Send a string to the Smart Contract through the web front end for storing its hash on the blockchain
Send Ethers to the Smart Contract to pay for the notarization service
Verify if a string’s hash has been previously stored on the blockchain
We need to inform the user if the string they are trying to notarize has already been saved in the blockchain.
We need to inform the user after the string has been successfully saved in the blockchain.
We also need to inform the user if the amount of Ether they sent to the contract is not of the correct amount.
So in this case, it is clear that there must be a communication mechanism to feed back to the user of the Smart Contract when certain events occur. And this is the focus of this chapter.
What Are Events in Solidity?
Tip
You are free to define the parameters of your event.
In the preceding example, DocumentNotarized is an event in Solidity. The parameters in the event – from, text, and hash – allow the Smart Contract to pass data back to the event listener.
Adding Events to the ProofOfExistence Contract
Added two events – DocumentNotarized and NotarizationError.
Before a document (string) can be notarized, it is first checked to see if the hash of the document already exists on the blockchain. If it does, the document cannot be notarized. In this case, you will fire the NotarizationError event to inform the user of this error. In this case, you also need to refund the user the Ether that was sent along this transaction, since the notarization was not successful . You do so using the msg.sender.transfer() function. The msg.value property contains the amount of Ether sent by the user.
The next check you need to perform is to ensure that the user sends exactly 1 Ether. If the user does not send the exact amount, the transaction is also rejected and you will fire the NotarizationError event to inform the user of the reason. Likewise, you will need to refund the user of the amount sent.
The DocumentNotarized event will be fired when a document has been successfully notarized.
Observe that in both events – NotarizationError and DocumentNotarized, we have the from parameter. This is because when an event is fired, it is sent to all event handlers listening for the event. The from parameter provides a way for event handlers to know if events raised are relevant to them. You will see its use in the next section.
Deploying the Contract
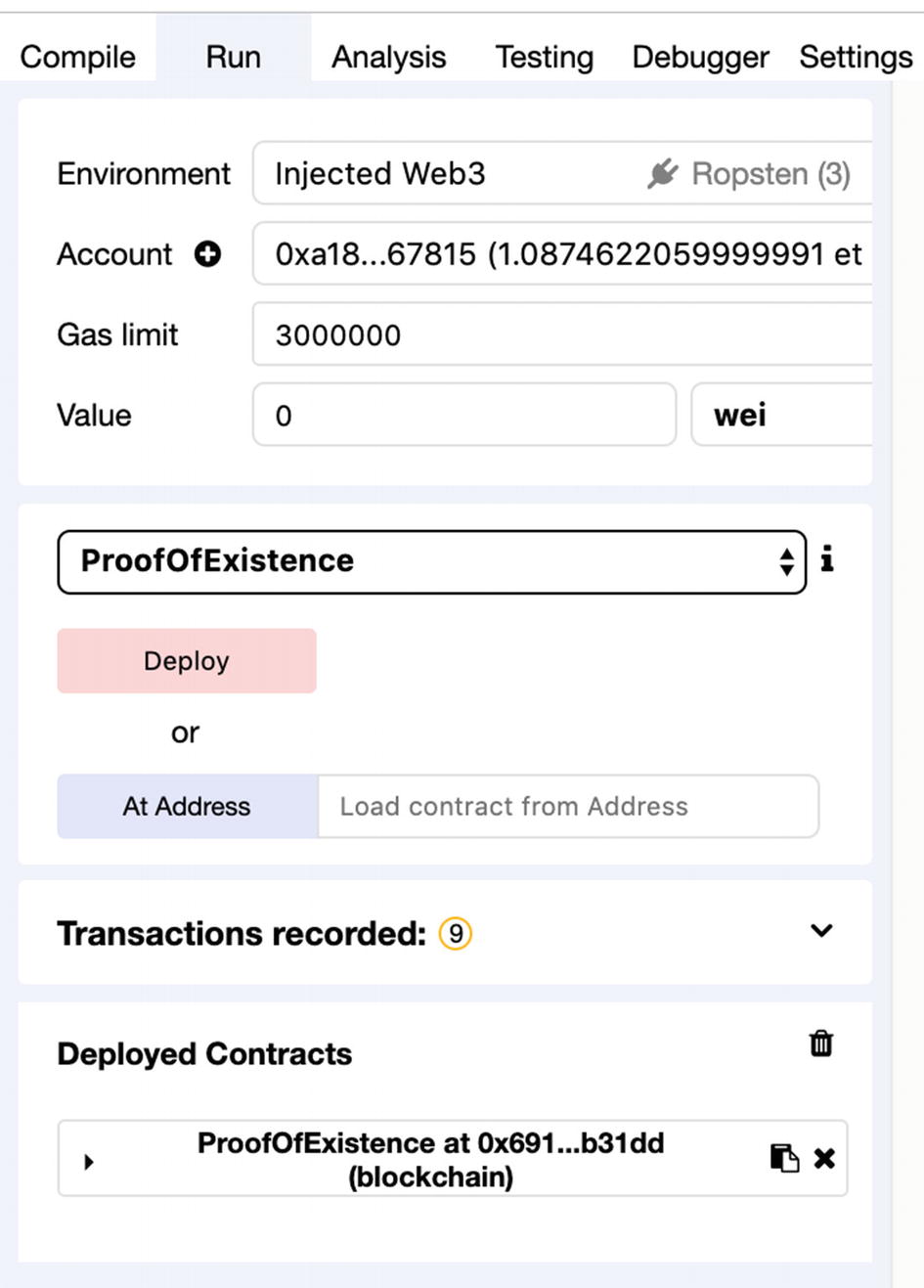
Deploying the modified contract in Remix IDE
Handling Events Using web3.js
The result argument in the callback function will contain a property named args, which in turn contains the parameters defined in your event. In the DocumentNotarized event, it will contain a reference to from, text, and hash. As mentioned earlier, when an event is fired, all clients listening to the events will be invoked. Hence it is important that you handle events only relevant to yourself. In this case, we checked the from argument and update the UI only if the value of the from argument is the same as the current account address.
Testing the Front End
To test the front end, load Chrome with the following URL: http://localhost:5000/DocumentNotarizerEvents.html.
Caution
Ensure that the serve command is running in the web3projects folder.
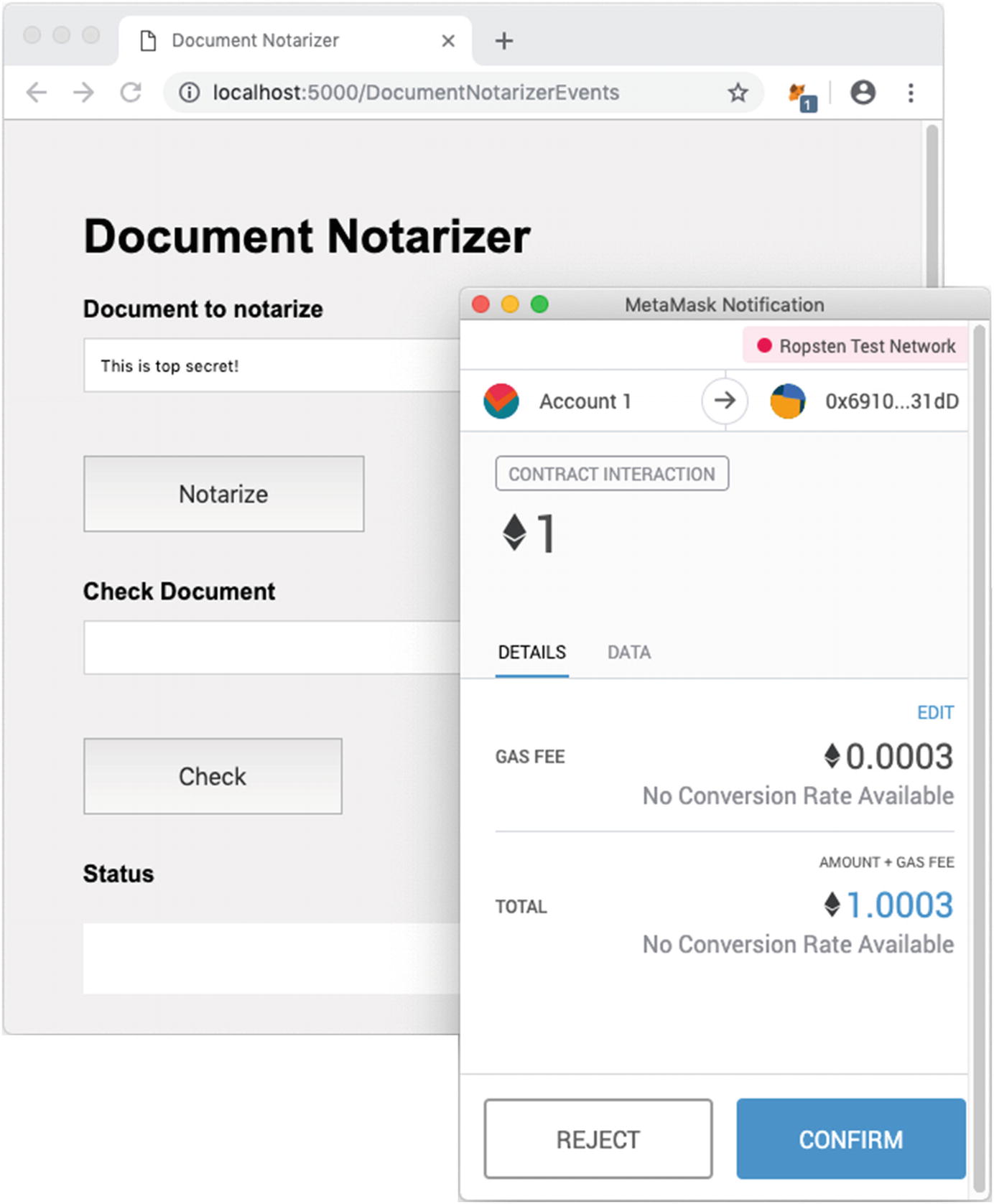
Notarizing a string
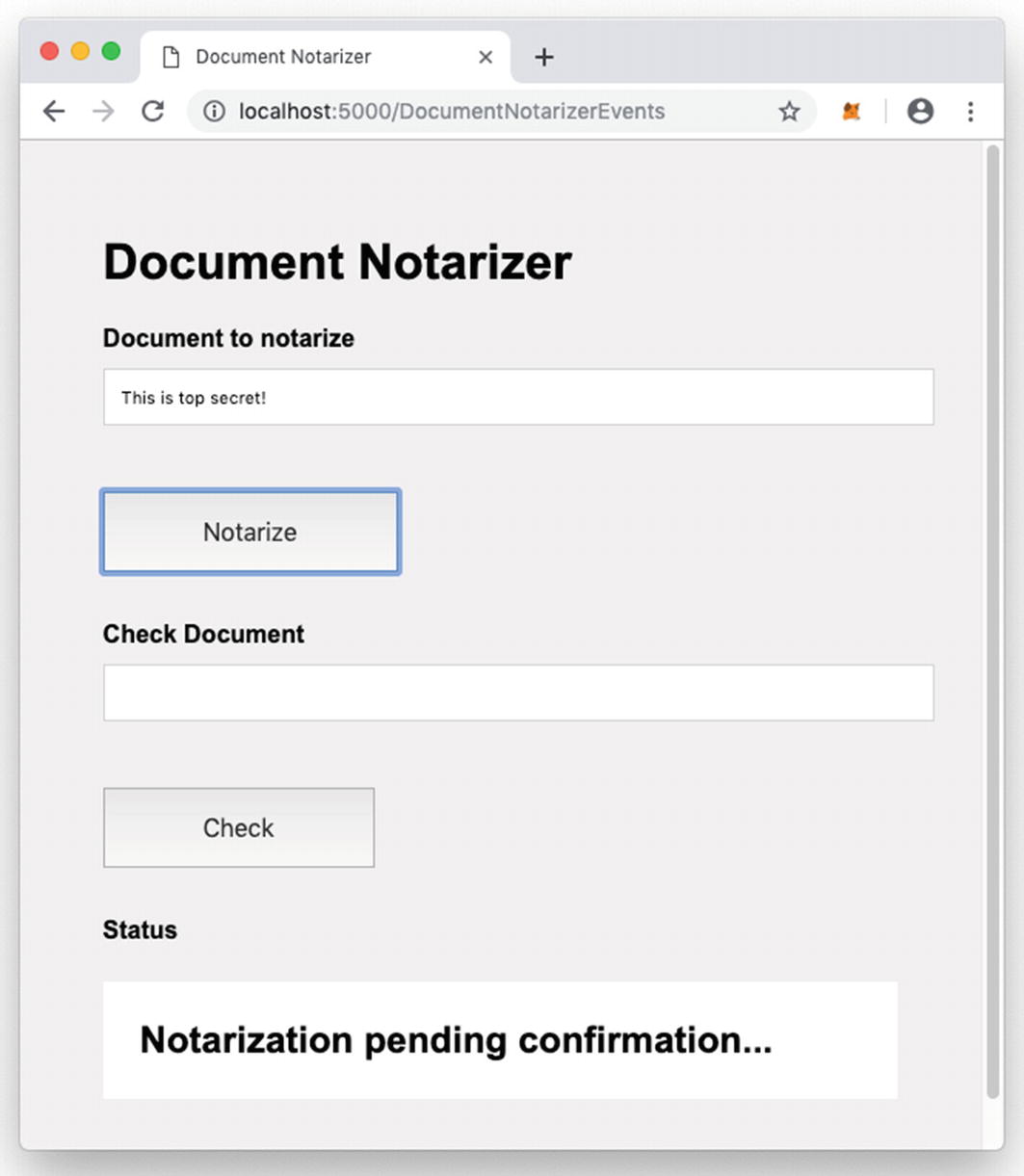
The transaction (notarization) is pending confirmation
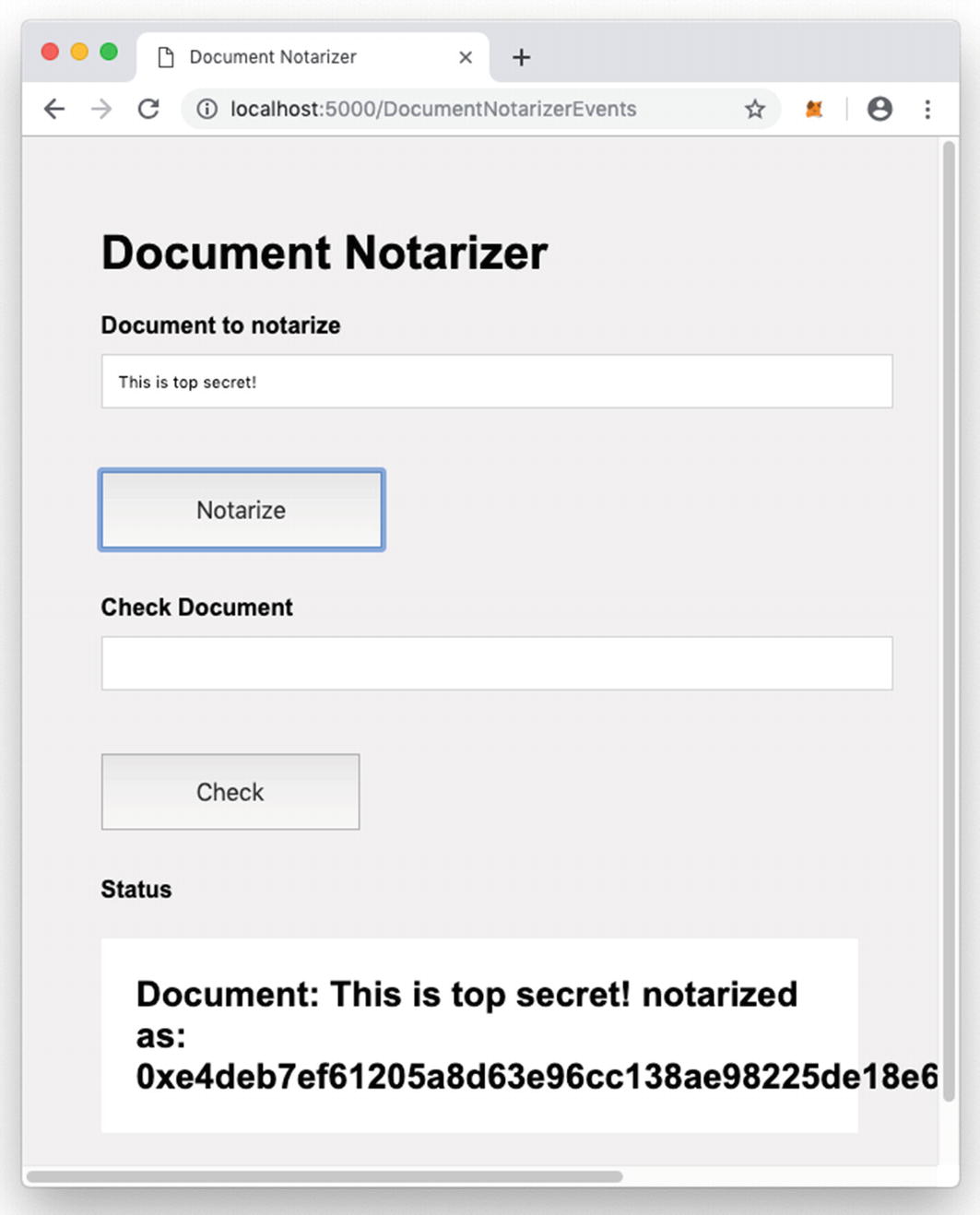
The document is notarized and its hash is returned via the event
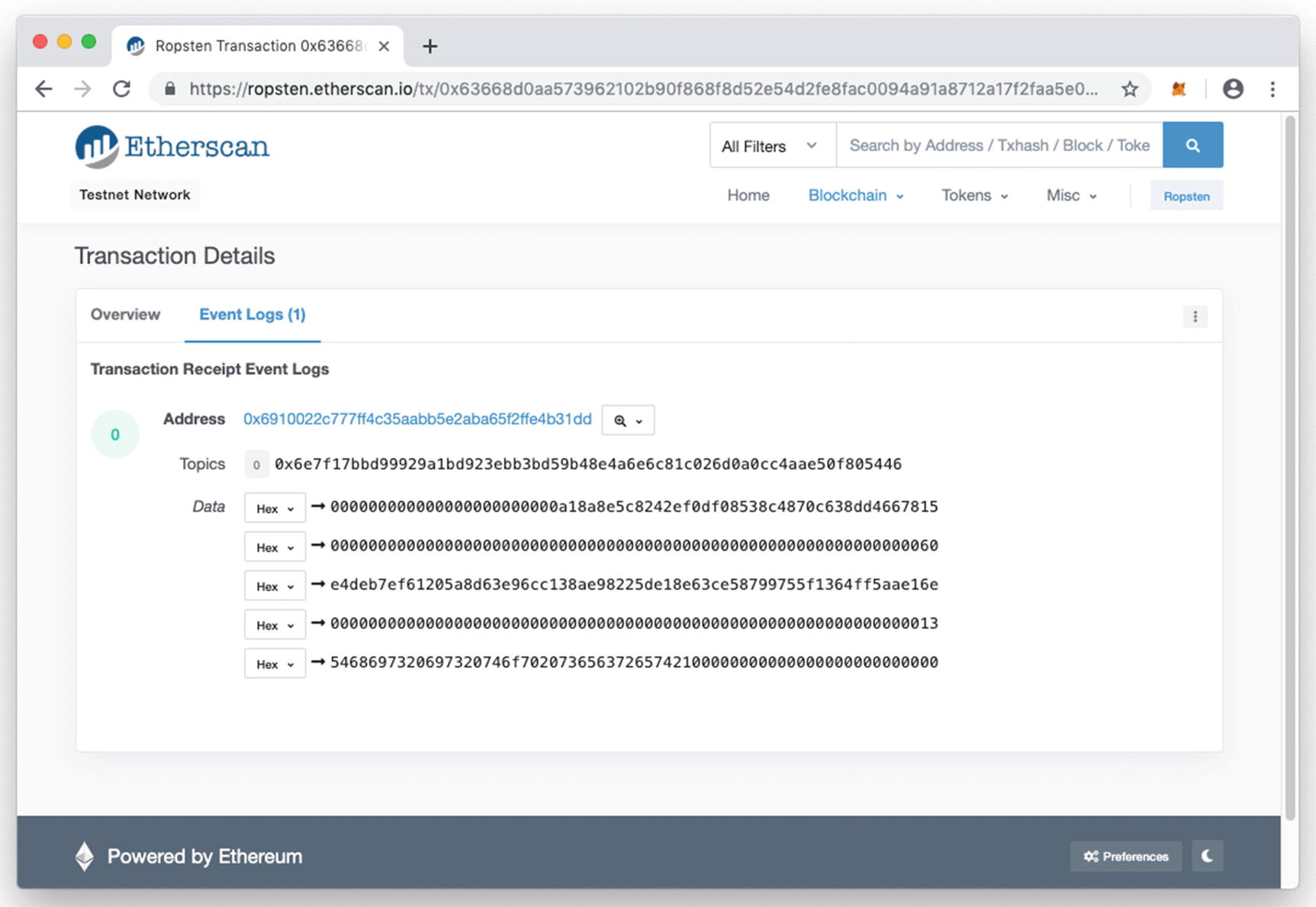
Locating the event fired by the Smart Contract
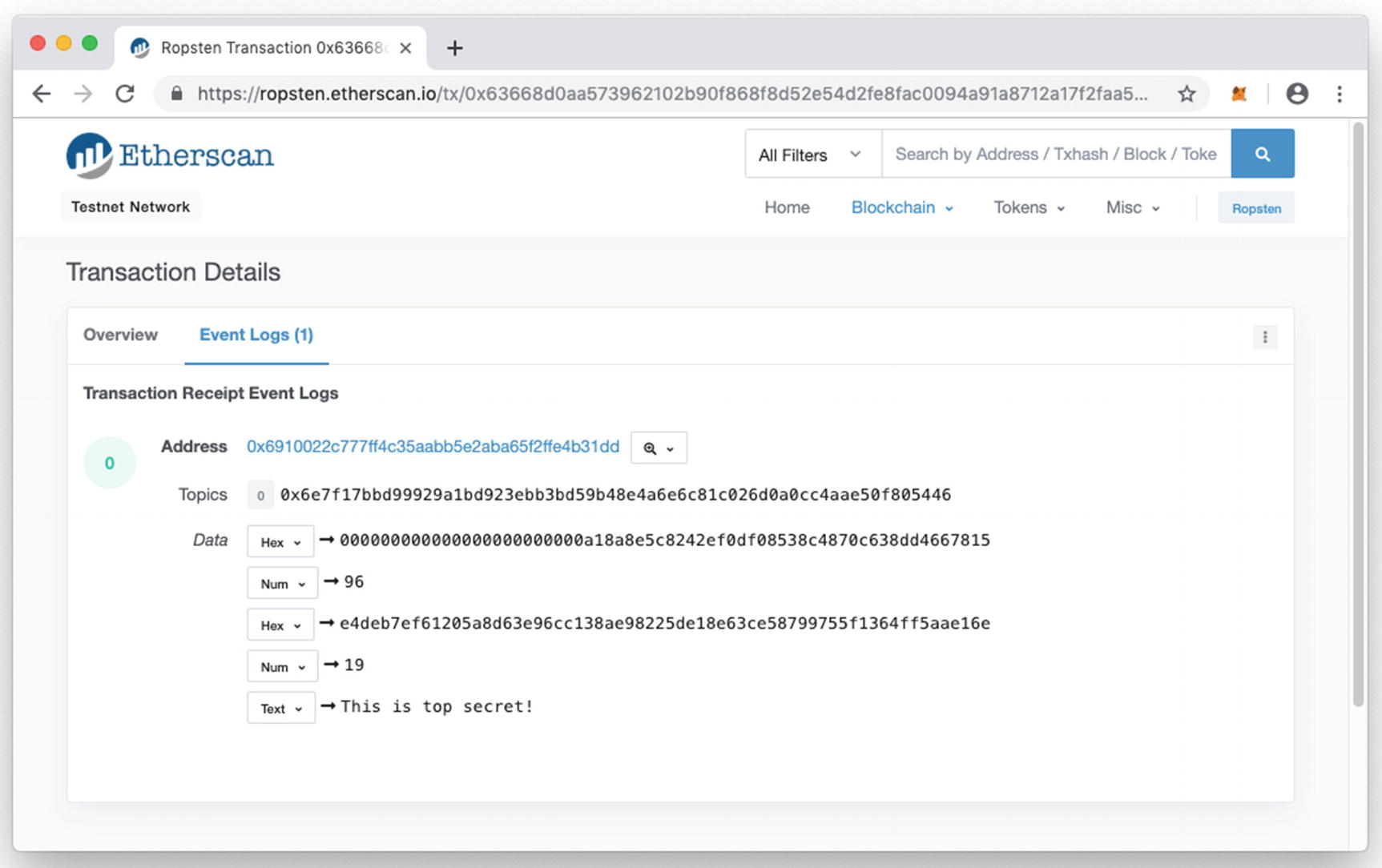
Viewing the data for the event displayed in the different format
Tip
You don’t really need to understand the data logged in the Data section. The web3.js will encapsulate all the arguments in the event.
Notarizing the Same Document Twice
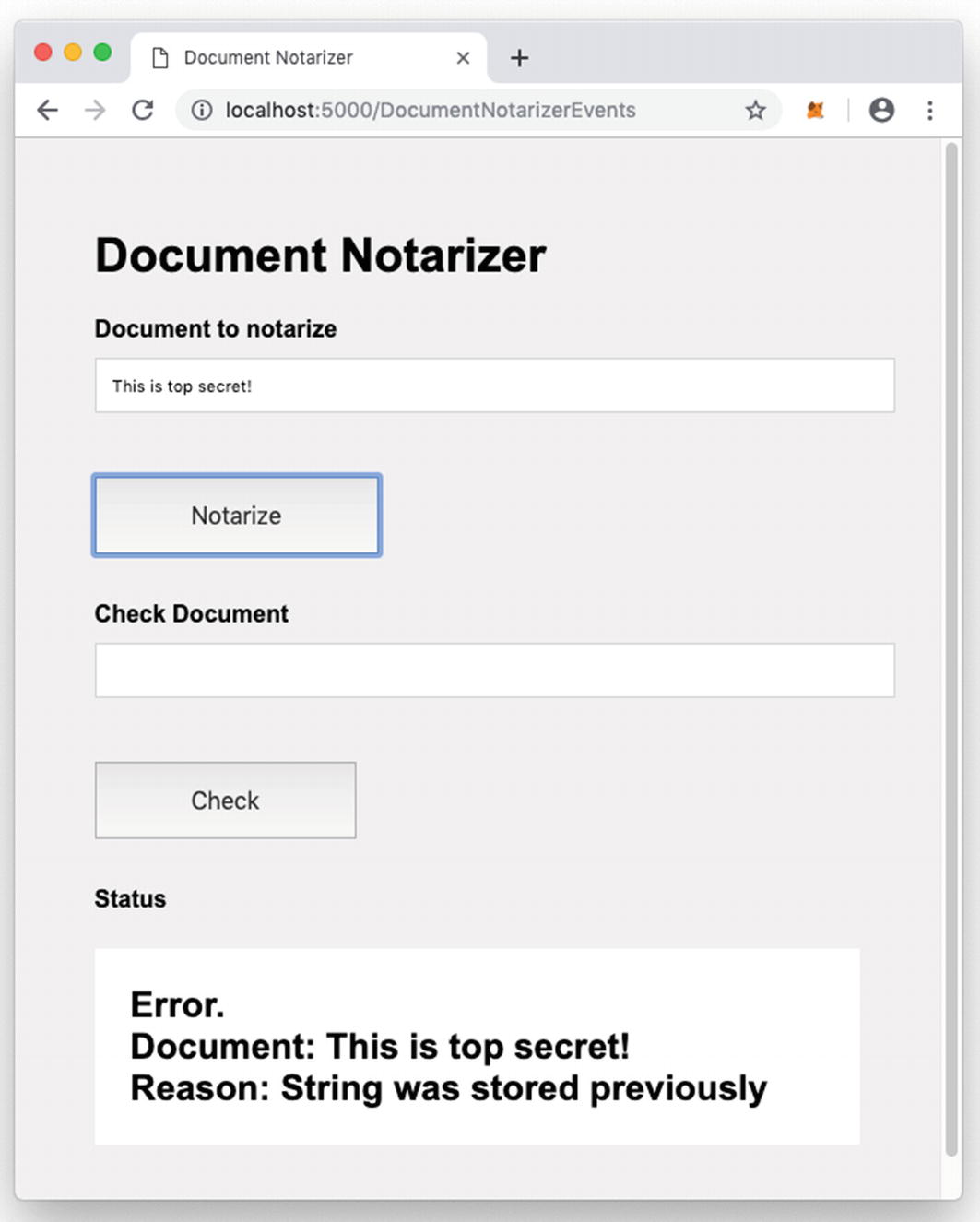
The notarization failed because it was previously notarized
Observe that besides firing the NotarizationError event, the contract also calls the msg.sender.transfer() function to refund the amount (msg.value) back to the user (msg.sender). In the next section, you will see the proof that the amount was indeed refunded back to the user.
Sending Incorrect Amount of Ether
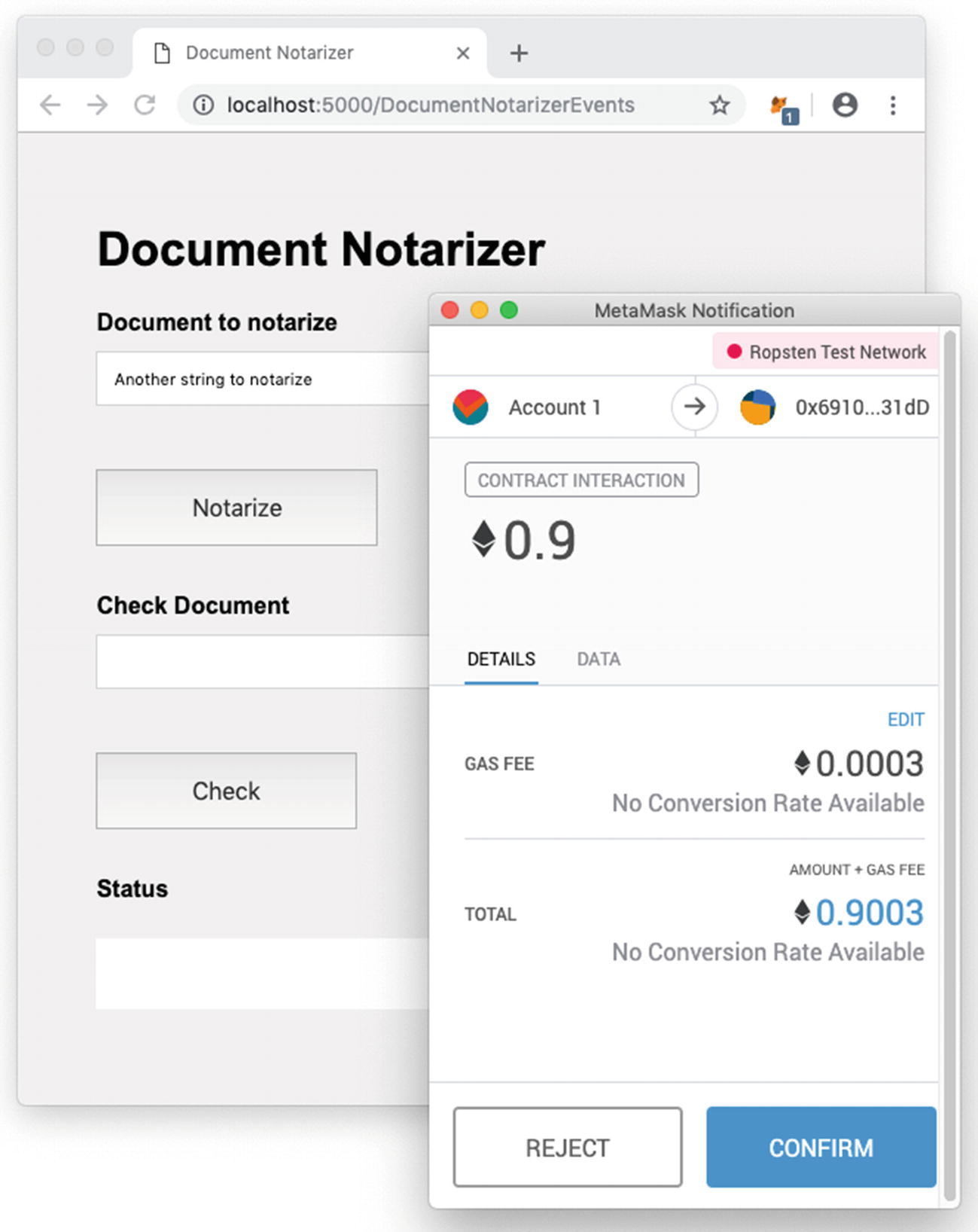
Sending insufficient amount of Ether to a Smart Contract
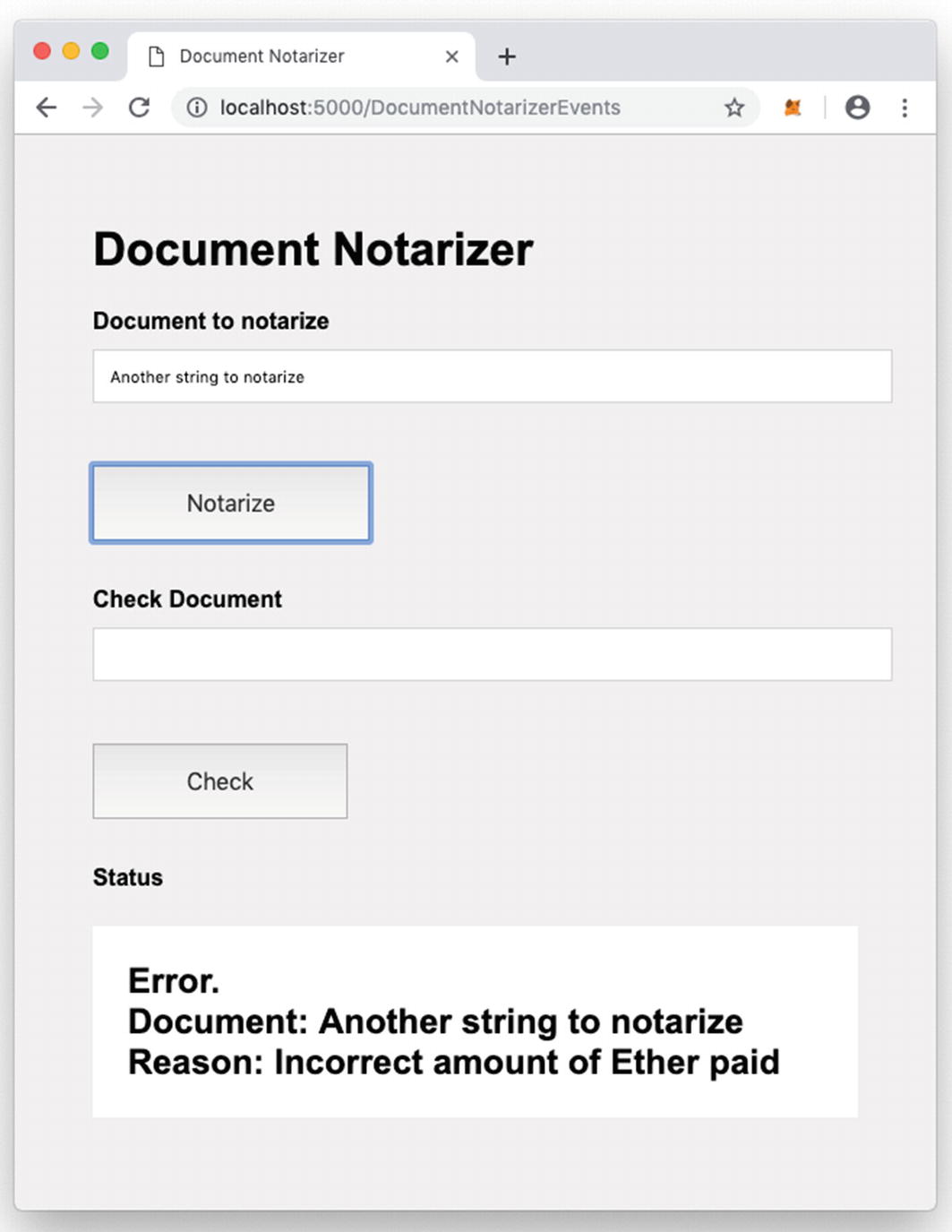
The event fired to reflect the insufficient amount of Ether sent
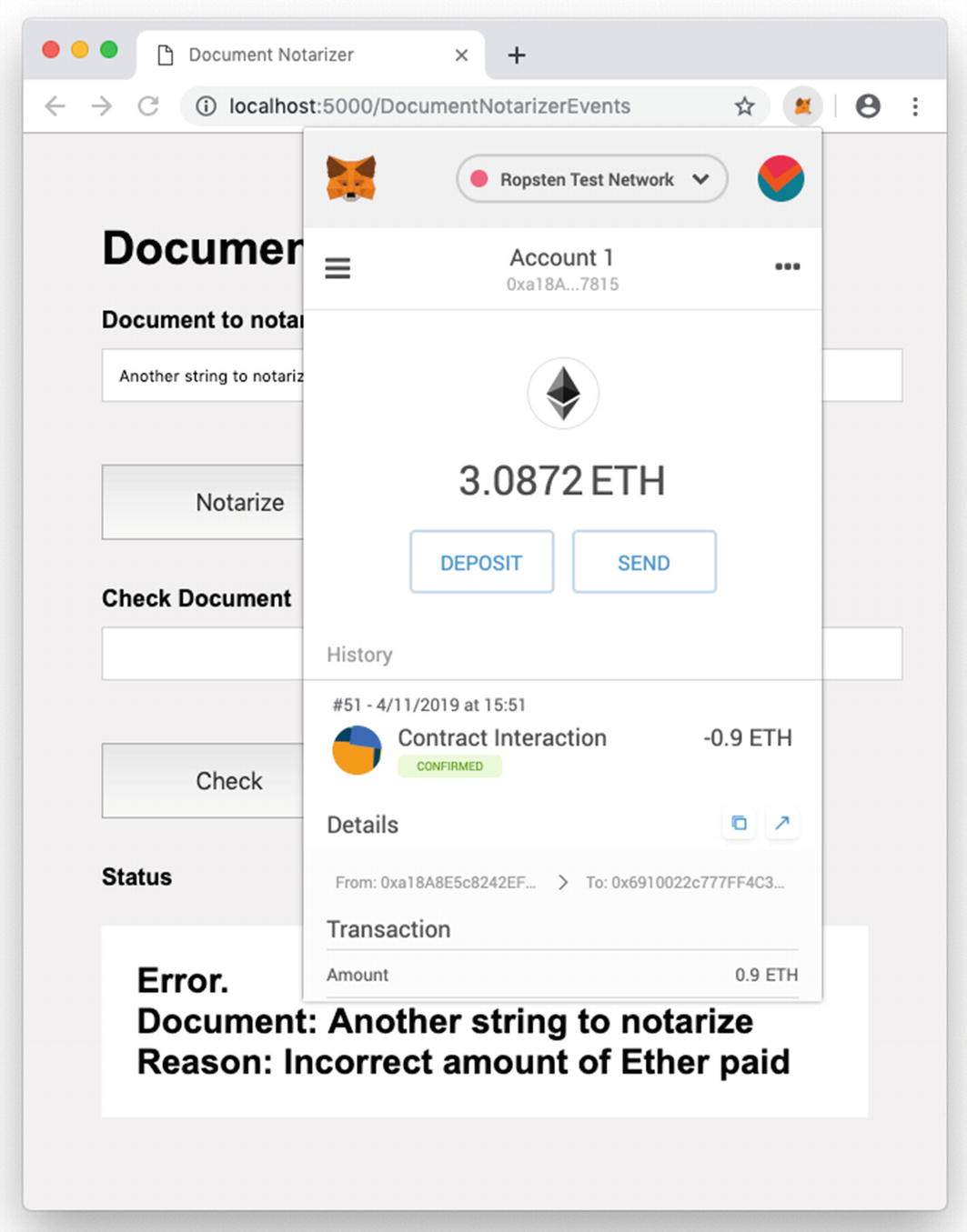
Examining the details of the transaction

The internal transfer made within the Smart Contract for the refund
Summary
In this chapter, you learned how Smart Contracts can fire events so that front-end applications that are interacting with them can handle them. Using events, you have made your document notarizer Smart Contract must more usable. In the next chapter, we will put all that we have learned about Smart Contract to build a lottery application.