Creating the structure of your application is an important step. In this chapter, I will detail how to use a multilayered architecture to separate concerns in the application. This will make your application easier to maintain and extend.
Core Project
The Core project is where the commonly used code shared across the entire application will reside. This is where the entity classes used by Entity Framework will live. This is also where common interfaces and utility code will exist.

New Class Library
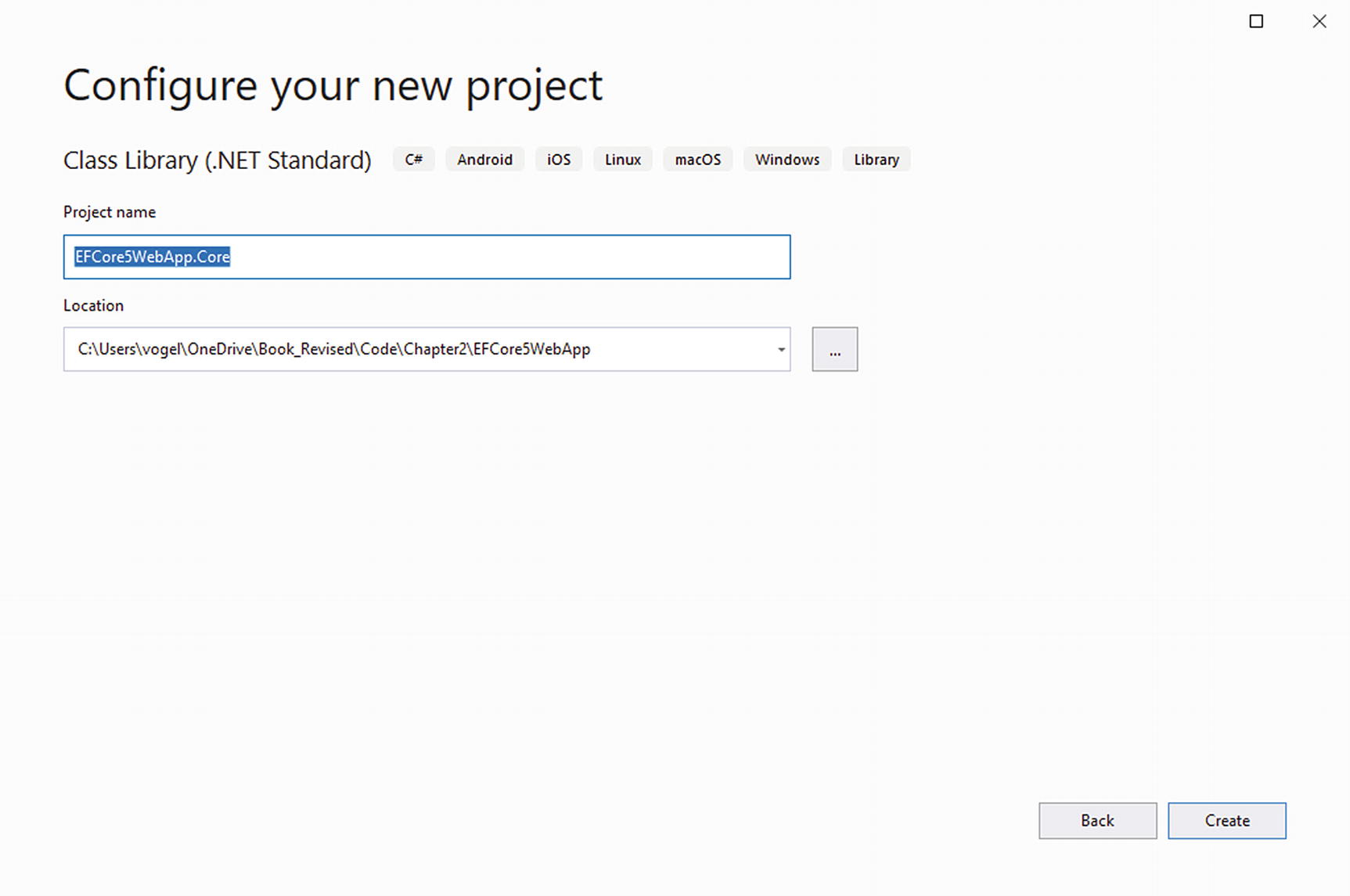
Naming the Core Project
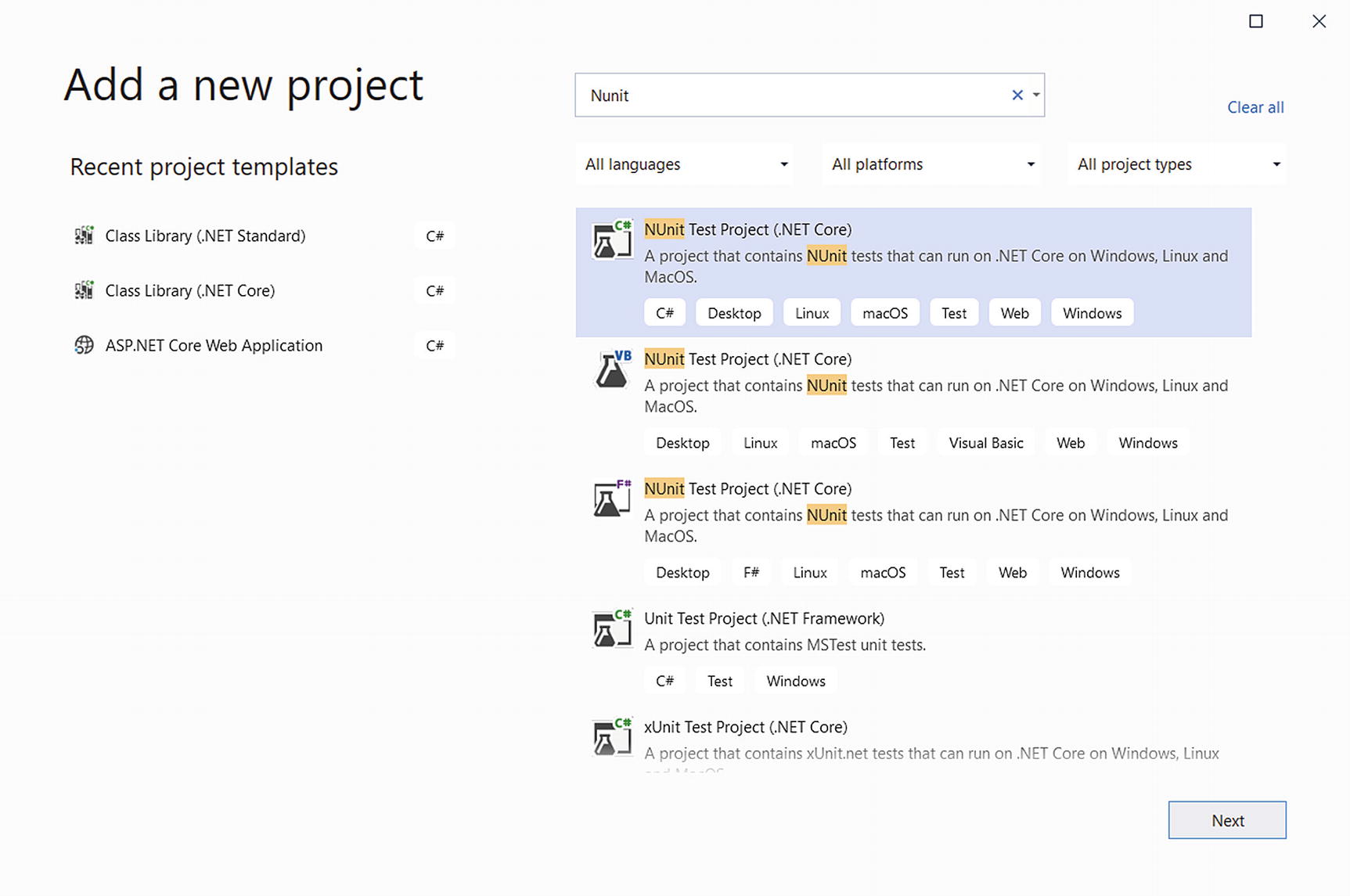
Adding a NUnit Project
After adding the Core.Tests project, add a project reference to the Core project to it.
Unit Testing Overview
NUnit is a commonly used unit testing framework. Unit tests have become popular with the whole Test-Driven Development (TDD) movement, in which you create unit tests first and then write the code to make the tests pass. Later in the book, we’ll be testing that our Entity Framework Core 5 code works correctly before integrating it into our web project.
A unit test tests a small set of code, usually at the method level. You test the happy path as well as potential edge cases to make sure your code is solid before it becomes integrated into the rest of the solution.
Data Access Layer Project

New .NET Core Class Library
Then add a project reference to the Core project to the DAL project. After that, add a NUnit Test Project to the solution named “EFCore5WebApp.DAL.Tests”. Add references to the DAL and Core projects to the DAL.Tests project. After that, add Core project reference to the DAL and DAL.Tests projects. The DAL.Tests project will be where we test all of our Entity Framework Core 5 code before putting it to use in the web project.

Move EF Core 5 Packages to the DAL Project
Main Project References
Lastly, we need to add project references to the ASP.NET Core MVC application project named “EFCore5WebApp”. Add project references to the Core and DAL projects to the root “EFCore5WebApp” project. This will ensure we can use the services and entities from our ASP.NET Core web app.
Summary
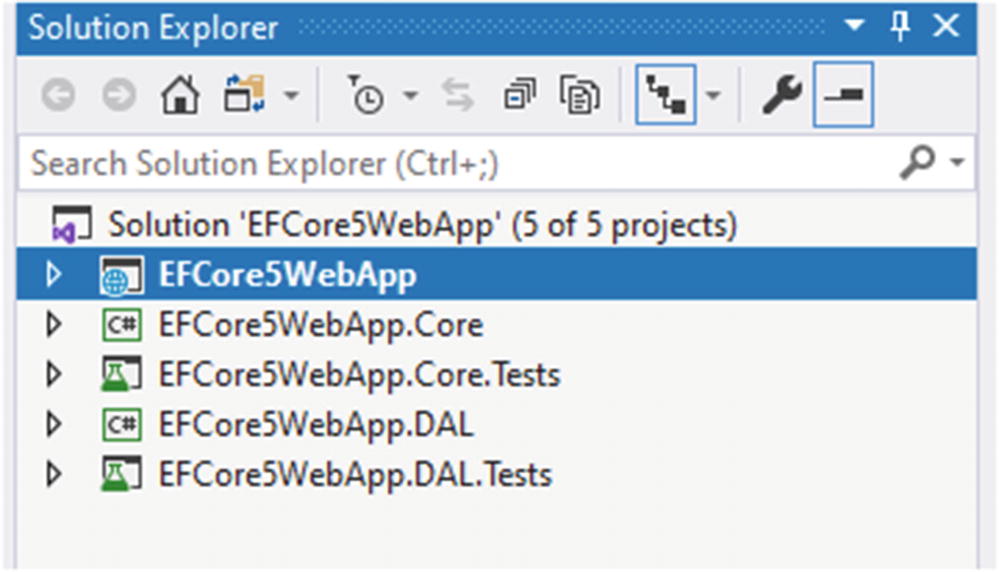
Project Structure
By separating your projects by application concerns, the project will be easier to maintain and extend over time. We’ve also architected the solution with unit testing support. This will speed up development time by making it easier to safely make changes to the application without worrying about introducing new side effect bugs, and functionality will be regression tested during development time.
In the next chapter, I’ll cover how to create the entity classes that will later be used by Entity Framework Core 5 in Chapter 4. Entity classes are used by Entity Framework Core 5 to map to tables in your database.