The place geometry manager is the most rarely used geometry manager in Tkinter. Nevertheless, it has its uses in that it lets you precisely position widgets within their parent frame by using the (x,y) coordinate system.
The place manager can be accessed by using the place() method on any standard widget.
The important options for place geometry include the following:
- Absolute positioning (specified in terms of x=N or y=N)
- Relative positioning (the key options include relx, rely, relwidth, and relheight)
The other options that are commonly used with place include width and anchor(the default is NW).
Refer to 1.08.py for a demonstration of common place options:
import tkinter as tk
root = tk.Tk()
# Absolute positioning
tk.Button(root, text="Absolute Placement").place(x=20, y=10)
# Relative positioning
tk.Button(root, text="Relative").place(relx=0.8, rely=0.2, relwidth=0.5,
width=10, anchor=tk.NE)
root.mainloop()
You may not see much of a difference between the absolute and relative positions simply by looking at the code or the window frame. However, if you try resizing the window, you will observe that the Absolute Placement button does not change its coordinates, while the Relative button changes its coordinates and size to accommodate the new size of the root window:
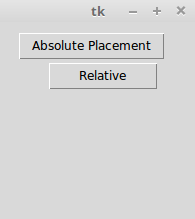
For a complete place reference, type the following command in the Python shell:
>>> import tkinter
>>> help(tkinter.Place)
The place manager is useful in situations where you have to implement custom geometry managers, or where the widget placement is decided by the end user.
While the pack and grid managers cannot be used together in the same frame, the place manager can be used with any geometry manager within the same container frame.
The place manager is rarely used because, if you use it, you have to worry about the exact coordinates. If you make a minor change to a widget, it is very likely that you will have to change the x,y values for other widgets as well, which can be very cumbersome. We will use the place manager in Chapter 7, Piano Tutor.
This concludes our discussion on geometry management in Tkinter.
In this section, you had a look at how to implement the pack, grid, and place geometry managers. You also understood the strengths and weaknesses of each geometry manager.
You learned that pack is suitable for a simple side-wise or top-down widget placement. You also learned that the grid manager is best suited for the handling of complex layouts. You saw examples of the place geometry manager and explored the reasons behind why it is rarely used.
You should now be able to plan and execute different layouts for your programs using these Tkinter geometry managers.