Contiki-NG uses the C programming language to develop applications for WSN motes. In this chapter, you will learn this basic language for creating Contiki-NG programs. Program samples are provided to accelerate your learning speed.
Contiki-NG programming model
Contiki-NG basic syntax
reviewing protothreads
extending the Contiki-NG library
Contiki-NG coding conventions
showing a demo for building a Contiki-NG application
Contiki-NG Programming Model
Contiki-NG uses the C programming language, which is component-driven. You build some components and then connect each component. Most WSN motes work in sleep mode. If there is any task to be executed, the program will perform the task through hardware interrupts. If the task is completed, the Contiki-NG program will go back to sleep mode.

Programming flow of a Contiki-NG program

Contiki-NG program structure
Information for Contiki-NG OS Folders
Folder | Description |
---|---|
arch | Contains CPU, platform, and dev for development |
arch/cpu | Specific MCU information |
arch/dev | External chip and devices |
arch/platform | Specific files and platform drivers |
os | Contiki-NG core files and libraries |
tools | Tools for flashing, debugging, simulating |
examples | Several Contiki-NG program samples |
tests | Several test programs |
In the next section, we will review the basics of C programming, such as the language syntax used to develop Contiki-NG programs.
Contiki-NG Basic Syntax
In general, Contiki-NG adopts the C programming language. In this section, we will review some C programming language basics. You can use any text editor to write C code.
Creating a Project

A collection of Contiki-NG project samples
You also can use program samples from the Contiki project ( https://github.com/contiki-os/contiki ). You should check if the one you choose is compatible with the Contiki-NG project ( https://github.com/contiki-ng/contiki-ng ).
Contiki-NG Basic Programming Language
Basic Programming for C Language
C Basic Programming | Example |
---|---|
Declare variables | int num; int_t a, b, c; unsigned int isDone; |
Assign variables | num = 3; isDone = 0; unsigned int m = 10; |
If-conditional | if(running){ doSomething(); }else { perform(); } |
Looping | int len = 10; for(int i=0;i<len;i++) { foo(); } |
Comment codes | // this comment /* this is also comment */ |
To improve your skills in the Contiki-NG programming language, I recommend you practice writing Contiki-NG programs.
Review Protothreads
Protothreads are lightweight threads designed for memory-constrained systems, such as small embedded systems or WSN nodes. You can see a protothreads implementation for Contiki-NG at <contiki_root>/os/sys/pt.h.
The following is the content of the pt.h file:
PT_INIT(pt) function is used to initialize a protothread.
PT_THREAD(name_args) is a macro that is used to declare a protothread.
PT_BEGIN(pt) is used to declare the starting point of a protothread.
PT_END(pt) is used to end a protothread.
PT_WAIT_UNTIL(pt, condition) is used for blocking the protothread until the specified condition is true.
PT_WAIT_WHILE(pt, cond) is used for blocking and waiting while the condition is true.
PT_WAIT_THREAD(pt, thread) is used to schedule a child protothread. The current protothread will block until the child protothread completes.
PT_SPAWN(pt, child, thread) is used to spawn a child protothread and waits until it exits.
PT_RESTART(pt) will block and cause the running protothread to restart its execution at the place of the PT_BEGIN() call.
PT_EXIT(pt) is used to exit from a protothread.
PT_SCHEDULE(f) is used to schedule a protothread. The return value of the function is non-zero if the protothread is running or zero if the protothread has exited.
PT_YIELD(pt) yields from the current protothread.
PT_YIELD_UNTIL(pt, cond) yields from the protothread until a condition occurs.
Extending the Contiki-NG Library
Contiki-NG OS consists of several libraries and apps that you can extend based on your case. To extend Contiki-NG OS functionalities, you can follow the same approach used while building C programs.
You add additional libraries by adding a C header and source code. Our Contiki-NG program will consume our libraries. For this demo, we create a header file called mycounter.h with the following code:
This C header provides one function, next_counter(). This function will be implemented into the mycounter.c file. We perform a counterincrement with a maximum value of 99. You can write the complete code as follows:
To access the extended library, we declare that header file. Then, we call library functions from our code. The following is a skeleton code sample to access the header file in a C program:
Next, we will implement our extended library and use it in a Contiki-NG program.
Contiki-NG Demo: Threading App
mycounter.h
mycounter.c
demo-counter.c
Makefile

Project structure for demo-counter project
In this demo, we create a header file called mycounter.h. This C header provides one function, next_counter(), that generates an incremented number based on the input. The next_counter() function will be implemented into the mycounter.c file. In the previous section, we declared this library.
Next, we access mycounter.h in our main program. It’s implemented in the demo-counter.c file. We use an event timer library from Contiki-NG, etimer. We generate an incremented number every three seconds.
The following is the complete code for demo-counter.c:
We also create a Makefile to configure our compiling. You can write these scripts for the Makefile. Change the CONTIKI value with your Contiki-NG root folder:
Save the program.
For testing, we run this program with a native target. You can open Terminal and navigate to the project folder. Then, you can compile and run the program. Type these commands:
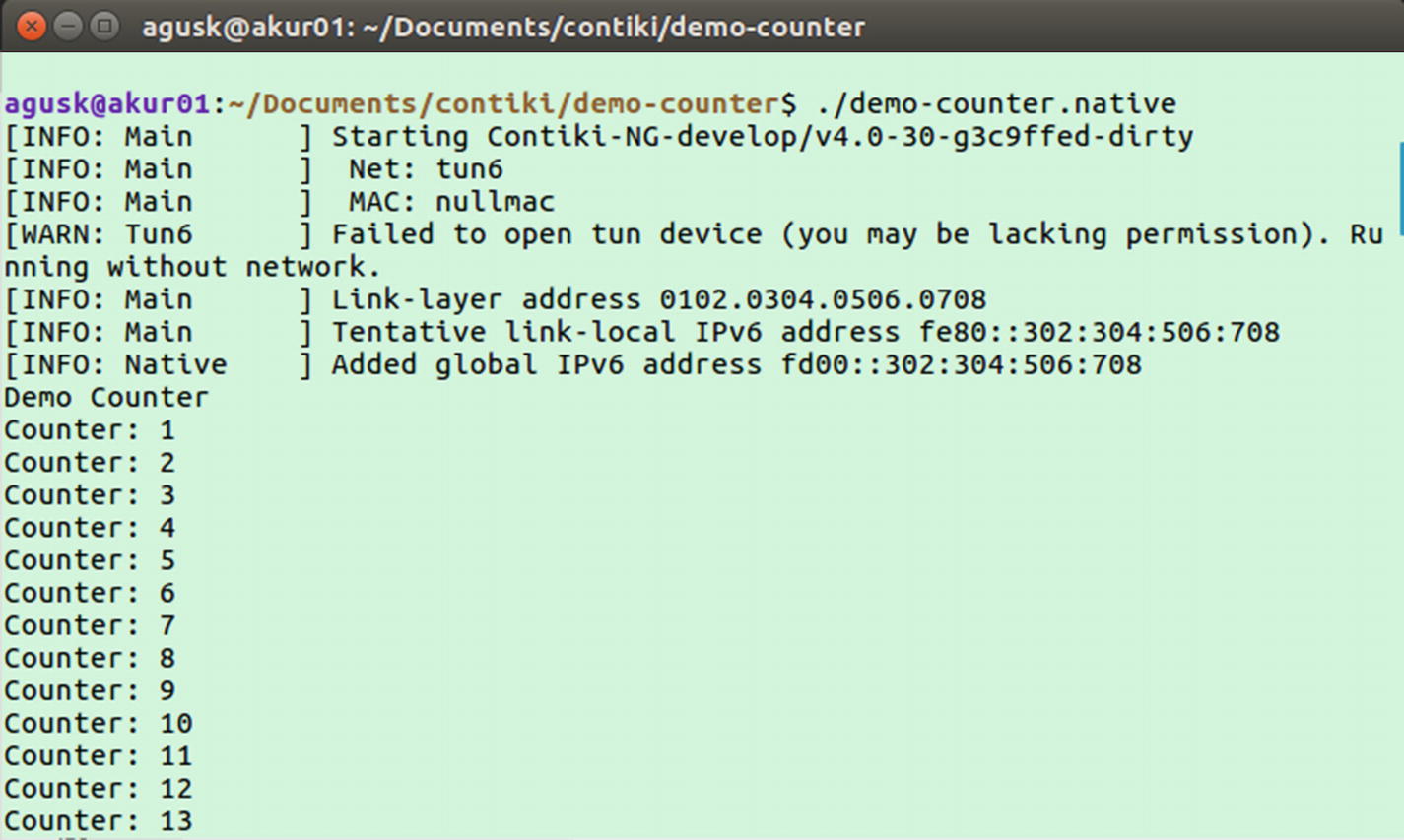
Program output for demo-counter
How does it work?
This program is simple. First, we declare our Contiki-NG process, counter_process. We also declare our event timer and number variable:
Inside the counter_process function, we perform looping with the event timer. After raising an event, we call the next_counter() function to get an incremented number. Then, we print this value in Terminal:
Contiki-NG Coding Conventions
Imagine you’re developing a program with a team of more than three developers. Each developer has a programming style and method. When the codes are merged, it may raise problems since the codes are not consistent in writing style. This problem could be solved if the code was written in the same style.
Contiki coding conventions are rules for a writing style. They consist of guidelines for how to write Contiki-NG programs. You can read the full coding conventions for Contiki at https://github.com/contiki-os/contiki/blob/master/doc/code-style.C .
Demo: Build Contiki-NG Application

A simple library for virtual sensor
mysensor.h is a header file that declares read_temperature() and read_humidity() functions.
mysensor.c implements read_temperature() and read_humidity() functions.
demo-sensor.c is the main program that uses mysensor library.
Makefile is used for compiler parameters.
Now, we implement all files, starting with mysensor.h. It's a header file that exposes two functions:
These sensor functions will be used as a general sensor that is implemented for temperature and humidity in the mysensor.c file. The following is the complete code for mysensor.c:
We generate a random number for our functions. Moreover, we use the Sensor library in our main program, demo-sensor.c. We use an event timer for the reading period. The following is the complete code for demo-sensor.c:
Last, we create a Makefile for compiler parameters. We set PROJECT_SOURCEFILES with mysensor.c. Change the CONTIKI value to your Contiki root folder. The following is the content of the Makefile:
Now, we can compile and upload the program into the WSN mote. We can compile and run it for targeting native. Open Terminal and navigate to the project folder. Then, type these commands:

Program output for demo-sensor application
Summary
We have explored the Contiki-NG basic programming language. This is a core language based on the C programming language that will help you to develop a Contiki-NG application. We built a Contiki-NG application that utilized a header library and event timer with Contiki-NG program features.
In the next chapter, we will learn how to work with concurrency in Contiki-NG programs in order to deal with multiple jobs.