The empty operator was discussed in Chapter 3, Functional Programming with ReactiveX. This operator is one of a triplet of similar operators: empty, never, and throw. The behavior of each of these operators should be clear from their names, as follows:
- The empty operator creates an empty observable that completes immediately
- The never operator creates an observable that never completes
- The throw operator creates an observable that immediately completes on error
The following figure shows the marble diagram of the never operator:
The following figure shows the marble diagram of the throw operator:
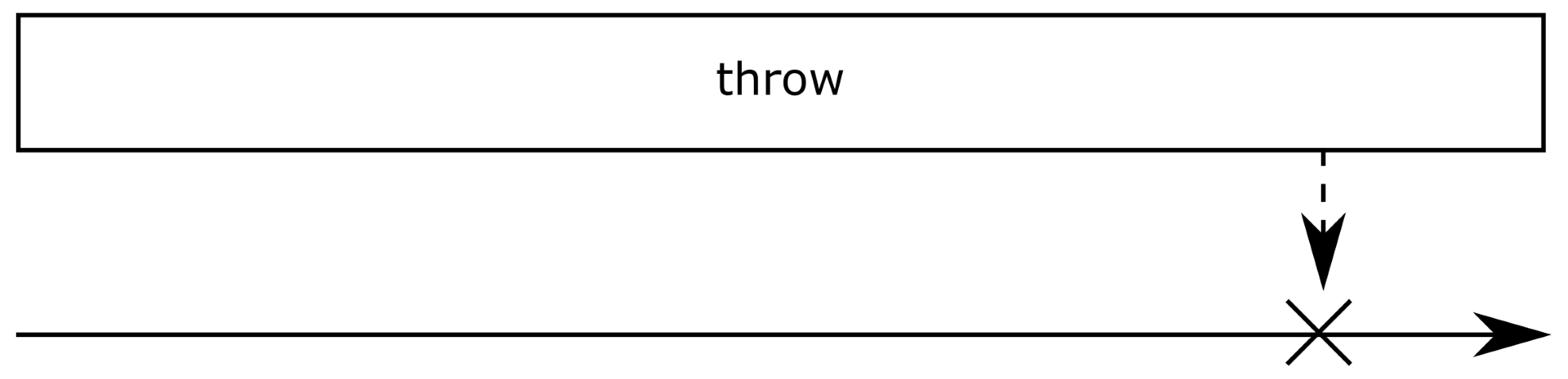
Their prototypes are as follows:
Observable.never()
Observable.throw(exception, scheduler=None)
Note that the never operator does not have a scheduler parameter. Since it never emits anything, there is no need for scheduling! The throw operator accepts any object in the exception parameter. If the provided value is an exception, then it is sent as-is. If the provided value is not an exception, then this value is encapsulated in an exception object before being notified. So, the observer of this observable will always receive an exception in its on_error handler.
The following code shows two ways to use the throw operator:
error = Observable.throw("Something wrong happened")
error.subscribe(
on_next=lambda i: print("item: {}".format(i)),
on_error=lambda e: print("error: {}".format(e)),
on_completed=lambda: print("completed")
)
exception = Observable.throw(NotImplementedError("I do nothing"))
exception.subscribe(
on_next=lambda i: print("item: {}".format(i)),
on_error=lambda e: print("error: {}".format(e)),
on_completed=lambda: print("completed")
)
The preceding code will provide the following results: