Since the computer’s memory (RAM) is volatile, it loses any stored data when the power is turned off. So any data that needs to be stored permanently must be saved in a file.
A file is a named location on a disk drive that is used to store data. Each file is identified by its filename.
Python contains inbuilt functions for reading data from files, as well as creating and writing to files.
In this chapter, we’ll take a look at how to open files, how to read from a file, and how to write to a file.
We’ll take a look at the difference between text files and binary files, as well as random access.
File Types
There are two types of files: text files and binary files. By default, Python reads and writes data in a text file.
Text File
A text file stores sequences of characters: plain text files, HTML files, and program source code.
Use these file modes when opening a file in text mode:
“r” opens a file for reading, error if the file does not exist.
“a” opens a file for appending, creates the file if it does not exist.
“w” opens a file for writing, creates the file if it does not exist.
“r+” opens a file for both reading and writing.
Binary
A binary file is stored in the same format as the computer’s memory (RAM): any images such as jpeg, audio, or program executable files.
Use these file modes when opening a file in binary mode:
“rb” opens a file for binary reading, error if the file does not exist.
“ab” opens a file for binary appending, creates the file if it does not exist.
“wb” opens a file for writing, creates the file if it does not exist.
“rb+” opens a file for both reading and writing.
Text File Operations

The Notepad window shows the filename with four test server names.
Example of a text file
Open Files

A diagram exhibits a process of a file going to the Python Interpreter through write or read.
How a file is accessed in Python
The file mode tells the Python interpreter what you intend to do with the file, that is, read, write, or append:
“r” opens a file for reading, error if the file does not exist.
“a” opens a file for appending, creates the file if it does not exist.
“w” opens a file for writing, creates the file if it does not exist.
“r+” opens a file for both reading and writing.
This depends on the purpose of your program. It’s good practice to open your file, perform your operations, and then close the file.
Write to a File
When opening a file for writing, use either of the following:
“a” opens a file for appending, creates the file if it does not exist. Adds new data to the end of file.
“w” opens a file for writing, creates the file if it does not exist. Overwrites any existing data in file.
Let’s take a look at a program. Open file.py.
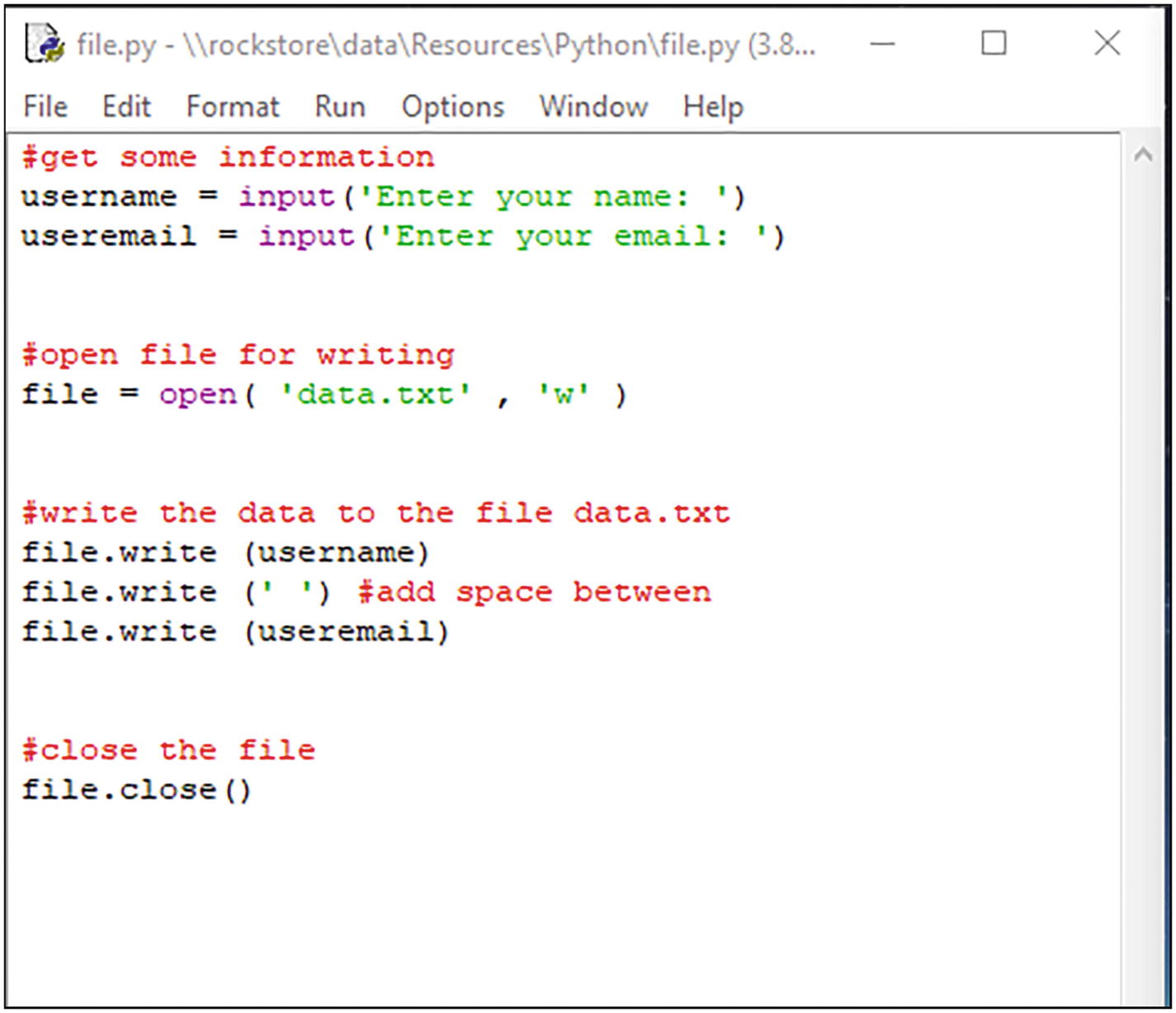
Python file consists of seven lines of programming code.

A diagram illustrates a file labeled data dot t x t assigned to a file.
We then write the username and email address to the file using the file object’s .write method .

A window of a file dot p y with a boxed 3-line code for username and user email points to another notepad file with texts, john, john at ele dot com.
Now remember, the ‘w’ file mode opens a file for writing. This also means any new data will overwrite any data already stored in the file.
Read from a File
When opening a file for writing, use either of the following:
“r” opens a file for reading, error if the file does not exist.
“r+” opens a file for both reading and writing.
Let’s take a look at a program. Open fileread.py.

A file read dot p y window has a 1-line code for data reading points to another notepad file with a list of four emails.
Here, we open a file called “data.txt” and assign it to an object called “file.”
Next , we read the data using the “file” object’s .read method and finally close the file.
Binary File Operations
Most digital data is stored in binary files as they are much smaller and faster than text files.

The Notepad window shows a file titled data dot d a t has a 10-line code of a binary.
Example of a binary file
Open Files
When you open a file, put the filename and the file mode in the parameters of the open()method.
The file mode tells the Python interpreter what you intend to do with the file, that is, read, write, or append:
“rb” opens a file for reading, error if the file does not exist.
“ab” opens a file for appending, creates the file if it does not exist.
“wb” opens a file for writing, creates the file if it does not exist.
“rb+” opens a file for both reading and writing.
This depends on the purpose of your program.
It’s good practice to open your file, perform your operations , and then close the file.
Write to a File
The .write() method writes data in text format, and if you try to write data in binary mode using this method, you’ll get an error message when you run your program.
To write your data in binary format, first we need to convert it to a sequence of bytes. We can do this with the pickle module using a process called pickling. Pickling is the process where data objects such as integers, strings, lists, and dictionaries are converted into a byte stream.
Let’s take a look at a program. Open filewritebin.py. First, we need to include the pickle module. You can do this by using an import command.
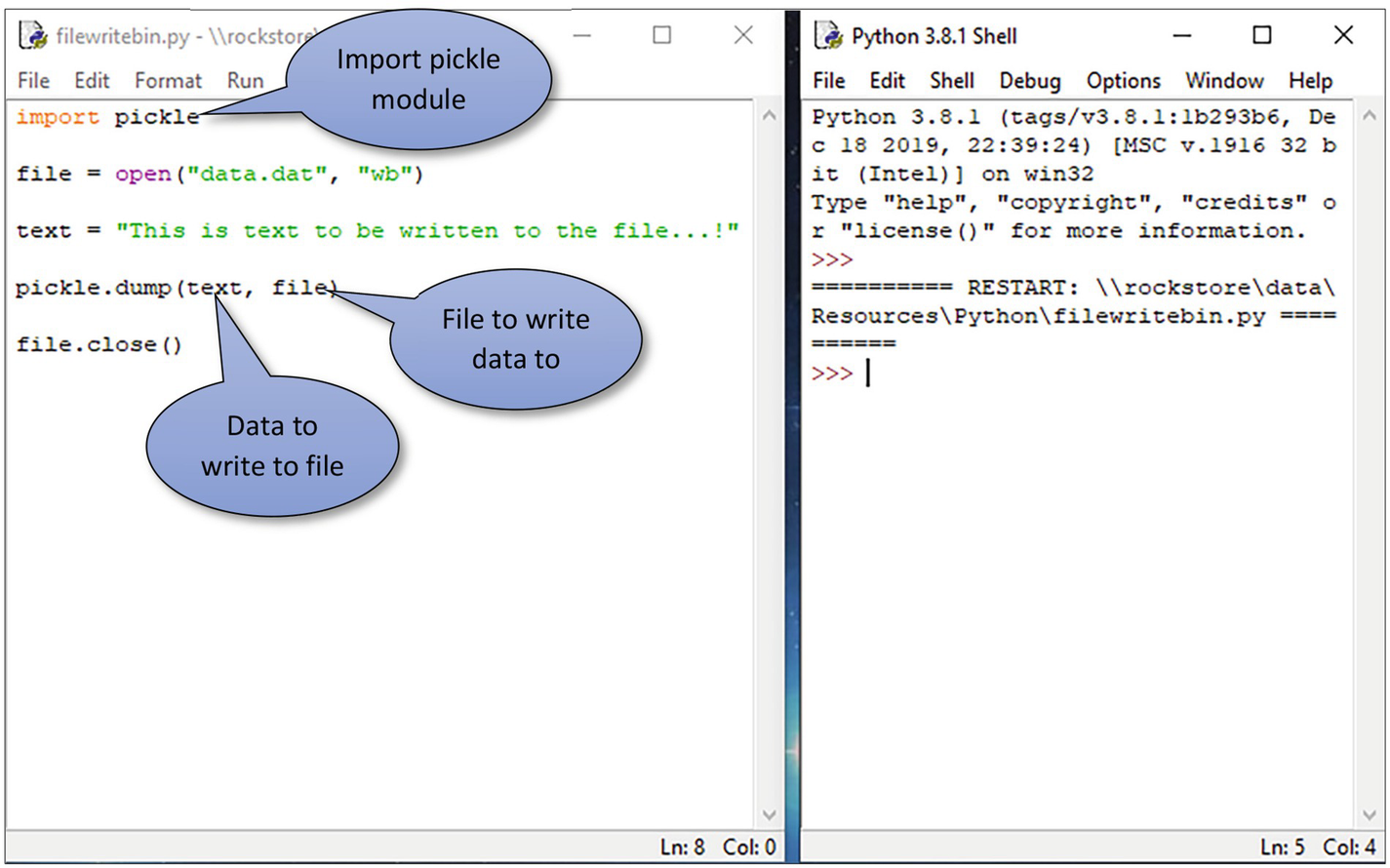
A window has a 5-line code with parts labeled import pickle module, file to write data to, and data to write to file.
Next, we open a file in the usual way, except we set the file mode to binary write (wb).
Now, to write the data to the file, we pickle the “text” object using the pickle.dump() method and finally close the file.
Read a File
Remember when we wrote our data to our binary file, we used a process called pickling. Well, to read the data from the file, we use a similar process.
Let’s take a look at a program. Open filereadbin.py. First, we need to include the pickle module. You can do this using an import command.
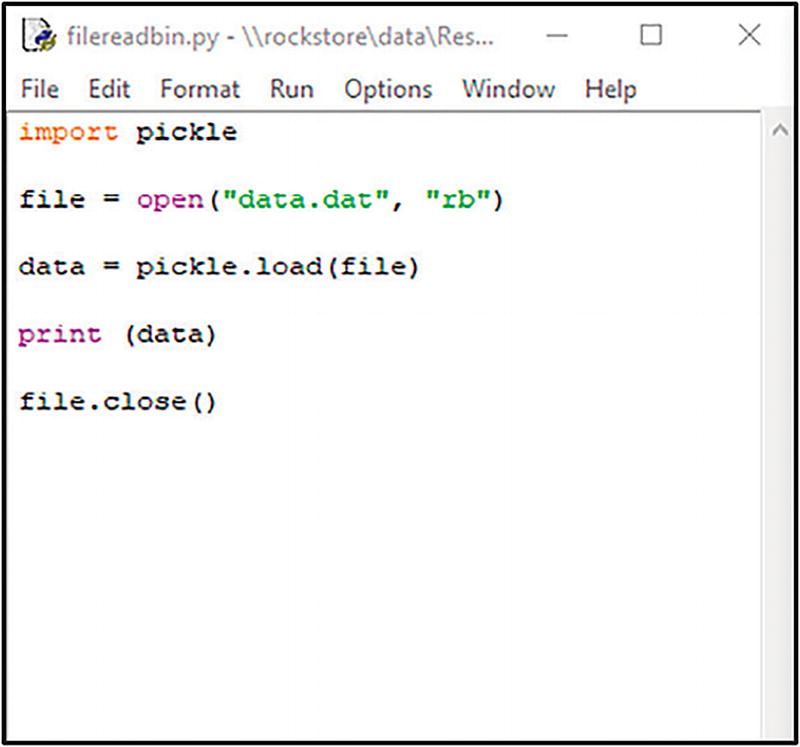
A file read bin dot p y window depicts a 5-line code about pickle import.
When your run the program , the data is read and assigned to the “data” variable.
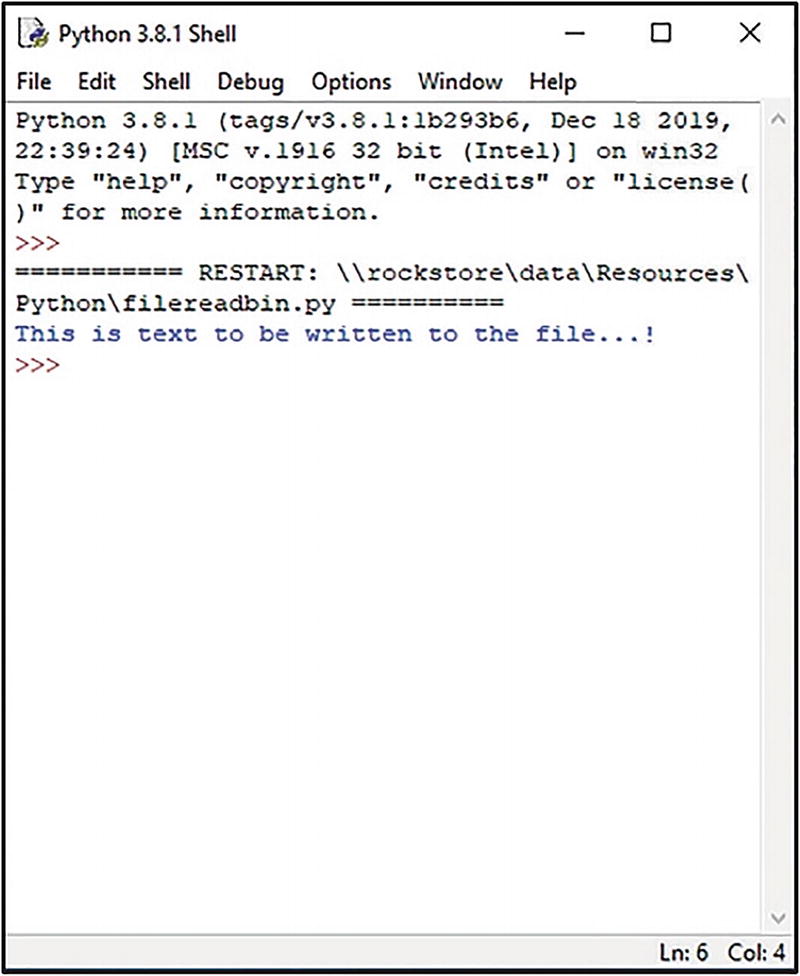
A Python 3 point 8 point 1 shell window depicts a 7-line code to run a program of a file.
Random File Access
When a file is opened, the Python interpreter allocates a pointer within the file. This pointer determines the position in the file from where reading or writing will take place. The pointer can be moved to any location in the file.
The first parameter (position-in-file) determines how many bytes to move. A positive value will move the pointer forward; a negative value will move the pointer backward. The position in the file is called an offset. In a file, each position could contain one byte or one character. Remember, the numbering system starts with 0.
Using our text file as an example file.seek(5) would move the file pointer to the sixth byte:

A window with the number label 012345, has a list of four names, Jack, Pete, Jill, and Mike, each with a username and a user email.
The second parameter (whence) determines where in the file to start from and accepts one of the three values :
0 – Sets the start point to the beginning of the file (the default)
1 – Sets the start point to the current position
2 – Sets the start point to the end of the file
Let’s take a look at a program. Here, we’re going to start reading the first line of the data.txt file starting from the sixth byte or character.
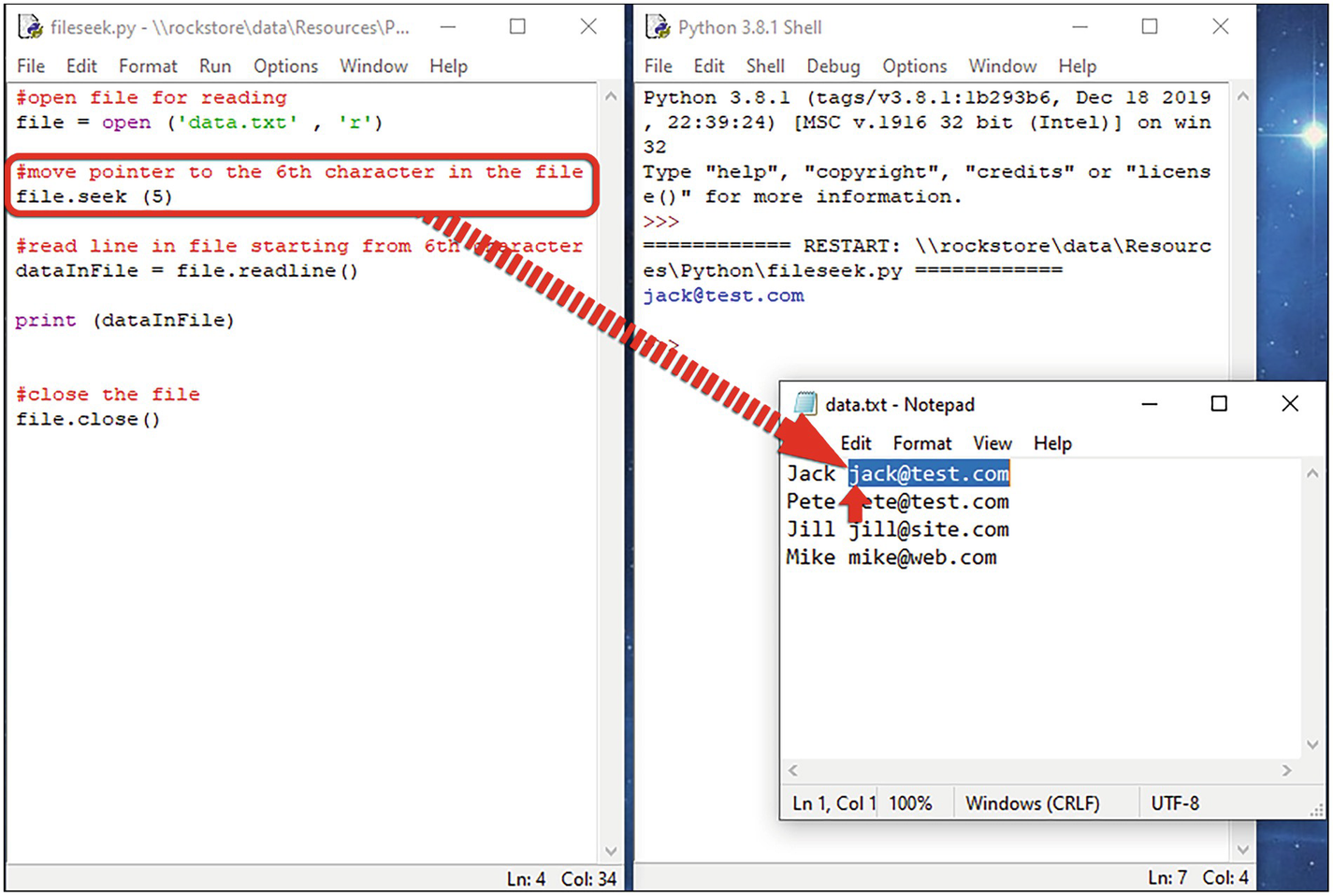
A file seek dot p y window with a boxed 2-line code to point to the sixth character of input points to email, jack at test dot com on a notepad window.
Lab Exercises
- 1.
Write a program that gets a string from the user and then writes it to a file along with the user’s name.
- 2.
Modify the program from exercise 1 so that it appends the data to the file rather than overwriting.
- 3.
Write a program to write a list of names to a file.
- 4.
Write a program to read a file line by line and store it in a list.
- 5.
What is the difference between a text file and a binary file?
Summary
A file is a named location on a disk drive that is used to store data. Each file is identified by its filename.
There are two types of files: text files and binary files. By default, Python reads and writes data in a text file.
A text file stores sequences of characters.
A binary file is stored in the same format as the computer’s memory (RAM).
Use the open( ) method to open a file.
To write data to a file, use the .write( ) method.
To read data from a file, use the .read() method to read the whole file.
To write to a binary file, first we need to convert it to a sequence of bytes. This is called pickling.