- Start by declaring the different libraries we would be using for this recipe in the assignment.rs script:
// Primitive libraries in rust
use std::{i8,i16,i32,i64,u8,u16,u32,u64,f32,f64,isize,usize};
use std::io::stdin;
The use statement tells the compiler that the program would use the following properties of the library. The std is an inbuilt library that comes along with the Rust compiler and doesn't need to be externally downloaded. i8 and i16 are different data types of the variable that will be used in the program, and stdin helps us accept user input from the user:
fn main() {
println!("Understanding assignment");
// Compiler will automatically figure out the data type if
not mentioned
// Cannot change the value
let num =10;
println!("Num is {}", num);
}
- The output of the preceding script is as follows:

- Replace the main function of the preceding script in assignment.rs file with the following code snippet below:
fn main(){
let age: i32 =40;
println!("Age is {}", age);
// Prints the max and min value of 32bit integer
println!("Max i32 {}",i32::MAX);
println!("Max i32 {}",i32::MIN);
}
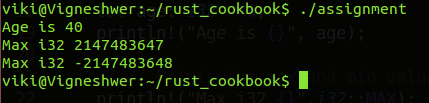
- In the previous code snippet, we declared a variable named age and explicitly told the compiler that it's a 32-bit integer type data and that we are printing the maximum and minimum values of the 32-bit int data type. Now, the next piece of code:
// Another way of variable assigning
let(f_name,l_name)=("viki","d");
println!("First name {0} and last name {1}",f_name,l_name);
Here, we have declared two variables in f_name and l_name using brackets(). This is a way to declare multiple variables in a single statement in Rust. Similarly, while printing them, we can number the position of the variable to determine which variable has to be printed first.