Depend on abstractions, not on concretions.
The name of this principle contains the word “inversion,” from which we may infer that without following this principle we would usually depend on concretions, not on abstractions. The principle tells us to invert that direction: we should always depend on abstractions.
Example of Dependency Inversion: the FizzBuzz Generator
Generate a list of numbers from 1 to n.
Numbers that are divisible by 3 should be replaced with Fizz.
Numbers that are divisible by 5 should be replaced with Buzz.
Numbers that are both divisible by 3 and by 5 should be replaced with FizzBuzz.
1, 2, Fizz, 4, Buzz … 13, 14, FizzBuzz, 16, 17 …
An Implementation of the FizzBuzz Algorithm
Given the assignment, this is a very accurate implementation of the requirements. Reading through the code, we are able to recognize every requirement in it: the rules about the divisibility of the numbers, the requirement that the list of numbers starts at 1, etc.
It should be possible to add an extra rule without modifying the FizzBuzz class.
Making the FizzBuzz Class Open for Extension
Currently the FizzBuzz class is not open for extension, nor closed for modification. If numbers divisible by 7 should one day be replaced with Whizz, it will be impossible to implement this change without actually modifying the code of the FizzBuzz class.
Pondering about the design of the FizzBuzz class and how we can make it more flexible, we note that the generateElement() method contains lots of details. Within the same class, though, the generateList() method is rather generic. It just generates a list of incrementing numbers, starting with 1 (which is somewhat specific), and ending with a given number. So the FizzBuzz class has two responsibilities: it generates lists of numbers, and it replaces certain numbers with something else, based on the FizzBuzz rules.
Extracting a Method for Generating Separate Elements Using “Rules”
A Class that Represents One of the FizzBuzz Rules
This is one step in the right direction. Even though the details about the rules (the numbers 3, 5, 3 and 5, and their replacement values) have been moved to the specific rule classes, the code in generateElement() remains very specific. The rules are still represented by (very specific) class names, and adding a new rule would still require a modification of the generateElement() method, so we haven’t exactly made the class open for extension yet.
Removing Specificness
Introducing Abstraction
Setting Up a FizzBuzz Instance with Concrete Rules
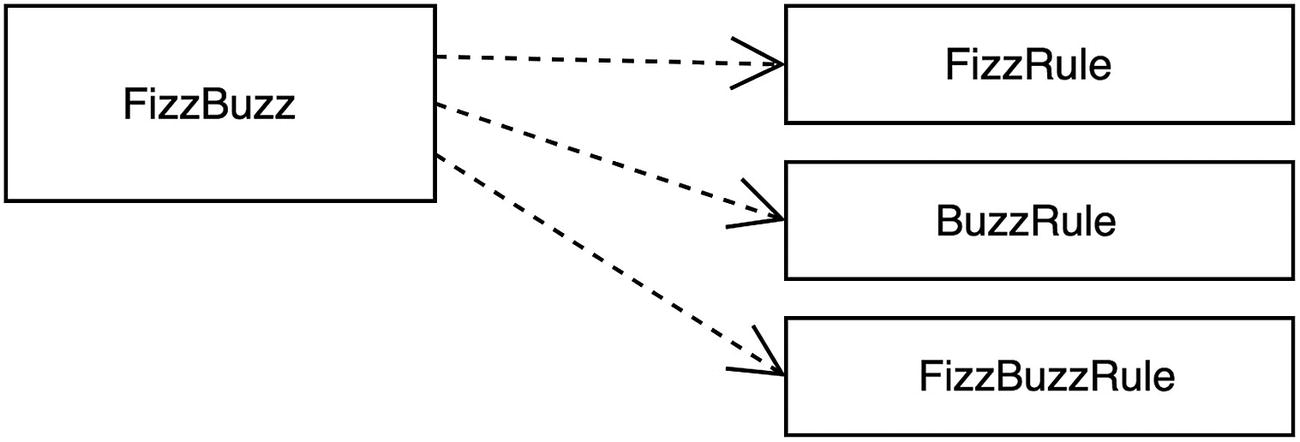
FizzBuzz with concrete dependencies
Now we have a highly generic piece of code, the FizzBuzz class, which “generates a list of numbers, replacing certain numbers with strings, based on a flexible set of rules”. There’s no mention of “FizzBuzz” in that description and there’s no mention of “Fizz” nor “Buzz” in the code of the FizzBuzz class . Actually, the FizzBuzz class may be renamed so that it better communicates its responsibility. Of course, naming things is one of the hardest parts of our job and NumberListGenerator isn’t a particularly expressive name, but it would better describe its purpose than its current name.
Looking at the initial implementation of the FizzBuzz class, it has become clear that the class had an abstract task from the start: to generate a list of numbers. Only the rules were highly detailed (being divisible by 3, by 5, etc.). To use the words from the Dependency Inversion principle: an abstraction depended on concrete things. This caused the FizzBuzz class to be closed for extension, as it was impossible to add another rule without modifying it.
Abstractions should not depend upon details. Details should depend upon abstractions.

FizzBuzz with abstract dependencies
Now that we’ve seen the Dependency Inversion principle in action, we can take a look at some situations where it’s clearly being violated.
Violation: A High-Level Class Depends on a Low-Level Class
The first violation arises from mixing different levels of abstraction . It’s an interesting concept that needs further explanation before we dive into the example.
On a daily basis we have to deal with a great diversity of things that exist in the universe. We wouldn’t be able to do anything meaningful if we’d consider every little detail of everything we talk about, everything we use while doing our job, everyone we love. So to preserve our sanity, we come up with abstractions all the time. “Abstraction” means “taking away the details”. What remains is a concept that can we can use to group all the specific things from which the abstraction has been created, and a name for what’s essential to all these specific things, ignoring the little differences.
In conversation we usually end up establishing some level of abstraction, so we can safely ignore the details (or the larger picture). When discussing software design, this means we zoom in or out until we can address the issue at hand. For instance, when discussing a code smell, we’ll be talking about method signatures, variable names, etc. so we can safely ignore the message queue software, or the particular Linux filesystem that we use on our server. When we talk about how the application gets data from the database, we discuss SQL queries, so we can ignore the underlying TCP protocol that’s being used.
Zooming in and out is the same as moving from abstraction to concretion and back again. The more we zoom in on a part of our software, the closer we get to the low-level details (also known as “internals”). The more we zoom out, the closer we get to a high-level view of the system; what features it aims to provide to its users.
In class design, we have to consider the same kind of zooming in and out. Every class has two levels of abstraction: the first is the one perceived by clients. The second is the one that’s going on inside. By definition, a class or an interface is going to hide some implementation details for its client, meaning that the clients will perceive it to be more abstract, while the class internally is more concrete.
So a class’s internals are always more concrete than the abstraction that the class represents. However, when a class depends on some other class, it should again depend on something that is abstract, not concrete. That way, the class itself becomes a client of something abstract and can safely ignore all the underlying details of how that dependency works under the hood.
The Authentication Class

The Authentication class depends on Connection
- 1.
Is it important for an authentication mechanism to deal with the exact location of the user data?
Well, definitely not. The only thing the Authentication class really needs is user data, as an array or preferably an object representing a user. The origin of that data is irrelevant.
- 2.
Is it possible to fetch user data from some other place than a database?
Currently it’s impossible. The Authentication class requires a Connection object, which is a database connection. You can’t use it to retrieve users from, for instance, a text file or from some external web service.
Looking at the answers, we have to conclude that both the Single Responsibility principle and the Open/Closed principle have been violated in this class. The Authentication class is not only concerned about the authentication mechanism itself, but also about the actual storage of the user data. Furthermore, it’s impossible to reconfigure the class to look in a different place for user data. The underlying reason for these issues is that the Dependency Inversion principle has been violated too: the Authentication class itself is a high-level abstraction. Nevertheless, it depends on a very low-level concretion: the database connection. This particular dependency makes it impossible for the Authentication class to fetch user data from any other place than the database.
Trying to rephrase what the Authentication class really needs, we realize that it’s not a database connection, but merely something that can provide the user data. Let’s call that thing a “user provider”. The Authentication class doesn’t need to know anything about the actual process of fetching the user data (whether it originates from a database, a text file, or an LDAP server). It only needs the user data.
It’s a good thing for the Authentication class not to care about the origin of the user data itself. All the implementation details about fetching user data can be left out of that class. At once, the class will become highly reusable, because it will be possible for users of the class to implement their own “user providers”.
Refactoring: Abstractions and Concretions Both Depend on Abstractions
Introducing the UserProvider Class

Authentication depends on UserProvider
Overriding Functionality of UserProvider
Introducing the UserProviderInterface and Some Implementations
The Authentication Class Now Accepts a UserProviderInterface
High-level modules should not depend upon low-level modules. Both should depend upon abstractions. 3
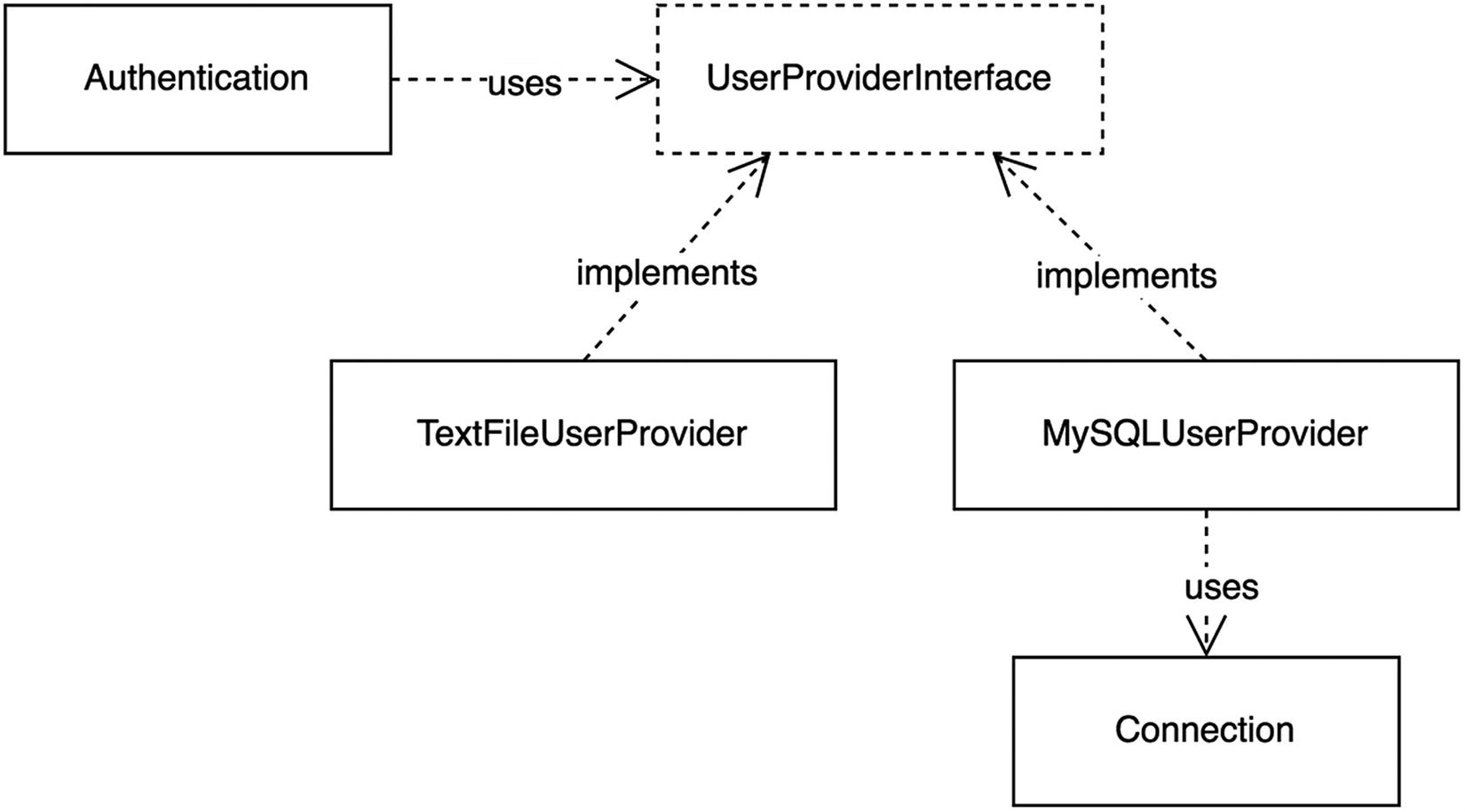
Authentication depends on UserProviderInterface
A nice side-effect of the changes we made is that the maintainability of the code has greatly improved. When a bug is found in one of the queries used for fetching user data from the database, there’s no need to modify the Authentication class itself anymore. The necessary changes will only occur inside the specific user provider, in this case the MySQLUserProvider . This means that this refactoring has greatly reduced the chance that you will accidentally break the authentication mechanism itself.
Simply Depending on an Interface is not enough
The step from UserProvider to UserProviderInterface was an important one because it helped users of the Authentication class easily switch between user provider implementations. But just adding an interface to a class is not always sufficient to fix all problems related to dependencies.
An Alternative UserProviderInterface
This is not a helpful interface at all. It’s an immediate violation of the Liskov Substitution principle. Not all classes that implement this interface will be able to be good substitutes for it. If one implementation doesn’t use a database table for storing user data, it most certainly won’t be able to return a sensible value when someone calls getTableName() on it. But more importantly: the UserProviderInterface mixes different levels of abstraction and combines something high-level like “finding a user” with something low-level like “the name of a database table”.
So even if we would introduce this interface to make the Authentication class depend on an abstraction instead of concretion, that goal won’t be reached. In fact, the Authentication class will still depend on something concrete and low-level: a user provider that is table-based.
Violation: Vendor Lock-In
The EventDispatcherInterface
An Event Class and an Event Listener
The Event Dispatcher from the Laravel Framework
Using the Laravel Event Dispatcher
It appears that you can do more or less the same things with both event dispatchers, i.e. fire events and listen to them. But the way you do it is different in subtle ways.
The UserManager Class
Using the Laravel Event Dispatcher in the UserManager
A couple of weeks later, you start working on a project built with the Symfony framework. You want to reuse the UserManager class, since it offers exactly the functionality that you need, and you install the package containing it inside this new project. Now, a Symfony application also has an event dispatcher readily available in its service container. But this event dispatcher is an instance of EventDispatcherInterface . It’s impossible to use the Symfony event dispatcher as a constructor argument for the UserManager class because the type of the argument wouldn’t match the type of the injected service. You have effectively prevented reuse of the UserManager class .
If you still want to use the UserManager class in a Symfony project, you would need to add an extra dependency on the illuminate/events package to make the Laravel Dispatcher class available in your project. You’d have to configure a service for it, next to the already existing Symfony event dispatcher and end up having two global event dispatchers. Then you’d still need to bridge the gap between the two types of dispatchers, since events fired on the Laravel Dispatcher won’t be fired automatically on the Symfony event dispatcher too. In fact, they even use incompatible types (event objects versus arrays).
The moment you picked the Laravel event dispatcher as the event dispatcher of your choice, you coupled the package to a specific implementation, making it harder or impossible to just use the package in a project that uses a different event dispatcher. Introducing such a dependency to your package is known as “vendor lock-in”; it will only work with third-party code from a specific vendor.
Solution: Add an Abstraction and Remove the Dependency Using Composition
Introducing an Abstraction and Using it in UserManager
The UserManager is now fully decoupled from the framework. It uses its own event dispatcher, which is quite generic and contains the least amount of details possible.
Concrete Implementation of the Abstract DispatcherInterface That Uses the Laravel Event Dispatcher
Alternative Implementation of DispatcherInterface That Uses the Symfony Event Dispatcher

The UserManager has a dependency on the concrete Laravel Dispatcher
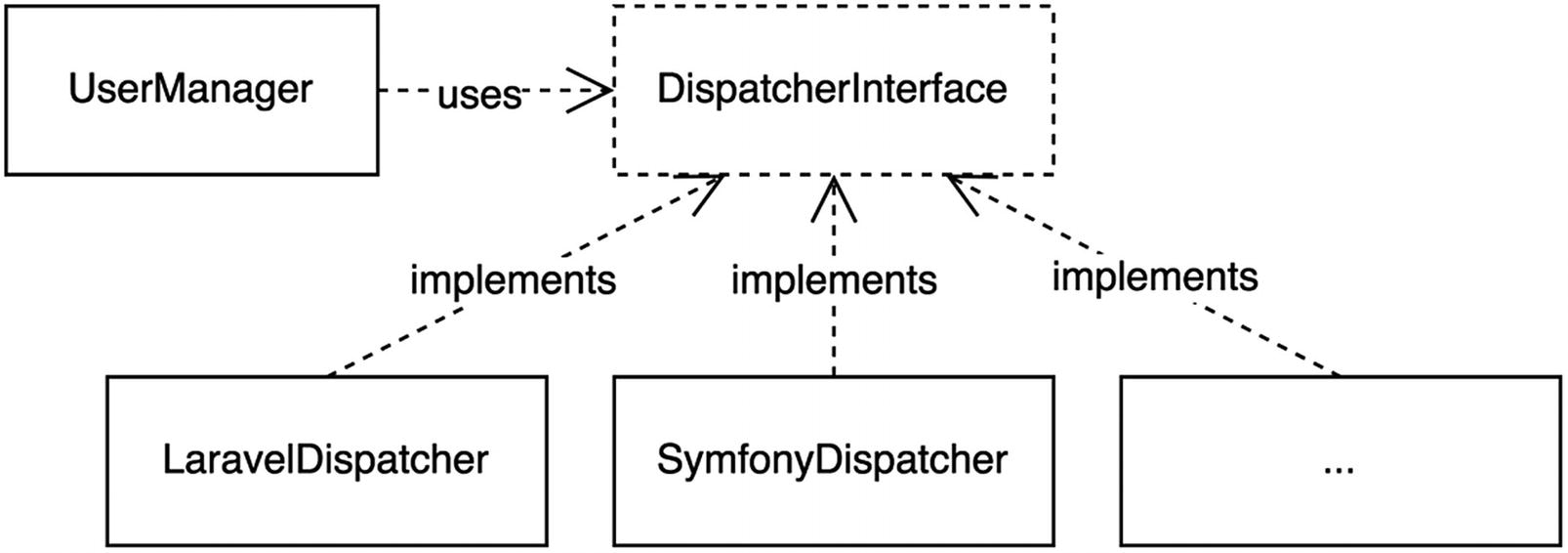
The UserManager has a dependency on an abstract dispatcher, on which several adapters depend
Packages and the Dependency Inversion Principle
Although the Dependency Inversion principle is a class design principle, it’s all about the relationship between classes. This relationship often crosses the boundaries of a package. Therefore the Dependency Inversion principle resonates strongly at a package level. According to this principle, classes should depend on abstractions, not on concretions. In parallel to this, packages themselves should also depend in the direction of abstractness, as we see in Chapter 11.
Depending on Third-Party Code: Is It Always Bad?
We got rid of vendor lock-in for the UserManager class and we now know the principle by which we can achieve the same thing in many other situations. However, in doing so, there’s a certain cost involved—the cost of defining our own interface and our own adapter implementations. Even if we use dependency inversion for every dependency of every class, there are still other ways in which our code will remain dependent on third-party code.
So the question is, in which cases should we allow ourselves to depend on third-party code and which cases definitely call for dependency inversion?
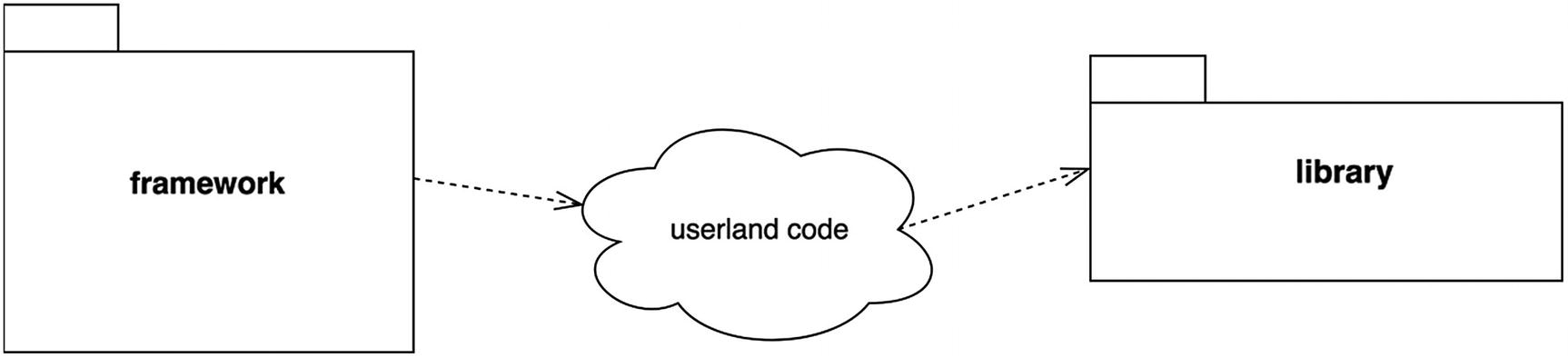
Framework calls userland code, which calls library code
If you’re a package developer who wants to extract part of the userland code and publish it as a package, you should only take out the part of the code that isn’t coupled to the framework. Make sure the code will be useful to all users, no matter what framework they put in front of it. The remaining part of the userland code that is coupled to the framework can be extracted into a framework-specific package, often known as a “bridge” package.

The dependency graph after extracting a package and making it independent of the framework and libraries
Not all third-party code requires you to apply the Dependency Inversion principle . If you’d never be allowed to depend on any third-party code directly, you’d have to reinvent everything again and again. In the next section, we enumerate the types of classes that definitely need an interface. What remains are classes that can be used as they are. In practice, I find that these will be classes that do one thing and do it well. Don’t be afraid to depend on those, in particular if their maintainers do a good job in terms of package design.
Assertions
Reflection
Mapping
Encoding/decoding
Inflection
You’ll likely be able to add some more examples. In practice, you may also decide to depend directly on other concrete third-party code, even though it would call for dependency inversion. You can always add an interface later, and for now enjoy building on top of other people’s ready-to-use building blocks, sacrificing flexibility for an earlier release.
There’s another option that you should think of when you consider using third-party code. Instead of working around their somewhat awkward API, or dealing with their unpredictable release cycle, you may also decide to copy their idea and build your own version of it. For example, if you need an event dispatcher, but none of the popular event dispatcher packages matches your expectations, consider writing one yourself. It isn’t much code anyway, and by doing it, you can design it just the way you want it to be. It’s not always the most efficient thing to do, but at least you have to consider it as a valid option. When reinventing the wheel, sometimes you end up with a better wheel.
When to Publish an Explicit Interface for a Class
In previous chapters, we’ve seen interfaces being used to make classes open for extension and closed for modification. We’ve learned how subclasses can be good substitutes for their interfaces. We’ve also discussed how to split interfaces according to how they are used. And finally we’ve learned how to invert dependency directions toward more abstract things. What we didn’t discuss in detail is which classes actually need an interface. As mentioned several times before, not every class needs an interface. So before we wrap up this chapter and dive into the package design principles, let’s first answer this question: when should you publish an explicit interface for a class? And when is a class without an interface sufficient?
If Not All Public Methods Are Meant to be Used by Regular Clients
The Original EntityManager Class and its Extracted Explicit Interface
However, regular clients of EntityManager won’t need access to the internally used Connection or Cache object, which can be retrieved by calling getConnection() or getCache() , respectively. You could even say that the implicit interface of the EntityManager class unnecessarily exposes implementation details and internal data structures to clients.
The Improved EntityManagerInterface
We’ve discussed this strategy in more detail in Chapter 4 when we covered the Interface Segregation principle, which tells you not to let clients depend on methods they don’t use (or shouldn’t use!).
If the Class Uses I/O
The CurlHttpClient and its Interface
If you’d like to know more about using test doubles to replace actual I/O calls, take a look at my article series on “Mocking at Architectural Boundaries”8.
If the Class Depends on Third-Party Code
The (implicit) interface wouldn’t be how you would’ve designed it yourself.
You’re not sure if the package is safe to rely on.
The FunkyDiffer
An Adapter for the FunkyDiffer
The advantage of this approach is that from now on you can always switch to a different library, without changing the bulk of your code. Only the adapter class needs to be rewritten to use that other library.
A Façade for FunkyDiffer
If You Want to Introduce an Abstraction for Multiple Specific Things
Example of a VoterInterface Implementation
In the case of the VoterInterface , the package maintainers serve the users of their package by offering them a way to provide their own authorization rules. But sometimes an abstraction is only there for the code in the package itself. In that case too, don’t hesitate to add it.
If You Foresee That the User Wants to Replace Part of the Object Hierarchy
In most cases, a final class is the best thing you can create. If a user doesn’t like your class, they can simply choose not to use it. However, if you’re building up a hierarchy of objects, you should introduce an interface for every class. That way the user can replace a particular piece of logic somewhere in that hierarchy with their own logic. It will make your code useful in as many situations as possible.
A nice example comes from Tactician13, which offers a command bus implementation.
The CommandBus Class (Abbreviated)
The Middleware Interface (Abbreviated)
Setting Up CommandHandlerMiddleware
Each collaborating object that gets injected into CommandHandlerMiddleware can easily be replaced by re-implementing the interfaces of these objects (CommandNameExtractor, HandlerLocator, and MethodNameInflector, respectively). Because CommandHandlerMiddleware depends on interfaces, not on concrete classes, it will remain useful for its users, even if they want to replace part of the built-in logic with their own logic, such as when they would like to use their favorite service locator to retrieve the command handler from.
By the way, adding an interface for those collaborating objects also helps the user decorate existing implementations of the interface by using object composition.
For Everything Else: Stick to a Final Class
If your situation doesn’t match any of the ones described previously, most likely the best thing you can do is not add an interface, and just stick to using a class, preferably a final class . The advantage of marking a class as “final” is that subclassing is no longer an officially supported way of modifying the behavior of a class. This saves you from a lot of trouble later on when you’re changing that class as a package maintainer. You won’t have to worry about users who rely on your class’s internals in some unexpected way.
Classes that model some concept from your domain (entities and value objects).
Classes that otherwise represent stateful objects (as opposed to classes that represent stateless services).
Classes that represent a particular piece of business logic, or a calculation.
What these types of classes have in common is that it’s not at all needed nor desirable to swap their implementations out.
Conclusion
As we’ve seen in this chapter, following the Dependency Inversion principle is helpful when others start using your classes. They want your classes to be abstract, only depending on other abstract things, and leaving the details to a couple of small classes with specific responsibilities.
Applying the Dependency Inversion principle in your code will make it easy for users to swap out certain parts of your code with other parts that are tailored to their specific situation. At the same time, your code remains general and abstract and therefore highly reusable.