Python is a large and complex language that I cannot hope to cover in one chapter. There are simply too many functions and structures and programming constructs that need to be detailed to make you into a decent Python user. What I will try to do however is apply the 80/20 rule here, also called the Pareto principle. Since we are learning Python for the purpose of programming microcontrollers with CircuitPython, I can omit a lot of things and still have you understand just enough to follow along with this book.
For that reason, we will learn the 20% of the language that you will need to know to work with 80% of the tasks you are likely to do with CircuitPython. This chapter will thus present a subset of the core language, covering in brief the most important aspects of the language. If you have experience programming with Python, you may think I should have added one or the other. The subset I cover here though is enough so that anyone coming from an Arduino or C background, for instance, will be able to pick up easily.
Writing Python Programs
Python programs are usually written in text files that carry a “.py” extension. Python comes in two versions which are Python 2, the legacy version of Python, and Python 3 which is current and well supported. For most purposes, we will focus on Python 3 since this is the version used in CircuitPython. Should at any point you feel the need to run any programs that are present in this chapter, you can use the Mu editor.
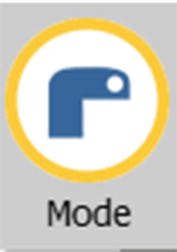
Mode Button

Python 3 Option
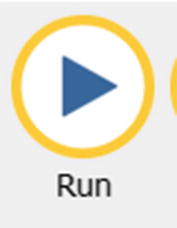
The Run Button
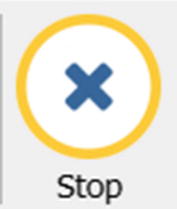
The Stop Button
Now that you know how to run Python programs, we can look at using the Python language.
Basic Python Program
In Python, we access methods which are blocks of code that only run when they are called by something called the dot notation. Our math module has a method called square root we would like to use, so we call the “math.sqrt” method. There is also a print statement. The print statement is used to output things to our display that we can use to get information from our program. This basic structure will be maintained for all Python programs, as you will see in later chapters going forward.
Whitespace
Something that should be addressed is that of whitespace in your Python programs. When we talk about whitespace, what we are really talking about are invisible characters such as tabs, blank space, newline characters, and the like.
Whitespace is ignored by some programming languages, but in Python whitespace plays a special role. Whitespace is used to structure your Python programs. While many programming languages use curly braces to indicate where blocks of code start and end, in Python whitespace is used to indicate blocks in the program. Pay attention to whitespace as it is particularly important in your Python code. The programs in this book are fairly short and don’t have much levels of indentation; however, you should still pay attention to the whitespace in your programs.
Comments
The comment is the text within your Python program that is there for the benefit of the programmer, as they are ignored by the interpreter. Good programming practice dictates that you include enough comments so that other programmers reading your code will be aware of what the program does. Comments will also remind you what your code did as you may have to update or maintain your code sometime in the future.
Though comments are necessary, it is important not to abuse comments and put too much. As in everything in life, it is important to have balance, so use comments, but don’t overuse them.
Single-Line Comment
Multiline Comment
Each comment type has their uses, and it is dependent on the code base you are maintaining or the team you are working with that will determine which comment style you will use.
Variables and Constants
Example Valid Python Variable Names
Example Invalid Python Variable Names
Reserved Keywords in Python
and | elif | if | or | yield |
as | else | import | pass | |
assert | except | in | raise | |
break | finally | is | return | |
class | False | lambda | true | |
continue | for | nonlocal | try | |
def | from | None | with | |
del | global | not | while |
When variables cannot be changed during program execution, we call these variables constants. In Python, we usually declare variables by writing them in capital letters and placing them in a constants.py file.
Declaring a Variable
Initializing a Variable
Declaring and Initializing a Variable
Data Types
Common Data Types
Data Type | Description |
---|---|
int (Int) | This is an integer or a number such as 10, 20, 100, 4000 |
float (Float) | This is a floating-point number or numbers with a decimal point, for example, 4.5, 60.9, 300.03, 1000.908 |
str (String) | A string is a set of characters between quotation marks (double or single), for example, “Andy” or ‘Maggie’ |
bool (Boolean) | A data type that can represent True or False |
To see the type of your variable, simply use “type(variableName)” and you will see the type of your keyword.
Operators
Common Python Operators
Operator | Type | Description | Usage |
---|---|---|---|
+ | Arithmetic | Adds two operands | X + Y |
- | Arithmetic | Subtracts the second operand from the first | X - Y |
* | Arithmetic | Multiplies both operands | X * Y |
/ | Arithmetic | Divides the numerator by the denominator | X / Y |
% | Arithmetic | The modulo operator gives the remainder of a division operation | X % Y |
** | Arithmetic | This is the exponent operator and performs power calculations | X**Y |
== | Comparison | If the two operands are equal, then Python will evaluate the condition as true | A==B |
!= | Comparison | If the two operands are not equal, then Python will evaluate the condition as true | A != B |
> | Comparison | If the operand on the left is greater than the operand on the right, then the condition is true | A > B |
< | Comparison | If the operand on the left is less than the operand on the right, then the condition is true | A < B |
Lists
Example List in Python
Get Index of List Item
Keep this in mind that the elements in a list start at zero as we move forward.
Tuples
A list as we learned in the previous section can store a collection of data types. However, sometimes you need another way to structure your data. For such a scenario, we can use the tuple.
Python Tuple
Access Items of Tuple
If Statement
if Statement
else Statement
else Statement
elif Statement
else if Statement
short if
One-Line if
for Loop
for Loop
Remember that Python counts from 0, so when the preceding statement is run, it will print numbers from 0 to 9 in the console, not 10.
while Loop
while Loop
The while loop is used in many embedded applications to keep a program running indefinitely, and when it does so, we call it a super loop.
Functions
Sometimes, within your Python programs, you may find yourself having to run the same block of code over and over. Instead of typing the same block of code multiple times, there is a feature in Python called a function that lets you call a block of code you have written and returns a value. These functions can take arguments on the input and then do something with them. If you need to return a value from a function, you would use the return statement.
Functions
Lambda Functions
Lambda Functions
Exception Handling
Exception Handling
Object-Oriented Programming
Python is what is known as an object-oriented programming language. What this means is that we can create special bits of code that act as a blueprint by which we can create other code. We call this special blueprint code a class. The formal name for this blueprint is an object constructor, and we use it to create instances of the class. We can also have functions that can exist within a class, and when we do, they are called methods.
Within Python, there exists a special method within the class that is called every time we use it to create an object called the __init__() method. In Python, we can modify instances of the class before it has been called with “self”; using self, we can access attributes and methods of the Python class that will be done to the object once it is initialized.
Python Class
We can print all the attributes of the class with the (__dict__) which is itself an attribute that objects have which contain all attributes defined for the object itself.
In Python, we will use many classes and their methods throughout this book; this little crash section in objects will be enough to take you through the rest of the book.
Random and Time
Using Random and Time
Python vs. CircuitPython
The most widely used distribution of Python is a variety we know as CPython. CPython is the “gold standard” implementation of the Python programming language because it is known as the reference implementation of the language. CircuitPython aims to be compliant with CPython; however, due to obvious reasons of lack of memory on the microcontrollers and for ease of use, some libraries that are available in CPython may not be available in CircuitPython. Most things from the core language do work however, and code written in CircuitPython will be valid in CPython but not necessarily the other way around. The core language however is there, and you can write a lot of amazing programs with CircuitPython.
How Does My Python Program Run?
If you are not familiar with microcontrollers and programming, you may be wondering how a Python program goes from your editor such as Mu into the microcontroller to be executed. So, in this section, I give a high-level overview of what happens when you run your Python program.
Python is what is known as an interpreted language, so what this means is that it takes each statement that is present in our source code and converts it into machine language our microcontroller can understand when the program is run. This is contrasted with a compiler that transforms code written into machine code before the program is run.
Initialization
Compilation
Execution
Before we begin looking at how our Python program is run, we must remember that the Python interpreter is written in C, and as such it is merely a program that is run in our C code. In the initial stage, when we write our program and load it into the C program, the program looks for the Py_main program which is the main high-level function within the main program. Other supporting functions that handle program initialization and the like are then called.
After this stage, we enter the compilation stage where things known as parse tree generation and abstract syntax tree (AST ) generation take place to help generate the bytecode. These bytecode instructions aren’t machine code; rather they are independent of the platform we are using. The bytecode is then optimized, and we get the object code we need to run. In CircuitPython, we have the option to make our code into bytecode before the program is run, and such files are given a “.mpy” extension. These files can be imported like regular files and are more compact, leading to more efficient memory usage.
Finally, our program is executed within the Python Virtual Machine (PVM). The virtual machine is a function within the interpreter program that runs these bytecode instructions. It’s just one big super loop that reads our bytecode instructions one by one and executes them.
Conclusion
In this chapter, we briefly looked at Python and some of the features of the language. Once you read this chapter, you should have a basic understanding of the Python programming language. Programming is something though that you learn over time, and as such it is important that we write code. Only by writing code will you learn the language you are using, and for that reason, in the next chapter, we will look at how we can use Python to control our microcontroller hardware.