This chapter offers a quick overview of the main classes, structs, and enums of the System.Security.Cryptography namespace, including cryptographic services, secure encoding and decoding process of the data, and many other operations, such as hashing, generation of random numbers, and message authentication. More details about cryptographic services and their implementations can be seen in Chapter 7.
The goal of this chapter is to provide a comprehensive roadmap for professionals, offering a clear overview of the cryptographic services in the.NET Framework.
Classes
System.Security.Cryptography Namespace
Class | Description |
---|---|
AesCng | Implements support for CNG for the Advanced Encryption (AES) algorithm |
CngAlgorithm | Encapsulates the name of an encryption algorithm |
CngAlgorithmGroup | The same as CngAlgorithm, but the encapsulation is done for a group of encryption algorithms |
CngKey | Defining the functionalities of the cryptographic keys used with CNG |
CngKeyBlobFormat | The key is declared as the BLOB format and is used with CNG objects. |
CngKeyCreationParameters | Advanced support for properties regarding the key creation |
CngPropertyCollection | Provides strong support for a typed collection related to CNG properties |
CngProvider | The name of the key storage provider (KSP) is encapsulated in order to be used further with CNG objects. |
CngUIPolicy | An optional configuration parameter for the user interface (UI) CNG displays when a protected key is being accessed. It also provides encapsulation for the configuration. |
DSACng | Support for the CNG implementation of the Digital Signature Algorithm (DSA) |
DSAOpenSsl | Offers implementation for DSA with OpenSSL support |
ECDiffieHellmanCng | Offers support for the CNG implementation of the Elliptic Curve Diffie-Hellman (ECDH) algorithm. The class’ purpose is to perform cryptographic operations. |
ECDiffieHellmanCngPublicKey | Creates a public key for ECDH which can be used with the ECDiffieHellmanCng class |
ECDiffieHellmanOpenSsl | Offers support for the implementation of the Elliptic Curve Diffie-Hellman (ECDH) algorithm supported by OpenSSL |
ECDsaCng | Offers CNG support and implementation for ECDSA |
ECDsaOpenSsl | Offers support for the implementation of the Elliptic Curve Digital Signature Algorithm (ECDSA) based on OpenSSL |
ProtectedData | Offers methods for encrypting and decrypting data. The class cannot be inherited. |
RSACng | Offers CNG support and implementation for the RSA algorithm |
RSAOpenSsl | Offers support and implementations for RSA with OpenSSL support |
SafeEvpPKeyHandle | Represents a special pointer (EVP_PKEY*) for OpenSSL |
TripleDESCng | Offers CNG support and implementation for the Triple Data Encryption Standard (3DES) algorithm |
Structs
Structs within the System.Security.Cryptography Namespace
Struct | Description |
---|---|
CngProperty | It provides encapsulation for the property of a CNG key or provider. |
Enums
Enums within the System.Security.Cryptography Namespace
Enum | Description |
---|---|
CngExportPolicies | Key export policies for a cryptographic key |
CngKeyCreationOptions | Options for key creations |
CngKeyHandleOpenOptions | Options for opening key handles |
CngKeyOpenOptions | Options for opening a key |
CngKeyUsages | Cryptographic operations for CNG keys that are used with it |
CngPropertyOptions | CNG key property options |
CngUIProtectionLevels | Protection level related to the key used in a user interface and its scenarios |
DataProtectionScope | Gets the scope of the data protection that can be applied using the Protect(Byte[], Byte[], DataProtectionScope) method |
ECKeyXmlFormat | Defines and offers support for XML serialization formats for elliptic curve keys |
Security Model in .NET Framework
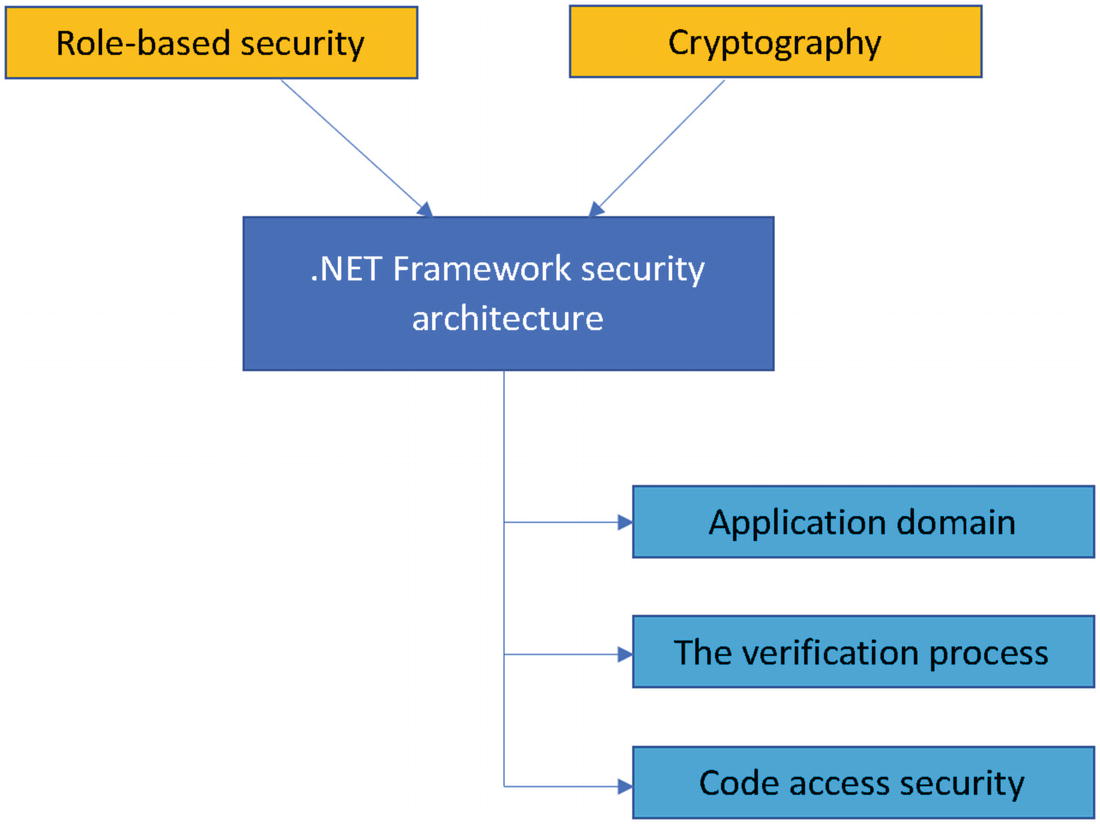
.NET Framework security architecture with role-based security
Looking at the components that form the security architecture during the process of implementing cryptographic mechanisms, it’s important to understand the definitions and borders of each of the components. The application domain provides a certain level of isolating processes and is necessary to make sure that the code running in the application cannot be affected by an adversary. Based on this aspect, the isolation boundary within the application domain provides an isolation boundary for security, reliability, and versioning. Most of the application domains are created during the runtime hosts.
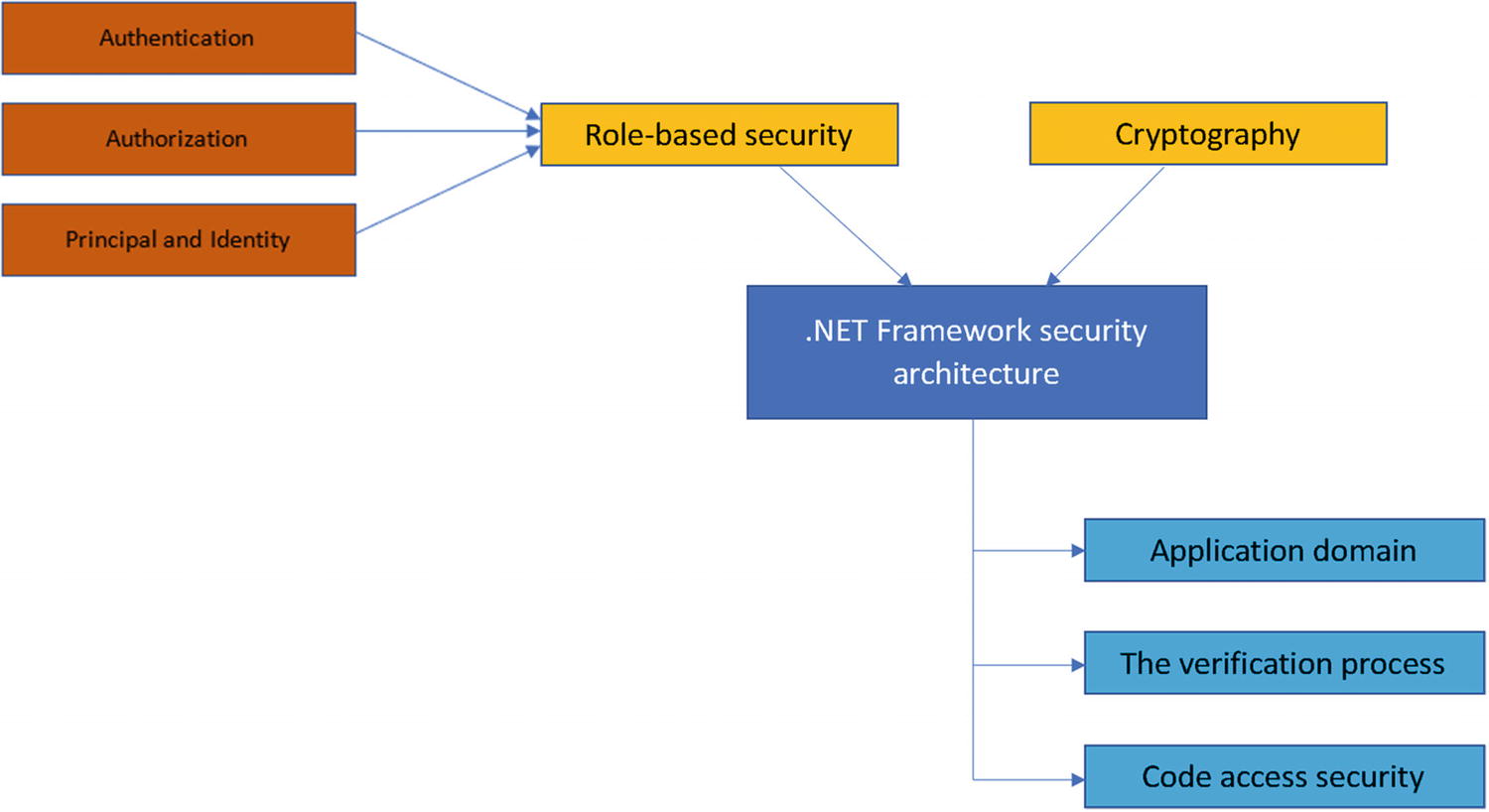
.NET Framework security architecture with role-based security
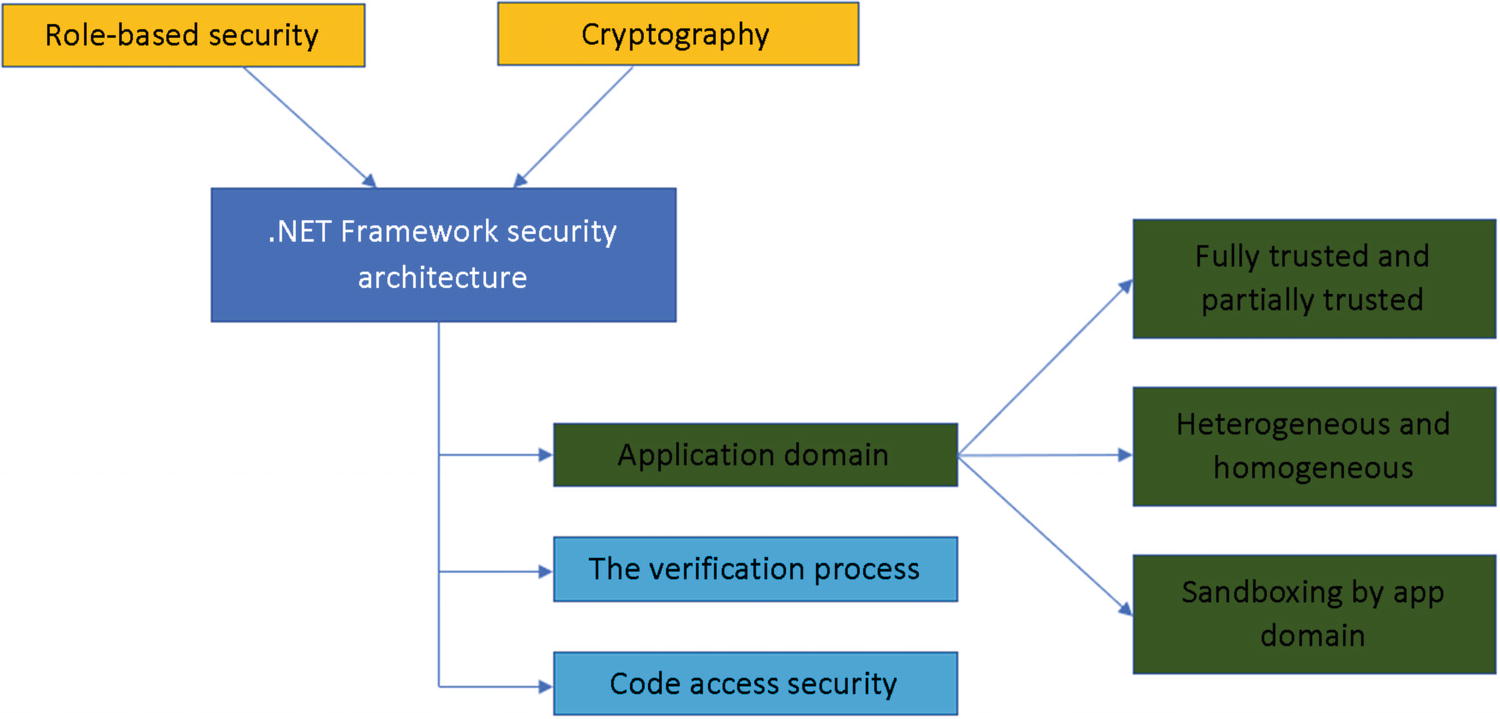
.NET Framework security architecture with application domain components
Fully Trusted or Partially Trusted Example
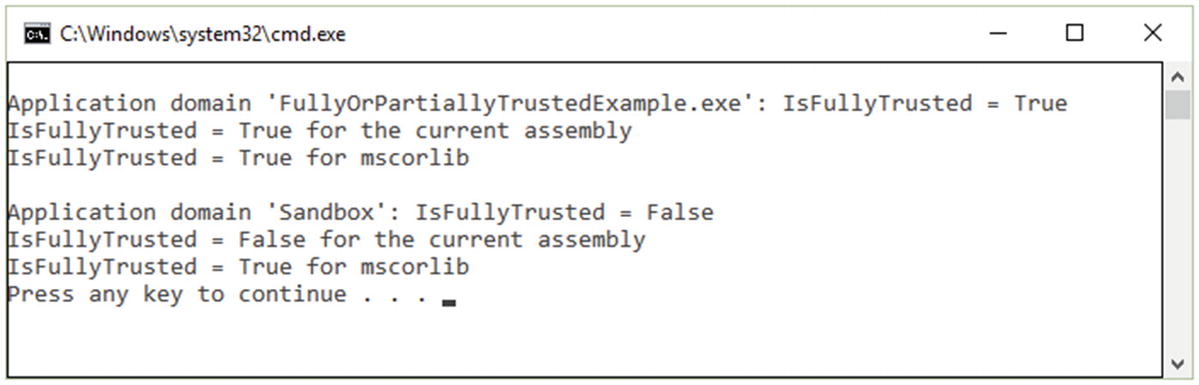
The output of the application domain
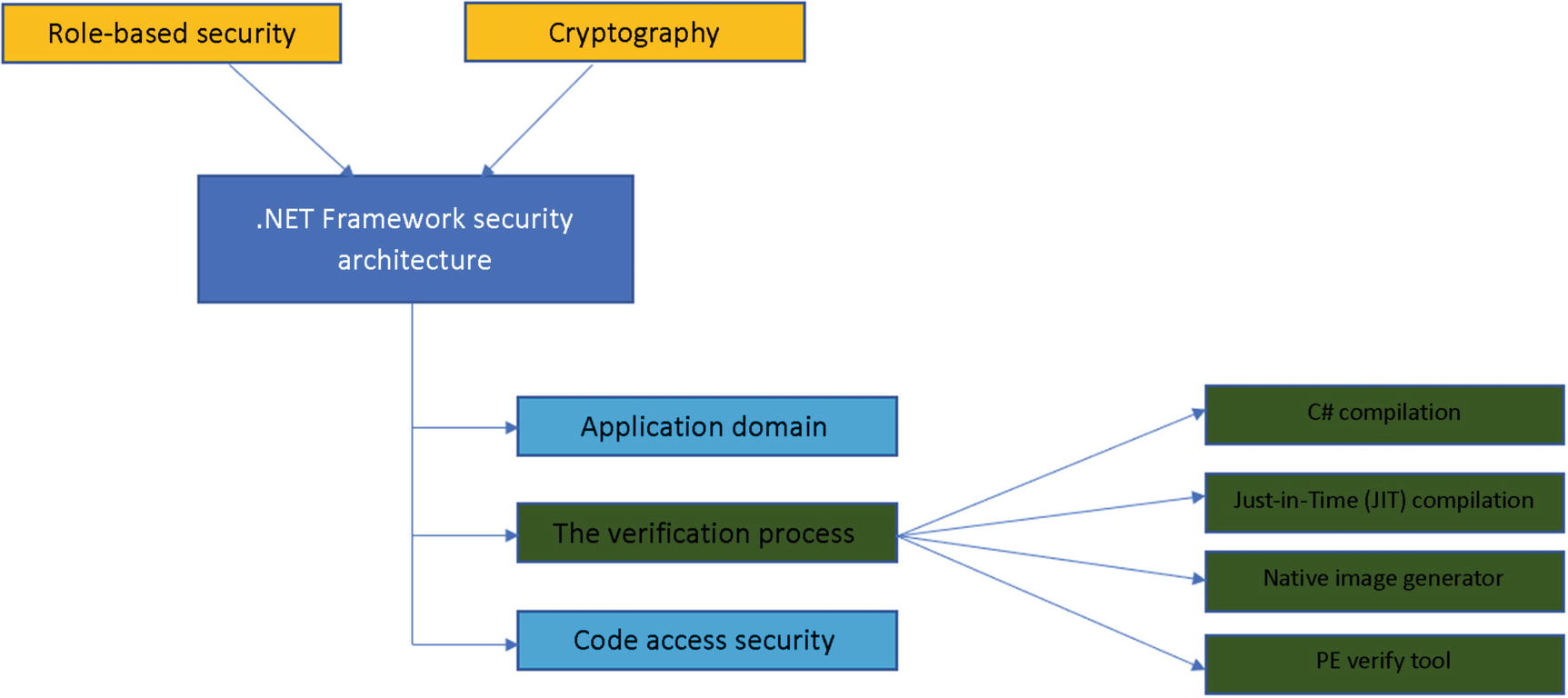
.NET Framework security architecture with the verification process components
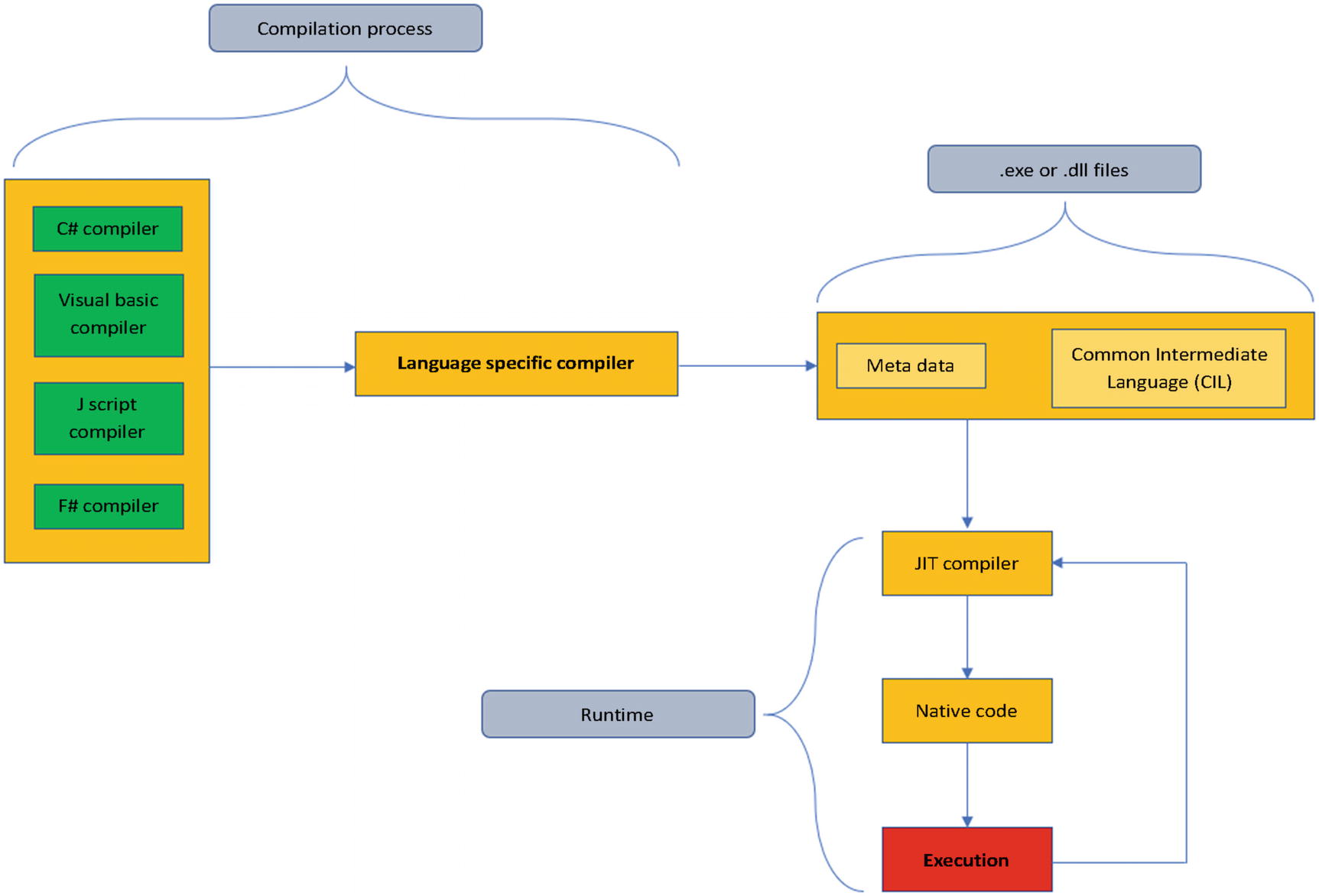
JIT compilation process
Many security specialists and professionals consider this a gap in the process of assuring the security of the application because of the multiple points that can be exploited by an attacker, such as dissembling .exe or .dll files with the proper tools and proceeding with software obfuscation attacks.
Once the JIT process is completed, we can move further to the native generator image (NGEN) . NGEN is a powerful tool that improves the performance of managed applications. NGEN, which can be found as Ngen.exe, creates (compiles) native images (for example, ISO files), which contain files that are compiled in a manner specific for processors as machine code, and deploys them on the native image cache of the end user’s local computer. This is a dangerous point due to the fact that we never know who and what the users will have on their computers and if a malicious user is waiting for the proper moment to exploit in order to find their vulnerability point.
The last step is based on the PE Verify Tool or Peverify.exe. It's a very useful tool that offers an a significant amount of help to developers who are generating Microsoft Intermediate Language (MSIL), such as compiler writers, with the goal of determining if the safety requirements are met and expected with their MSIL code.
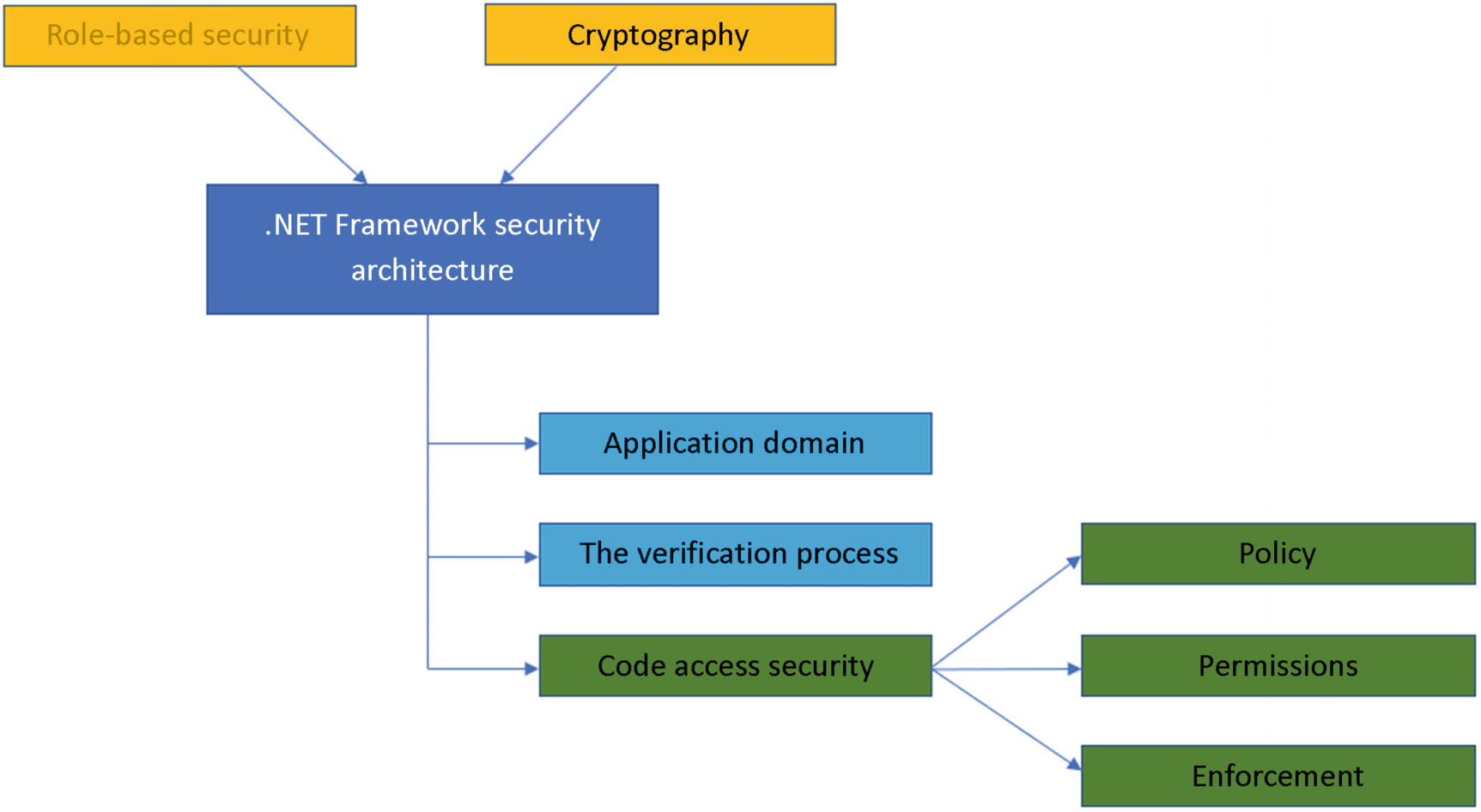
The .NET Framework security architecture with the code access security components
Policy , permissions , and enforcement should be seen as a whole unit, as a “heart” that beats for each level of trust on different code that is running in the same application.
Type-safe code: A special type of code that accesses only those types that are well-defined
Imperative and declarative syntax: The code that targets a common language runtime has the ability to have an interaction with the security system. The interaction is to request permissions and override certain security settings.
Secure class libraries: The libraries have a usage of security demands and their main goal is to ensure that those who are using and calling the library have the proper permissions to access the library and its resources.
Transparent code: With .NET Framework 4 professionals have the ability to determine if the code can run as security-transparent. Also, identifying permissions is one of the tasks that need to be fulfilled by determining the transparence allowance.
Declarative Security
The syntax that is used by the declarative security is based on the attributes that are used in order to insert security information within the metadata of the code written by professional developers.
Example of Declarative Security
The SecurityAction.Demand specifies that the invokers need that permission for running the code.
Imperative Security
The syntax provided by imperative security invokes a security call to create a new instance of the object that holds the permission. The imperative security can be used to invoke demand and to override. It is very important that no requests are done. This is impossible to fulfill within imperative security.
Example of Imperative Security
Conclusion
In this chapter, we discussed the System.Security.Cryptography namespace and we gave an overview of its classes, structs, and enums by pointing out their main designation. We continued our journey by explaining why professionals should work based on the .NET Framework security model, respecting their components and following the workflow as a necessary guideline.
A big picture of the System.Security.Cryptography namespace
An understanding of the main designations of the classes, structs, and enums
An understanding of the vital points of the security model within .NET Framework which can lead to a security disaster
A clear image of how the main components work and communicate, such as the application domain, verification process, and code access security
An in-depth understanding of JIT compilation and its process
An understanding of how the verification process can expose security breaches
An understanding of how, when, and why to use declarative and imperative security and an overview of their importance during the implementation process
Bibliography
- [1]
Sarcar Vaskaran. Interactive C#: Fundamentals, Core Concepts and Patterns. Apress, 2018.
- [2]
Andrew Troelsen and Philip Japikse. Pro C# 7 with .NET and .NET Core. Apress, 2017.
- [3]
Joshi Bipin. Beginning XML with C# 7: XML Processing and Data Access for C# Developers. Apress, 2017.