In this chapter, you will see the basics of JSON syntax. You can use it with many modern languages, and Swift is no exception. In fact, Swift’s integration with JSON is strong, powerful, and easy to use. If you add in Swift Playgrounds which is available on iPad and in Xcode on your Mac, you get a powerful cross-platform data exchange format that also is easy to test with a playground (so that you don’t have to write an app—even a stripped-down app—to explore the data, syntax, and code).
In this chapter, you will see how to explore JSON with Swift Playgrounds as well as how to explore the iOS/Swift interfaces that are available.
Note
These features are shown using Xcode 9 and Swift 4. These include significant changes from previous versions of Xcode and Swift.
Getting Started with a JSON Swift Playground
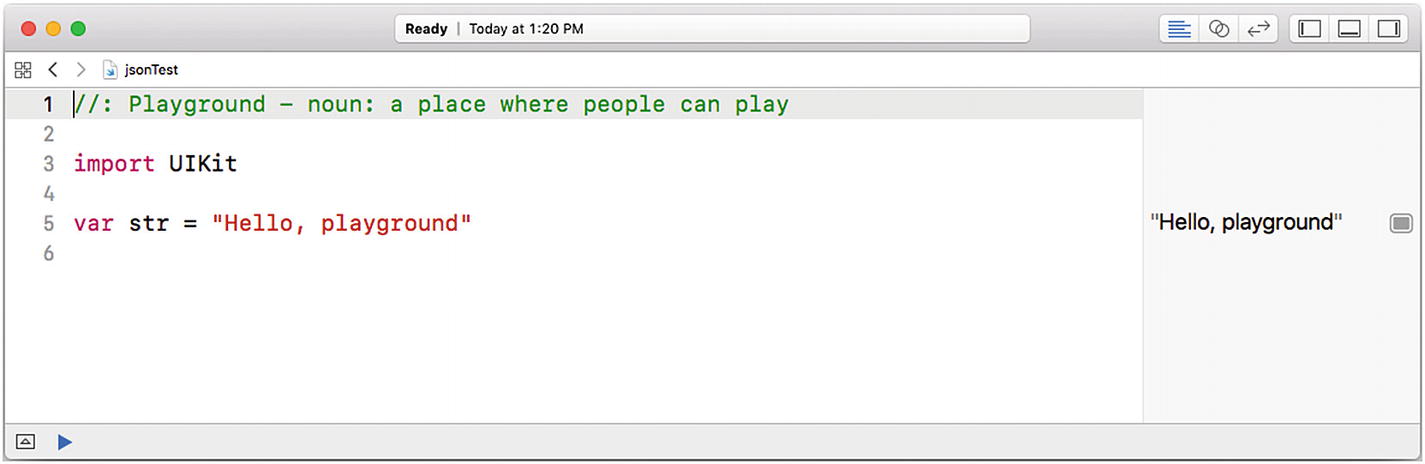
Create a Swift playground
Note
The examples in this chapter are shown using Playgrounds in Xcode on macOS. Xcode is the tool you use for writing code, and a project such as this one may be easier to work with on macOS than on iOS, but you can use either one.
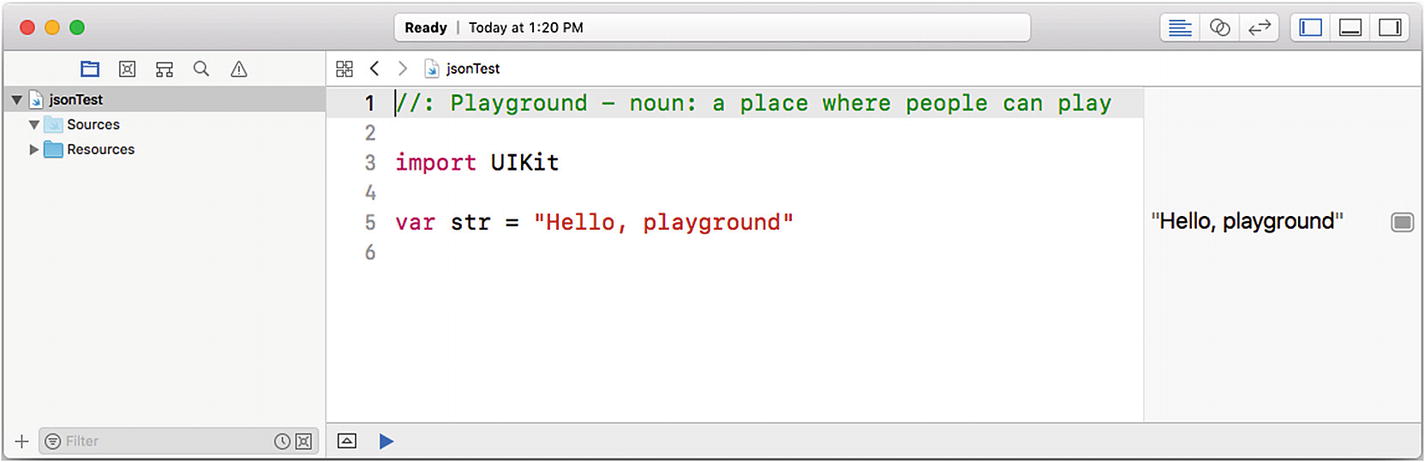
Open the project navigator at the left side of the Xcode window
Note
A Swift Playgrounds project consists of a single file in the Finder, but that file is a package containing the files you see in the project navigator. You can open the package using Control-click in the Finder or by using the right button while you click on the package. Working within Swift Playgrounds and the Xcode project navigator is simpler and more direct.
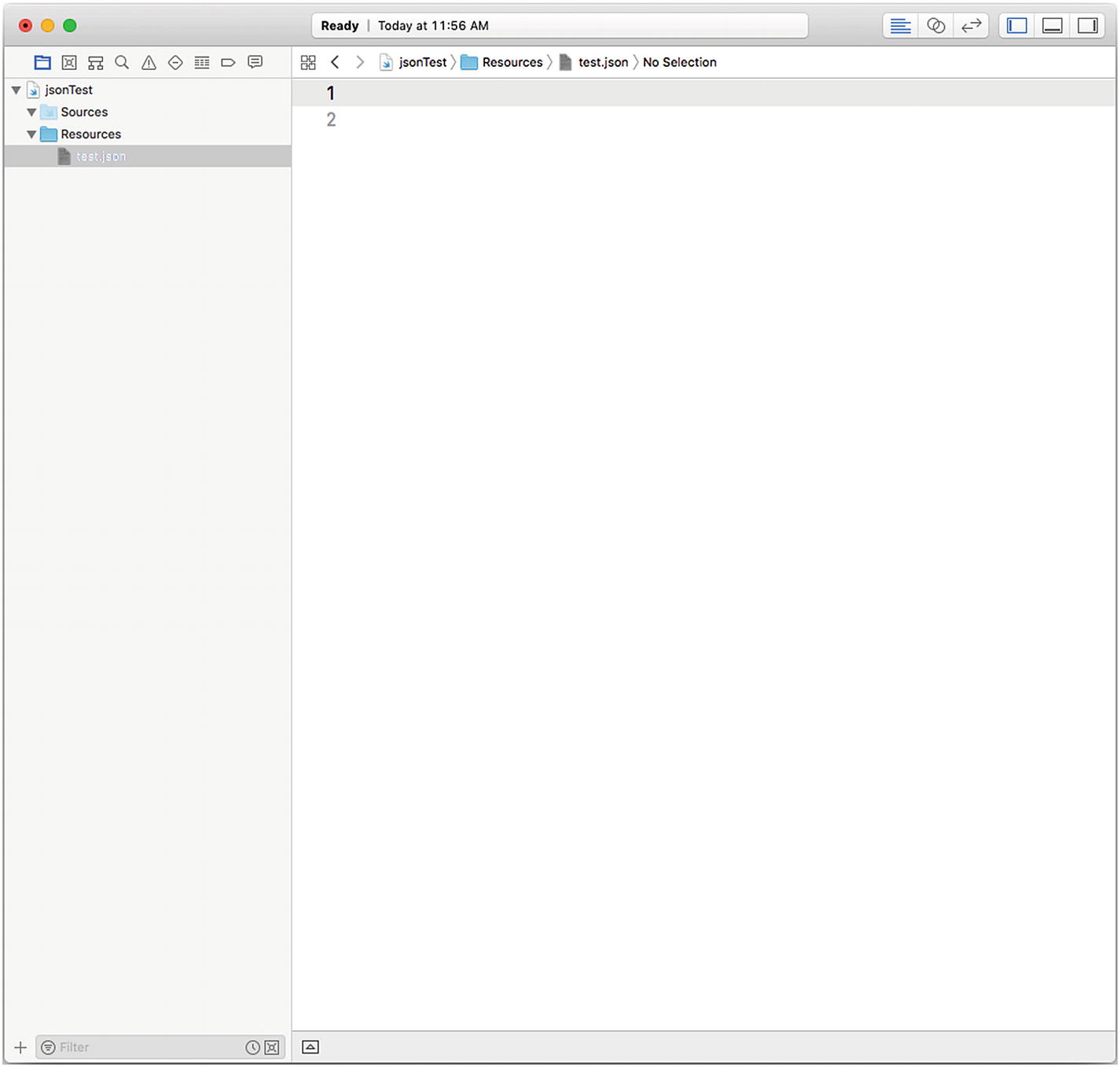
Create a new file
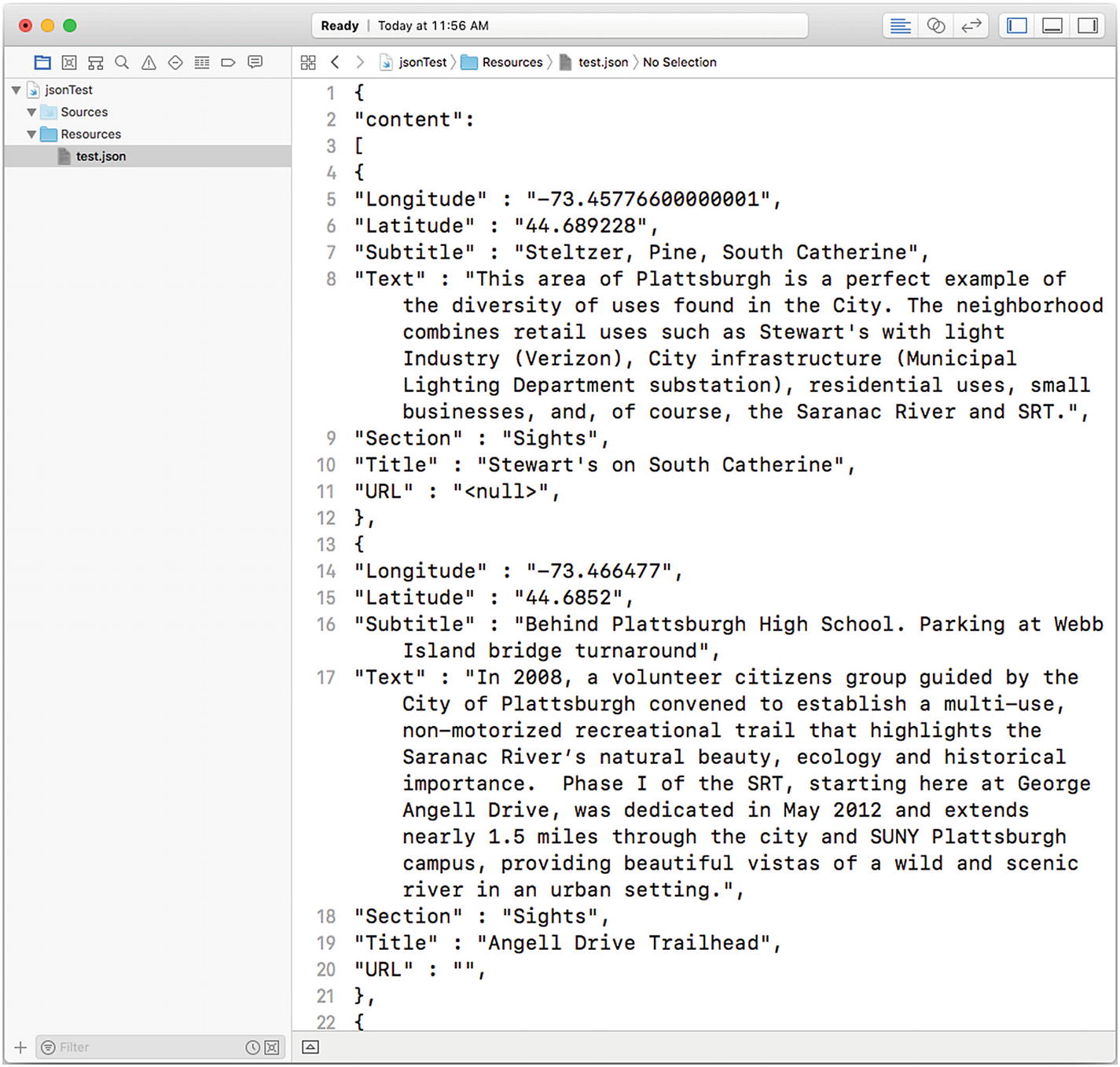
Type or paste code into your file
Change the file name and extension name for your own file.
You can customize the variable name for the content (jsonCode) and the text for the fatalError string . Otherwise, you can use the code as is. (You can also change fatalError to another method of catching an error if you want to.)

Enter the code to read the file
Check to make certain that you see the code properly and don’t have an error. (This is the step that may catch you up until you’re used to creating files inside a playground.)

Review the JSON code as it runs
Using the JSON Integration Tools in Swift
What you have seen so far is how to create a file inside a playground and how to read its contents. The reading process is the same whether the file is in a playground or somewhere else—perhaps even being sent over a network.
What is more common is reading from a file and handling its contents not as a string but as JSON data. That is what this section will cover.
Integrating a Swift Array
Begin with some JSON code that you can create in an editor like BBEdit or in Xcode (or even in TextEdit).
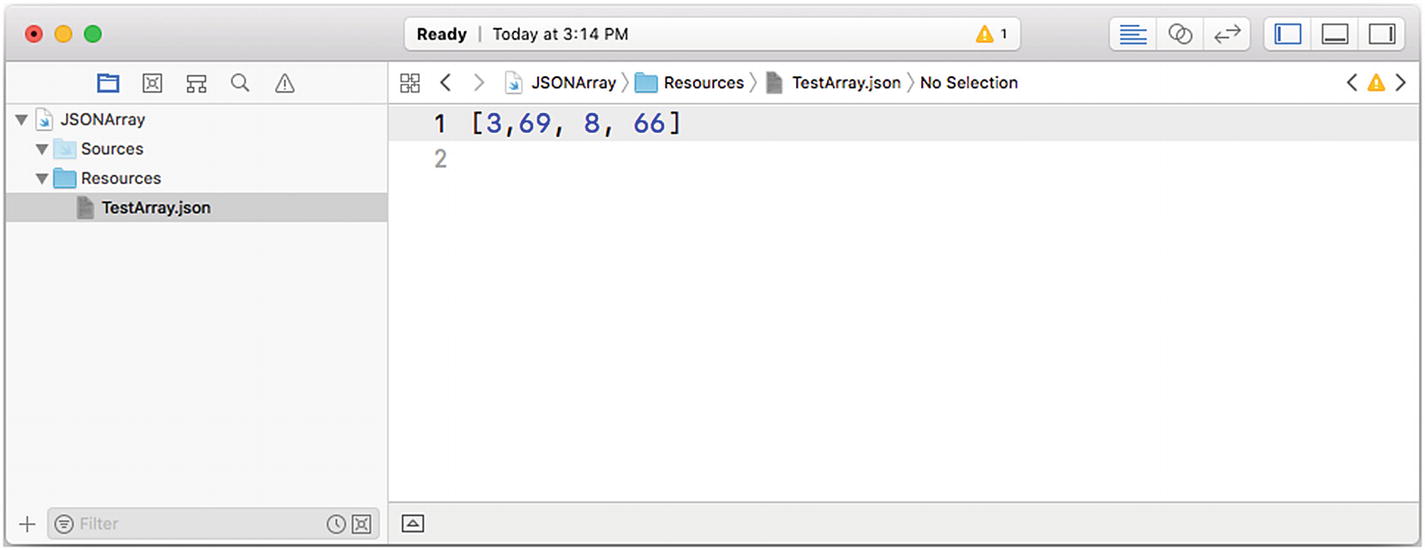
Create a JSON array in a file inside your playground bundle
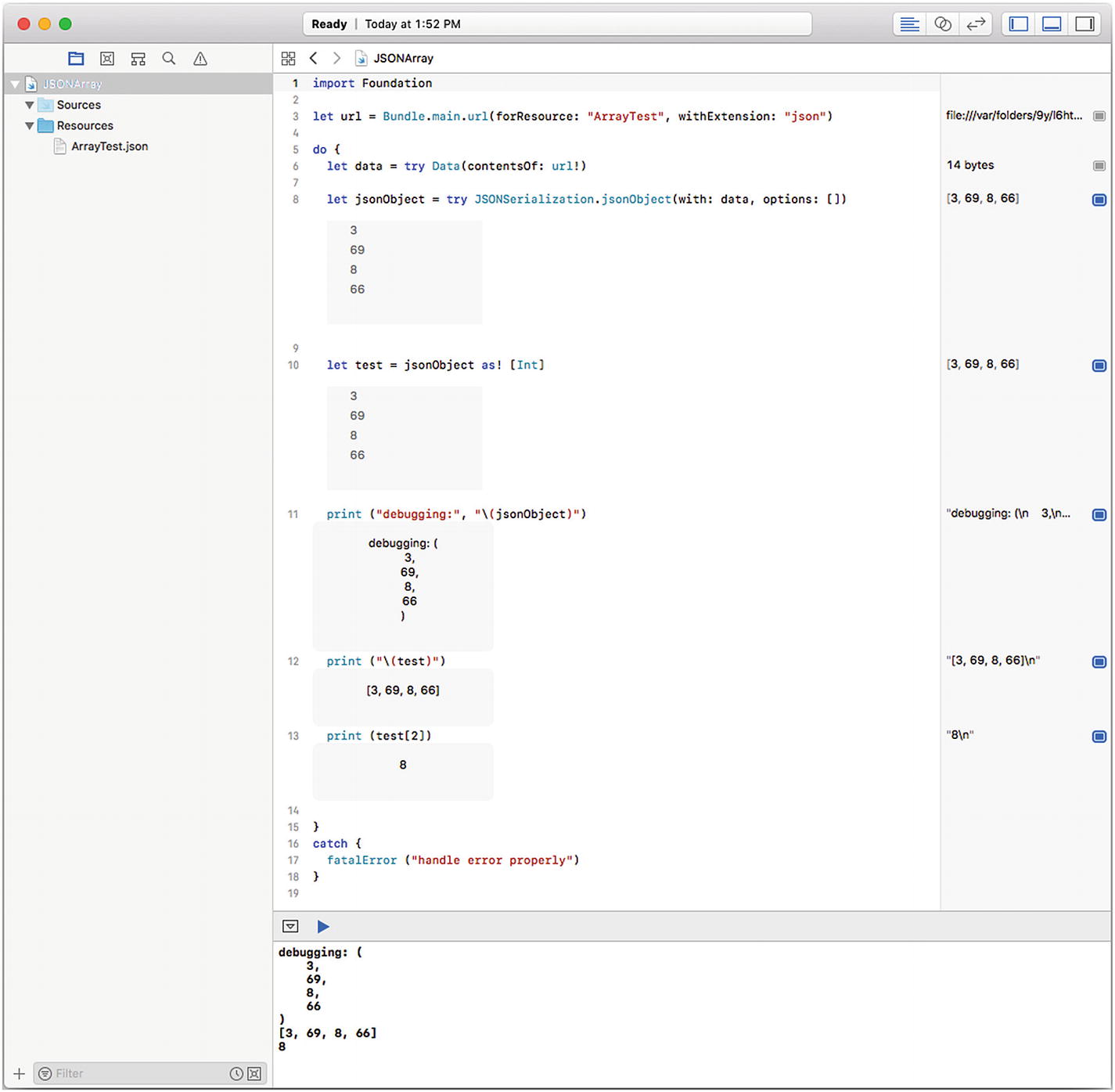
Catch a failure
You can see this in line 10 of Figure 4-9. (In practice, you would use as? to catch an error in the conversion.)
Line 13 creates the test variable with the typed array, as noted previously in this section. The reason that line 13 is so important is that the JSON code that was probably specified as a typed-in string now is converted to a real object, and you can use the subscript [2] to access the data—just like any other Swift data.
Integrating a Swift Dictionary
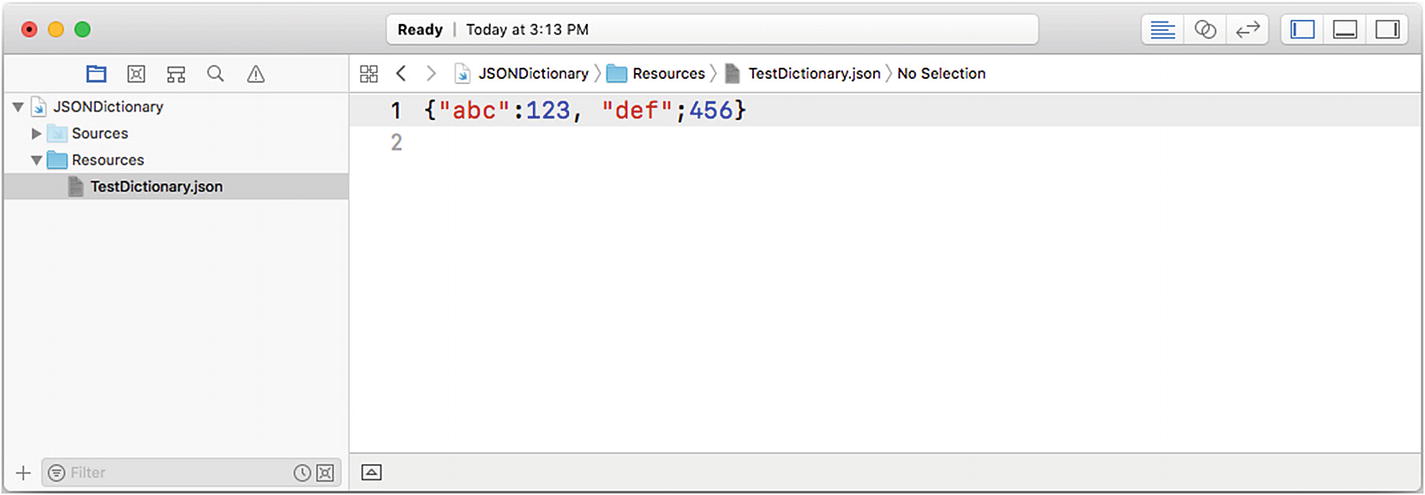
Use a dictionary with Swift and JSON
Note that the brackets are curly brackets, which is the style that JSON uses for its objects. Square brackets (as shown in Figure 4-8) are for a JSON array.
Note
This is the area you need to pay attention to. The rules of JSON and Swift are similar but not identical. For example, a Swift array has elements of a common type, but that is not necessarily the case in JSON. That is why you must always catch failures that may occur when using JSONSerialization.json.
Summary
The ability to move data back and forth using a common syntax like JSON is important both within a single app and between multiple apps. This chapter has showed you the basics.
Note that beginning with Xcode 9, the Codable protocol is provided to further enhance Swift’s JSON capabilities.
You have not yet seen the basic types of building blocks that let you share code and data across apps and platforms. In the next chapter, you will begin to look at very specific uses of these building blocks by examining Facebook logins that you can use in iOS (and other) apps.