- All the examples in this chapter that deal with cameras return when there is a single failed or corrupted frame that leads to the detection of an empty frame. What type of modification is needed to allow a predefined number of retries before stopping the process?
const int RETRY_COUNT = 10;
int retries = RETRY_COUNT;
while(true)
{
Mat frame;
cam >> frame;
if(frame.empty())
{
if(--retries < 0)
break;
else
continue;
}
else
{
retries = RETRY_COUNT;
}
// rest of the process
}
- How can we call the meanShift function to perform the Mean Shift algorithm with 10 iterations and an epsilon value of 0.5?
TermCriteria criteria(TermCriteria::MAX_ITER
+ TermCriteria::EPS,
10,
0.5);
meanShift(backProject,
srchWnd,
criteria);
- How do you visualize the hue histogram of the tracked object? Assume that CamShift is used for tracking.
Having the following function:
void visualizeHue(Mat hue)
{
int bins = 36;
int histSize[] = {bins};
int nimages = 1;
int dims = 1;
int channels[] = {0};
float rangeHue[] = {0, 180};
const float* ranges[] = {rangeHue};
bool uniform = true;
bool accumulate = false;
Mat histogram, mask;
calcHist(&hue,
nimages,
channels,
mask,
histogram,
dims,
histSize,
ranges,
uniform,
accumulate);
double maxVal;
minMaxLoc(histogram,
0,
&maxVal,
0,
0);
int gW = 800, gH = 100;
Mat theGraph(gH, gW, CV_8UC3, Scalar::all(0));
Mat colors(1, bins, CV_8UC3);
for(int i=0; i<bins; i++)
{
colors.at<Vec3b>(i) =
Vec3b(saturate_cast<uchar>(
(i+1)*180.0/bins), 255, 255);
}
cvtColor(colors, colors, COLOR_HSV2BGR);
Point p1(0,0), p2(0,theGraph.rows-1);
for(int i=0; i<bins; i++)
{
float value = histogram.at<float>(i,0);
value = maxVal - value; // invert
value = value / maxVal * theGraph.rows; // scale
p1.y = value;
p2.x = float(i+1) * float(theGraph.cols) / float(bins);
rectangle(theGraph,
p1,
p2,
Scalar(colors.at<Vec3b>(i)),
CV_FILLED);
p1.x = p2.x;
}
imshow("Graph", theGraph);
}
We can call the following right after the CamShift function call to visualize the hue of the detected object:
CamShift(backProject,
srchWnd,
criteria);
visualizeHue(Mat(hue, srchWnd));
- Set the process noise covariance in the KalmanFilter class so that the filtered and measured values overlap. Assume that only process noise covariance is set of all the available matrices for the KalmanFilter class's behavior control.
setIdentity(kalman.processNoiseCov,
Scalar::all(1.0));
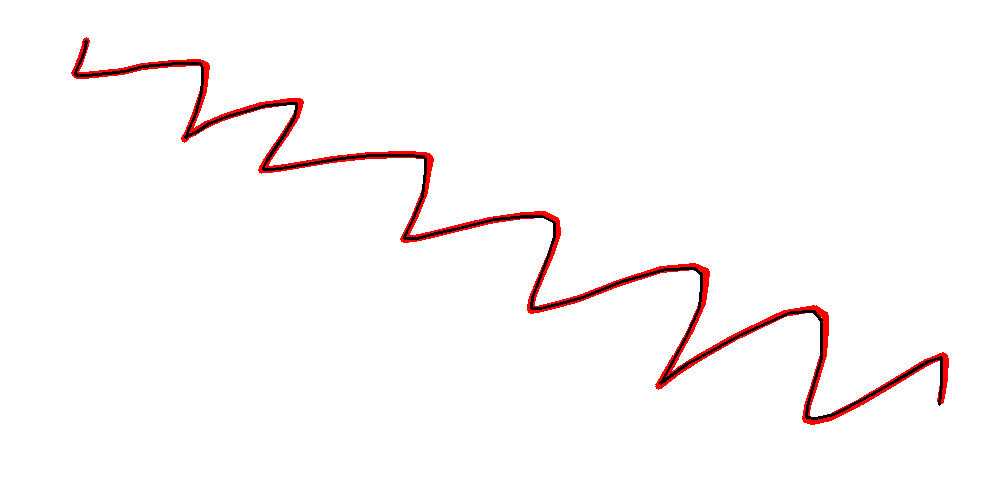
- Let's assume that the Y position of the mouse on a window is used to describe the height of a filled rectangle that starts from the top-left corner of the window and has a width that equals the window's width. Write a Kalman filter that can be used to correct the height of the rectangle (single value) and remove noise in the mouse's movement, resulting in a visually smooth resizing of the filled rectangle.
int fillHeight = 0;
void onMouse(int, int, int y, int, void*)
{
fillHeight = y;
}
int main()
{
KalmanFilter kalman(2,1);
Mat_<float> tm(2, 2); // transition matrix
tm << 1,0,
0,1;
kalman.transitionMatrix = tm;
Mat_<float> h(1,1);
h.at<float>(0) = 0;
kalman.statePre.at<float>(0) = 0; // init x
kalman.statePre.at<float>(1) = 0; // init x'
setIdentity(kalman.measurementMatrix);
setIdentity(kalman.processNoiseCov,
Scalar::all(0.001));
string window = "Canvas";
namedWindow(window);
setMouseCallback(window, onMouse);
while(waitKey(10) < 0)
{
// empty canvas
Mat canvas(500, 500, CV_8UC3, Scalar(255, 255, 255));
h(0) = fillHeight;
Mat estimation = kalman.correct(h);
float estH = estimation.at<float>(0);
rectangle(canvas,
Rect(0,0,canvas.cols, estH),
Scalar(0),
FILLED);
imshow(window, canvas);
kalman.predict();
}
return 0;
}
- Create a BackgroundSubtractorMOG2 object to extract the foreground image's contents while avoiding the shadow changes.
Ptr<BackgroundSubtractorMOG2> bgs =
createBackgroundSubtractorMOG2(500, // hist
16, // thresh
false // no shadows
);
- Write a program to display the current (as opposed to sampled) background image using a background segmentation algorithm.
VideoCapture cam(0);
if(!cam.isOpened())
return -1;
Ptr<BackgroundSubtractorKNN> bgs =
createBackgroundSubtractorKNN();
while(true)
{
Mat frame;
cam >> frame;
if(frame.empty())
break;
Mat mask;
bgs->apply(frame,
mask);
bitwise_not(mask, mask);
Mat bg;
bitwise_and(frame, frame, bg, mask);
imshow("bg", bg);
int key = waitKey(10);
if(key == 27) // escape key
break;
}
cam.release();