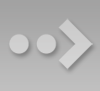
The next highest level is procedural cohesion. This involves actions that are procedur-
ally related, which means that they are related in terms of some control sequence. This is
clearly a design that shows a tighter relationship than the previous one.
Communicational cohesion is a level at which the design is related by the sequence
of activities, much like procedural cohesion, and the activities are targeted on the same
data or the same sets of data. Thus, designs at the communicational cohesion level dem-
onstrate even more internal closeness than those at the procedural cohesion level.
The last two levels, sequential and functional cohesion, are the top levels where the
design unit performs one main activity or achieves one goal. The difference is that at the
sequential cohesion level the delineation of a “single” activity is not as clear as the func-
tional cohesion level. It is not totally clear that having a design unit at a functional cohe-
sion level is always achievable. Clearly, a design unit at this level, being single-goal ori-
ented, would require very few changes for reuse if there is a need for a design to achieve
the same goal. The sequential cohesion level may still include some elements that are not
single-goal oriented and would require some modifications for reuse.
These levels are not always clear cut. They may be viewed as a spectrum of levels that
a designer should try to achieve as high a level as possible. In design, we would strive
for strong cohesion. Although this approach to viewing cohesion is a good guideline, an
example of a more tangible and quantitative measure of cohesion is introduced next.
Program Slice- and Data Slice-Based Cohesion Measure Bieman and Ott (1994)
introduced several quantitative measures of cohesion at the program level based on
program and data slices. We will briefly summarize their approach to measuring cohe-
sion. First, several definitions will be given, and then an example will follow. Consider a
source program that includes variable declarations and executable logic statements as
the context. The following concepts should be kept in mind:
n
A data token is any variable or constant.
n
A slice within the program or procedure is all the statements
that can affect the value of some specific variable of interest.
n
A data slice is all the data tokens in a slice that will affect the
value of a specific variable of interest.
n
Glue tokens are the data tokens in the procedure or program
that lie in more than one data slice.
n
Superglue tokens are the data tokens in the procedure or pro-
gram that lie in every data slice in the program.
From these basic definitions, it is clear that glue tokens and
superglue tokens are those that go across the slices and offer the
binding force or strength of cohesion. Thus, when quantitatively evaluating the program
cohesion, we would be counting the number of these glue and superglue tokens. More
specifically, Bieman and Ott (1994) define the following two measures for measuring
functional cohesion.
Weak functional cohesion = Number of glue tokens / Total number of data tokens
Strong functional cohesion = Number of superglue tokens / Total number of data
tokens
Data token Any variable or constant.
Program or procedure slice All the state-
ments that can affect the value of some
specific variable of interest.
Data slice All the data tokens in a slice
that will affect the value of a specific vari-
able of interest.
Glue tokens The data tokens in the pro-
cedure or program that lie in more than one
data slice.
Superglue tokens The data tokens in the
procedure or program that lie in every data
slice in the program.
8.3 “Good” Design Attributes
173
91998_CH08_Tsui.indd 173 1/10/13 6:45:29 AM