For many people getting into programming, one of their first issues is figuring out what they can build. Programming is seldom learned by just reading. Many people think they can read couple of guides and look at the syntax and then easily learn how to program. But programming takes hands-on practice.
For this reason, this book includes a sample Python project. The project is not very complicated at the start, but it’s easy to continue working further on the project, extending and customizing it as you gain experience.
About the Project
This book assumes you have basic knowledge of Python and have Python 3.6 or above installed.
To help you get acquainted with Docker, the book teaches you how to take an existing Python app, run it from the Python command line, introduce different Docker components, and then transition the app into a containerized image.
The Python app is a simple application with a bot interface using Telegram Messenger to fetch the last 10 stories from Reddit. Using Telegram, you can subscribe to a list of subreddits. The web application will check the subscribed subreddits for new posts and, if it finds new topics, it will publish the topics to the bot interface. That interface will deliver the message to Telegram Messenger, when requested by the user.
Initially, you will not be saving the preferences (i.e., the subreddit subscriptions) and will focus on getting the bot up and running. Once things are working fine, you will learn how to save the preferences to a text file and, eventually, to a database.
Setting Up Telegram Messenger
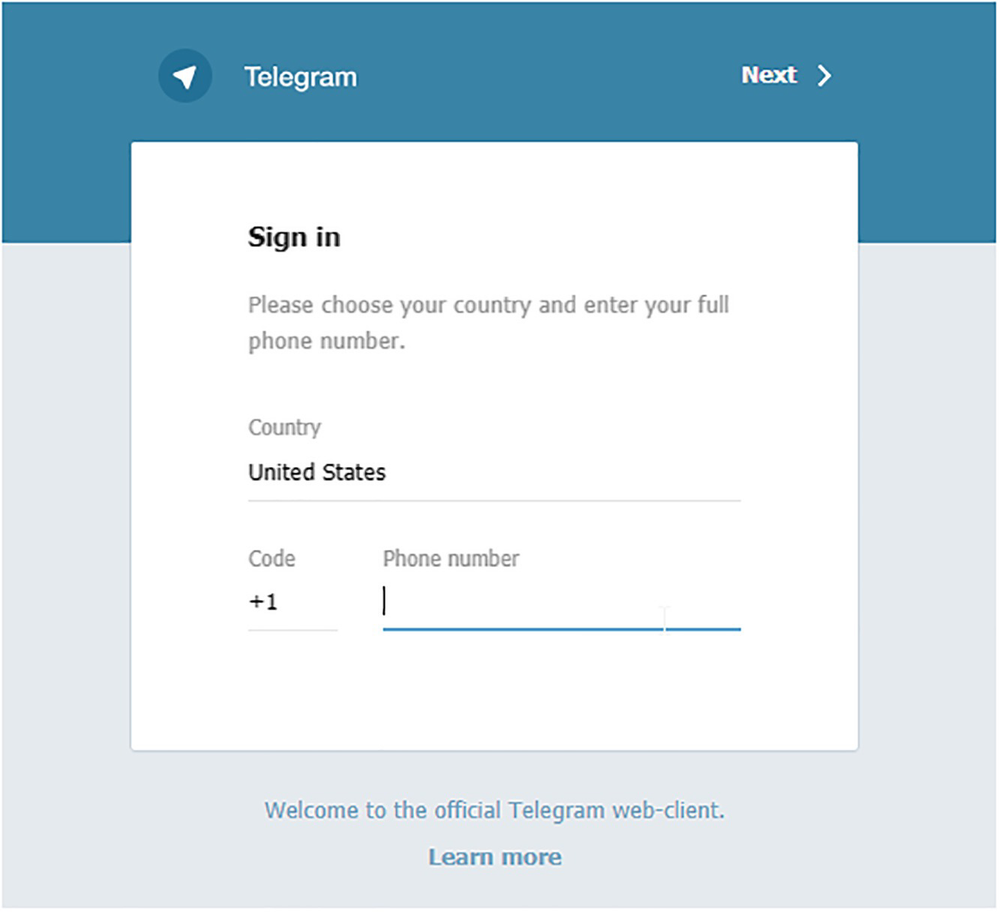
Telegram signup page
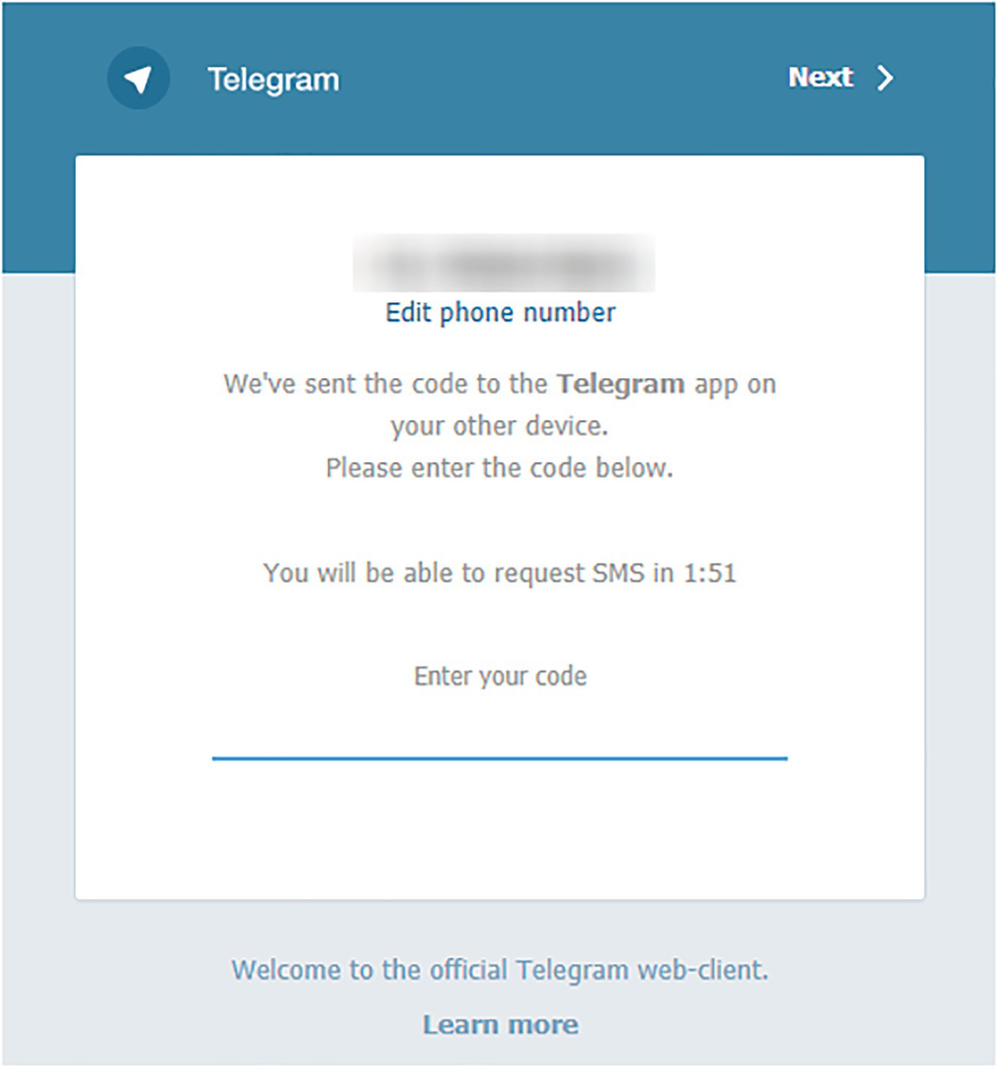
Telegram’s one-time password
BotFather: Telegram’s Bot Creation Interface
Telegram uses a bot called “BotFather” as its interface for creating new bots and updating them. To get started with BotFather, in the search panel type BotFather. From the chat window, type /start.
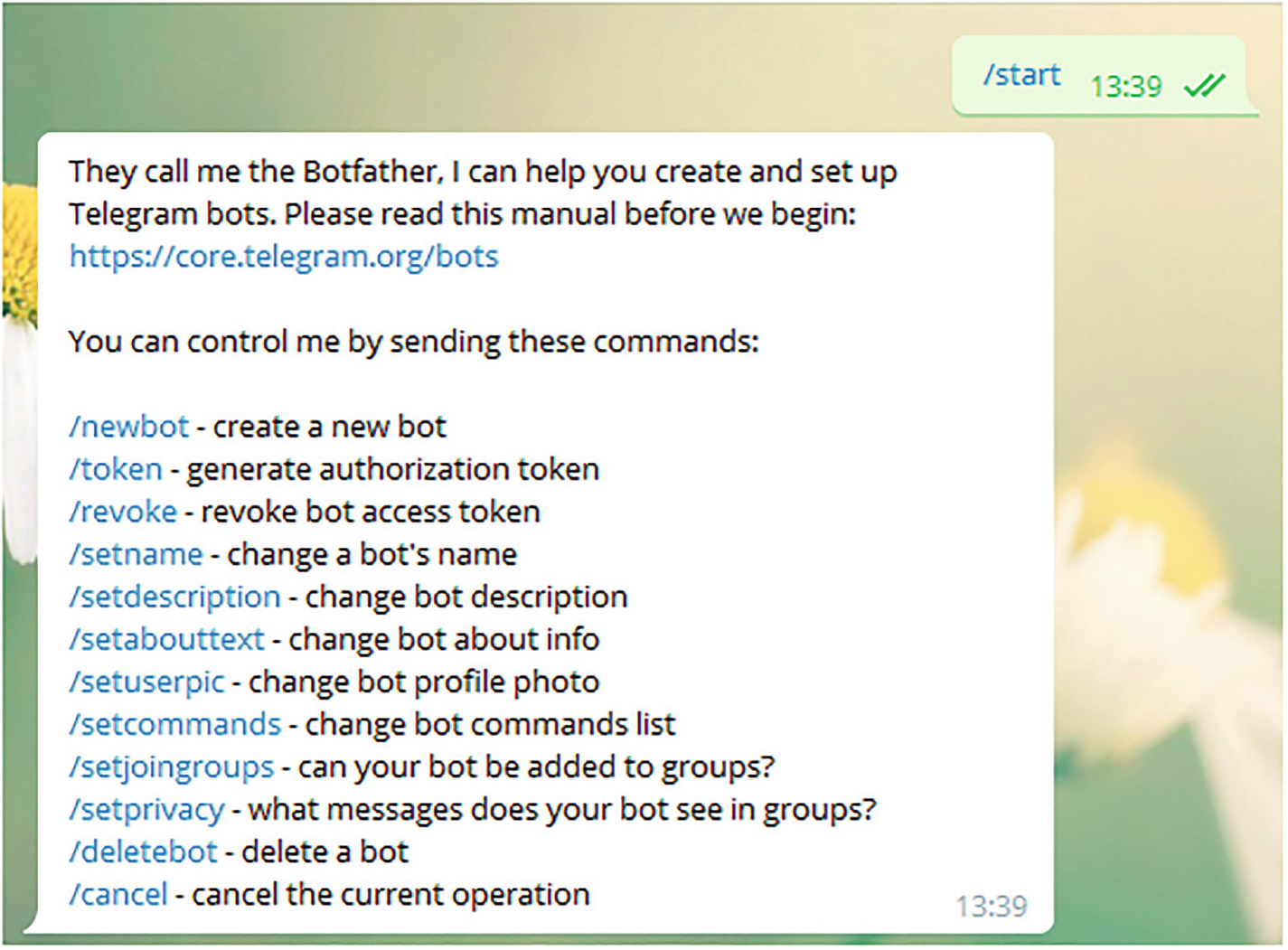
BotFather’s options
Creating the Bot with BotFather

Telegram bot ready for action
Along with the link to the documentation, you will notice that Telegram has issued a token. HTTP is a stateless protocol—the webserver does not know and does not keep track of who is requesting the resource. The client needs to identify itself so that the webserver can identify the client, authorize it, and serve the request. Telegram uses the API token (henceforth, referred to as <token>, including in the code samples) as a way of identifying and authorizing bots.
The token is extremely sensitive. If it’s leaked online, anyone can post messages as your bot. Do not check it in with your version control or publish it anywhere!
When working with APIs you are not familiar with, it’s always good to use a good tool to test and explore the endpoints instead of typing the code right away. Some examples of REST API test tools include Insomnia, Postman, and curl.
Telegram’s Bot API documentation is available at https://core.telegram.org/bots/api. To make a request, you have to include the <token>. The general URL is as follows:
https://api.telegram.org/bot<token>/METHOD_NAME
Making a curl Request to Telegram API Without a Token
Making a curl Request to Telegram API with a Valid Token
You can see that, with the proper token, Telegram identifies and authorizes the bot. This confirms that the bot token is proper, and you can go ahead with the app.
Newsbot: The Python App
Continuously polls the Telegram API for new updates being posted to the bot.
If the keyword for fetching new updates was detected, it fetches the news from the selected subreddits.
If there’s a new message starting with /start or /help, it shows simple help text about what to do.
If there’s a message starting with /sources followed by a list of subreddits, it accepts them as the subreddits from the applicable Reddit posts.
Praw or Python Reddit API Wrapper, for fetching posts from subreddits.
Requests, one of the most popular Python libraries for providing a simpler, cleaner API for making HTTP requests.
Getting Started with Newsbot
To get started with Newsbot, download the source code of the bot. The source code is available on the GitHub repository of the book, at https://github.com/Apress/practical-docker-with-python.
pip (Pip Installs Packages) is a package manager that installs Python libraries. pip is included with Python 2.7.9 and later, and Python 3.4 and later. pip3 indicates that you are installing libraries for Python 3. If pip is not installed, install it before proceeding.
The -r flag tells pip to install the required packages from requirements.txt.
The Output from a Successful pip Install
If some packages were already installed, pip will not reinstall them and will inform you that the dependency is installed with a "Requirement already satisfied" message.
Running Newsbot
Let’s start the bot. The bot requires an authentication token from Telegram that you created previously (referred to as <token>). This needs to be set to an environment variable named as NBT_ACCESS_TOKEN . Without this token, the bot will not run. To set this token, open a terminal and enter the following command, depending on your platform.
Output from Newsbot When It Is Running and Listening to Messages from Telegram
Sending Messages to Newsbot
In this section, you try to send a message to Newsbot to see if it accepts requests. From the BotFather window, click the link to the bot (alternatively, you can also search using the bot username). Click the Start button. This will trigger a /start command, which will be intercepted by the bot.
The Newsbot Responding to Commands

The response from Newsbot to the start message

Sources assigned
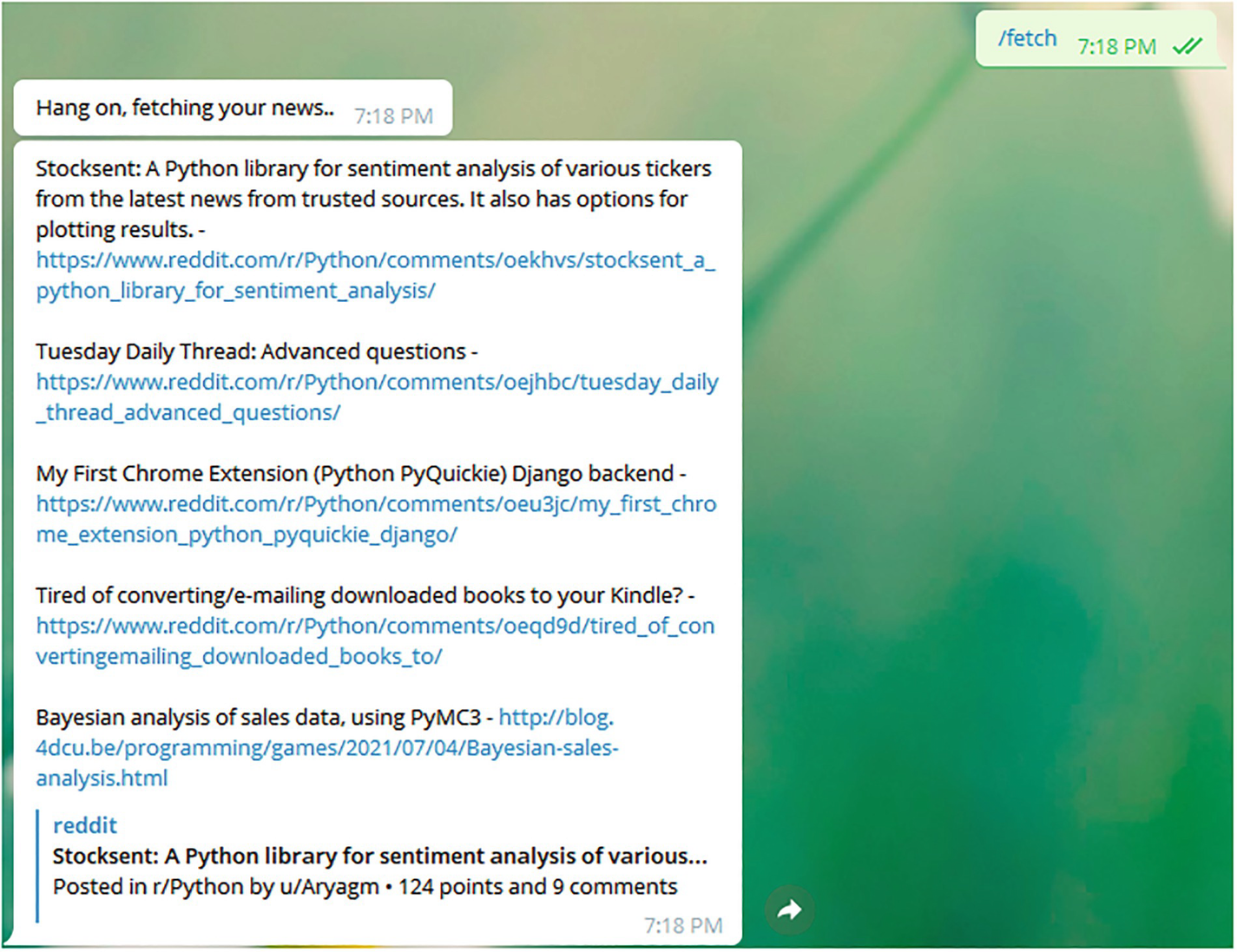
Newsbot posting the top news from the Python subreddit
The bot works; it’s fetching the top posts as expected. In the next series of chapters, you learn how to move Newsbot to Docker.
Summary
In this chapter, you learned about the details of the book’s Python Project, which is a chatbot. You also learned how to install and configure Telegram Messenger, how to use Telegram’s BotFather to create the bot, how to install the dependencies for the bot and, finally, how to run the bot and ensure that it works correctly. In the next chapter, you dive deep into Docker, learn more about Dockerfiles, and containerize the Newsbot app by writing a Dockerfile for it.