In Parts III – V of this book you see how to put third-party components together with Cocoa and its frameworks. These components can be concepts, standards, or open source tools such as JSON, or they can be specific tools, such as the login using Facebook that is described in Part III.
In this part, a more general tool will be introduced: Amazon Web Services. (Coming up in Part V is RxSwift.) You will see an overview of how to create an AWS account for your app to use, install the appropriate downloads from AWS, and provide integration with your app. The focus of AWS integration is data management, but you can use AWS for other purposes as well.
Comparing Components
JSON is a tool for reading and writing structured text in a simple way. It is so common these days that many languages, frameworks, and environments (including Cocoa and Cocoa Touch) support the use of JSON for both reading and writing.
The Facebook Login tool (described in Part III) differs from JSON in that it is designed for a single simple purpose: checking someone’s credentials. Rather than the tight integration that JSON has with Cocoa and Cocoa Touch, Facebook Login has no integration from the Cocoa or Cocoa Touch side: You simply add some methods, classes, or frameworks from Facebook to your app, and then a Facebook-provided class goes off to check the credentials and pass back a yes or no for access. (This is a simplification of the process.)
Amazon Web Services (AWS) is a different type of tool with a different type of integration. It is not explicitly integrated with Cocoa and Cocoa Touch. AWS functionalities are critical to most apps, and they are provided in a number of different ways. They can be provided with code that you write for your app; code found in one of the many frameworks or libraries available on GitHub and elsewhere; or code and frameworks that are part of Cocoa and Cocoa Touch. AWS becomes the data manager for your app, and you interact with it constantly as your app runs (depending, of course, on the specific design of your app).
Note
For the remainder of this chapter, unless a distinction needs to be made, Cocoa is used to refer to both Cocoa and Cocoa Touch.
Using AWS with Cocoa
As noted, there are many ways in which you can integrate AWS with Cocoa and Cocoa Touch. In the simplest way, you use AWS (more specifically, one or more components of AWS) just as you would use the Facebook Login tool or even as you would use an open source tool, framework, or standard like JSON. In those cases, think about an iOS or macOS app that incorporates AWS.
At the other extreme, you can use AWS (more specifically, one, more, or many components) as the heart of your app.
In both of these scenarios, the user interface is envisioned as being built with Cocoa or Cocoa Touch. The question is, where does the implementation of the basic functionality of the app take place—is it in AWS or is it in Cocoa?
Note
It is possible to place some of your app’s functionality on the Cocoa side of things and other parts of the functionality in AWS. Depending on your environment, it may be advisable to consolidate the functionality on one side or the other, but that is a personal observation and not a suggestion.
Sharing data with others
Using data across platforms
Playing to your strengths
Playing to your users’ expectations
Each of these points will be discussed in the following sections.
Sharing Data with Others
When you talk to people about apps, they commonly think and talk about entering data on their phone and letting other people (or themselves at other times) view and modify the data on another device—maybe even on a PC sitting on a desktop half a world away.
That common scenario that we all recognize is complicated to implement. Typically, the data is going to be stored somewhere, and that “somewhere” cannot be solely on the phone from which it originated, because it needs to be visible to people when the phone is out of range or even powered off.
One of the most common ways of storing this persistent data is in one of the cloud-based services , such as AWS, Box, Azure, Dropbox, or FileMaker (version 17 or greater). You can also use the web (particularly with HTML5) to store the data, but it must be somewhere that is reachable.
Using Data Across Platforms
There are many ways to store data that needs to be shared. People who have worked with data of all kinds and on all types of projects commonly agree that changing a data-management strategy is not easy, and it gets more complicated as more and more data becomes involved, as is the case with an old system. Too often, people skip over the “magic” that they expect to happen automatically to the data.
With the exception of a project that does not store any data—and never will—it’s worth thinking about how data will be shared. Here are some of the basic considerations and suggestions for how you might approach the data-management issue. Planning for data-management strategies that will only be implemented in the future is far, far better than leaving it to chance (or “magic”). In other words, you don’t have to do it at the start, but you have to have at least one plan for managing data in the future, even if that plan is to revisit the issue in the future. Just make certain it’s a revisit and not a first visit to the idea of data management.
Note
This section focuses on data, but it is now possible to move some or more of an app’s processing to the cloud. In fact, the distinction between data and processing is hard to define in many cases because one can frequently be converted to the other.
None. No data is stored and never will be.
One user/device. Data is stored only for one user and one device. This is generally a special case for temporary data. It would apply to a calculator app that remembers its last total (just as calculators do). You can use the Cocoa UserDefaults.standard to store relatively small amounts of data on the device.
“Relatively small amounts” has grown over time, but Apple’s documentation indicates that it depends on the device. This makes sense because devices get more and more storage over time. Reports of 4 GB being stored are found in web discussions.
The data may be backed up with normal backups of the device (if they are turned on by the user).
One user/multiple iOS devices. This scenario is easiest to implement with the user’s Apple ID and iCloud. There is a limit to the amount of space available, which depends on what iCloud data plan the user subscribes to and how much other data is being stored.
The iCloud data is backed up automatically as part of the iCloud service. Nevertheless, if one of the devices is not reachable, its data may not be uploaded to iCloud in a timely manner, and, unless the app properly handles iCloud conflicts (as with user resolution of conflicts), the data may not be what you and the user think it should be.
Multiple users/multiple iOS devices. CloudKit is a good tool for handling this situation. Because it relies on iCloud, backups are done automatically.
Multiple users/multiple devices (or one user with only a non-iOS device). This case is usually best served with an on-demand cloud service such as AWS, Box, Dropbox, Google Drive, OneDrive, or similar. Backups are part of the service.
Not all apps need on-demand storage, but that is a feature available from most of the services mentioned here.
Playing to Your Strengths
With the availability of cloud-based computing as well as data, you can choose where to put each one. In the previous section, there were some suggestions with regard to data, but with any project it makes sense to make your choices not just on technical grounds, but also with consideration given to the skills and strengths of your developers, whether it is a team of 50 or just yourself.
Playing to Your Users’ Expectations
When it comes to users’ expectations of shared data, the word “overused” is not far from the mark for unrealistic expectations. But there’s another expectation that is just as dangerous: an expectation that shared data will be structured and shared using the latest and greatest technology from the age of mainframe computers and sometimes even from the age of punched cards. Data structures have a very long life span. In part, that is the result of what is sometimes called “the drag of the installed base”—the need to keep things running even though times and capabilities have changed.
One benefit of using shared-data tools such as AWS is that you and your users may be confronted with technologies and interfaces that may be new to you all.
Exploring AWS
After considering the issues of shared computing and shared data, it is time for a high-level overview of AWS. This section will provide that overview. In the next chapter, “Managing AWS in Cocoa,” you’ll drill down a bit more into AWS and how to integrate it with your apps. Remember that AWS is a very rich set of tools and that there is much more to find out than can fit in these chapters. The goal here is to give you an idea of what is available so you can at least make the decision of whether or not to delve further into AWS for your project.
Note
AWS is a web-based technology, and that applies to its website as well as its technology. The screenshots you will see and the steps you will take in this chapter may differ; however, the general process will probably be the same. It’s fair to assume that some of the AWS components illustrated in this chapter may be enhanced, new ones may be added, and some may be deprecated.
Getting Started with AWS
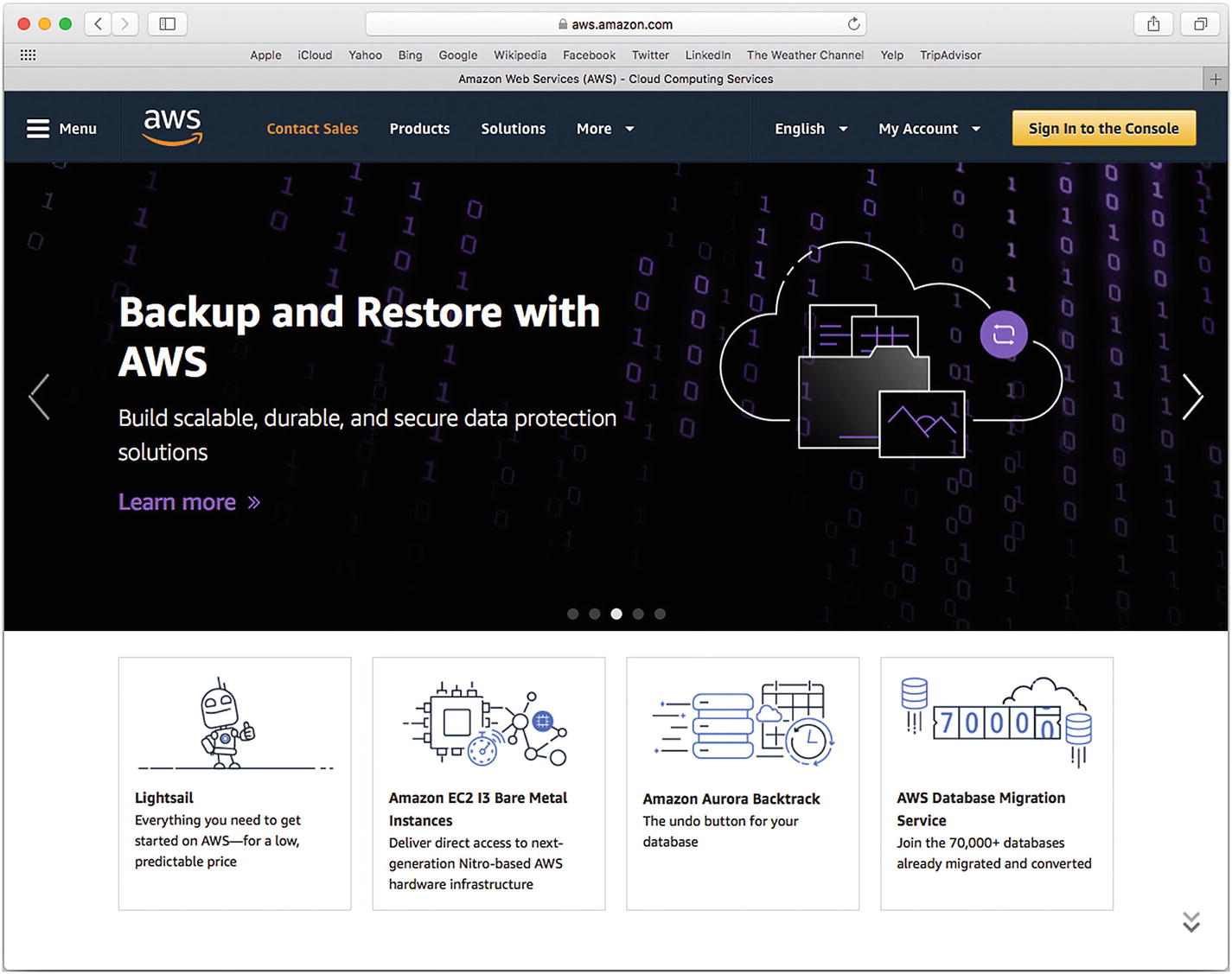
Begin to work with AWS
As a developer, your interactions with AWS are done through the console using the button at the top right. You’ll find other links to the console throughout the AWS site. You are able to browse the site without logging in to the console, but to actually do anything, you will need an account. You’ll see how to set up your account in Chapter 10, “Managing AWS Logins.”
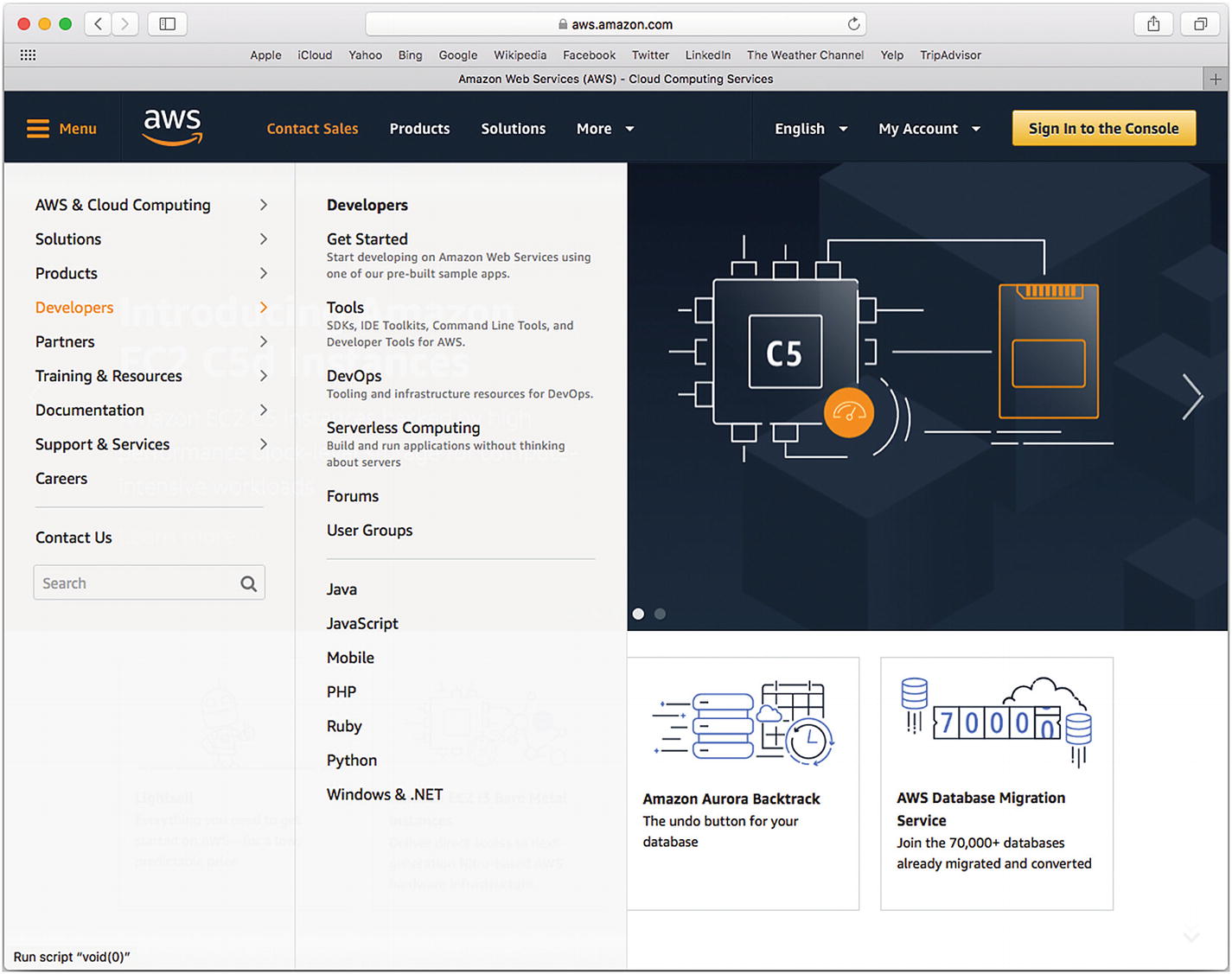
Browse developer resources on AWS
Figure 8-2 gives you a high-level overview of resources available for developers. The Developers menu remains pretty constant even as AWS changes.
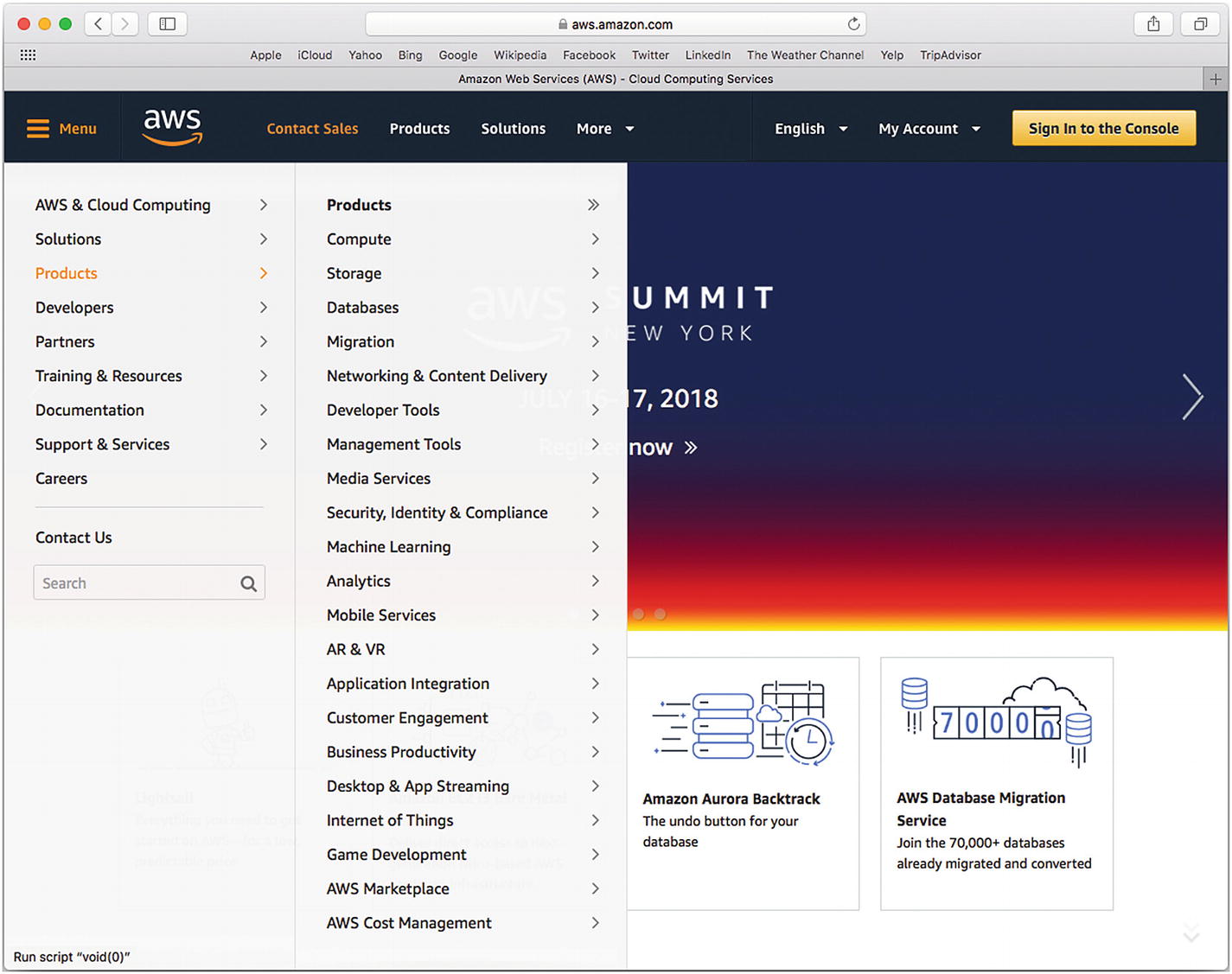
AWS products
Comparing Cocoa and AWS Products for Data Management
You can build a complete app from these AWS products (that’s the idea of AWS of course). The only thing that’s missing is the user interface. You can provide that with web-based tools like HTML5. However, for the most powerful and flexible interface, Cocoa and Cocoa Touch are the tools we prefer.
If you look at the list of products, you’ll see that they are basic building blocks for the back end of apps. One of the most common forms of integration between AWS and Cocoa is data management, which is the topic explored in this part of the book. The tools available for data management in Cocoa are focused on individuals, so shared data management needs to be implemented (at least at this point) with tools found outside of Cocoa.
SQLite is built into Cocoa , but it is a personal data-management library; it doesn’t manage sharing. iCloud is the Apple technology that handles data sharing, but that is primarily focused on sharing within one AppleID. (CloudKit does provide some broader data sharing.)
Core Data is a powerful data-persistence tool that is part of Cocoa. It is not a data manager; rather, it was designed as a front end to any data-management tool that conforms to the Core Data structure. Over the years, a variety of data-management tools have been integrated with Core Data in various forms of Apple products.
Summary
This chapter has introduced a high-level view of Amazon Web Services (AWS) and how its tools can work with Cocoa. AWS can be used to build an entire app, but commonly AWS provides a back end and Cocoa provides the front-end interface and functionality.
In the next chapter, you’ll see how to log in and begin to integrate AWS with an app.