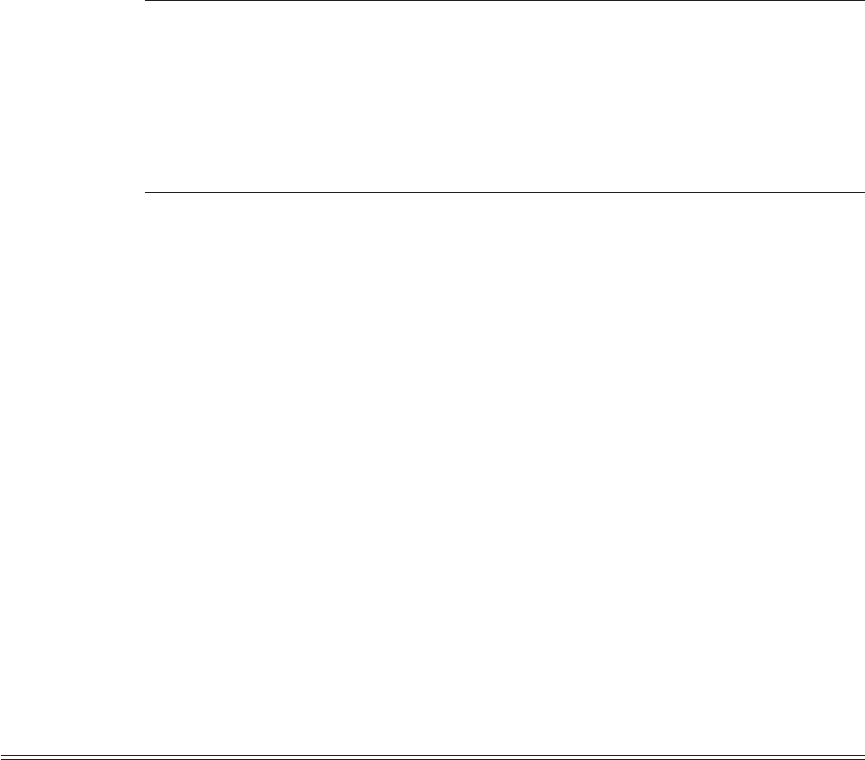
130 5. Sprites and Animation
width/2*getScale();}
// Return rotation angle in degrees
virtual float getDegrees() {return spriteData.angle*(180.0f/
(float)PI);}
// Return rotation angle in radians
virtual float getRadians() {return spriteData.angle;}
// Return delay between frames of animation
virtual float getFrameDelay() {return frameDelay;}
// Return number of starting frame
virtual int getStartFrame() {return startFrame;}
// Return number of ending frame
virtual int getEndFrame() {return endFrame;}
// Return number of current frame
virtual int getCurrentFrame() {return currentFrame;}
// Return RECT structure of Image
virtual RECT getSpriteDataRect() {return spriteData.rect;}
// Return state of animation complete
virtual bool getAnimationComplete() {return animComplete;}
// Return colorFilter
virtual COLOR_ARGB getColorFilter() {return colorFilter;}
Listing 5.25. e image’s get functions.
5.5.3 Image set Functions
A number of set functions are included in image.h (Listing 5.26).
// Set X location
virtual void setX(float newX) {spriteData.x = newX;}
// Set Y location
virtual void setY(float newY) {spriteData.y = newY;}
// Set scale
virtual void setScale(float s) {spriteData.scale = s;}
// Set rotation angle in degrees
// 0 degrees is up. Angles progress clockwise
virtual void setDegrees(float deg) {spriteData.angle = deg*((float)
PI/180.0f);}
// Set rotation angle in radians
// 0 radians is up. Angles progress clockwise
virtual void setRadians(float rad) {spriteData.angle = rad;}
// Set visible
virtual void setVisible(bool v) {visible = v;}
// Set delay between frames of animation
virtual void setFrameDelay(float d) {frameDelay = d;}
// Set starting and ending frames of animation
virtual void setFrames(int s, int e){startFrame = s; endFrame = e;}
// Set current frame of animation
virtual void setCurrentFrame(int c);
// Set spriteData.rect to draw currentFrame
virtual void setRect();
// Set spriteData.rect to r
virtual void setSpriteDataRect(RECT r) {spriteData.rect = r;}
// Set animation loop. lp = true to loop
virtual void setLoop(bool lp) {loop = lp;}