Blazor is a .NET framework you use for building single-page applications (SPA), just like you can use popular JavaScript frameworks such as Angular, React, and Vue.js. But what is a SPA? In this chapter, you will use routing to jump between different sections of a SPA and send data between different components.
What Is a Single-Page Application?
At the beginning of the Web, there were only static pages. A static page is an HTML file somewhere on the server that gets sent back to the browser upon request. Here the server is really nothing but a file server, returning HTML pages to the browser. The browser renders the HTML. The only interaction with the browser then was that you could click a link to get another page from the server. Later came the rise of dynamic pages. When a browser requests a dynamic page , the server runs a program to build the HTML in memory and sends the HTML back to the browser (this HTML never gets stored to disk; of course, the server can store the generated HTML in its cache for fast retrieval later). Dynamic pages are flexible in the way that the same code can generate thousands of different pages by retrieving data from a database and using it to construct the page. Lots of commercial websites like amazon.com use this. But there is still a usability problem. Every time your user clicks a link, the server must generate the next page from scratch and send it to the browser for rendering. This results in a noticeable wait period, and of course, the browser re-renders the whole page.
Then web pages started to use JavaScript to retrieve parts of the page when the user interacts with the UI. One of the first examples of this technique was Microsoft’s Outlook Web Application. This web application looks and feels like Outlook, a desktop application, with support for all user interactions you expect from a desktop application. Google’s Gmail is another example. They are now known as single-page applications. SPAs use certain sections of the web page that are replaced at runtime depending on the user’s interaction. If you click an email, the main section of the page is replaced by the email’s view. If you click your inbox, the main section gets replaced by a list of emails, and so on.
A SPA is a web application that replaces certain parts of the UI without reloading the complete page. SPAs use JavaScript (or C# when you’re using Blazor) to implement this manipulation of the browser’s control tree (also known as the DOM), and most of them consist of a fixed UI and a placeholder element where the contents are overwritten depending on where the user clicks. One of the main advantages of using a SPA is that you can make a SPA state-full. This means that you can keep information loaded by the application in memory. You will look at an example of a SPA, built with Blazor, in this chapter.
Using Layout Components
Let’s start with the fixed part of a SPA. Every web application contains UI elements that you can find on every page, such as a header, footer, copyright, menu, and so on. Copy-pasting these elements to every page would be a lot of work and would require updating every page if one of these elements needed to change. Developers don’t like to do that so every framework for building websites has had a solution for this. For example, ASP.NET WebForms uses master pages; ASP.NET MVC has layout pages. Blazor also has a mechanism for this called layout components.
Blazor Layout Components
Layout components are Blazor components . Anything you can do with a regular component, you can do with a layout component, like dependency injection, data binding, and nesting other components. The only difference is that they must inherit from the LayoutComponentBase class.
The LayoutComponentBase class
MainLayout.razor
On the first line, the MainLayout component declares that it inherits from LayoutComponentBase. Then you see a sidebar and main <div> element, with the main element data-binding to the inherited Body property.

The MainLayout component
Selecting a @layout Component
A second layout component
Choosing a different layout with @layout
Run the application and watch the layout change (the text of the link in the top right corner) as you alternate between Home and Counter.
You can also use the LayoutAttribute if you’re building your component completely in code.
Default layout pages are defined in App.razor
_Imports.razor
_Imports.razor
Any component in this folder (or subfolder) that does not explicitly declare a @layout component will use the MainLayout2 component.
Anything that is shared between all your components can be put in _Imports.razor , especially @using statements. A component can always override the @layout by explicitly adding the layout as in Listing 7-6.
Nested Layouts
A simple nested layout
The Counter component is using the nested layout
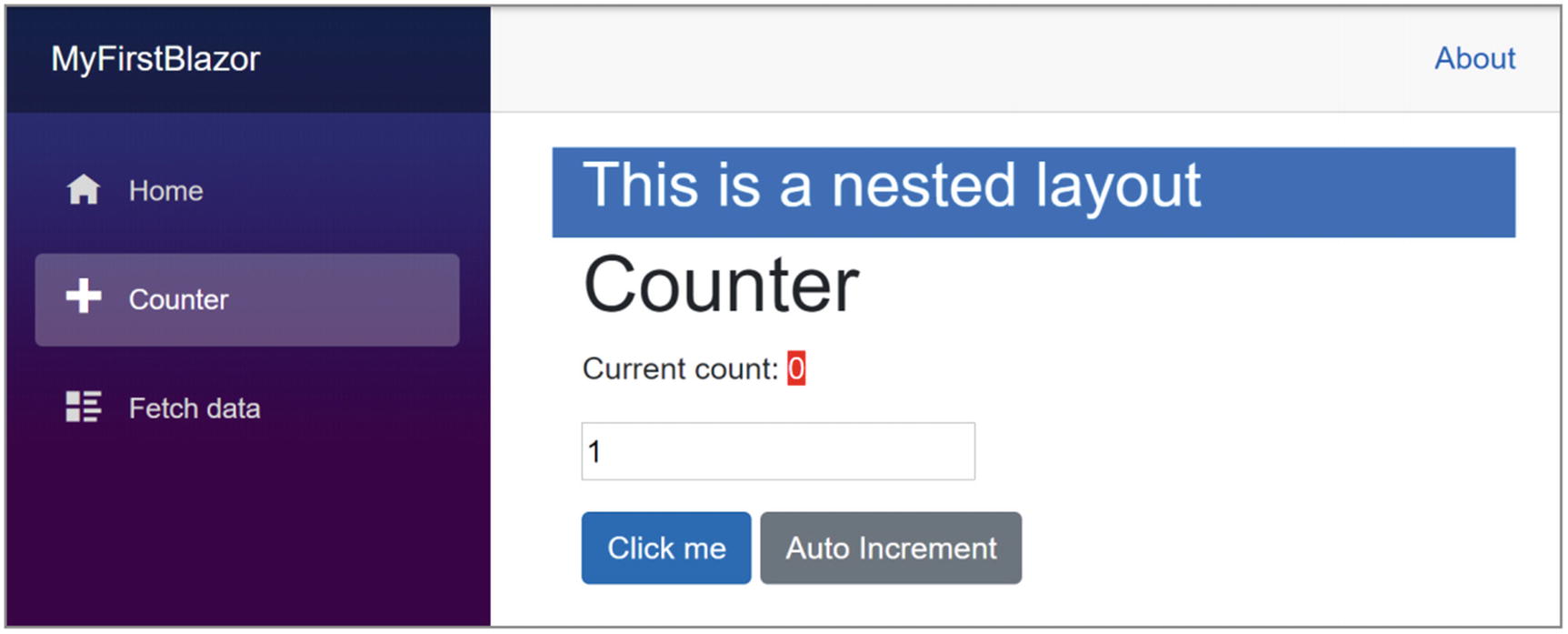
The Counter component using the nested layout
Understanding Routing
Single-page applications use routing to select which component gets picked to fill in the layout component’s Body property. Routing is the process of matching the browser’s URI to a collection of route templates and is used to select the component to be shown on screen. That is why every component in as Blazor SPA uses a @page directive to define the route template to tell the router which component to pick.
Installing the Router
The App component containing the router
The Router component is a templated component with two templates. The Found template is used for known routes, and the NotFound is shown when the URI does not match any of the known routes. You can replace the contents of the last to show a nice error page to the user.
The Found template uses a RouteView component which will render the selected component with its layout (or default layout).
The router will look for all components that have the RouteAttribute (the @page directive gets compiled into a RouteAttribute) and pick the component that matches the current browser’s URI. You will look at setting this RouteAttribute a little later in this chapter, but first, you need to look at the NavMenu component.
The NavMenu Component
The NavMenu component

Your application on a narrow display shows the toggle button
The remaining markup contains the navigation menu, which consists of NavLink components. Let’s look at the NavLink component.
The NavLink Component
The counter route’s NavLink
The default route’s NavLink
When the browser’s URI is empty (except for the site’s URL), the NavLink from Listing 7-12 will be active. But here you have a special case. Normally, NavLink components only match the end of the URI. For example, /counter/55 matches the NavLink from Listing 7-11. But with an empty URI, this would match everything! This is why in the special case of an empty URI you need to tell the NavLink to match the whole URI. You do this with the Match property, which by default is set to NavLinkMatch.Prefix . If you want to match the whole URI, use NavLinkMatch.All as in Listing 7-12.
Setting the Route Template
The Routing component from Blazor examines the browser’s URI and searches for a component’s route template to match. But how do you set a component’s route template? Open the counter component shown in Listing 7-4. At the top of this file is the @page "/counter" directive. It defines the route template. A route template is a string matching a URI, and that can contain parameters, which you can then use in your component.
Using Route Parameters
Defining a route template with a parameter
Listing 7-13 adds route template @page "/counter/{CurrentCount:int}". This tells the router component to match a URI like /counter/55 and to put the number in the CurrentCount parameter of your Counter component. You encase parameters in curly brackets. The Router component will put the value from the route in the property with the same name. You can also specify multiple parameters. Blazor does not allow you to specify default parameters, and therefore you need to specify two route templates. The first route template will pick the Counter component , with the CurrentCount set to its default value of 0. The second route template will pick the Counter component and set the CurrentCount parameter to an int value. It must be int because of the int route constraint.
Filter URIs with Route Constraints
Just like routes in ASP.NET MVC Core , you can use route constrains to limit the type of parameter to match. For example, if you were to use the /counter/Blazor URI, the route template would not match because the parameter does not hold an integer value and the router would not find any component to match.
Constraints are even mandatory if you’re not using string parameters; otherwise, the router does not cast the parameter to the proper type. You specify the constraint by appending it using a colon, for example, @page, "/counter/CurrentCount:int".
Routing Constraints
Route Constraints |
---|
Bool |
Datetime |
Decimal |
Double |
Float |
Guid |
Int |
Long |
If you are building your components as pure C# components, apply the RouteAttribute to your class with the route template as an argument. This is what the @page directive gets compiled into.
Redirecting to Other Pages
How do you navigate to another component using routing? You have three choices: use a standard anchor element, use the NavLink component, and use code. Let’s start with the normal anchor tag.
Navigating Using an Anchor
Navigation using an anchor tag
This link has been styled as a button using Bootstrap 4. Run your application and navigate to the Counter component. Click the Home button to navigate to the Index component whose route template matches "/".
Navigating Using the NavLink Component
The NavLink component uses an underlying anchor, so its usage is similar. The only difference is that a NavLink component applies the active class when it matches the route. Generally, you only use a NavLink in the NavMenu component, but you are free to use it instead of anchors.
Navigating with Code
Navigating in code is also possible, but you will need an instance of the NavigationManager class through dependency injection. This instance allows you to examine the page’s URI and has a helpful NavigateTo method . This method takes a string that will become the browser’s new URI.
Using the NavigationManager
You tell dependency injection with the @inject directive to give you an instance of the NavigationManager and put it in the uriHelper field. Then you add a button that calls the StartFrom50 method when clicked. This method uses the NavigationManager to navigate to another URI by calling the NavigateTo method. Run your application and click the “Start from 50” button. You should navigate to /counter/50.
Understanding the Base Tag
Index.html
If you are using Server-Side Blazor, the base tag can be found in _Host.cshtml.
When you deploy in production, all you need to do is to update the base tag. For example, you might deploy your application to https://online.u2u.be/selfassessment. In this case, you would update the base element to <base href="/selfassessment" />. So why do you need to do this? If you deploy to https://online.u2u.be/selfassessment, the counter component’s URI becomes https://online.u2u.be/selfassessment/counter. Routing will ignore the base URI so it will match the counter as expected. You only need to specify the base URI once, as shown in Listing 7-16.
Sharing State Between Components
When you navigate between different Blazor components with routing, you will probably encounter the need to send information from one component to another. One way to accomplish this is by setting a parameter in the destination component by passing it in the URI. For example, you could navigate to /pizzadetail/5 to tell the destination component to display information about the pizza with id 5. The destination component can then use a service to load the information about pizza #5 and then display this information. But in Blazor, there is another way. You can build a State class (most developers call this State, but this is just a convention and you can call it anything you want; State just makes sense) and then use dependency injection to give every component the same instance of this class. This is also known as the Singleton Pattern . This way, all your components share the same State instance, and this makes it easy to communicate between components that are not in a parent-child relationship. Your PizzaPlace application is already using a State class, so it should not be too much work to use this pattern.
Using dependency injection to get the State Singleton instance
Configuring dependency injection for the State singleton
Run the application. Everything should still work! What you’ve done is to use the Singleton Pattern to inject the State singleton into the Index component. Let’s add another component that will use the same State instance.
Adding a CurrentPizza property to the State class
Adding an anchor to display the pizza’s information
When someone clicks this link, it should set the State instance’s CurrentPizza property. But you don’t have access to the State object. One way to solve this would be by injecting the State instance in the PizzaItem component . But you don’t want to overburden this component, so you add a ShowPizzaInformation callback delegate to tell the containing PizzaList component that you want to display more information about the pizza. Clicking the pizza name link simply invokes this callback without knowing what should happen.
You are applying a pattern here known as “Dumb and Smart Components.” A dumb component is a component that knows nothing about the global picture of the application. Because it doesn’t know anything about the rest of the application, a dumb component is easier to reuse. A smart component knows about the other parts of the application (such as which service to use to talk to the database) and will use dumb components to display its information. In our example, the PizzaList and PizzaItem are dumb components because they receive all their data through data binding, while the Index component is a smart component which talks to services.
Adding a PizzaInformation callback to the PizzaList component
You added a ShowPizzaInformation callback to the PizzaList component, and you simply pass it to the PizzaItem component. The Index component will set this callback, and the PizzaList will pass it to the PizzaItem component.
The Index component navigates to the PizzaInfo component
The Index component tells the PizzaList component to call the ShowPizzaInformation method when someone clicks the information link from the PizzaItem component. The ShowPizzaInformation method then sets the State’s CurrentPizza property and navigates using the NavigateTo method to the /PizzaInfo route.
If you call NavigateTo as part of a callback, Blazor returns to the original route. That is why I use a background Task so Blazor will navigate after the callback…
Adding a PizzaInfo component
At the bottom of the markup, you add an anchor (and make it look like a button using bootstrap styling) to return to the menu. It’s an example of changing the route with anchors. Of course, in a real-life application, you would show the ingredients of the pizza, a nice picture, and other information. I leave this as an exercise for you.
Summary
In this chapter, you looked at two things, layouts and routing.
Layouts allow you to avoid replicating markup in your application and help keep your application’s look consistent. You also saw that layouts can be nested.
Routing is an important part of building single-page applications and takes care of picking the component to show based on the browser’s URI. You define route templates using the @page syntax where you use route parameters and constraints. Navigation in your single-page application can be done using anchor tags and from code using the NavigationManager class. You then modified the PizzaPlace application to show how to share information between different routes in a Blazor application.