This chapter throws light on building representational state transfer (REST)-based services. Since we’ll be learning RESTful Web Services through our chosen language, Node.js, and using an awesome framework like hapi, it’s first necessary to introduce ourselves to REST APIs and then understand how hapi makes the process easy. Along the ride, we’ll learn about HTTP verbs, resource handling, stateless constraints, and tying it all into representational state transfer.
First Steps
If you really need to know why we’re all talking about APIs in the early twenty-first century, you need to understand that while developed software is what the end user sees, at the back end, it’s really data exchange. And who is exchanging that data? Two or multiple entities and layers of software. What is the format of such data? XML was a very popular data exchange format in the early stages of data talk – and while we look into the reasons of using JSON as a data exchange format in our capstone project later, we need to understand how typical data exchange looks and how it’s different from the presentation layer.
Consider a scenario where we need information about how many students signed in to the chemistry lab on a particular date. Let’s assume that the information is in a register with the chemistry lab manager. So what you’re doing is asking for a data exchange between that register and yourself, via the lab manager. What does the lab manager do? He serves the information (that’s a service) and provides information in the format you want (that’s the data set returned) and the medium, in this case would be whatever mechanism he chooses to filter such information from the register. Fortunately for us, the world moved away from paper to digital long ago; and instead of registers and books, we came up with the programmable Web.
API
The term API means an application programming interface, where an interface is a medium of communication. When we exchange data, our medium is a service that will use logic to talk to a database or another application layer for fetching data.
If you’ve spent a fair share of your time in web programming, you already know what the request and response cycle is about. For those who’ve migrated from other backgrounds, all you’re expected to know is that all of the Web is ultimately a request and response cycle from and to a server. So typically, one caller – the “client” – calls out to the “server” and asks for data sets, and the latter serves the request and returns a response in an object over a protocol, a set of predefined rules of communication. After all, all we’re doing is talking, aren’t we?
REST API
REST APIs and RESTful Web Services made through REST APIs rely on an architectural style called representational state transfer . If you’re a beginner, just remember that you are representing the state of an object or a resource and transferring it over the Web. It becomes more of a contract while we are dealing with resource exchange, with a set of rules.
The Web ultimately is a hub of resources. Remember the example of the lab manager? You’re asking for resource information when you want data of all students who signed in on a particular date. Such resource information given by services on the Web needs to be scalable, easy to implement, maintainable, and extensible because it’s not going to be a one-time request like our real-world example of the lab manager, hence the emphasis on design. A RESTful design performs extremely well where all you need is data to be consumed by a consumer – this consumer can be any client software, for instance, a mobile app. All your handheld apps request some resources, and those resources are served by hitting REST API URLs.
The mobile app that is requesting the data should be able to understand the format in which the response is given.
Breaking the representation tactfully into smaller resources is a good way of creating the response and utilizing it.
In the following URL, you are querying the resource users and filtering them further to a user called Jake Wharton (the field username is a parameter): https://api.github.com/users/JakeWharton.
We’ll look into approaches of addressing resources and how REST introduces a clean way of doing it. Before that, let’s brush up on HTTP request and response objects and methods.
A Brushup on HTTP
Why a brushup on HTTP? Well, it’s the most commonly used protocol for implementing REST APIs. The architectural style doesn’t know any protocol on its own. It speaks of verbs and activities which are already present in HTTP very explicitly, hence the choice. For greater understanding, it is highly recommended that the reader chooses to browse through the gist of other protocols such as SOAP, SPDY (deprecated), and GOPHER. It serves well to know how these work and why HTTP becomes a more natural choice for implementing RESTful Web Services. A quick sidenote, prior to REST, web services were designed according to another architectural style named RPC. This was complex and not scalable. The SMB market was thus not exposed to the API world. The HTTP reliance has in fact introduced RESTful Web Services to the world of Web and mobile, which is what rules the present.
I always recommend understanding HTTP through OOP (Object-Oriented Programming) – objects and activities. The client and service talk to each other via messages. Your request message is an object, and like OOP, your object has attributes and activities/methods. Coming back to the manager example, you requested the manager for information on students who logged in on, say, January 29, 2018. Your message to the manager is an object in itself. You asked him to GET the information from a register (a URL in case of the WWW) and return a formatted RESPONSE. Along with it, your request is best served when the manager knows that the information is confidential, to be well secured, and so on – which you communicate through request metadata.
An activity – The kind of request you’re making, GETting the data from the register.
A URI – The name of the register or the resource locator.
Metadata - Settings of the request object, giving more information about the request.
Request body – In a RESTful service, that’s where the representations of resources sit.
HTTP version – Is the version of HTTP.
What a Request Cycle Entails
Client | Request Object | Response |
---|---|---|
Mobile or Web | VERB – HTTP methods like GET, PUT, POST, DELETE, OPTIONS | Service layer (REST API) |
URI – Answers the question “Which service layer do I want?” | ||
Metadata – Headers like the content lengthand others | URI ➤ Router ➤ Program method for execution | |
Request body – Additional content with the request like files, form data, etc. | ||
HTTP version |
HTTP Request Methods
We’ll only be touching briefly on the methods here, and more in detail when we use them in our applications. We’ll explore two in greater detail, the ones most commonly used: GET and POST.
GET Method
Let’s just understand the GET method first, which says that GET me this and that data (represented by parameters) from this URL.
Let’s examine the following URL when we hit it in the browser and in a client tool like POSTMAN: https://api.github.com/. This is a public API provided by GitHub, and many such API endpoints can be studied on developer GitHub pages.
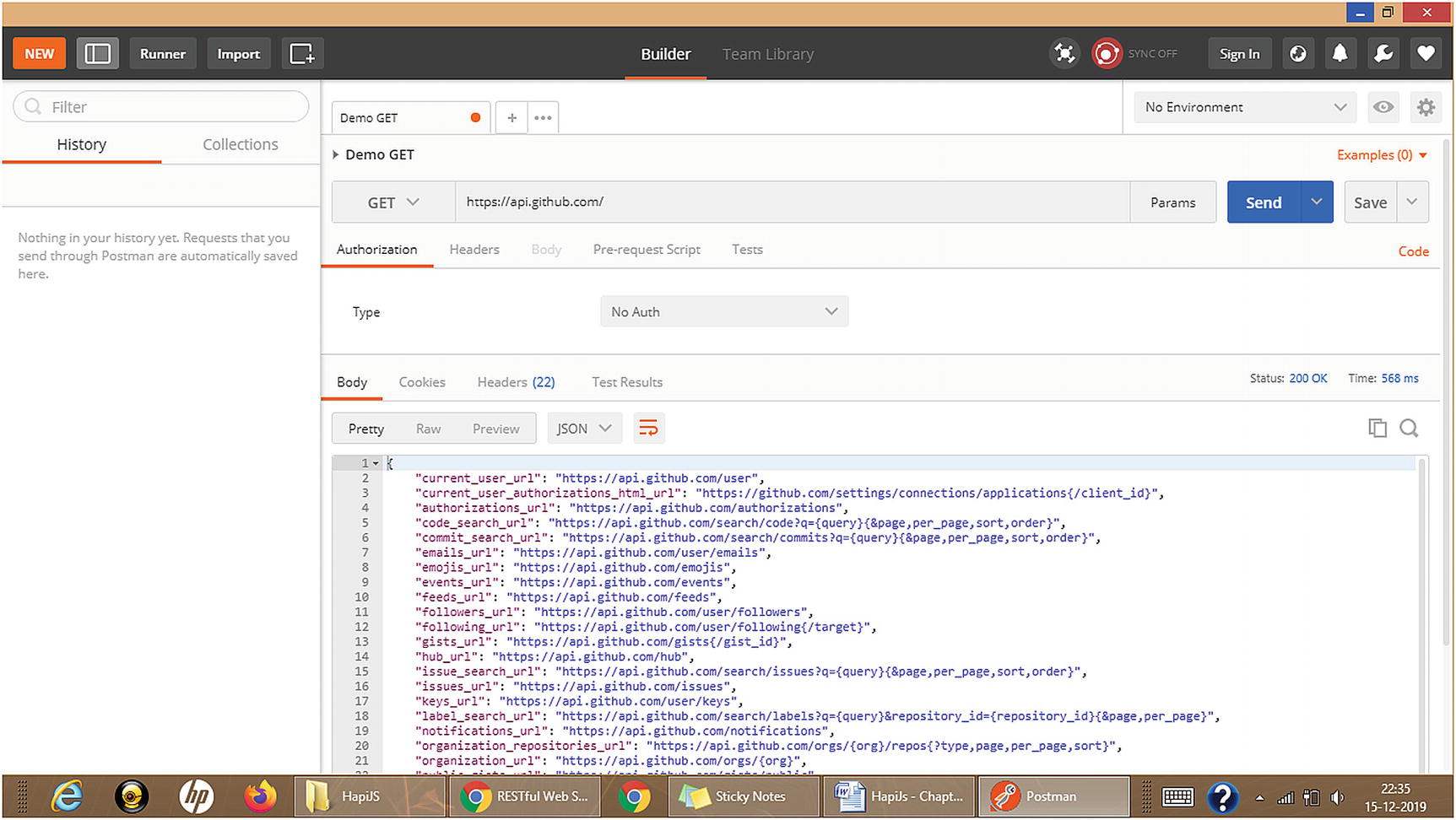
Request and Response Object in HTTP
A Partial View of the Response from the github api
Go through the API list on GitHub and see what each API provides. It’s a very interesting insight.
Addressing Resources
The REST Architecture Approach in Addressing Resources
REST requires each resource to have at least one URI.
A RESTful service uses a directory hierarchy like human-readable URIs to address its resources, separated by slashes as shown in the preceding text. The format is almost always by convention and good practice:
Protocol://ServiceName/ResourceType/ResourceID
The Query Parameter Approach in Addressing Resources
What we saw earlier was the REST style of addressing resources. For instance, http://schoolOfDelhi/candidates/1 is a newer way of addressing resources as opposed to the query parameter approach: http://schoolOfDelhi/candidates?id=1.
Readability – Your URLs are extremely readable in the REST fashion, that is, a directory hierarchy format.
Coherence – If you need to provide file format information or information which isn’t directly related to the resource you are fetching, you could mix and match the two approaches:http://schoolOfDelhi/candidates/1?format=xml&encoding=UTF8.
Search engine–friendly – Your URL might not remain user-friendly if you make it too complex with query parameters. SEO guides could tell you how to structure your URLs, most often keeping the name of the resource directly after the service name, followed by the unique identifier.
Initial purpose – Query parameters were initially intended for providing parameter values to a process. For instance, in the preceding URL where we provide the format as xml and encoding as UTF-8, using these as parameters to a service is one thing and specifying the ID as a mandate is another.
Consider this URL:
https://api.github.com/search/users?q=repos:>42+followers:>100
If you use this URL on a browser directly, you get a list of users where the number of repos is greater than 42 and the number of followers is greater than 100, for each. Notice how the URL is structured; it gives the name of the HOST, the service or the OPERATION, and most importantly the RESOURCE – all this in the REST style of structuring a URL. The parameters that further decide the filters of the resource and are complex to structure in a folder hierarchy manner are presented in a query string format.
POST Method
The POST method helps you to write data to a database using a service over the HTTP protocol.
The POST method is used to submit an entity to the specified resource, often causing a change in state or side effects on the server.
In May 2015, HTTP/2 was officially standardized. By July 2016, 8.7% of all websites were already using it, representing more than 68% of all requests then. HTTP 1.1, however, has been the most stable version of the protocol, even though many extensions provide alternate ways of compressing headers and improving performance and others.
PUT, PATCH, DELETE, and OPTIONS are not needed at first but are important in the journey of developing a RESTful Web Service. In other words, when you are handling resources, you should know what the HTTP protocol provides, and therefore we will look into all of these along with what are safe and idempotent operations as we go on. We will also notice how RESTful Web Services handle Cross-Origin Headers, cookies, caching, authentication, and much much more. The reason of not going forward with such definitions here is that we’d better understand them in the context of hapi.js and our applications.
Examining the Response Object
Client | Response Object | Response |
---|---|---|
A business routine | The version of the HTTP protocol | Mobile or web app |
A status code, indicating if the request was successful, or not, and why, and a status message | ||
Metadata – Headers like headers for requests | ||
A body containing the fetched resource |
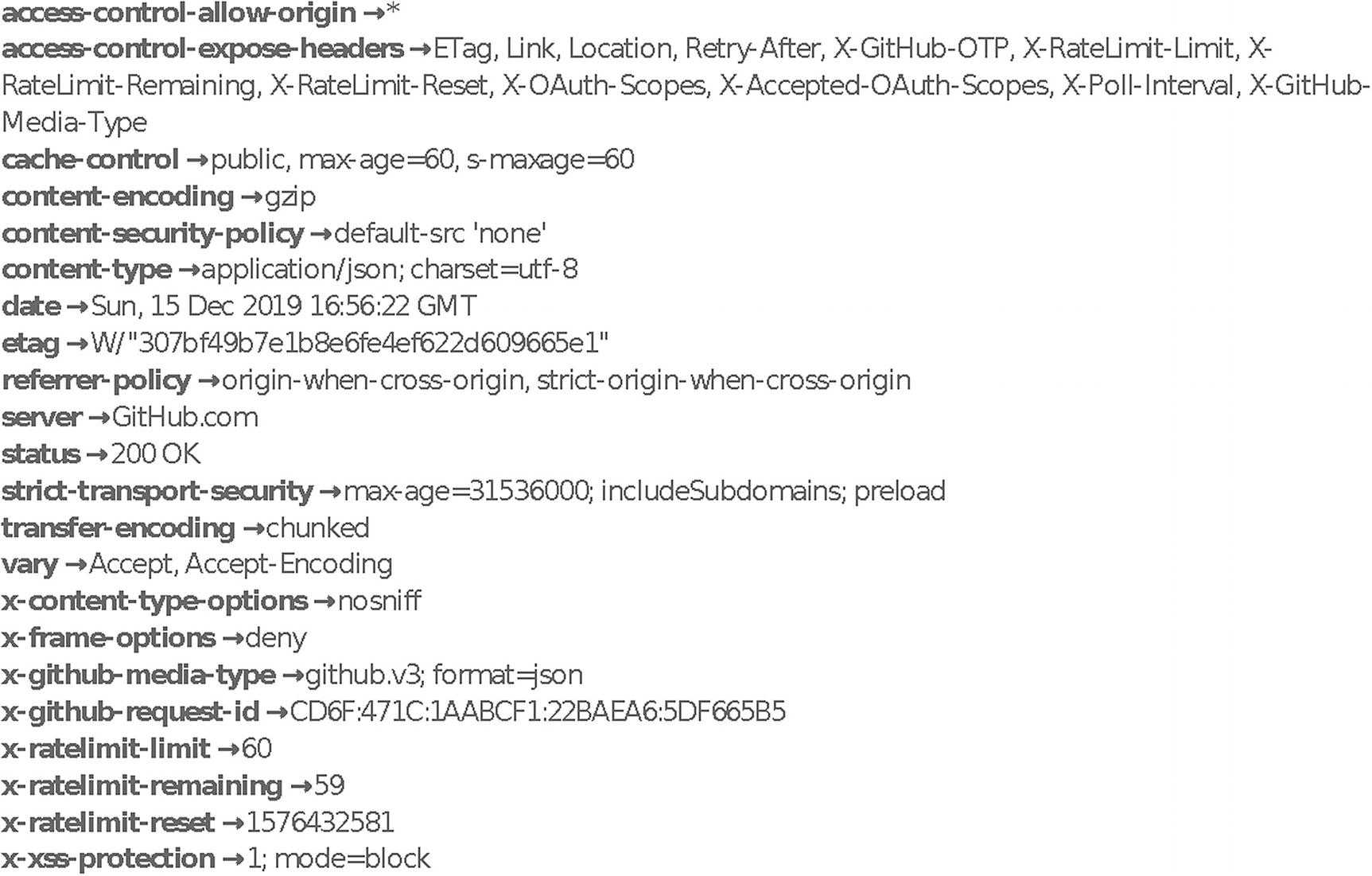
Response Headers for a Sample GET Request
The Stateless Constraint
A very important factor in the REST architecture is statelessness. Each request from client to server must contain all of the information necessary to understand the request and cannot take advantage of any stored context on the server. Session state should be therefore kept entirely on the client. Every HTTP request happens in complete isolation. Previously, there was a way of storing the session on the server, and while that reduced the complexity, storing the application state on the server had various disadvantages including having to monitor the state of the application from the server, which in fact is the client’s job. The server is best suited for the resource state, which it returns as a response. In short, your request should not depend on any other request or the session on the server. A web service in REST only needs to care about your application state when you’re actually making a request. The rest of the time, it should not even know you exist. This means that whenever a client makes a request, it must include all the application states the server will need to process it.
Summary
The main aim of this chapter was to give you a glimpse of what you’re doing when you’re coding a RESTful Web Service. You’re requesting information, through an API, and you’re sending objects and receiving objects to do the same. What is important to remember is that REST is just an architectural style and is independent of any protocol. We discussed HTTP because the protocol provides VERBS that RESTful architecture uses.
Architectures can be based on other application layer protocols if they already provide a rich and uniform vocabulary for applications based on the transfer of meaningful representational state.
When styling in REST, you need to treat every resource on a separate URL. Guidelines for stateless design make the application easily scalable and fast to access. Our recommendations in Further Reading also include a dissertation – should you actually care to read it – which gives an insight into the real need of REST.
Further Reading
Developer docs on Mozilla – https://developer.mozilla.org/en-US/docs/Web/HTTP
A comprehensive listing of public APIs – www.programmableweb.com/api-university
The REST Wiki – https://en.wikipedia.org/wiki/Representational_state_transfer
A paper worth reading regarding the original need of REST – https://github.com/otaviofff/restful-grounding/blob/master/papers/official-paper-icwi.pdf