You have seen an object act as a data conglomerate, or container. You have seen the data it contains as its properties that can be accessed after appending a dot to the object’s name. Accessing a property means either questioning its value or assigning a new value to it.
You have also discovered that (possibly many) actions can be associated with an object. Actions are called methods, and like properties, they can be accessed after appending a dot to the object’s name. Moreover, accessing a particular method of an object requires you to add a pair of parentheses to the method’s name, with possible parameter values inside them. Accessing a method (usually you would say calling it) means to launch the operation it implements and to execute the statements it contains inside (without you knowing them).
These are the tenets of programming with objects, the basics of which you studied in the previous two chapters. This chapter will round out your knowledge of objects by exposing you to further insights.
Let’s dig into objects a bit more now.
Text as an Object
In C#, even ordinary text behaves like an object; you can add a dot to text and get plenty of possibilities. Let’s take a look.
Task
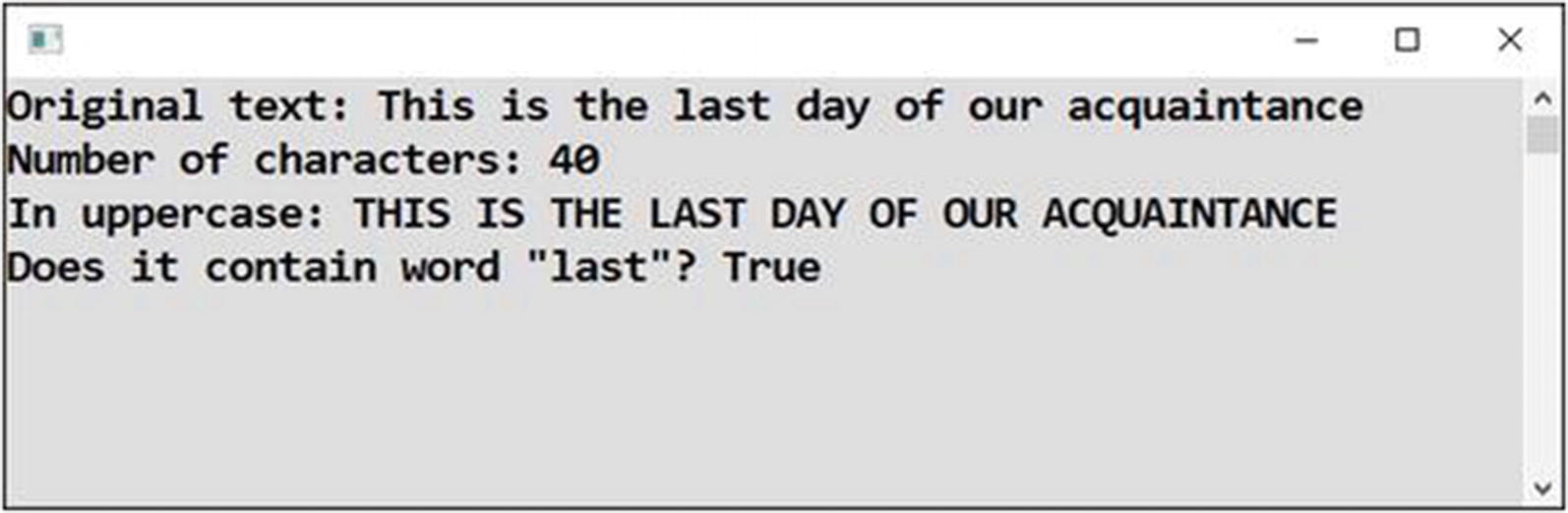
The final program
Solution
Discussion
Data members (e.g., Length) are not accompanied by parentheses, contrary to methods (such as ToUpper, Contains), which always need parentheses even if there is nothing between them.
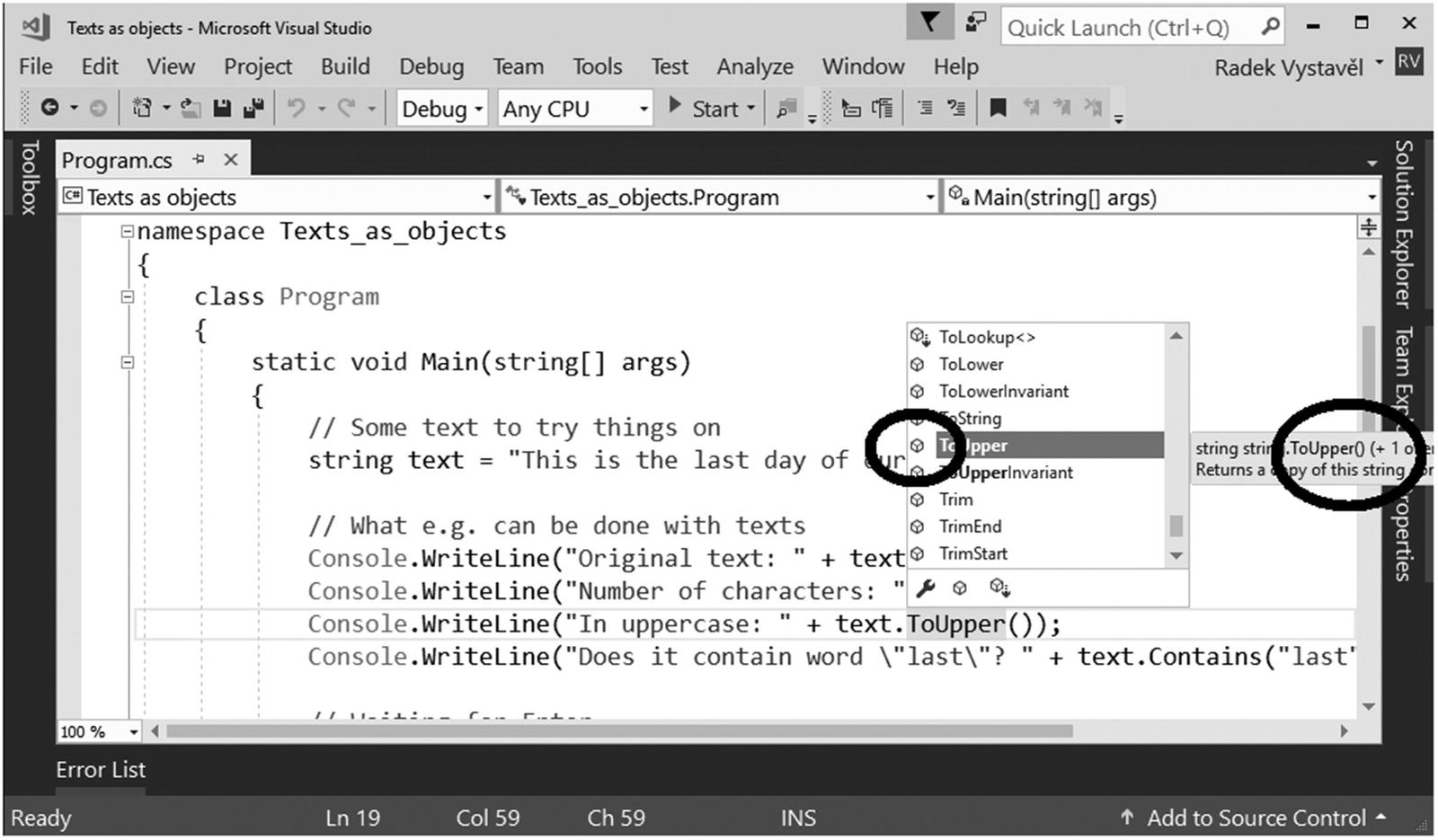
Checking whether something is a method
Numbers as Objects
In the previous exercise, you saw that ordinary text—a value of type string—can behave like an object and show internal components such as properties and methods. Now you will see that even numbers can behave like objects, although their actions are much sparser. Actually, the only one worth mentioning is the action of converting to text.
Task
You will explore what pops up after appending a dot to a numeric variable, and you will learn how to convert from a number to text.
Solution
Discussion
You can see that a value of type int cannot be directly assigned to a variable of type string. You have to convert it to text form first.
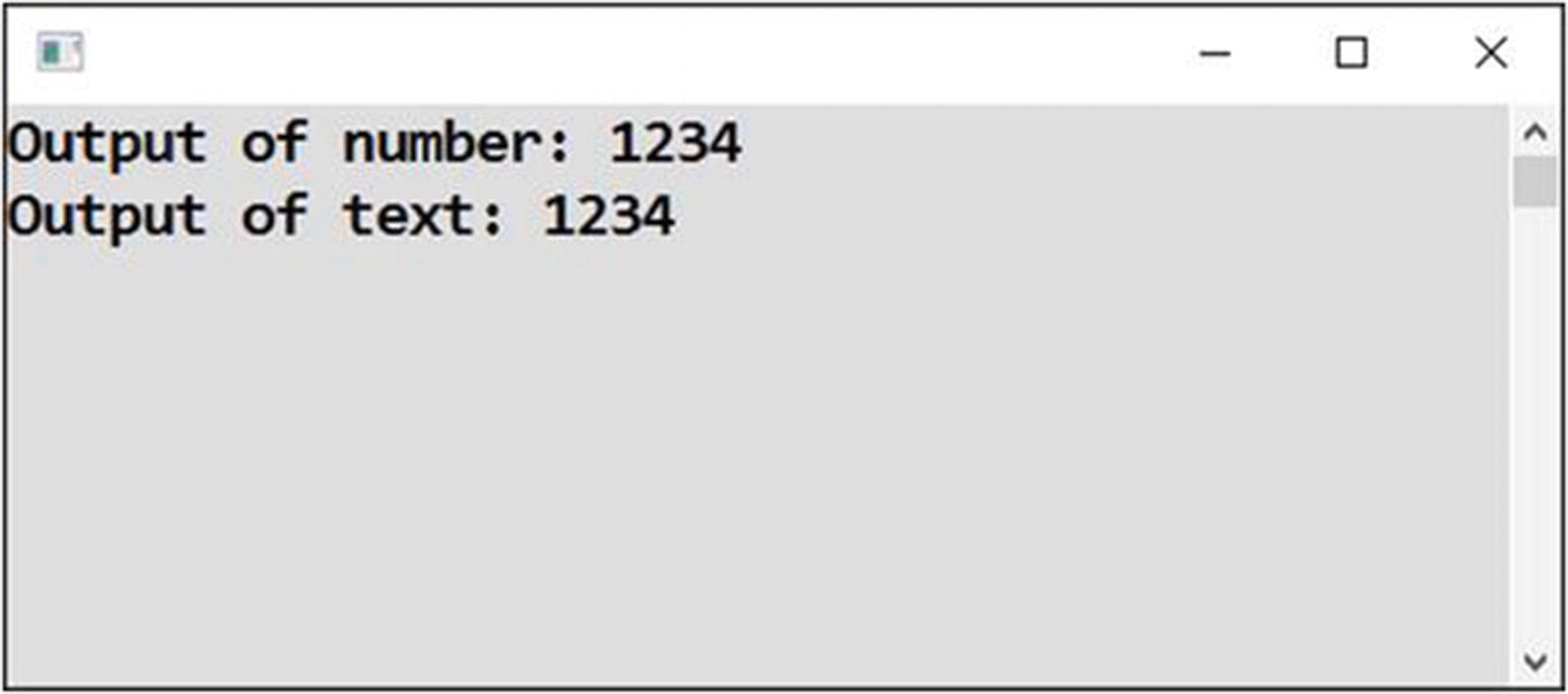
The output
However, many times you will need to convert a number into text without immediately displaying it. Then you will store the text form of the value in a string-typed variable, which is what you have just seen.
The Console.WriteLine method converts everything it gets into text. It does this silently using the ToString conversion behind the scenes.
If you join some text with a number using the plus sign, the number gets automatically converted to text in C#. If you desire greater control, always write down .ToString() in connection with numbers.
Formatting Numbers
In the previous exercise, you were busy with converting numbers into their textual representations. However, there are multiple ways that a single number can be expressed in a text form. You will now learn about decimal places, rounding, thousands separation, and so on.
Task
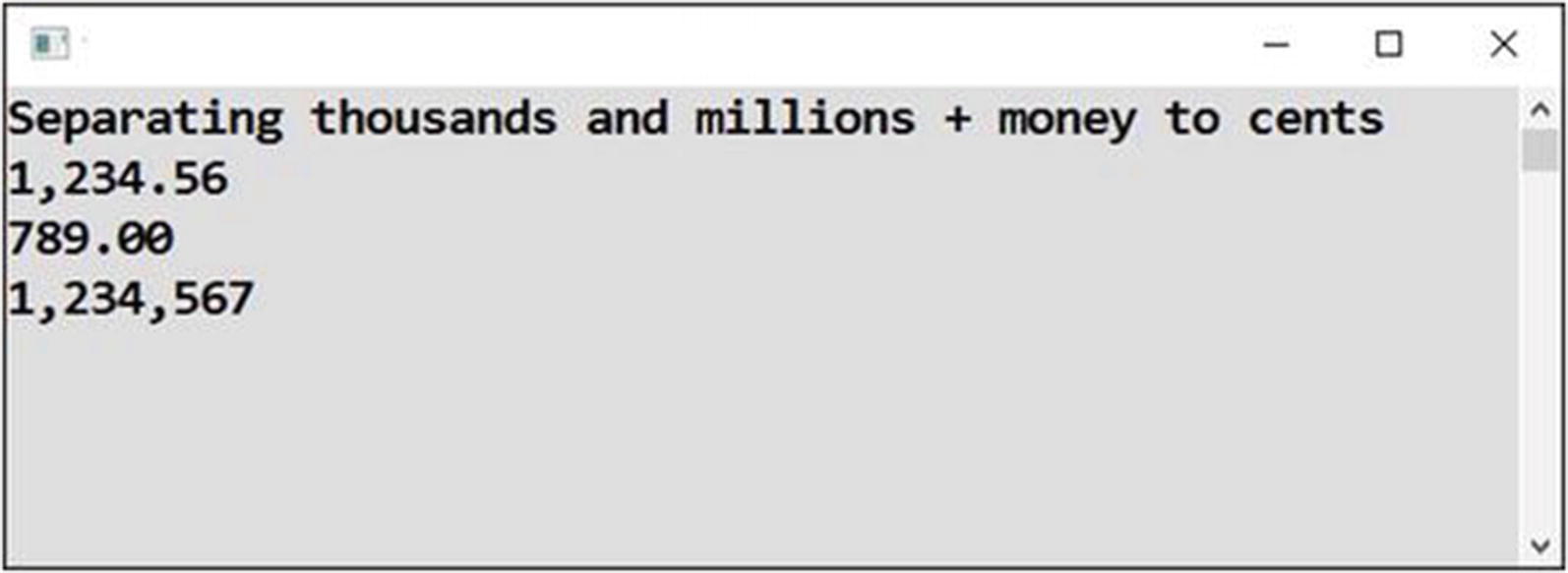
Nicely formatted output
Solution
Contrary to the earlier exercise, the ToString method call now has a parameter between parentheses. The format string specifies the way the output should look.
In the format strings used here, N means thousands separation is required, and two and zero denote the number of decimal places in the output.
Localized Output
Ordinary number formatting (like in the previous task) works according to the Windows language setting. However, sometimes you do not want the output to depend on user settings. You may want a fixed-language setting, such as American, Czech, or whatever.
Task
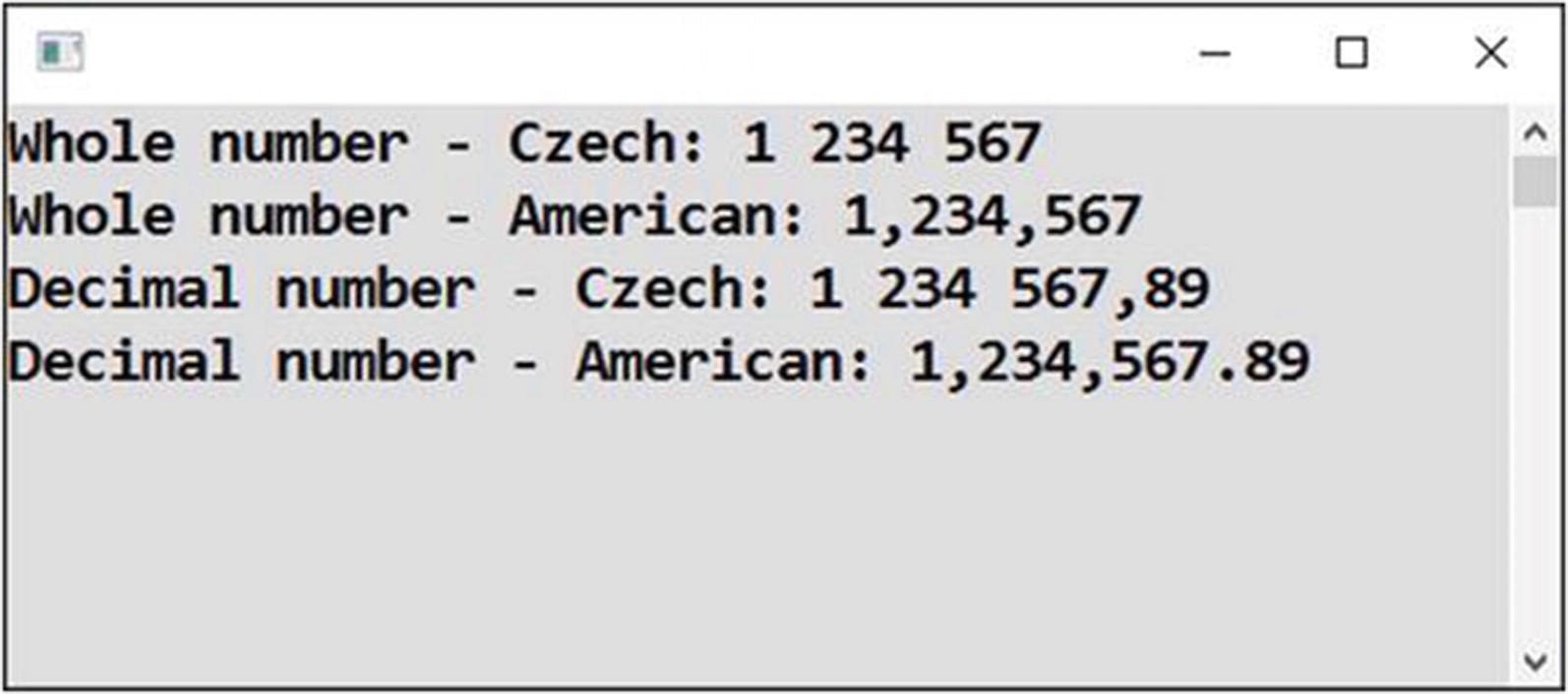
Two different number styles
As you can see, in the Czech language, you use a space as the thousands separator and a comma as a decimal separator. The same comma is used as a thousands separator in American formatting, so you can imagine that letting a computer decide what language to use (according to Windows settings) can sometimes lead to a confusion and incorrect program behavior.
Solution
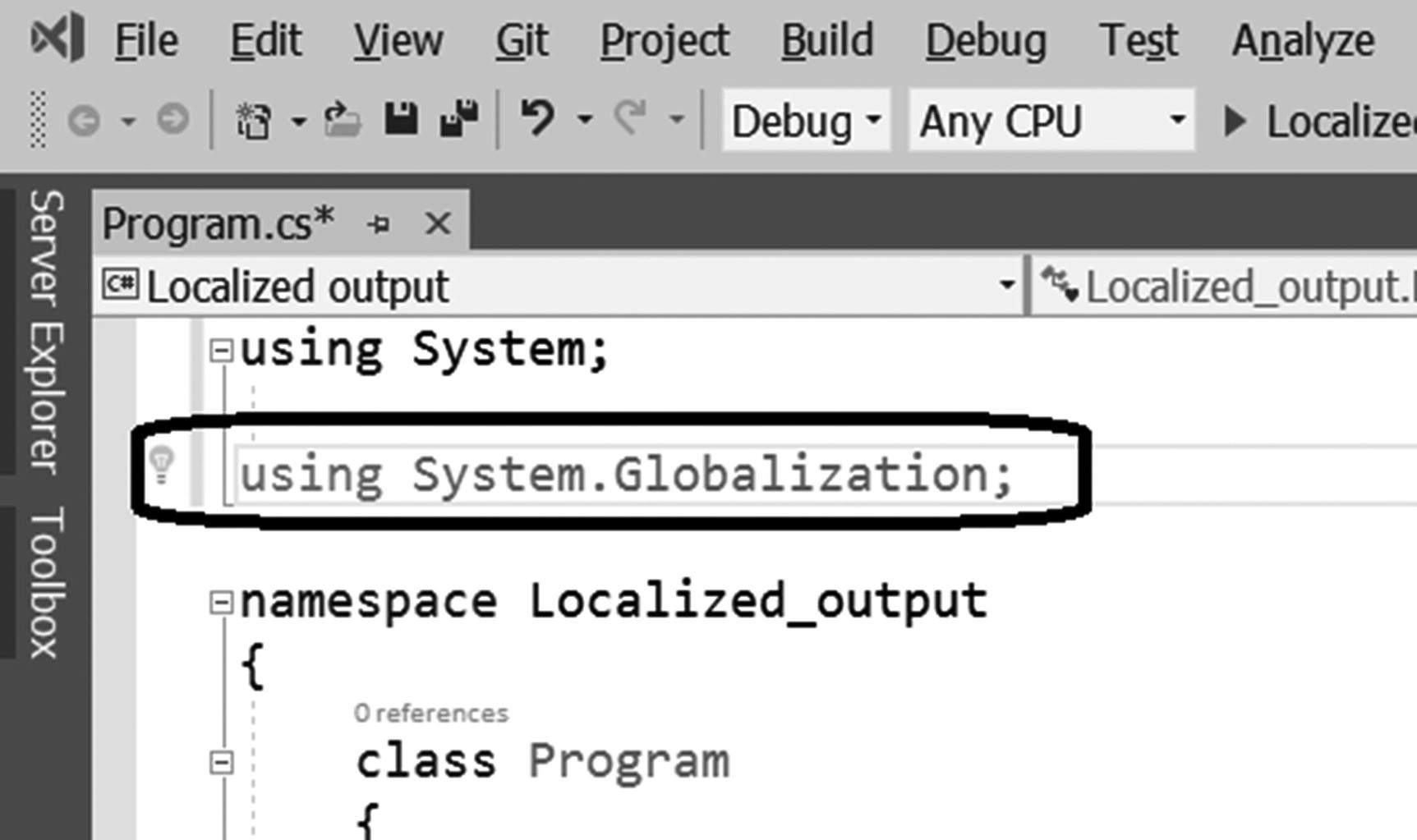
Adding a using line
Concluding Notes
To finalize your knowledge of objects within the scope of this book, I will introduce you to some more object concepts. Please do not be worried if you do not understand them exactly right now. It is okay to just get an introduction to them at this stage of your study.
Static Objects
First, I want to draw your attention toward the existence of two kinds of objects. There are “classic” objects such as DateTime, Random, and CultureInfo, and there are “static” objects such as Console, Environment, and Math.
You can have as many classic objects as you want in your program. For example, you had two DateTimes in the variables today and tomorrow. You also had three Randoms in the variables randomNumbers, randomNumbers1, and randomNumbers2.
Contrary to classic objects, static objects are always single—you have just one Console, just one Environment, and also just a single Math.
Also, you always create classic objects on demand, while the static ones exist since the program’s start without any effort on your part.
Strictly using the official terminology, I should be talking about “classes with static components” rather than about “static objects.” However, I prefer the latter, beginner-friendly term. It’s better to be approximately beautiful than exactly ugly.
Classes
Every documentation or textbook dealing with objects abounds with usage of the word class . So, what does this mean? To put it simply, a class is a synonym for an object data type. Instead of “an object of Random type,” you can speak about “an object of the Random class.” This means a class can also be viewed as the name of a certain type of objects.
Relation Between Class and Object
From a different perspective, a class is also a C# source code defining what an object of a particular kind contains and how it behaves. You can also say that classes serve as templates for objects. For example, the Random class source code (which Microsoft has, not you) defines what properties and what methods all Randoms will have.
As a consequence, all Random objects behave in the same way because all of them are created from the same template, or from the same class. The same can be said about all DateTimes, all CultureInfos, and so on.
To put it another way, as a well-known maxim of object-oriented programming, an object is a class instance. The word instance means a single realization, or a single occurrence.
Special Classes
Data Type | Corresponding Class |
---|---|
string | String |
int | Int32 |
double | Double |
bool | Boolean |
... | ... |
In C#, you can use string and String interchangeably, int and Int32 interchangeably, and so on. Of course, you need a using System; line at the top of your source code since all the corresponding classes belong to that particular namespace.
Structures
In C#, you may encounter the term structure or struct, as well. What are structures?
Well, you can view them as something like lightweight classes. At a beginner’s level, they are almost indistinguishable from normal classes, so you may simply substitute the word class wherever you see structure or struct for a long time.
For example, DateTime is the prime example of a structure. However, for the sake of simplicity, everywhere in this book I treat DateTime on equal terms with normal classes like Random or CultureInfo. The only subtle difference you might perceive when working at the level of this book is that DateTime objects do not necessarily have to be explicitly created, for example, via a constructor call. It is enough, though possibly not practical, to declare a variable of that type.
Summary
Length property, plus ToUpper and Contains methods of text/strings
ToString method of numbers
In the latter case, you also saw that the output generated by the ToString method can be controlled by format strings (like N2, etc.) and language specifications (CultureInfo objects). In the future, you will find it convenient to use the ToString method with absolutely everything in C#, not just with numbers.
Contrary to ordinary objects, static objects always exist in a single copy. While you can have as many DateTimes as you like, you always have precisely one Console.
The word class is synonymous to “object data type.” You will often read about objects of a specific class, which means “object of a particular type.”