We were moving too fast. We haven't tested the code and made sure it works before committing it. This is a bad practice and should be avoided. No matter how eagerly we want to commit the code, we must make sure it compiles and passes tests in the local environment first.
Now, let's run the mvn install command to see how it works. Unfortunately, we have a build failure. Our only test, which was generated automatically by Spring Initializr, has failed, as you can see from the following output in the console:
...
[ERROR] Tests run: 1, Failures: 0, Errors: 1, Skipped: 0, Time elapsed: 2.499 s <<< FAILURE! - in com.taskagile.TaskAgileApplicationTests
[ERROR] contextLoads(com.taskagile.TaskAgileApplicationTests) Time elapsed: 0.007 s <<< ERROR!
...
And, if you go through the entire error log, you can see the following information provided by Spring Boot:
***************************
APPLICATION FAILED TO START
***************************
Description:
Failed to configure a DataSource: 'url' attribute is not specified and no embedded datasource could be configured.
Reason: Failed to determine a suitable driver class
It seems like that we forgot to add the database driver and configure the datasource. In Chapter 3, Spring 5 - The Right Stack for the Job at Hand, we learned that we need to add the dependency, mysql-connector-java, which contains the MySQL driver class. Let's add the following to pom.xml:
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
And let's also add the following datasource configuration to application.properties:
spring.datasource.url=jdbc:mysql://localhost:3306/task_agile?useSSL=false
spring.datasource.username=<username>
spring.datasource.password=<password>
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
<username> and <password> are just placeholders. Remember to replace them with your own.
Before we move further, let's initialize the task_agile database using database-setup.sql, which we created in Chapter 5, Data Modeling - Designing the Foundation of the Application. Once that is done, let's run another mvn install command to see how things work. There are no more errors. The build has succeeded, as you can see from the output in the console:
[INFO] BUILD SUCCESS
Even though the build has passed, you might well have spotted something wrong in what we just did. That's right, we put the database's username and password in application.properties, which will be pushed to a public repository that anyone can access. This is a security leak, and it is also problematic when we need to deploy the application to staging and production servers because the MySQL's username and password in those environments will definitely be different, as well as the URL of the database. Furthermore, it will cause issues with team collaboration because it forces everyone to use the same username and password for their local dev environment.
A practical way is to use Spring Profiles to segregate the data source configured so that a different environment has its own settings. And, by default, in a Spring Boot application, the active profile is dev. Now, let's change the username and password of the datasource in application.properties to placeholders. The reason we do not remove those settings completely is that we want to use application.properties as a complete view of our configuration. And we create a different configuration file for each environment to override those settings that will be different in each environment. For now, let's focus on the local dev environment and create application-dev.properties with the following properties:
spring.datasource.url=jdbc:mysql://localhost:3306/task_agile?useSSL=false
spring.datasource.username=<change to your own username>
spring.datasource.password=<change to your own password>
If you run the mvn install command again, you can see we still have a successful build. It means the Spring Boot has picked up the settings in application-dev.properties. And to keep these settings only visible in the local dev environment, let's add application-dev.properties into .gitignore.
Now, let's commit the following files and push them to a remote origin, as shown in Figure 8.3:
- .gitignore
- pom.xml
- application.properties
The following screenshot shown the preceding commit operation:
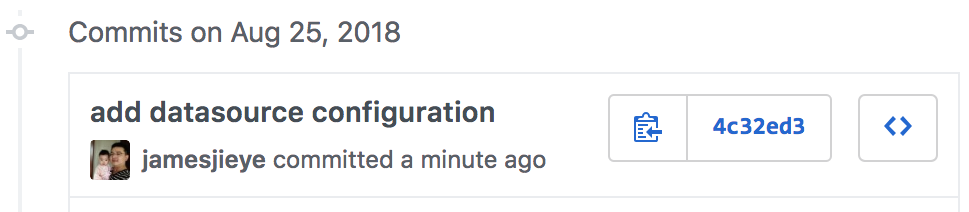
Since we're in the local dev environment, it would be better to make sure we're running in the dev profile. As we will mention in Chapter 14, Health Checking, System Monitoring - Getting Ready for Production, there are many ways to specify the active profile. For a local dev environment, let's use the environment variable to set the active profile.
If you're in a Unix/Linux OS, you can set it by using export spring_profiles_active=dev in your bash profile. And if you're in Windows, you will need to add an environment variable.
By now, we have created the backend scaffold of the TaskAgile application. And, as mentioned before, we can use the mvn spring-boot:run command to start the Spring Boot application.
Now, let's move on to the next step.