With a Facebook developer account, you can add Facebook features (such as logins) to your iOS app. This chapter will walk you through that process. It is useful for many of the Facebook tools that you may want to integrate with an iOS app. Furthermore, the steps used to integrate Facebook tools are similar in some ways to the steps you would use to integrate other tools, such as Amazon Web Services (AWS), which is the topic of the next part of this book.
Also, it’s important to note that there are a number of ways to handle this integration. CocoaPods (described in Chapter 2) are a very common way of handling integration. If you look into CocoaPods, you’ll see that what you have is an automated tool for managing your Xcode project files along with having versions automatically downloaded from GitHub by CocoaPods.
The heart of the integration is Xcode, its files, and its frameworks. Although a CocoaPods interface is available for the Facebook interface, this chapter will show you what happens on the source code/Xcode side of things. Remember that regardless of the integration technique that you use, the same basic structure (updating and integrating your Xcode project) is what has to happen.
First, though, it’s the Facebook login.
Starting to Integrate the Facebook SDK with an iOS app
You need a Facebook developer account (see the previous chapter).
You need Xcode and a basic familiarity with it.
Although you can work offline with Xcode as you develop an app, you cannot work offline to create apps for Facebook or iOS, because you need to interact with the Facebook and iOS environments. If you have anything other than an ordinary Internet connection (for example, if you are behind a firewall that limits the sites you can visit), check out the basic steps to get started to make certain that you don’t need permission from another part of the organization.
Note
Both the Facebook and iOS developer sites change from time to time, so you may have to search around to find sections that have moved.
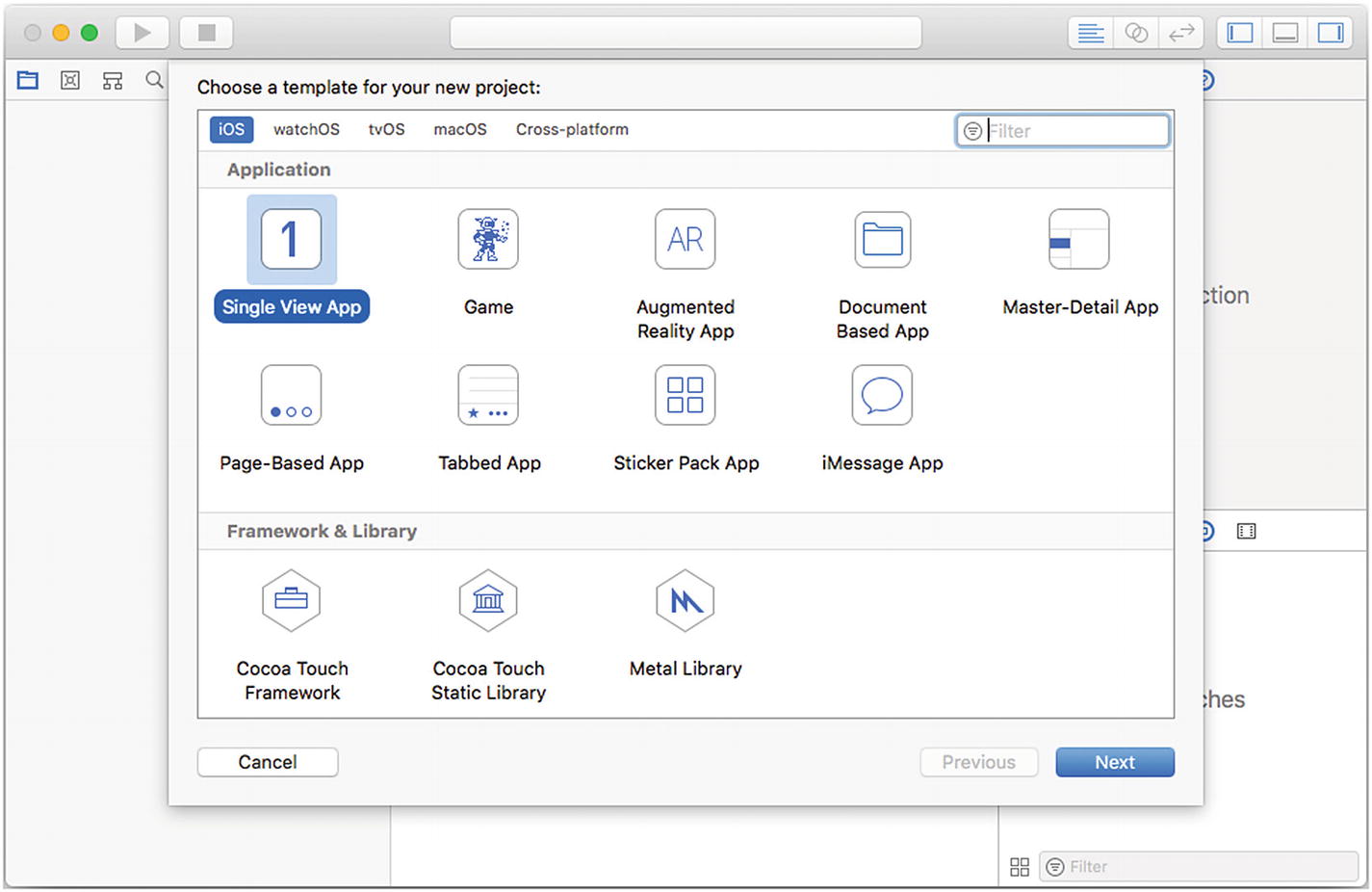
Create a single-view app to test with Facebook
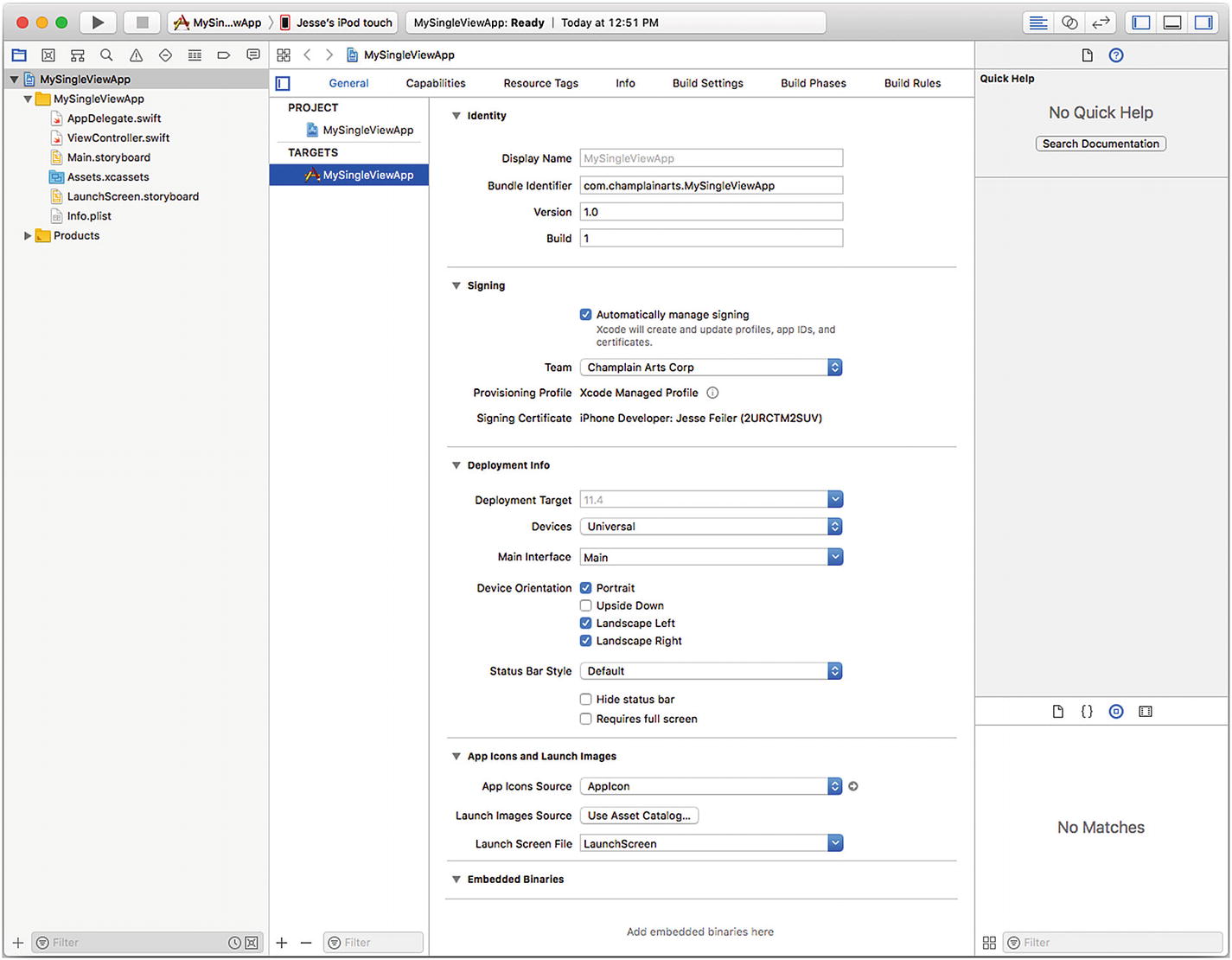
Make a note of the bundle identifier
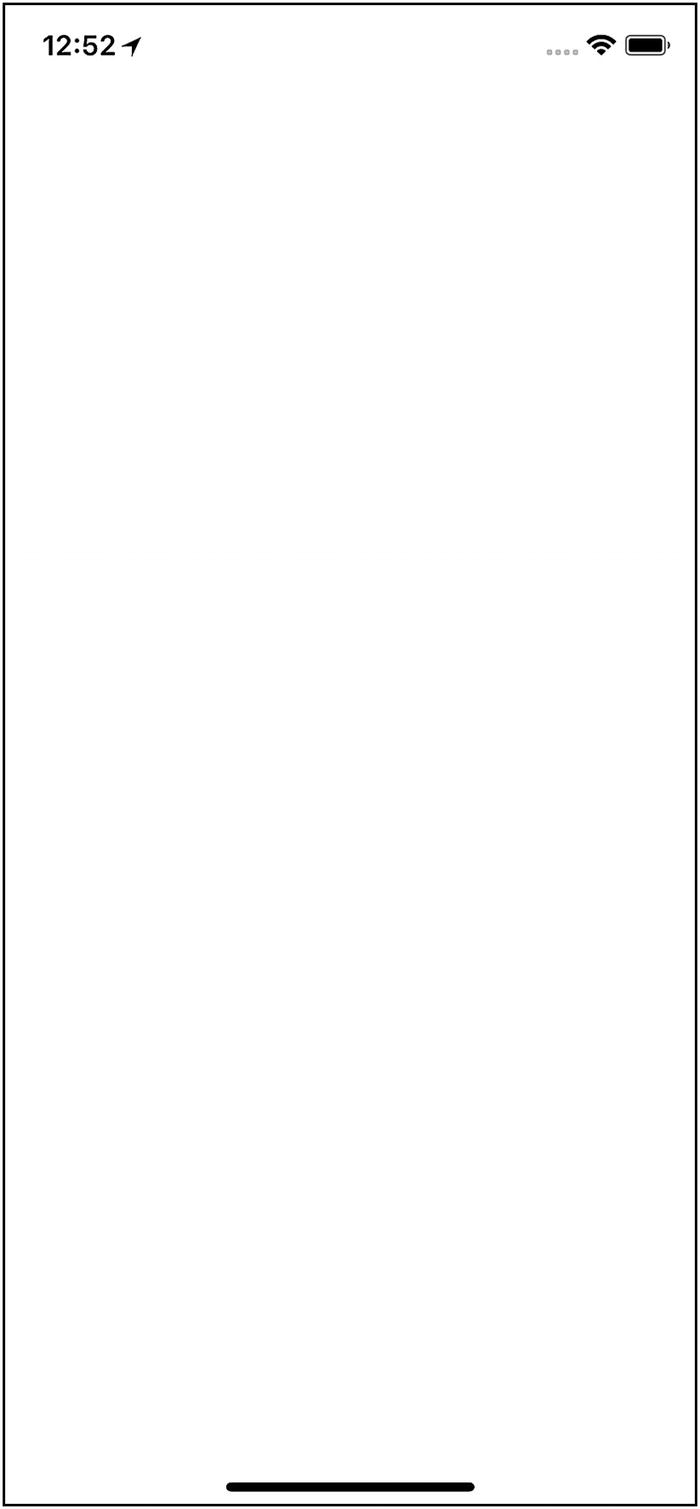
Test the app
Yes, a successful implementation of the single-view app shows nothing. As you will see later in this chapter, you can easily add a label. All you should be concerned with at this point is that the app doesn’t fail or crash when you run it on Xcode .
Download the Facebook SDK for Swift
Log in to your developer account on developers.facebook.com. You’ll see choices to download the Facebook SDK for iOS, Android, and PHP, as well as for other platforms. As of this writing, the basic iOS SDK is still written in Objective-C, but you can download the Swift version if you want (you’ll see how to do that in this section).
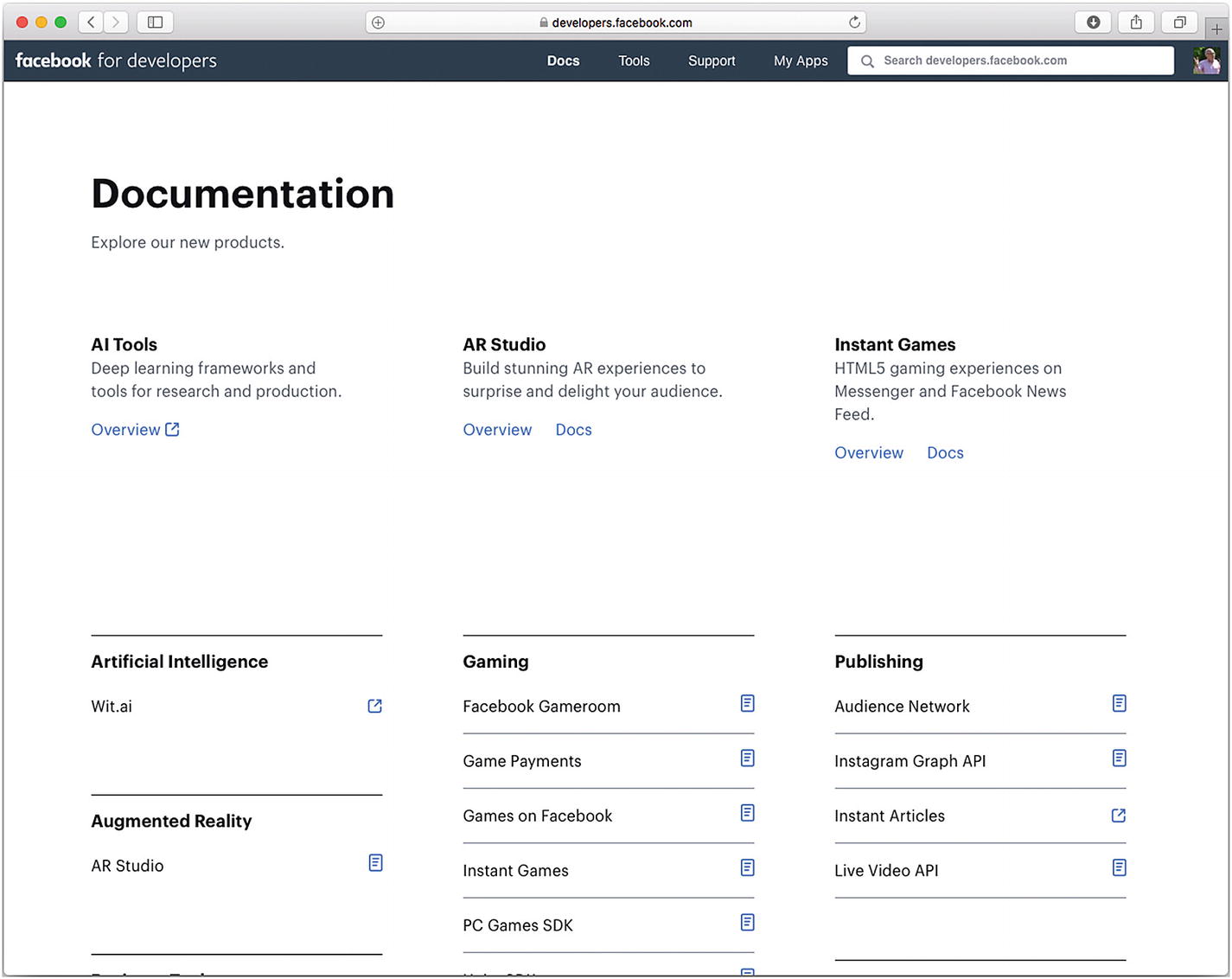
Look in Documentation for the Facebook SDK for iOS/Swift
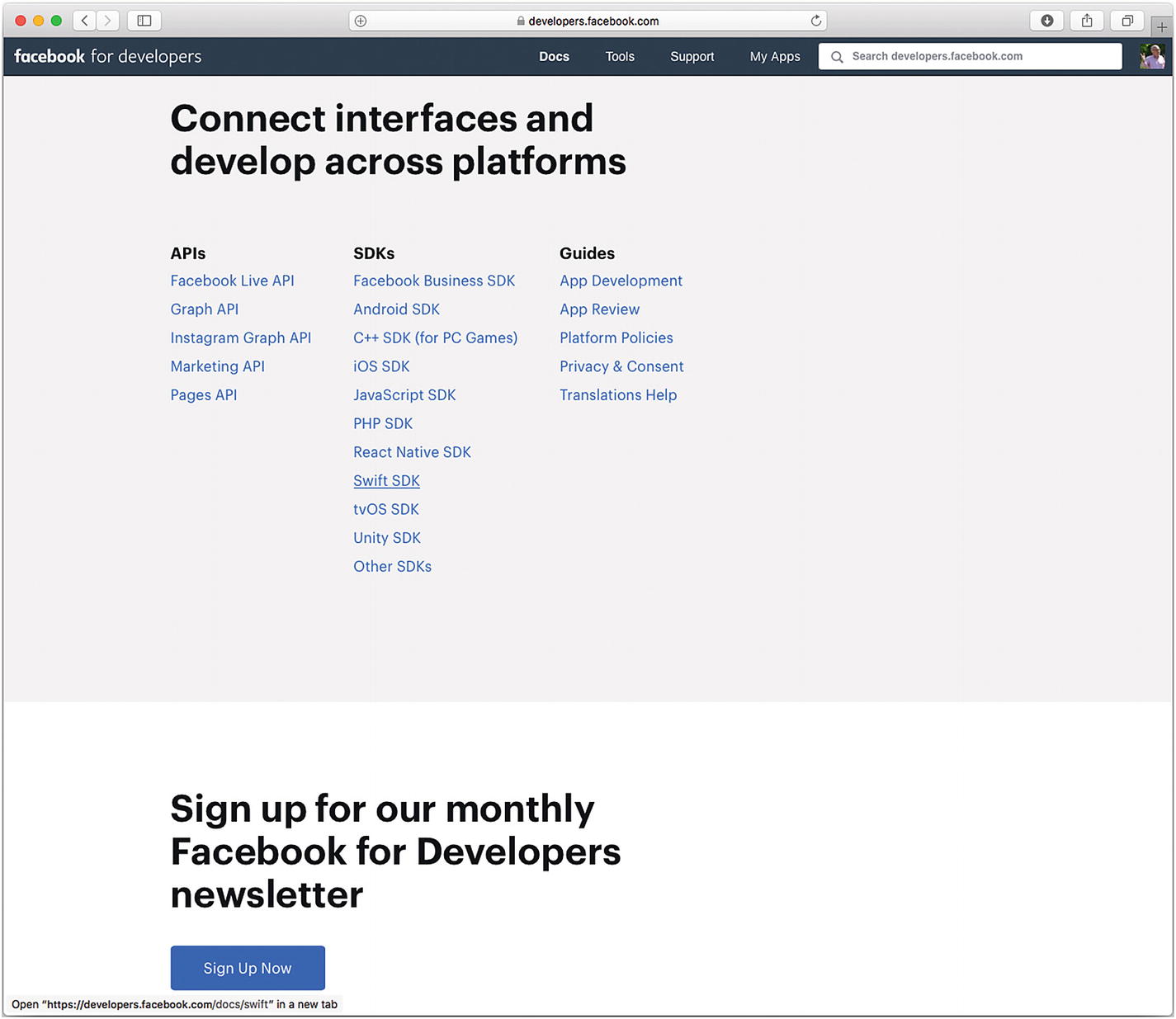
The Facebook Swift SDK is available with all of the others
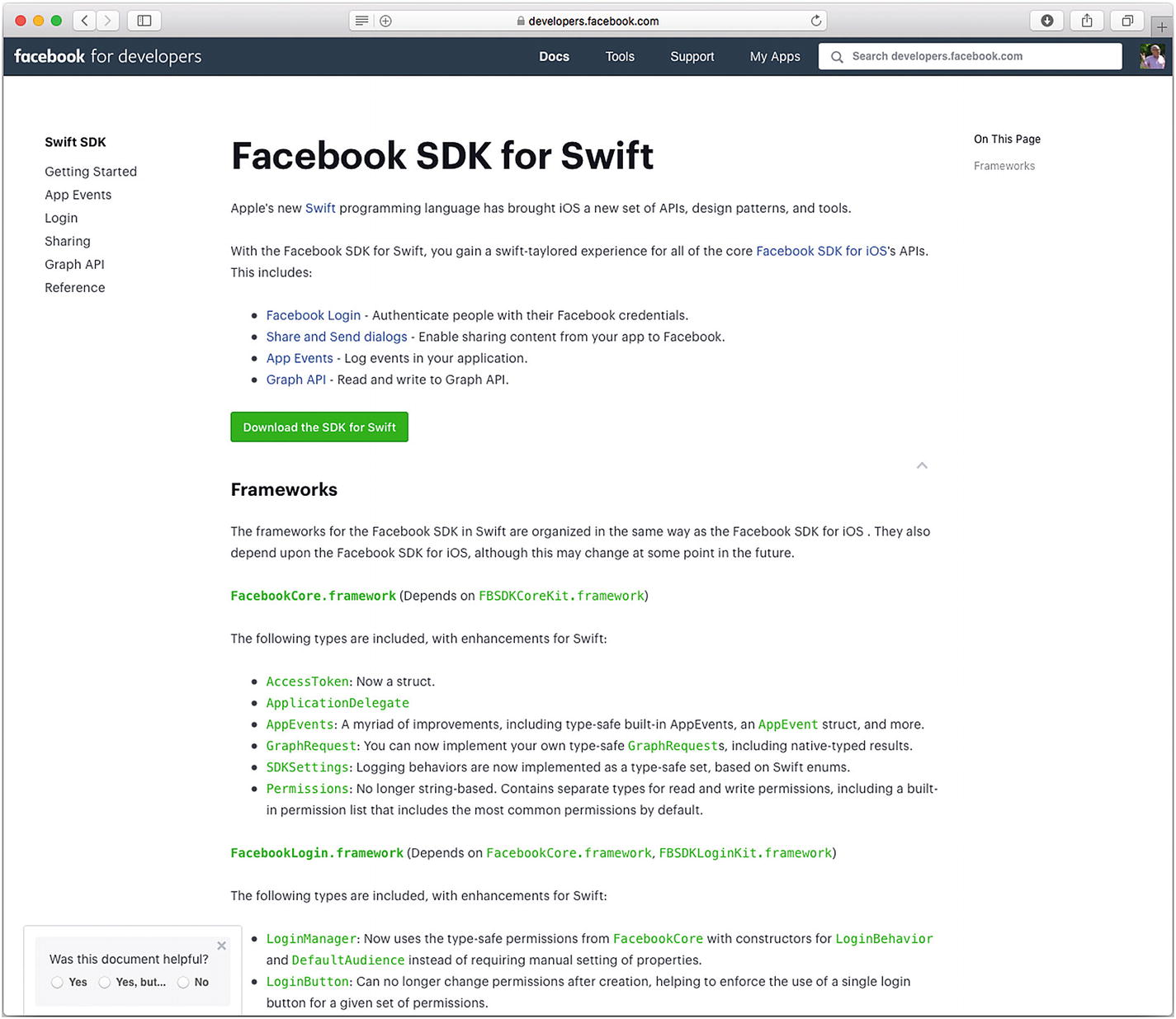
Download the Facebook SDK for Swift
The frameworks shown in Figure 7-6 are the heart of the Facebook SDK for Swift. All frameworks for iOS were originally written in Objective-C. Today, some of the new frameworks are written in Swift, but when you build a Swift-based app, it is not a problem that some (or all) of the frameworks are written in Swift. Thus, when you download the SDK for Swift, you’ll wind up with frameworks that are often written in Objective-C, and that doesn’t matter. When you download the SDK for Swift, as shown in Figure 7-6, some of the frameworks have enhancements specifically for Swift, so keep these files safe and just add them as needed to your app.
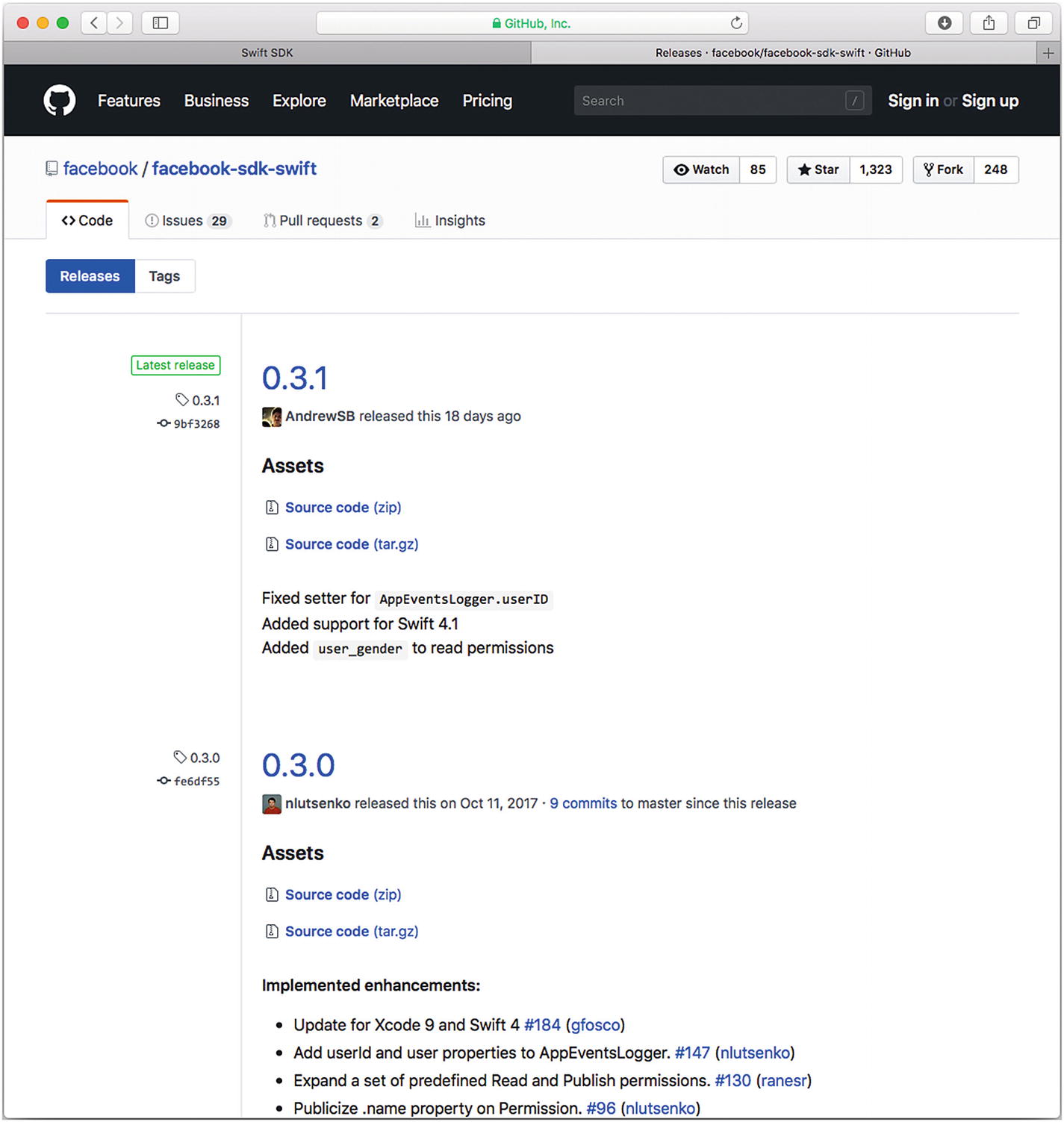
Download the latest Facebook SDK for Swift
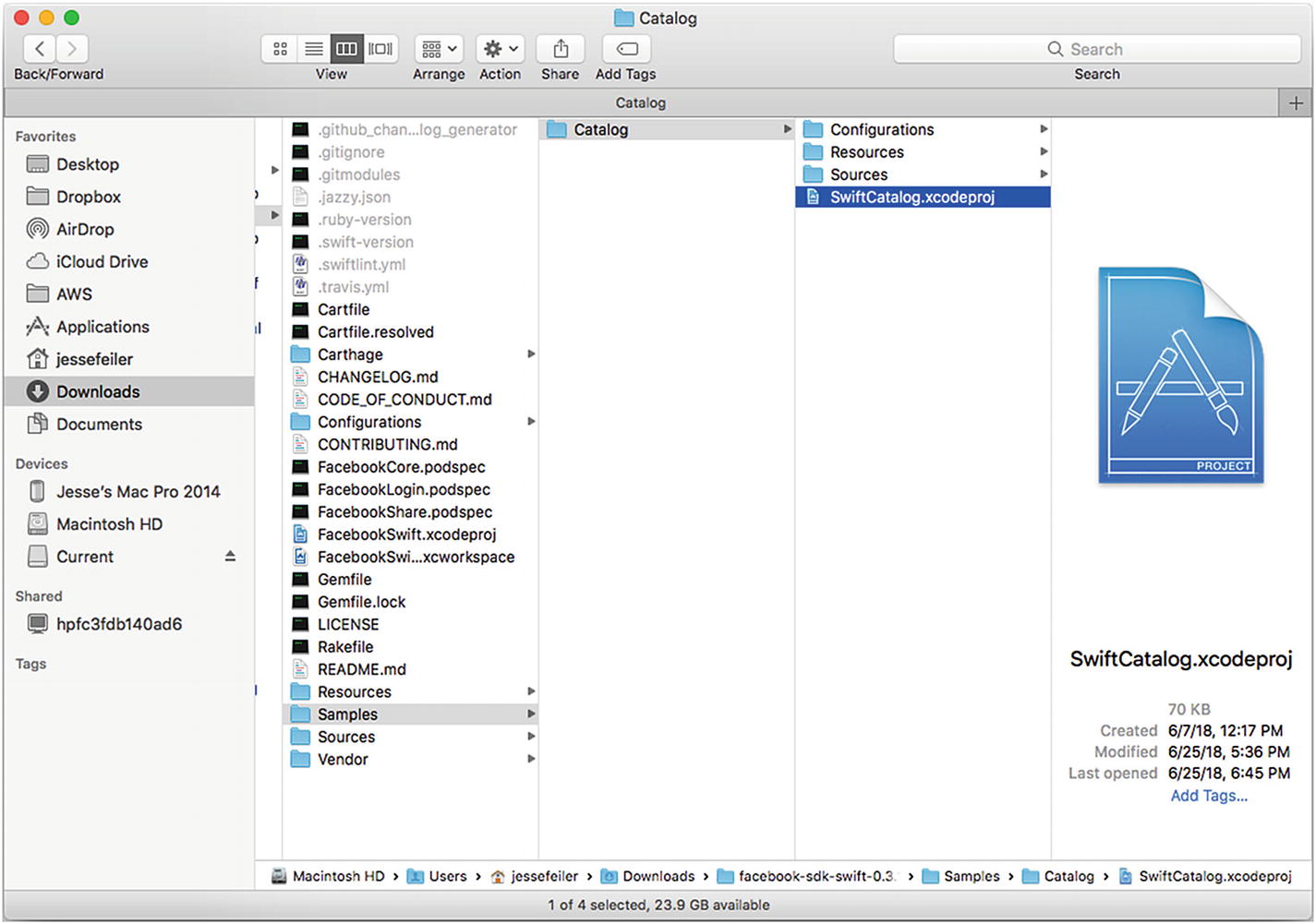
Downloaded Facebook files from GitHub
Adding Frameworks and Functionality to Your Facebook App
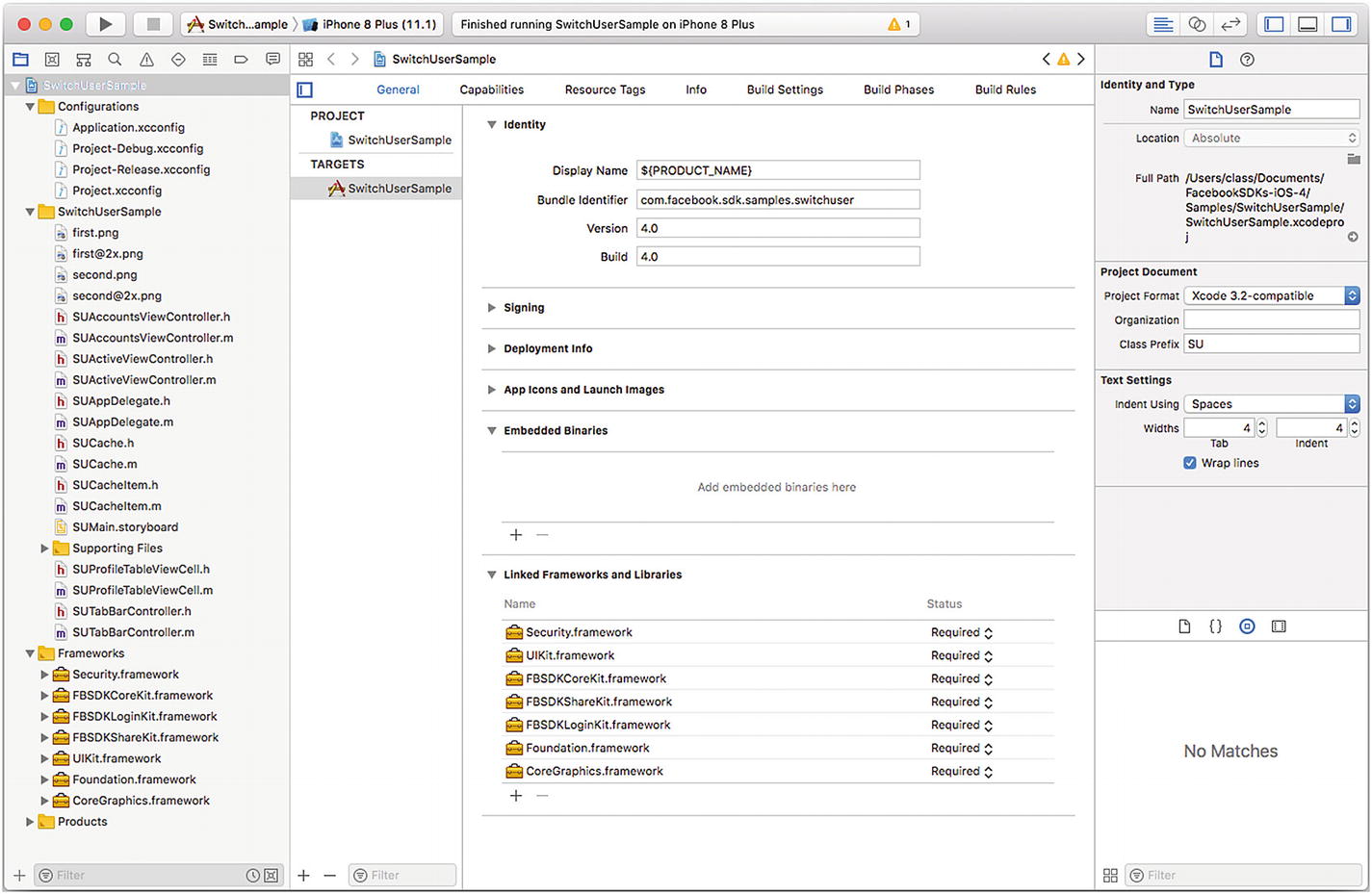
Build a sample to get frameworks
If you drag the needed frameworks into your own app, Xcode will put them in the right place in your project.
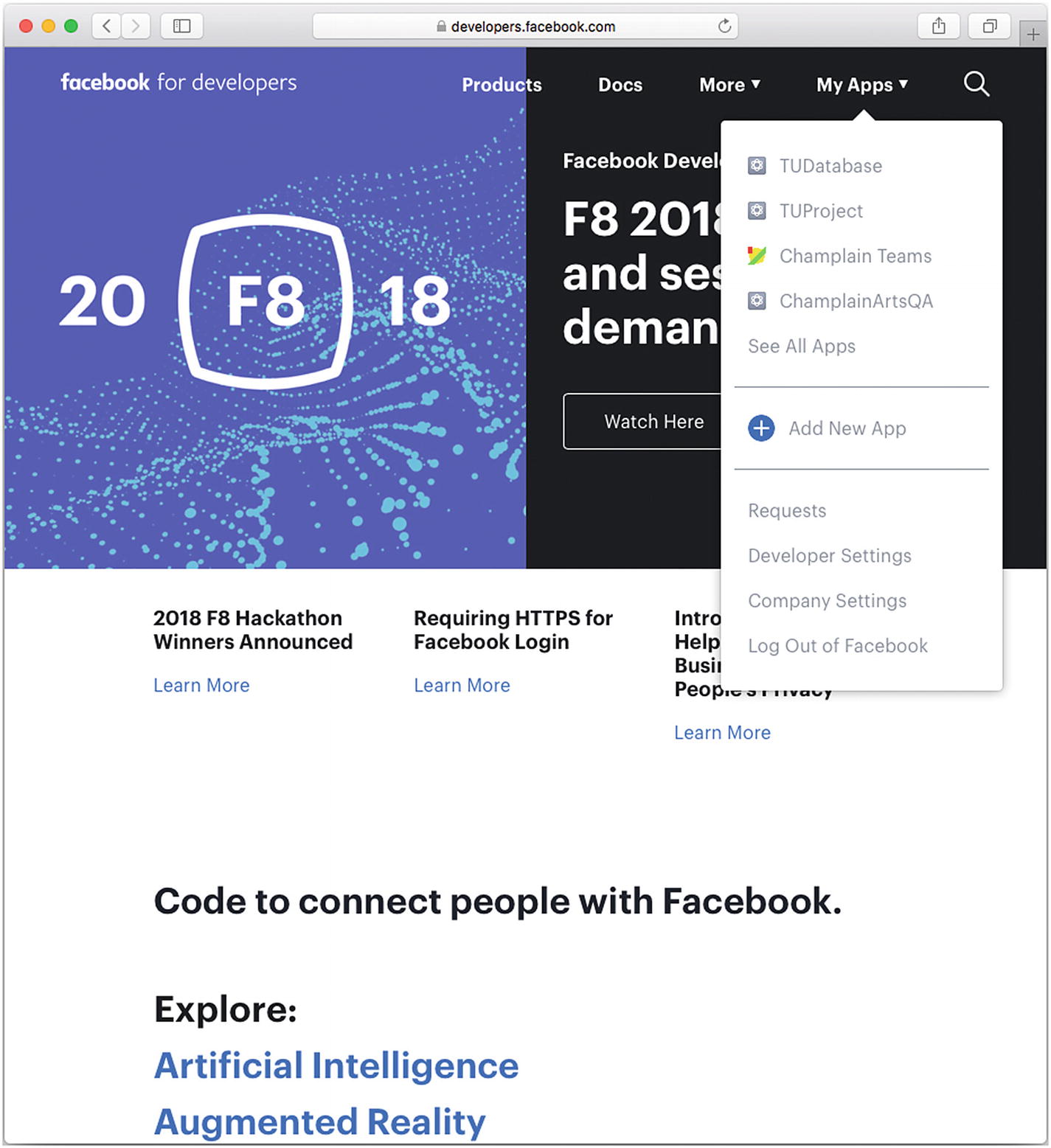
Add and modify your Facebook app frameworks and features
Enhancing Your App
If you have followed along with this chapter, you have produced the app shown previously in Figure 7-3. It runs and displays its storyboard, which happens to be blank. In order to move forward, it makes sense to add something to your storyboard.
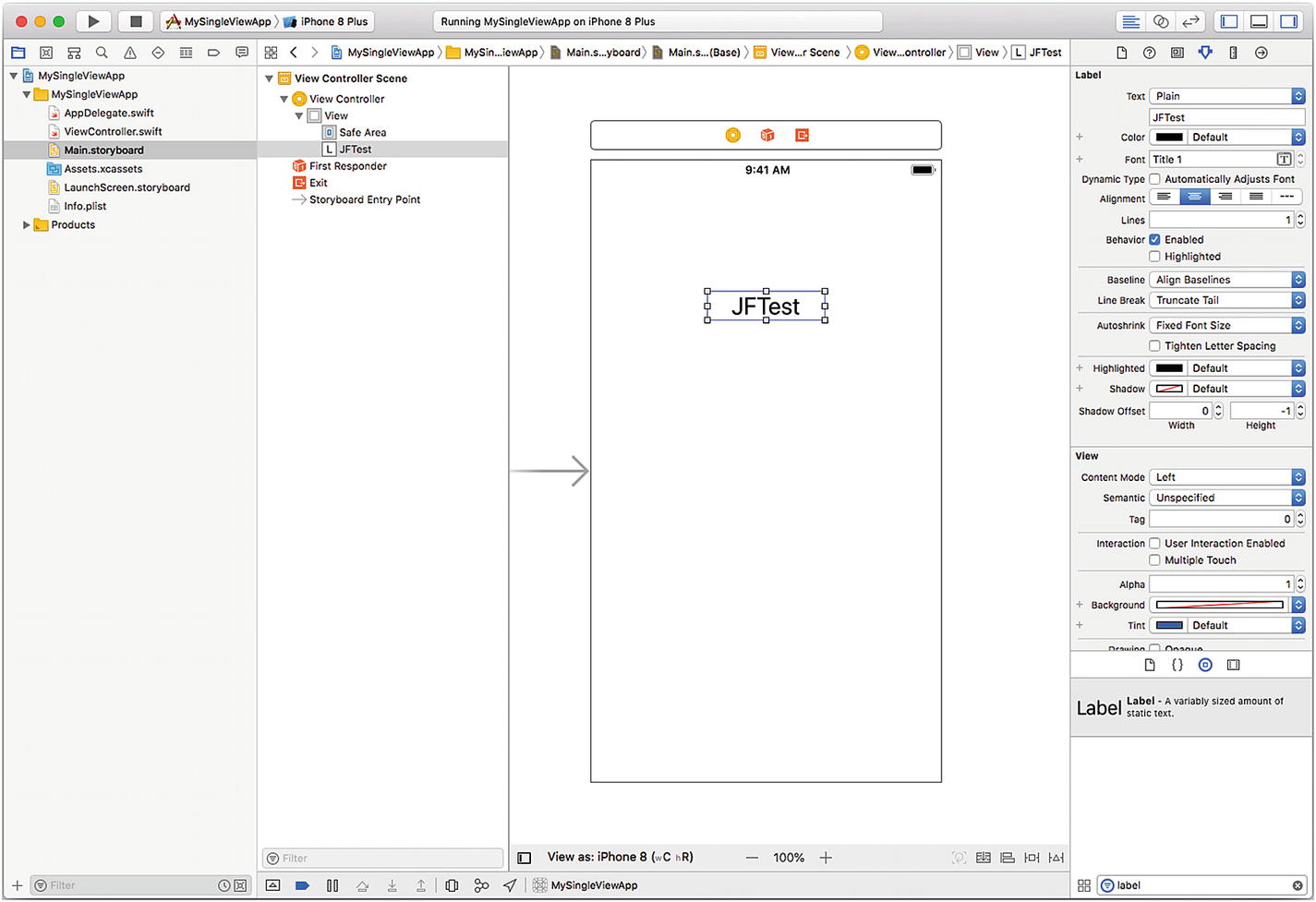
Add a label to your app
Next, add a Facebook login button to your app. If you have followed the sequence shown previously in which you built the SwitchUserSample example, you have the Facebook Login framework in that app and you can drag it into your new app. Alternatively, add Facebook Login from the products shown in Figure 7-11 by modifying one of your apps (shown in My Apps in Figure 7-10).
Once Facebook Login is added to your app, the code to add the button is simple. Add it to the viewDidLoad method of your app. (If it’s built on the SingleViewController template as described in this chapter, the app has one view controller called ViewController.
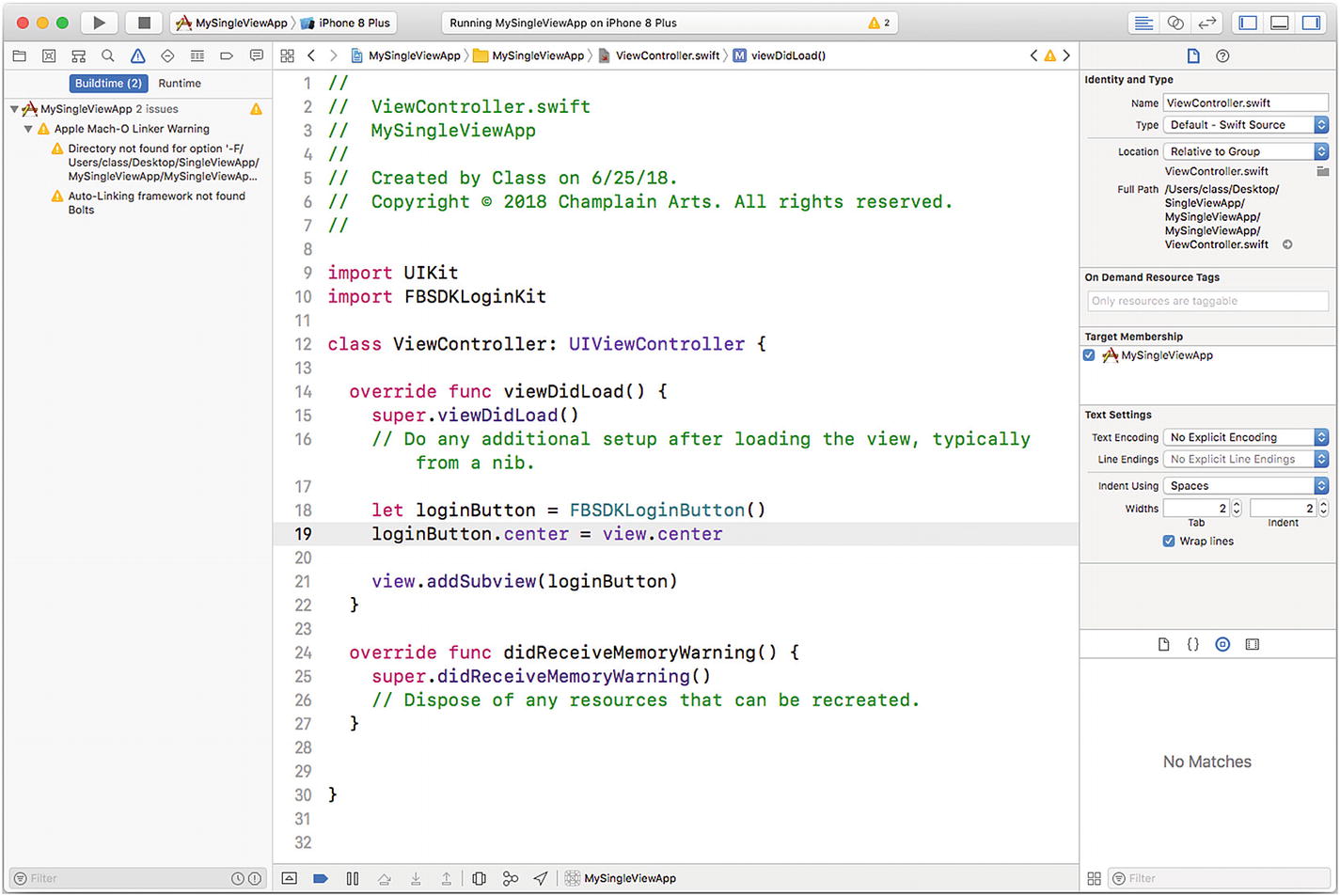
Add the Facebook login button code to viewDidLoad
Note
The basic viewDidLoad method with a stub is already part of the SingleViewApp template.
Add the Facebook Login Button
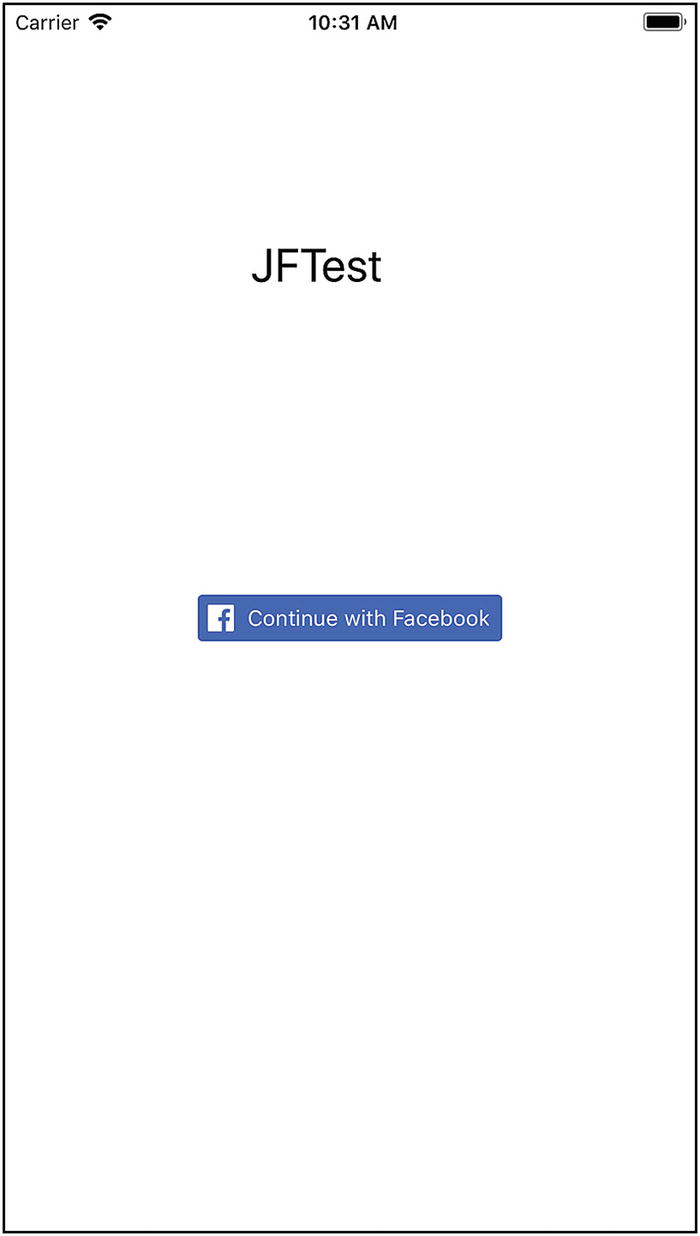
Try your app with the label and login button
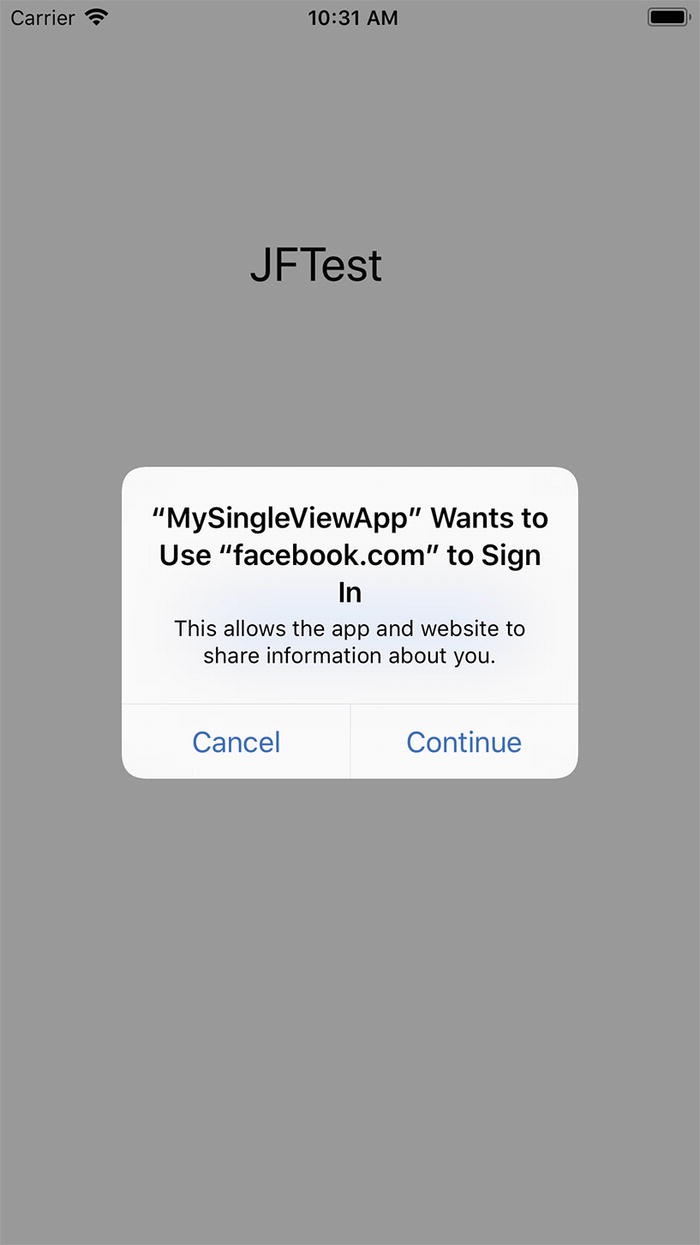
Test Facebook login integration
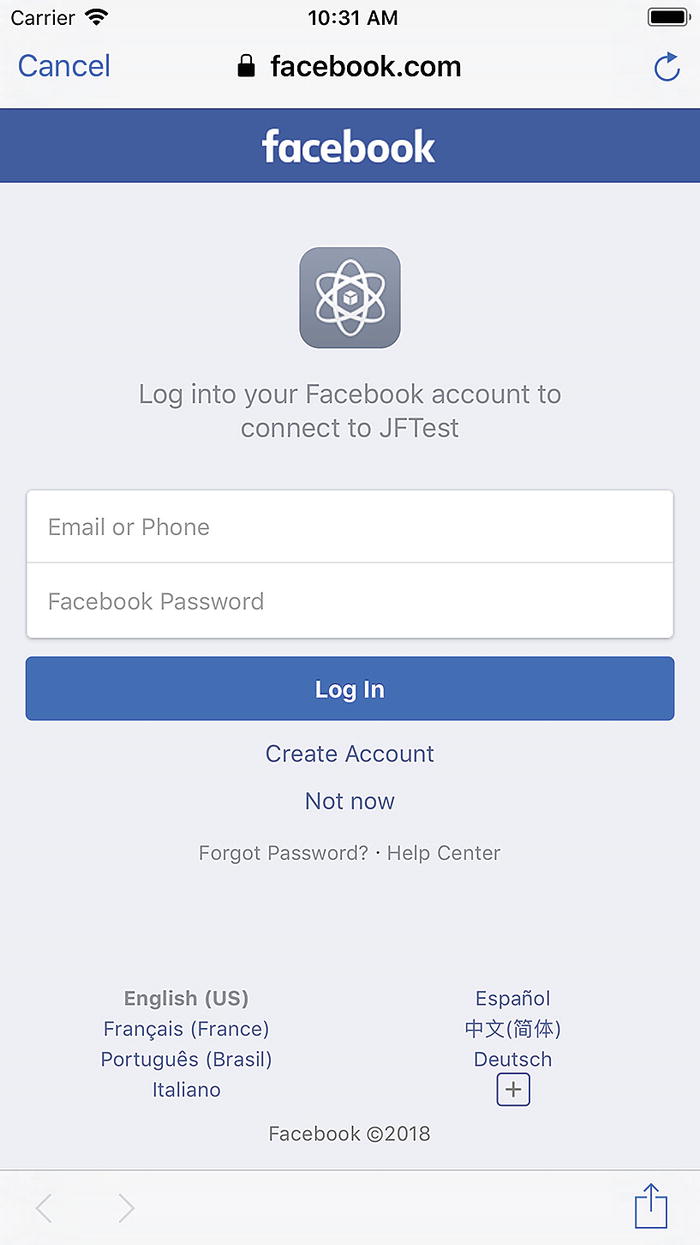
Test the Facebook login button
You can also test with your own Facebook account. In addition, you can search developers.facebook.com to get test accounts you can use so that you don’t create fake accounts or interfere with your own account.
Note
Some developers test logging in with their own account but use the Facebook test accounts for adding information.
Summary
This has been an overview of integrating Facebook and iOS. The details may change from time to time, but the overview remains basically the same. And, in fact, it’s the same for all components of Facebook and other frameworks that you may want to integrate with your app.