International weather information is displayed on a touch screen where the user selects weather information for different cities and chooses between two screens of displayed information. Initially, this chapter describes displaying text and shapes on the screen, calibrating the touch function of the screen, and creating images by pressing the touch screen. Once sketches are developed to display information and utilize the touch function of the touch screen, the focus shifts to accessing international weather data from OpenWeatherMap.org and the reformatting of the OpenWeatherMap data to display on a touch screen.
Displaying text and shapes on a touch screen or creating images by pressing the touch screen does not require Internet access. An Arduino Uno or Nano is sufficient to run the sketches, but a logic-level converter is required to reduce the voltage to the touch screen to 3.3 V, as the Arduino Uno and Nano operate at 5 V. Accessing OpenWeatherMap data requires connection to the local Wi-Fi network, which is provided by an ESP8266 or ESP32 microcontroller.
ILI9341 SPI TFT LCD touch screen

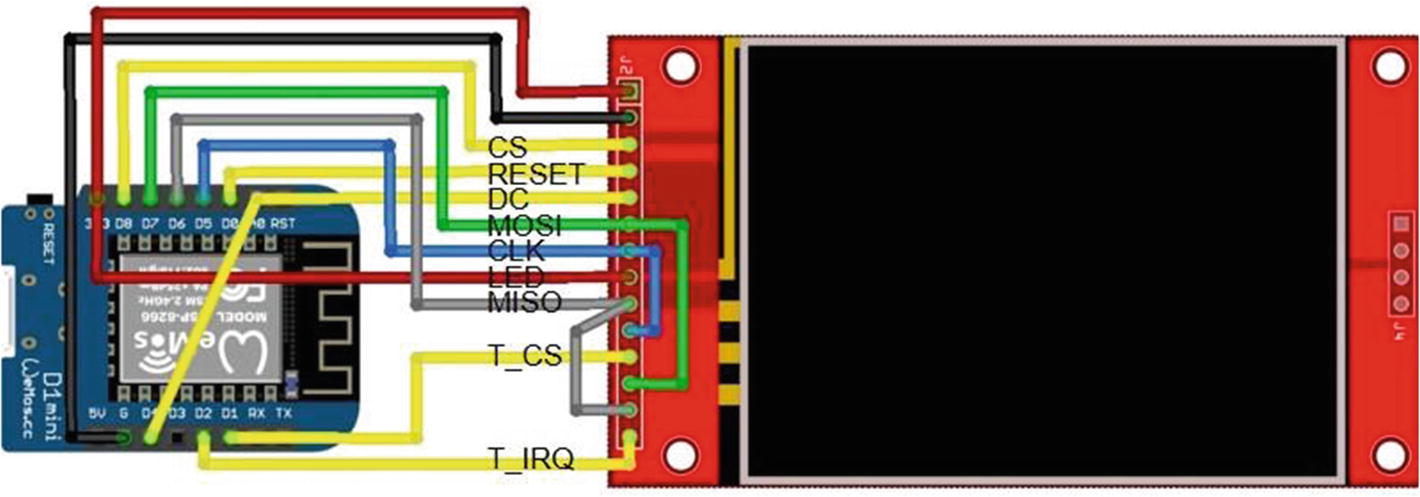
ILI9341 SPI TFT LCD screen and the LOLIN (WeMos) D1 mini development board

ILI9341 SPI TFT LCD screen and the ESP32 DEVKIT DOIT development board
ILI9341 SPI TFT LCD screen and ESP8266 and ESP32 development boards
Component | Pin Function | ESP8266 Pin | ESP32 Pin |
---|---|---|---|
ILI9341 TFT screen VCC 3.3V | 3V3 | 3V3 | |
ILI9341 TFT screen GND | GND | GND | |
ILI9341 TFT screen CS | Chip select | D8 | GPIO 5 |
ILI9341 TFT screen RESET | D0 | GPIO 25 | |
ILI9341 TFT screen DC | Data command | D4 | GPIO 26 |
ILI9341 TFT screen SDA (MOSI) | Serial data in (DI) | D7 | GPIO 23 |
ILI9341 TFT screen SCL (CLK) | Serial clock | D5 | GPIO 18 |
ILI9341 TFT screen LED | 3V3 | 3V3 | |
ILI9341 TFT screen SDO (MISO) | Serial data out | D6 | GPIO 19 |
"touch" | |||
ILI9341 TFT screen T_CLK | Serial clock | D5 | GPIO 18 |
ILI9341 TFT screen T_CS | Chip select | D1 | GPIO 27 |
ILI9341 TFT screen T_DIN | Data input | D7 | GPIO 23 |
ILI9341 TFT screen T_DO | Data output | D6 | GPIO 19 |
ILI9341 TFT screen T_IRQ | Interrupt | D2 | GPIO 13 |
Display text and shapes
Pin definitions for ESP8266 and ESP32 development boards
Touch screen calibration
TFT-eSPI library User_Setup settings for ESP8266 and ESP32 development boards
Calibration of the ILI9341 SPI TFT LCD screen
For example, the calibration parameters for the ILI9341 SPI TFT LCD screen used in this chapter of 450, 3400, 390, 3320, and 3 are copied into the calDat array of the instruction uint16_t calData[] = {450, 3400, 390, 3320, 3} of subsequent sketches. However, the calibration parameters for your ILI9341 SPI TFT LCD screen would be included in the sketches.
Painting on-screen

Paintpot with TFT-eSPI library
ESP8266-specific touch screen calibration and paint
The advantage of the TFT-eSPI library is that one library incorporates screen display and touch functionality, with the applicability to both ESP8266 and ESP32 microcontrollers in conjunction with the ILI9341 SPI TFT LCD screen. The Adafruit_ILI9341esp library, adapted specifically for the ESP8266 microcontroller, has an excellent touch screen painting function. The Adafruit_ILI9341esp library by NailBuster Software is contained in the tft28esp.zip file that is downloaded from nailbuster.com/?page_id=341. The Adafruit_ILI9341esp library requires the XPT2046 library by Spiros Papadimitriou, which is downloaded from github.com/spapadim/XPT2046.
Calibration for the ILI9341 SPI TFT LCD screen with the XPT2046 library differs from calibration with the TFT-eSPI library. In the calibration sketch (see Listing 3-6), two crosses, at 20-pixel margins from the screen edges, are displayed on the ILI9341 SPI TFT LCD screen, which the user presses with a screen pen. Four calibration parameters are then displayed on the ILI9341 SPI TFT LCD screen, that are included in the touch.setCalibration instruction of subsequent sketches.
Calibration of the ILI9341 SPI TFT LCD screen for the XPT2046 library
Paintpot with the XPT2046 library
Weather data for several cities
Another example of using the ILI9341 SPI TFT LCD touch screen displays detailed weather information for several cities, with weather data from OpenWeatherMap.org. OpenWeatherMap data is free to access within limits defined on the website. The OpenWeatherMap data requires a username, a password, an API
(Application Programming Interface) key, and the city identity (ID) code. Details on opening an account and obtaining an API key for OpenWeatherMap are available at openweathermap.org/appid. The API key identifies the client to the web server. The city ID is obtained, from the OpenWeatherMap.org website, by entering the city name in the Your city name search box. Select the relevant city, and the city ID is the number at the end of the URL. For example, the Berlin URL is openweathermap.org/city/2950159.
The ESP8266WiFi or WiFi, ArduinoJson, and Time libraries are required for Wi-Fi communication, interpreting JSON (JavaScript Object Notation) formatted data, and calculating the date and time. Both the ArduinoJson library by Benoît Blanchon and the TimeLib library by Michael Margolis are available in the Arduino IDE, with the latter listed under Time. Several data providers format the date and time as the number of seconds since January 1, 1970, the Unix epoch time. The Time library converts the Unix epoch time to the corresponding minute, hour, day, month, and so on.
regarding a data error are deleted, then they must be replaced with the instruction client.find(" ").
The JSON formatted data consists of the name and value pairs, such as lat and 55.95, that form the JSON document, which is defined as the character array, jsonDoc[] , in the sketch. The value of a name and value pair is identified by the name and extracted with the instruction jsonDoc['name'].as<x>(), where x refers to float, long, or char* for a real number, an integer, or a string, respectively. For example, latitude = jsonDoc['lat'].as<float>().
OpenWeatherMap data example
Listing 3-9 displays OpenWeatherMap data for a selected city with weather information displayed on different screens. The first screen displays the current minimum and maximum temperature and the forecasted weather, with the current humidity and pressure. The second screen displays cloud cover, the wind speed and direction, and more details on forecasted weather, with sunrise and sunset times. A city is selected from the city abbreviations on the right of the screen, and the screen displays are alternated by touching the ⊕ symbol on the left of the screen. The sketch is lengthy, due to processing the comprehensive weather data, and is split into functions to make the sketch more readily interpretable. The weather information is reduced by deleting the secondScreen function and the reference to it in the getWeather function.
Values are referenced according to the class outside the curly brackets, {}, the category within the curly brackets, and the variable name, with each term enclosed by square brackets, [ ]. For example, the humidity value of 62 is referenced as [“main”][“humidity”]. The weather class may have two levels, referenced as [0] and [1]. Times are expressed in Unix epoch time. For example, the sunrise value of 1591846048, referenced as [“sys”][“sunrise”], is 04:27 GMT on June 11, 2020.

OpenWeatherMap information by screen
OpenWeatherMap information
Summary
The Wi-Fi functionality of the ESP8266 and ESP32 microcontrollers enabled Internet access to weather data from OpenWeatherMap. The ILI9341 SPI TFT LCD touch screen function enabled a city to be selected from the touch screen menu. The JSON-formatted weather data was extracted and displayed on the ILI9341 SPI TFT LCD screen. Use of the TFT-eSPI and XPT2046 libraries illustrated two screen calibration processes and differences in ILI9341 SPI TFT LCD touch screen function applied to a screen painting example.
Components List
ESP8266 microcontroller: LOLIN (WeMos) D1 mini or NodeMCU board
ESP32 microcontroller: DEVKIT DOIT or NodeMCU board
SPI TFT LCD touch screen: ILI9341, 2.4 inches, 240 × 320 pixels