You have your computer ready now, so let’s start programming! In this chapter, you will create your first program in the C# language and learn all the steps that you need to perform to do this.
Seeing It in Action

Your first program
Creating the Project
You start every new program by creating a new project, so let’s do that now.
Launching Visual Studio

Visual Studio Start screen

Choosing for a new project

Selecting a project template
Creating New Project
The dialog that appears (see Figure 2-4) requires you to choose a new project template. Select the Console App C# template and press the Next button.

Entering a name for your new project
Writing the Program Code
The most important step is writing the program’s code, so read on.
The Look of the Development Environment
The main part of the development environment window is occupied by the source code editor. In it, the Program.cs file is opened, as is suggested by the tab’s title. Program.cs is the main file of your new Console project. As you can see, it already contains some source code.
You might wonder where this code came from. You haven’t written any line of code yet! The answer is that Visual Studio generated the code when you selected the Console App template. As you saw when creating the project, Visual Studio contains many different templates; these templates are ready-made project skeletons for different types of programs.
You can see that the code contains some strange words like using, namespace, class, and so on. I am not going to explain these now because you do not need a detailed understanding of them at this time. But Visual Studio needs these lines, so just leave them alone. What you do need to know is where to write your own statements, which is what I will explain next.
Knowing Where to Write Statements

Where you write your statements
In all previous Visual Studio versions, the space between curly brackets was left blank. Now the IDE creators decided to include a single line of code which you may delete or modify subsequently.
Writing the Code

Entering your first code statements
Please double-check that you typed the statements in the same place as I did. Again, they have to be between the brackets. Also, be careful of the brackets. Do not accidentally delete any of them.
Understanding Your First Statements
Console.WriteLine outputs (writes) a single line to the user.
Console.ReadLine, in general, reads a line of text that the user enters with the keyboard. In this case, however, the purpose of the statement is to make your program wait for the user to press Enter when everything is done. In other words, you do not want the program window to disappear immediately.
Everything following the two slashes (//) until the end of a corresponding line is ignored. This text contains your remarks/comments. Visual Studio colors them in green.
Using IntelliSense

Using IntelliSense
The part of Visual Studio that provides you with these hints is called IntelliSense. Get used to relying on it as much as you can. It is the best way to avoid unnecessary typos.
Saving the Project
You have written several lines of code, so you probably want to save them. According to the default settings of Visual Studio, projects are automatically saved before every program launch. However, sometimes you want to save the changes manually. In that case, choose File ➤ Save All from the Visual Studio menu, or click Ctrl+S.
Launching Your Program
Having written your program, you usually want to launch it to see it “in action” and to check whether it does what you meant it to do.
Prepare yourself. The great moment of your first program launch is coming! Choose Debug ➤ Start Debugging from the Visual Studio menu, or just press the F5 key.
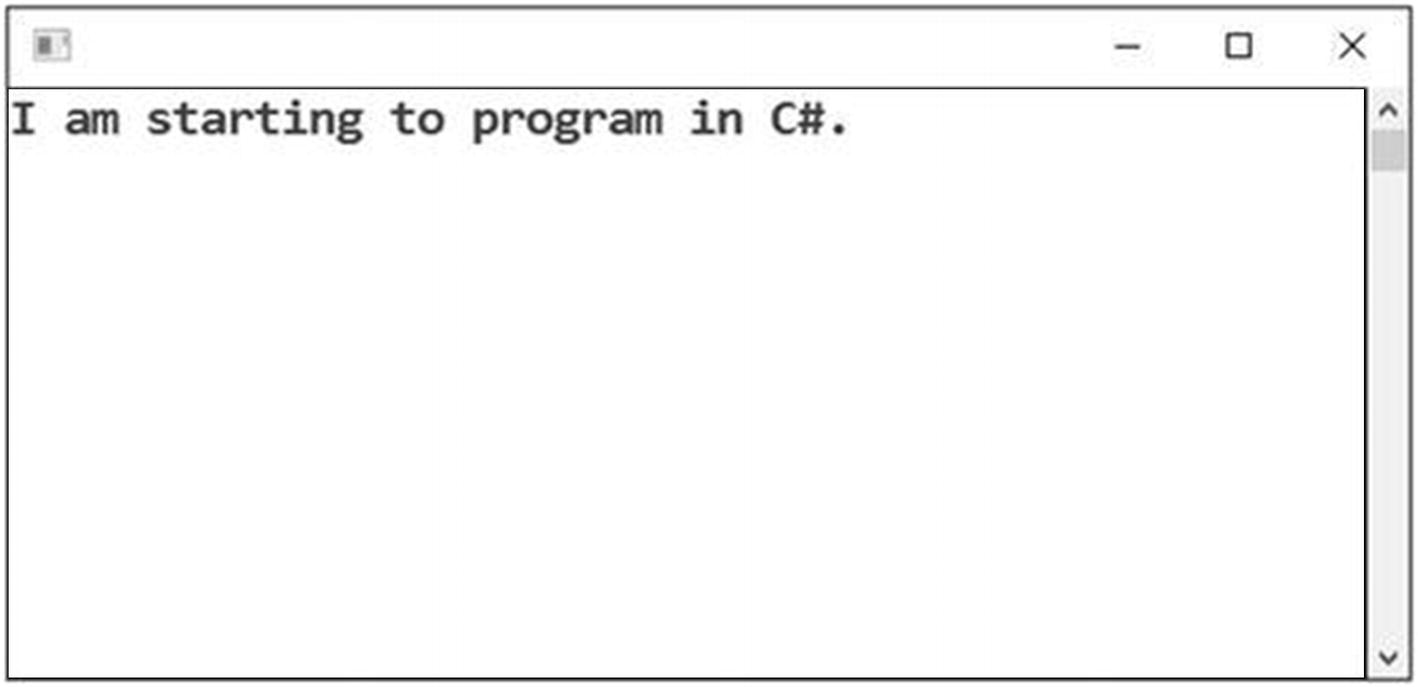
Launching your program

The added measure preventing the window from disappearing
Since Console.ReadLine() prevents the window from disappearing also when running outside of Visual Studio, I will be including this statement in all our programs. If you want, you can disable the new Visual Studio measure according to the instructions given in the message of Figure 2-11.
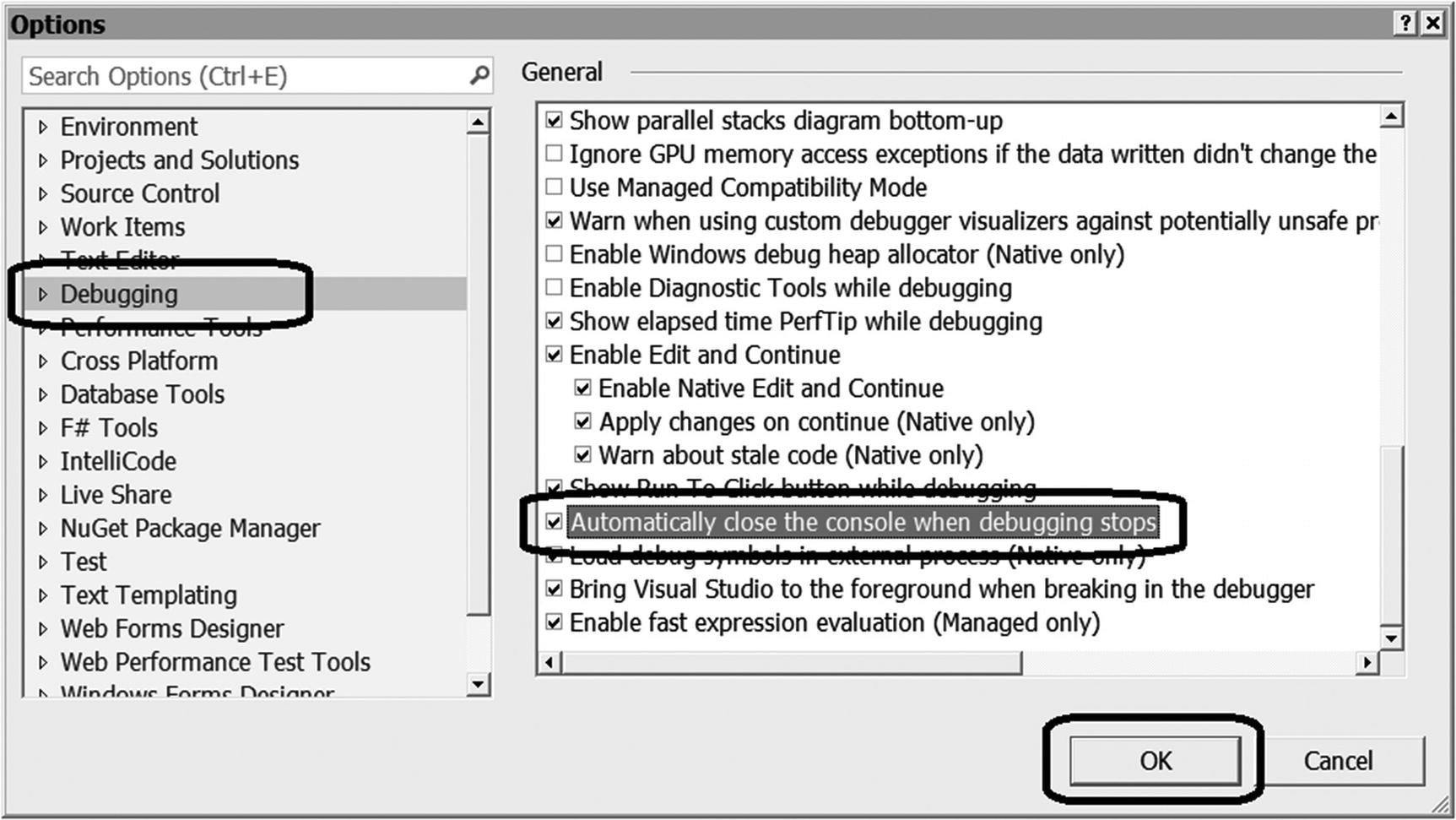
Disabling the new measure
Note
With the default settings of your computer, your programs will appear with white type on a black background, as you can see in Figure 2-10. However, for better readability, I will show all the later screenshots in black type on a light background (see Figure 2-11).
Changing Text Size
Do you think the outputted text is too small? Do you need to enlarge the font your programs will use?

Choosing Default settings
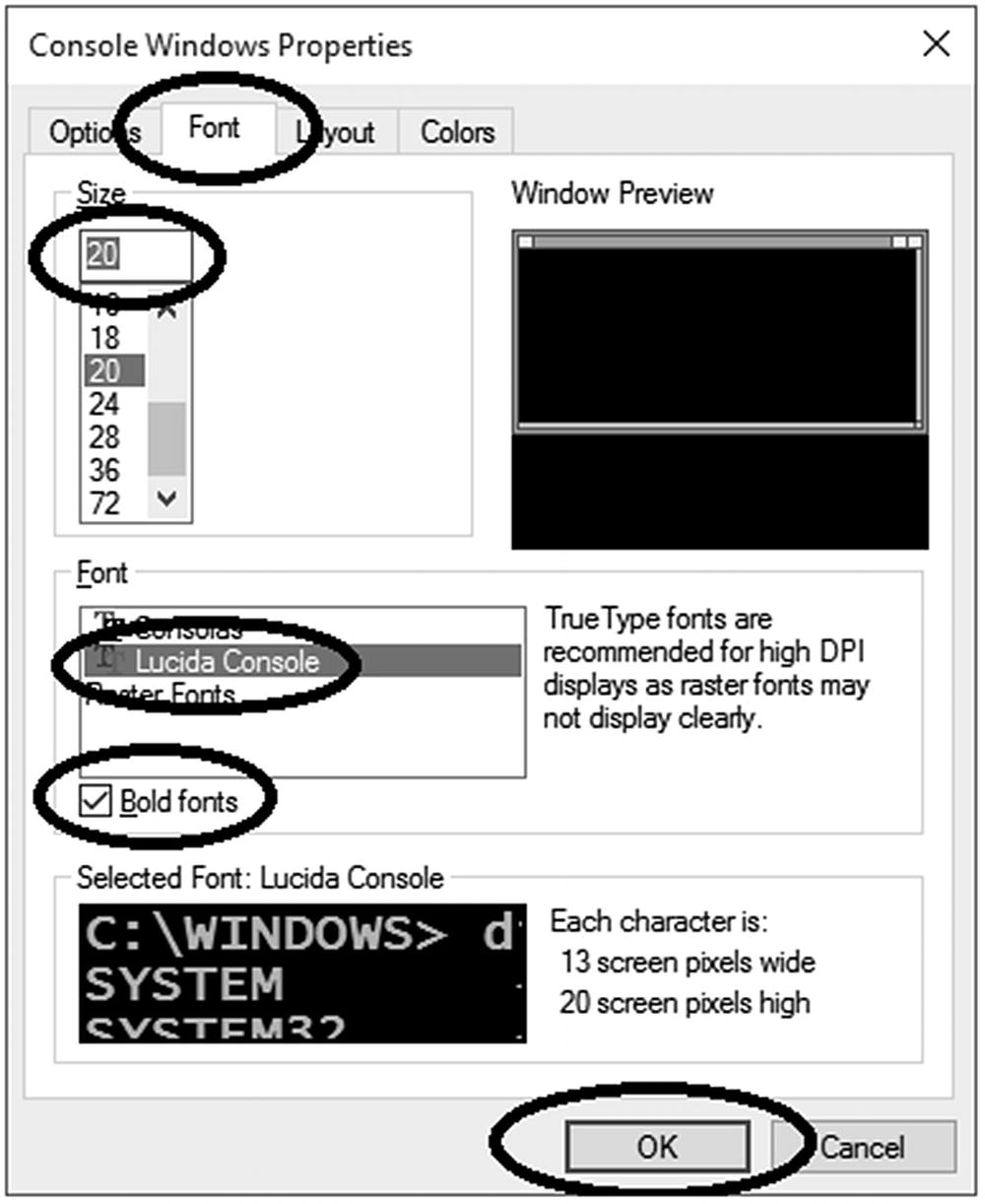
Changing the font
When the next program launches, the new font will be used.
Dealing with Errors
If you did not write the statements exactly the way I showed you or you wrote them in the wrong place, the program build would terminate unsuccessfully with errors.
Let’s try this! Delete the semicolon at the end of the line with the Console.WriteLine statement.

Getting an error
In this dialog, always click No; you do not want to run some older version of your program (if it exists).

Error list
Return the deleted semicolon, and everything will be fine again. In the future, it may be more difficult to find what you did wrong, especially at the beginning of your programming career. That’s OK—my opinion is that you can’t become an expert in a field until you have made all the possible mistakes there are.
Finishing Your Work
You have just gone through all the essential steps of program development. You will proceed along the same lines in every future project you do.
You now need to learn how to terminate your work and how to get back to it later. The former is simple; you can finish your work on this project by choosing File ➤ Close Solution from the menu or by closing the whole Visual Studio program.
Restoring Your Work
From the Start Page: This is the page that appears immediately after Visual Studio starts and contains links to your recent projects (see Figure 2-17). Simply click the right one.

Using the Start Page
From the Open Project dialog: Select File ➤ Open ➤ Project/Solution from the Visual Studio menu. The Open Project dialog appears in which you should locate your project (see Figure 2-18). Specifically, look for files with the .sln extension. If you cannot see the file extensions, turn their display on in Windows File Explorer (on the View tab, select the “File name extensions” check box), as shown in Figure 2-19.

Opening your program with the Open Project dialog

Showing extensions
From the Recent Projects menu: Select File ➤ Recent Projects and Solutions. Visual Studio remembers what project you were working on recently. Just choose the appropriate project (see Figure 2-20).

Opening your project from the File menu
Transferring Your Work
You may also be interested in how to transfer your project to somewhere else from your computer, so you can work on it later on a different computer. The answer is simple. If you’re using a flash drive, OneDrive, or something similar, just transfer the project’s whole folder.
Using Solution Explorer

Opening a project without a source code editor

Opening your source code via Solution Explorer

Opening Solution Explorer
Summary
Creating the project
Editing the source code
Saving the source code
Launching the program
Detecting and removing possible errors
Transferring your project onto another computer of yours to work on it elsewhere