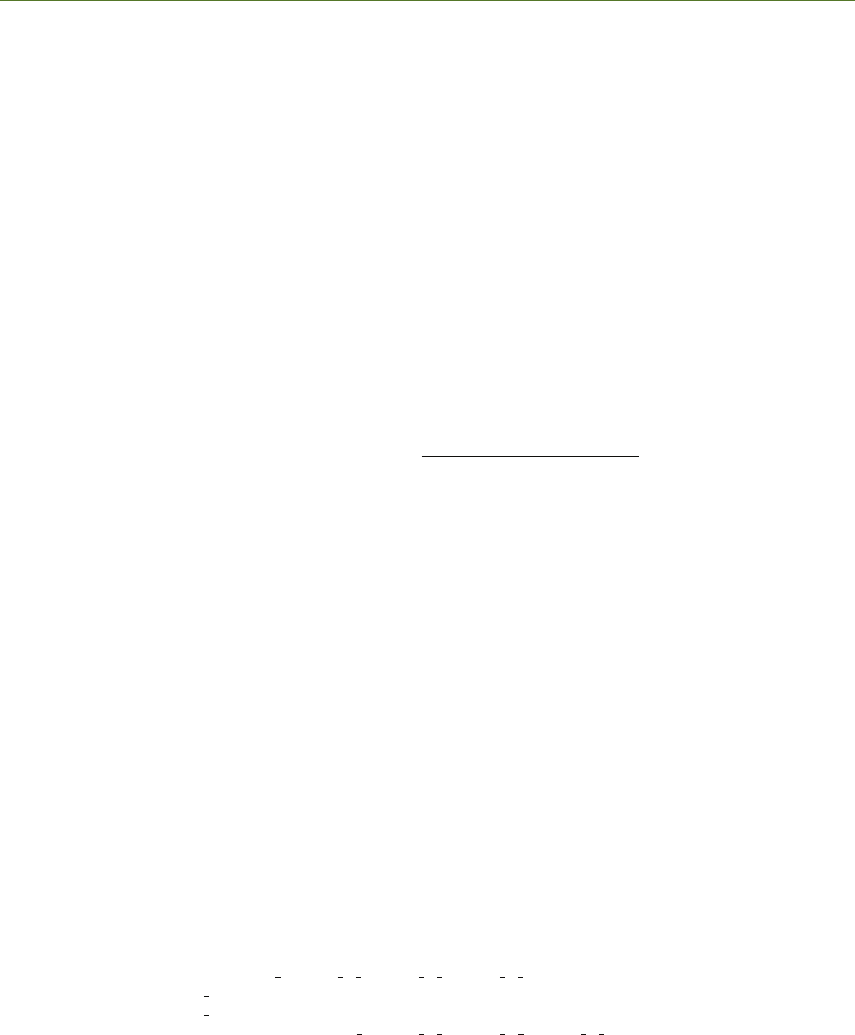
i
i
i
i
i
i
i
i
356 VI 3D Engine Design
2.7 Cross Bilateral Sampling
The cross bilateral filter has been popularized as a means to provide geometry-
aware upsampling. If a screen-space buffer is blurred or upsampled using a simple
bilinear filter, the features in the low-resolution buffer will bleed across depth
boundaries, creating artifacts. The basic idea behind the bilateral filter is to
modify the reconstruction kernel to avoid integrating across depth or normal
boundaries in the scene. This is achieved by storing a depth and/or normal for
each low-resolution sample and assigning filter weight according to not only the
distance in screen space to each sample, but also distance in depth and/or normal
space. Bilateral filters usually use Gaussian weighting functions in both depth and
screen space, however [Yang et al. 08] proposed to use a simple tent function in
screen space, mimicking the effect of a bilinear upsample and therefore requiring
only four depth/image samples. No matter what type of weighting function is
used, the filter weight is accumulated such that the sample can be normalized by
the total accumulated weight:
c
H
i
=
Σc
L
j
f(ˆx
i
, x
j
)g(|z
H
i
− z
L
j
|)
Σf(ˆx
i
, x
j
)g(|z
H
i
− z
L
j
|)
In this example f () is the normal linear filtering weight while g() is a Gaussian
falloff based on the difference in depth between the high-resolution and low-
resolution depths. One disadvantage of bilateral upsampling is its cost compared
with simple bilinear filtering. While a bilinear upsample requires only one hard-
ware filtered sample, a bilateral upsample will require at minimum four point
samples and four depth samples. This cost is incurred at the high resolution,
thus it often partially defeats the purpose of performing calculations at a lower
resolution in the first place. Obviously, if the calculation costs less than eight
samples, it will be less expensive to just compute the value at the high resolu-
tion.
The bilateral filter is one example where PQA works without any of the draw-
backs mentioned in the previous section. Since bilateral upsampling occurs in
screen space, we can set up our low-resolution buffer such that all the pixels in
the same high-resolution quad will share the same low-resolution samples. All
that is needed then is to share the samples across the quad and let each pixel
perform the bilateral filter independently. Here is an example for a 2X upsample
of a low-resolution AO texture. To optimize this further to only one sample, the
depth can be packed into extra channels of the AO texture.
// Gather quad h o r i z o n t a l / v e r t i c a l / d i a g o n a l sa mple s
f l o a t 2 AO D, AO D H, AO D V , AO D D ;
AO D. x = tex2D ( lowResDepthSampler , c oor d ) . x ;
AO D. y = tex2D ( lowResAOSampler , coo rd ) . x ;
QuadGather2x2 ( AO D, AO D H , AO D V, AO D D ) ;