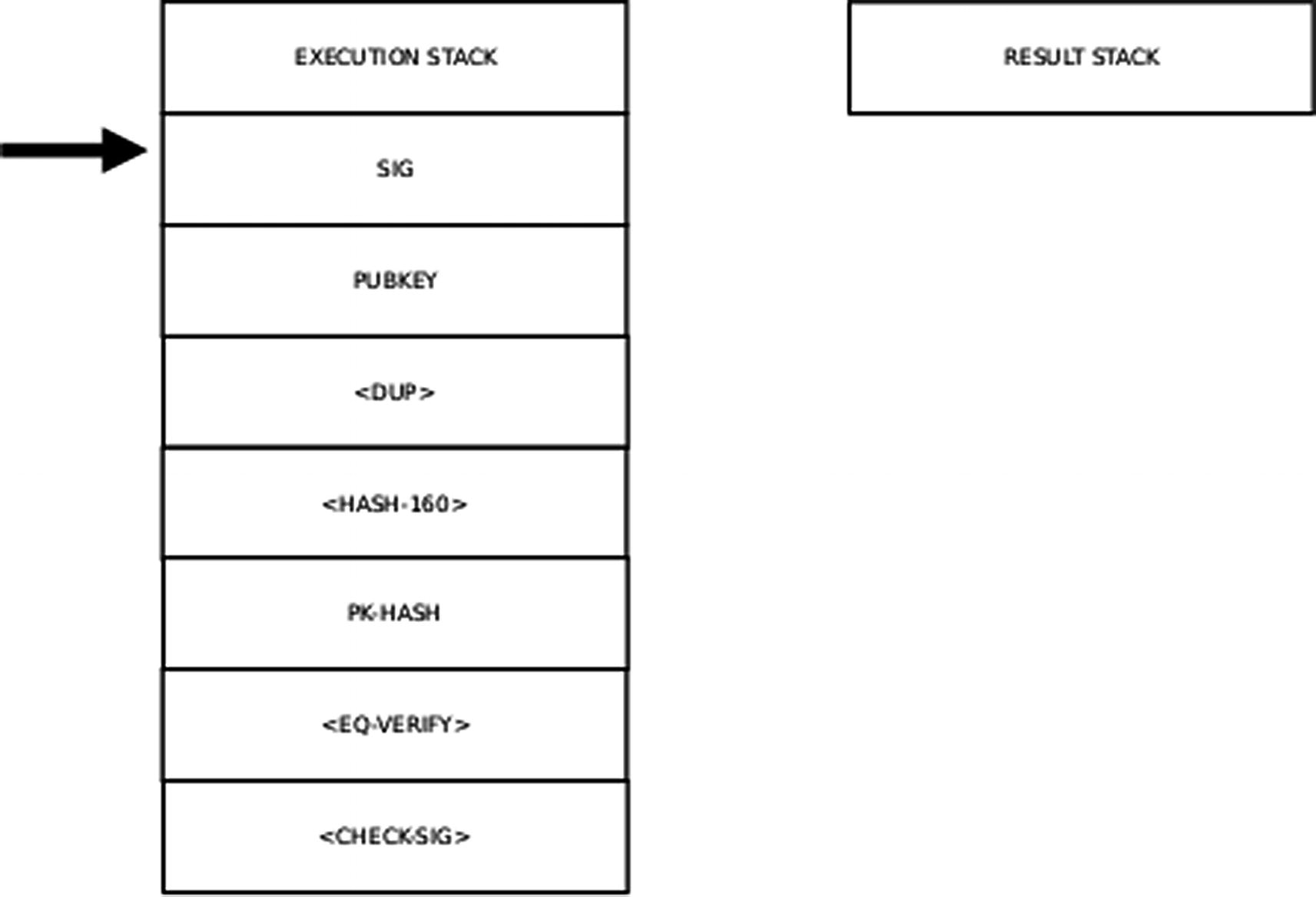
Step 1 of the Execution Stack
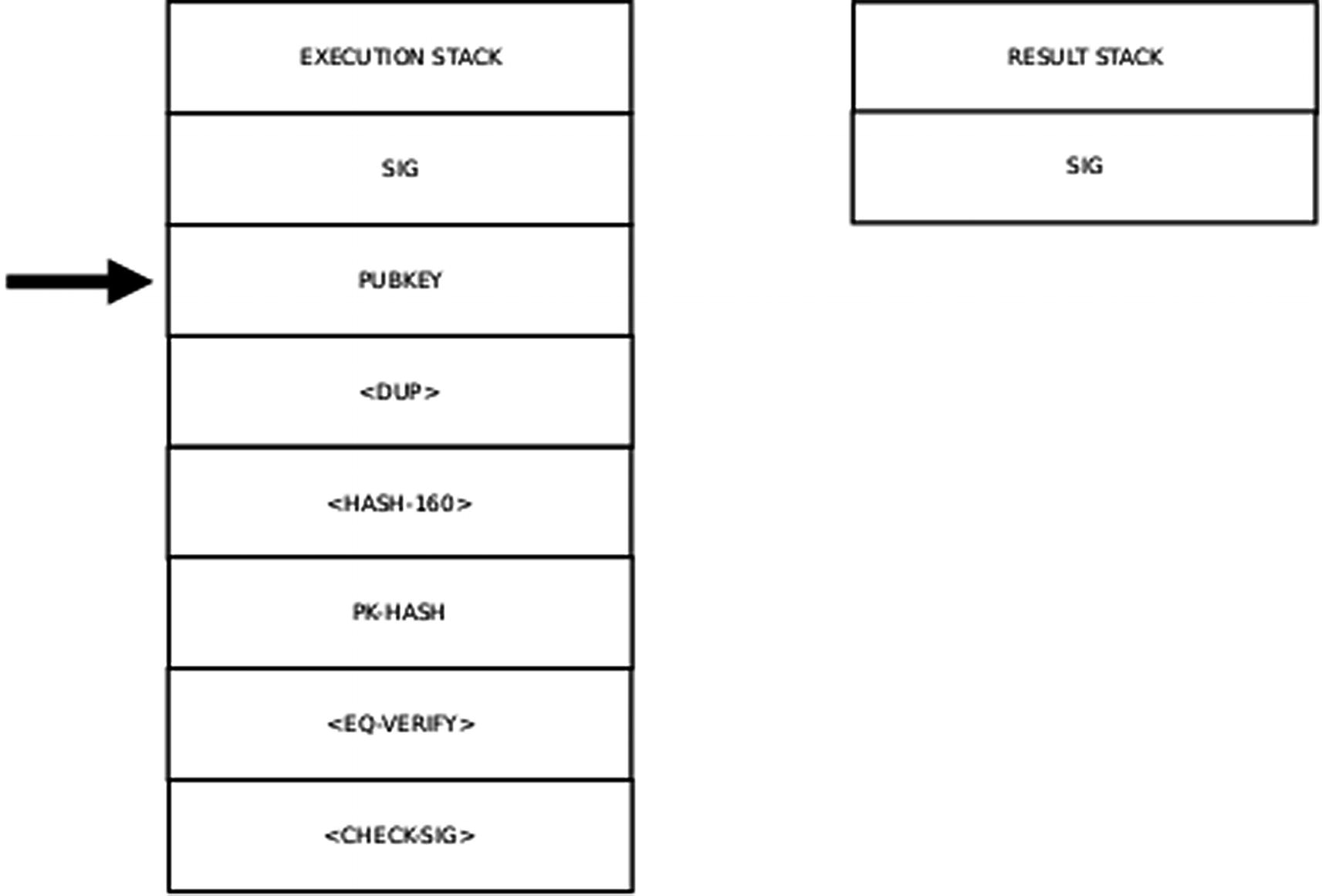
Step 2 of the Execution Stack
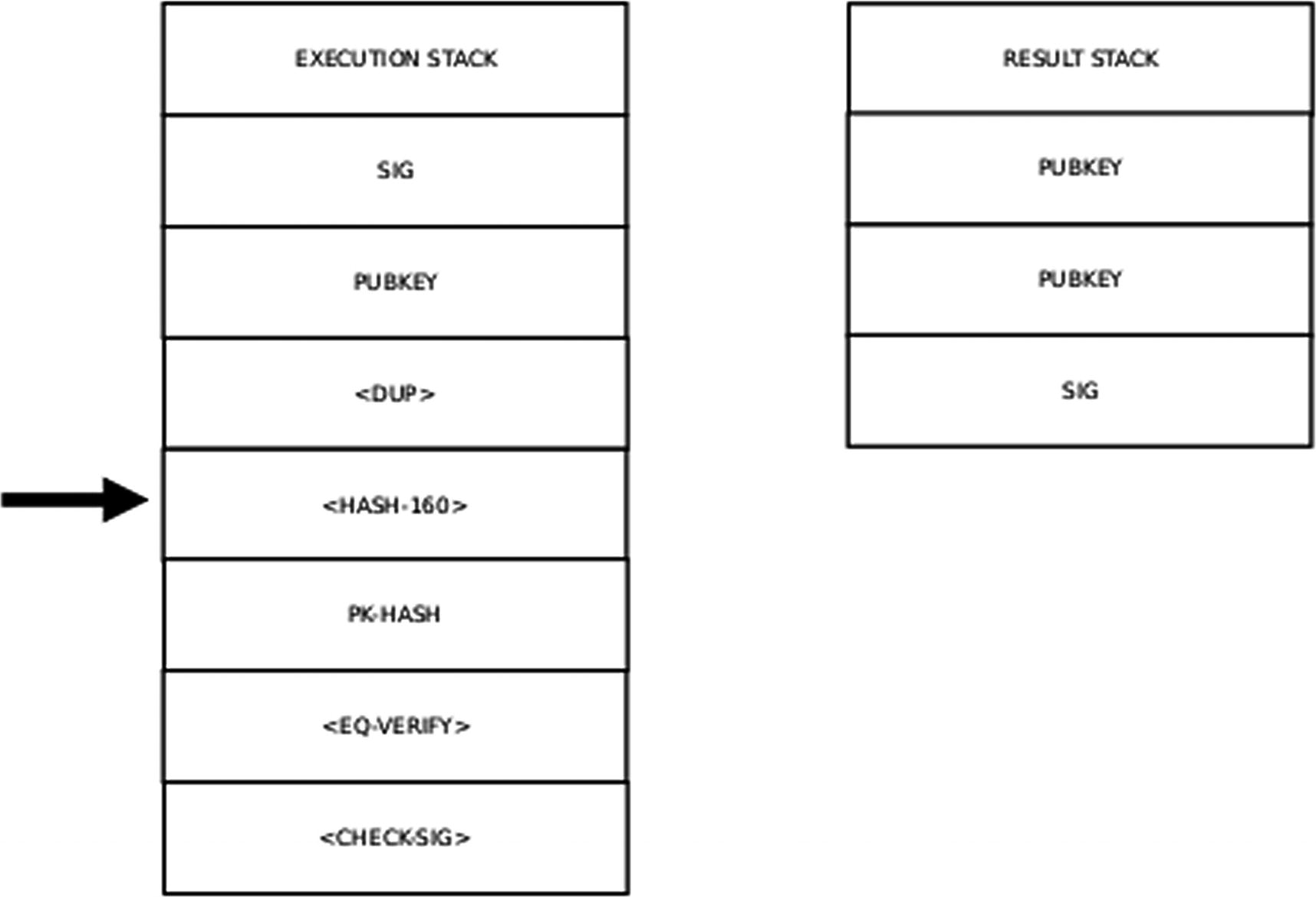
Step 3 of the Execution Stack
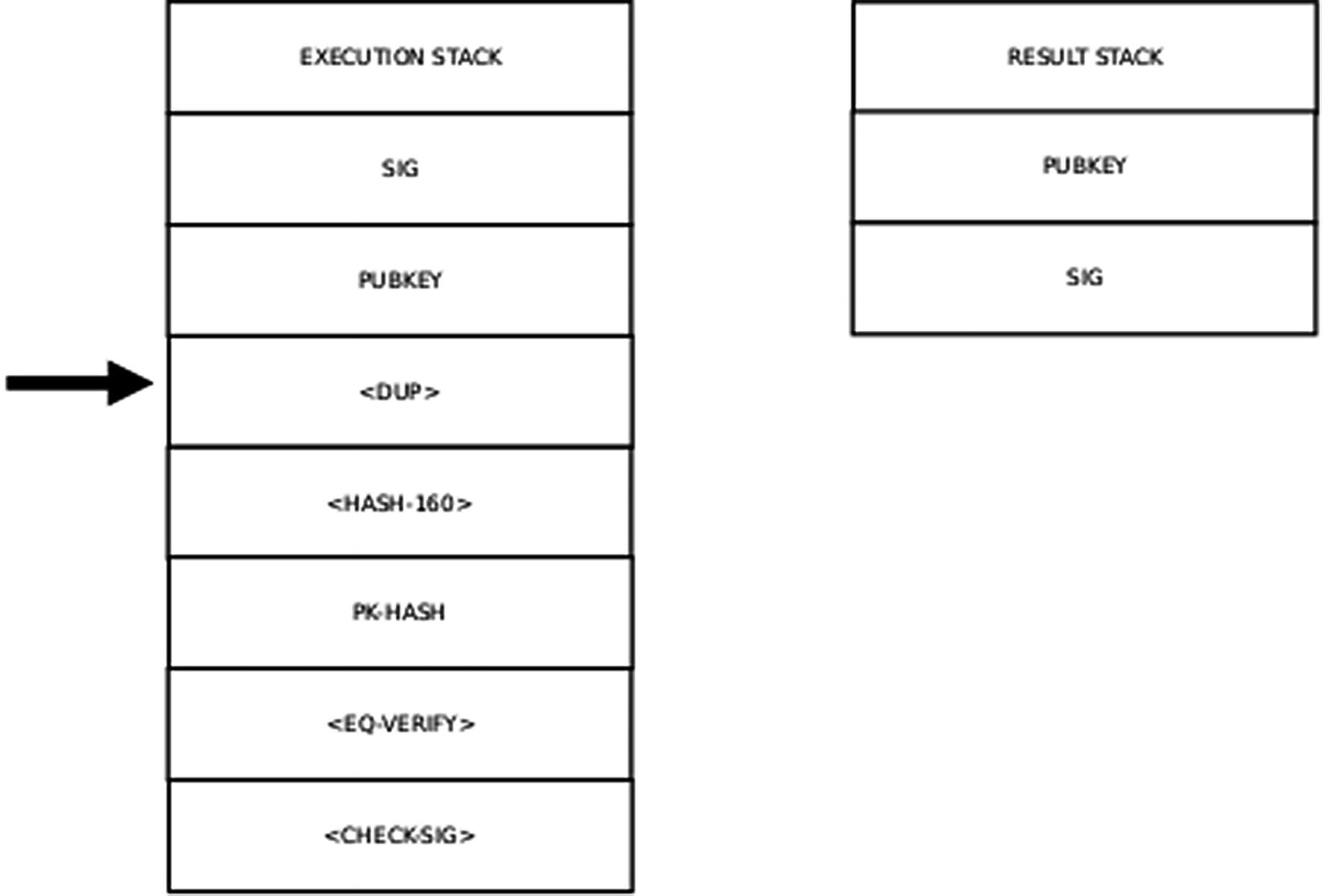
Step 4 of the Execution Stack

Step 9 of the Execution Stack

Step 10 of the Execution Stack
The next command on the opcode stack is <EQUAL_VERIFY>. This opcode requires us to pop the two values at the head of the result stack and compare their values. If the two RIPEMD-160 hashes are not equal, the unlocking of the input fails since the party alleging to own the value in the previous transaction has not provided the correct public key. If Alice uses the public key that she used for her previous transaction input to compute the hash, the equality verification succeeds.
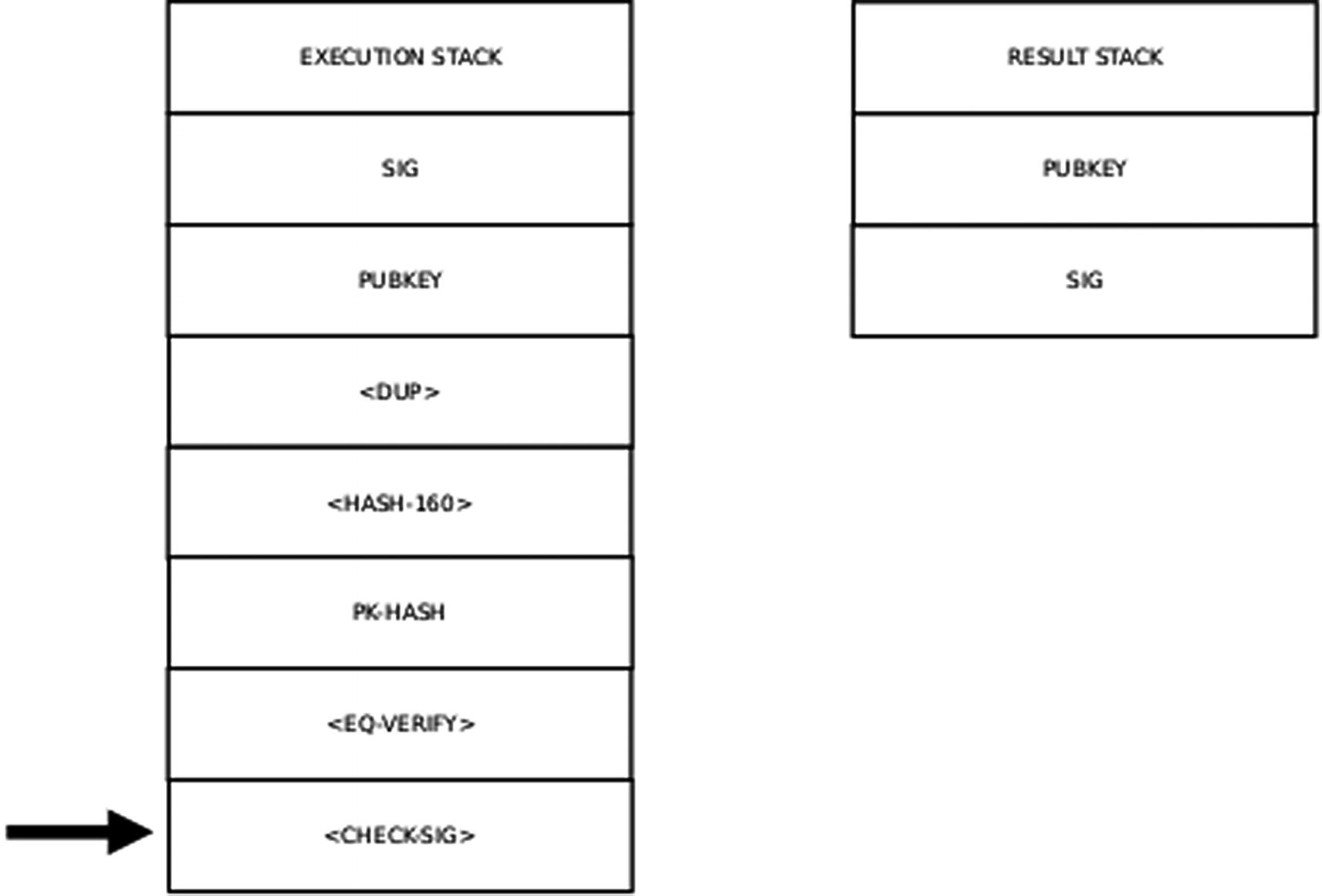
Step 11 of the Execution Stack
- 1.
Given the public key that Alice used for the prior transaction output, verify that the private key corresponding to this public key has generated the signature of the value
- 2.
If the signature is valid, pop the two elements off the result stack. The vin element of the transaction has validly unlocked the output of the previous transaction (Figure 9-12).

Transaction Output Unlocked
If all of the vin elements in the present transaction are unlocked, then we say that the transaction is unlocked.
Multisig Transactions
Consider a fiat currency checking account that requires both spouses to sign a check. A valid transfer of value in this case requires the signature of both spouses. The delivery of such a cheque to the drawee is a multi-signature or multisig transaction. In a cryptocurrency network, a multisig transaction refers to a transaction involving n > 1 entities, where each entity provides a public address for the transaction. Then at least m of these n entities have to provide their signatures before value will be transferred to a recipient. This type of transaction is frequently called a n:m transaction; it is implemented through a script that verifies multiple signatures. Our cheque example is a 2:2 transaction.
There are several interesting use cases for multisig transactions.
In a 2:2 escrow transaction, one of the parties is a neutral arbitrator whose function is to oversee that agreed conditions for the transfer of value have been satisfied. The arbitrator only provides his or her signature if these conditions are satisfied. We can also have m:n escrow transactions where m < n arbitrators have to sign a transaction.
In a two-factor authentication transaction, an output in the previous transaction is locked by two public addresses. The first public address is generated by an online wallet. The second address is generated from keys which are kept in cold storage.6 The output from the previous transaction will be transferred when both signatures are verified.
The simple stack language that we have specified can be used to create scripts for multisig transactions as well as other scenarios. Note that since this stack language does not support control flow constructs (such as if … else if …) and loops, it is not Turing complete. This was a deliberate omission by Satoshi Nakamoto since it was a design objective to make script processing fast and simple.
Transaction Fees
A person transferring value in a transaction typically provides miners with an incentive to include the transaction in a new block to be mined. This transaction fee is the difference between the sum of the inputs and the outputs. It is not necessary to provide a transaction fee, but the failure to do so may significantly delay the processing of the transaction, as miners are not incentivized to include the transaction in candidate blocks to be mined.
Transaction Validation
Once a Helium transaction has been created, it is broadcast on the Helium peer-to-peer network. A miner who receives this new transaction can elect to include it in a block that he is mining. The miner’s decision to include the transaction will be partially influenced by the transaction fee offered by the transaction.
Before including the transaction in a block, the miner will test the transaction to determine its validity. Only valid transactions will be included in the block that is to be mined.
If a miner mines a block that has an invalid transaction, other nodes in the network will refuse to incorporate this new block in their local versions of the blockchain since each node that receives a mined block tests the validity of the block as well as the transactions in the block.
- 1.
The transaction’s syntax is valid; this means that the required transaction fields are present and their types are valid.
- 2.
The transaction inputs are positive integers. In particular, they are not floating-point numbers.
- 3.
The transaction outputs are positive integers. In particular, they are not floating-point numbers.
- 4.
Transaction inputs and outputs are not less than a Helium cent.
- 5.
The sum of the transaction inputs is less than the value of MAX_HELIUM_COINS in the hconfig module.
- 6.
The sum of the transaction outputs is less than the value of MAX_HELIUM_COINS in the hconfig module.
- 7.
The sum of the transaction inputs is equal to or greater than the sum of the transaction outputs.
- 8.
The locktime of the transaction is not less than zero.
- 9.
The locktime is not greater than the value of LOCKTIME_INTERVAL in the hconfig module.
- 10.
Each transaction is at least 100 bytes long.
- 11.
The number of transaction inputs is less than MAX_INPUTS in the hconfig module.
- 12.
The number of transaction outputs is less than MAX_OUTPUTS in the hconfig module.
- 13.
The ScriptSig script has valid values.
- 14.
The ScriptPubKey script has valid values.
- 15.
The ScriptSig and ScriptPubKey scripts succeed in transferring value.
- 16.
The outputs of a coinbase transaction are not spent until the blockchain grows by COINBASE_INTERVAL blocks from the block in which the coinbase transaction is located. We will discuss coinbase transactions subsequently.
- 17.
A coinbase transaction does not have any inputs (the vin list is empty).
- 18.
The genesis block does not have any inputs.
Conclusion
The fundamental unit of computation in a cryptocurrency network is a transaction. The blocks in a blockchain contain transactions. Blocks are added to a blockchain as transactions are processed by nodes on the cryptocurrency network. These nodes are called miners, and the process of adding a block to a blockchain is called mining.
In this chapter, we have looked at the structure of a canonical cryptocurrency transaction and examined all of the steps that must be followed to unlock the inputs in previous transactions so that they can be spent. Nodes that want to add a block to the cryptocurrency blockchain must include transactions in their candidate block. These nodes must, at the outset, verify that the transactions included in a block are valid. We have examined transaction validation tests. If a mining node successfully adds a block to the blockchain, it is entitled to the transaction fees in respect to all of the transactions in the block as well as a mining reward embodied in a coinbase transaction.
In the next chapter, we are going to study Merkle trees. These tree structures are used to ascertain whether a list of transactions has been tampered with. Merkle trees can also be used to ascertain whether a particular transaction is in a block.
Reference
https://github.com/bitcoin/bitcoin, last accessed on February 8, 2020