What is a computer program? A computer is a device that processes instructions to achieve a task. This set of instructions is called a computer program.
A computer program usually takes some data such as a string or a number and performs calculations to produce results. We usually refer to the data as the program’s input and the results as the program’s output.
To write computer programs, we use a computer programming language. There are many different languages such as BASIC, C, C++, and Python. In this guide, we are going to concentrate on the Python programming language.
Every computer program manipulates data to produce a result, so most languages allow the programmer to choose names for each item of data. These items are called variables or constants . A variable, as the name suggests, is an item that can contain different values as the program is executed. A constant stays the same.
For example, if we wrote a program to calculate the volume of a sphere, we could have variables for the radius and one for the result. We can also have a constant for the value of Pi as it never changes.
In larger programs, we often need to make decisions based on user input, a calculated result, or condition. In this case, we use an if statement. This is called selection.
Some blocks of code might also need to be repeated; in this case, we use a loop. This is called repetition.
The Python programming language has specific facilities to enable us to implement the concepts outlined earlier. Many of these will be introduced throughout this book.
What Is Python
Python is a high-level language developed by Guido van Rossum in the late 1980s and is used in web development, scientific applications, gaming, AI, and is well suited to education for teaching computer programming.
Python is designed to be an easily readable language. Therefore, it uses an uncluttered formatting style and often uses English keywords where other languages use a symbol.
Python is an interpreted programming language, meaning Python programs are written in a text editor and then put through a Python interpreter to be executed.
Python is used in the field of artificial intelligence and can be found in many day-to-day applications. Streaming services such as Spotify use Python for data analysis, particularly users’ listening habits in order to offer suggestions on which artist to follow, other music a particular user might be interested in, and so on. Python is also used within Netflix’s machine-learning algorithms for recommending relevant content to users, monitoring browsing habits, and marketing.
In the world of games development, Python is used as a companion language, meaning Python scripts are used to add customizations to the core gaming engine, script AI behaviors, or server side elements. The performance of Python isn’t fast enough for coding graphics-intensive, higher-end games; however, you can create simple games with Python using the pygame module.
Python is used in web development and allows a web developer to develop dynamic web apps very quickly.
Python is a multi-platform language and is available for Windows, MacOS, Linux, and the Raspberry Pi.

A photograph of a programmer typing computer codes in a software window on his laptop.
To start coding, you’ll need a computer – either Windows, MacOS, or Linux – and an integrated development environment (IDE) with the Python interpreter.
Getting Started
In this section, we’ll take a look at how to install the Python interpreter and development environment. You can install Python on Windows, Mac, or Linux.
Setting Up
Before we start writing programs, we need to set up our development environment. We’ll take a look at installing Python on Windows, Mac, and Linux.
Install on Windows
In our lab, we’re using Windows workstations, so we’ll need to install the Python integrated development environment for Windows.
Open your web browser and navigate to the following website:
www.python.org/downloads/windows
From the Downloads page, select the “executable installer” of the latest stable release.

A section of the downloads webpage labeled Python releases for windows highlights the executable installer under stable releases.
Click “run” when prompted by your browser. Or click “python-x.x.x-amd64.exe” if you’re using Chrome.

A section of the downloads webpage labeled Python releases for windows depicts the downloaded file at the bottom of the browser window.
Once the installer starts, make sure “Add Python 3.x to PATH” is selected , and then click “Customize installation” to run through the steps to complete the installation.

A software installer dialog box labeled Python 3.8.1, 64-bit setup depicts the instructions to install the software.
Make sure you select all the tick boxes for all the optional features.

A software installer dialog box labeled Python 3.8.1, 64-bit setup depicts the tick boxes for the optional features.
Click “Next.”
Make sure “Install for all users” is selected at the top of the dialog box. Click “Install” to begin.

A dialog box labeled Python 3.8.1, 64-bit setup depicts the tick boxes for the advanced options, at the top of the list is installed for all users.
Click “Disable path length limit” to make sure Python runs smoothly on Windows and allow long filenames.

A setup complete dialog box labeled Python 3.8.1, 64-bit setup has hyperlinks to tutorial and documentation, and the disable path length limit option.
Click “Close” to finish the installation.
You’ll find the Python integrated development environment (IDLE) and the Python interpreter in the Python folder on your start menu.

A Windows start menu depicts the expanded Python 3.7 folder, containing I D L E, Python 3.7, manuals, and module docs, on the list of applications.
To write our programs, we’ll use IDLE Python. This is Python’s integrated development environment.

A shell and code editor windows labeled Python 3.7.3 shell and starter dot p y depict the user interface and the options at the top of each window.
Here, you can write your code in the editor and then execute and debug your code. You’ll also notice the code editor provides syntax highlighting, meaning keywords and text are highlighted in different colors, making code easier to read.
Install on MacOS
To install Python 3 with the official installer, open your web browser and navigate to the following website:
www.python.org/downloads/macos
Click Download Python.

The image contains a section of the downloads webpage labeled download the latest version for mac O S highlights the button, download Python 3.10.5.
You’ll find the package in your Downloads folder. Double-click on the package to begin the installation.

A downloads folder window highlights the Python 3.10.5 installer package for mac OS.
Run through the installation wizard. Click “Continue.”

A software installer dialog box labeled install Python depicts the welcome page of the installation wizard, with an introduction to the software.
Once the installation is complete, you’ll find Python in the Applications folder in Finder or on the launchpad.
Install on Linux
If you are running a Linux distribution such as Ubuntu or have a Raspberry Pi, you can install Python using the terminal. You’ll find the terminal app in your applications. You can also press Control+Alt+T on your keyboard.

A Linux terminal command prompt window depicts the field to enter the commands to install Python.
Once installed, you’ll find IDLE in your applications.

A Linux application menu highlights I D L E on the applications list opened via the grid button on the vertical dock on the left side of the screen.
Arrange your windows so you can see your code window and the shell.
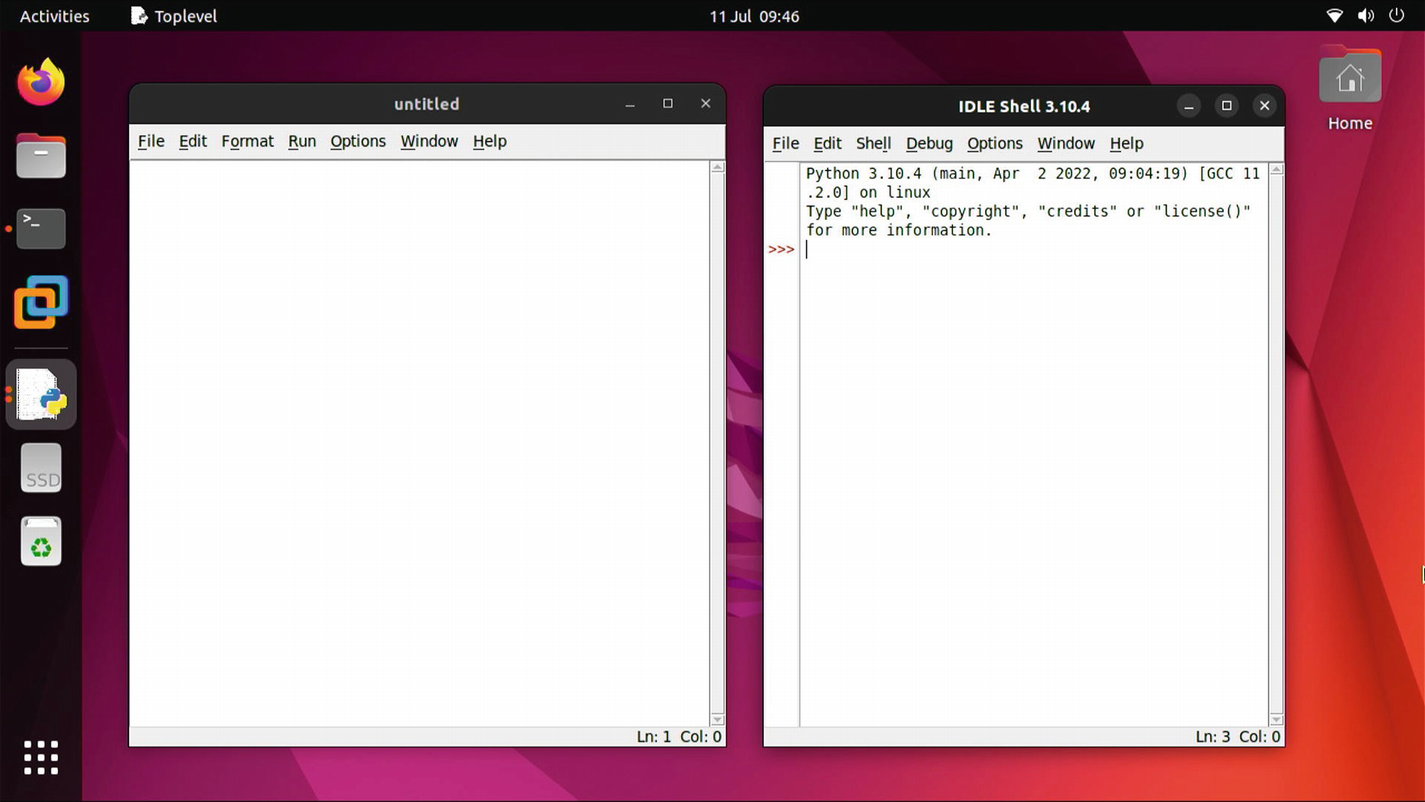
A code editor and shell windows labeled untitled and I D L E shell 3.10.4 depict the user interface and the options at the top of each window.
Summary
Python is a high-level language whose code is executed by an interpreter to produce output.
Python is a multi-platform language and is available for Windows, MacOS, Linux, and the Raspberry Pi.
To write our programs, we use IDLE Python. This is Python’s integrated development environment.