The audio program must update some information as soon as an audio track starts playing. Broadly speaking, there are two kinds of updates that the program needs to monitor and update:
- One-time updates: Examples of this include the name of the track and the total length of the track.
- Continuous updates: Examples of this include the position of the seek bar knob and the elapsed play duration. We also need to check continuously whether a track has ended either to play the next track, play the current track again, or stop playing, depending on the loop choice made by the user.
These two kinds of updates will affect sections of the audio player, as shown in the following screenshot:
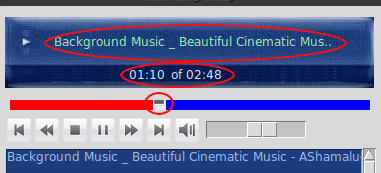
Let's start with the one-time updates, as they are relatively simple to implement.
Since these updates must occur when the playback starts, let's define a method named manage_one_time_updates() and call it from within the start_play() method of the View class, as follows (see code 5.06—view.py):
def manage_one_time_track_updates_on_play_start(self):
self.update_now_playing_text()
self.display_track_duration()
Next, define all the methods called from within the preceding method, as follows:
def update_now_playing_text(self):
current_track = self.model.play_list[self.current_track_index]
file_path, file_name = os.path.split(current_track)
truncated_track_name = truncate_text(file_name, 40)
self.canvas.itemconfig(self.track_name, text=truncated_track_name)
def display_track_duration(self):
self.track_length = self.player.track_length
minutes, seconds = get_time_in_minute_seconds(self.track_length)
track_length_string = 'of {0:02d}:{1:02d}'.format(minutes, seconds)
self.canvas.itemconfig(self.track_length_text, text=track_length_string)
These two methods simply find out the track name and track duration and update the related canvas text by using a call to canvas.itemconfig.
Just like we use config to change the value of widget-related options, the Canvas widget uses itemconfig to change the options for individual items within the canvas. The format for itemconfig is as follows:
canvas.itemconfig(itemid, **options).
Let's define two helper methods in a new file named helpers.py and import the methods in the view namespace. The two methods are truncate_text and get_time_in_minutes_seconds. The code for this can be found in the 5.06—helpers.py file.
That takes care of one-time updates. Now, when you run 5.06—view.py and play some audio file, the player should update the track name, and the total track duration in the top console, as shown in the following screenshot:

We will take care of periodic updates in the next iteration.