Here is an example that enhances the model's admin for better presentation and functionality. You can look at the difference between the two following screenshots to see how a few lines of code can make a lot of difference:

After the admin customizations explained in this section are made, the same information will be presented in a much more accessible manner, as shown in the following screenshot:
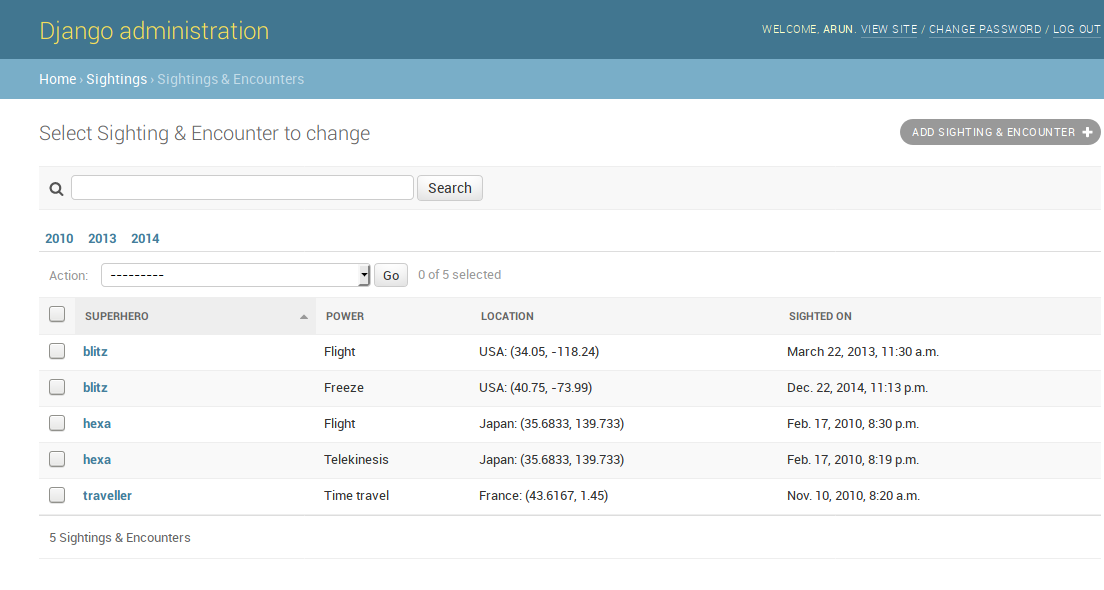
The admin app is smart enough to figure out a lot of things from your model automatically. However, sometimes the inferred information can be improved. This usually involves adding an attribute or a method to the model itself (rather than to the ModelAdmin class).
Here is the enhanced Sightings model:
# models.py class Sighting(models.Model): superhero = models.ForeignKey( settings.AUTH_USER_MODEL, on_delete=models.CASCADE) power = models.CharField(max_length=100) location = models.ForeignKey(Location, on_delete=models.CASCADE) sighted_on = models.DateTimeField() def __str__(self): return "{}'s power {} sighted at: {} on {}".format( self.superhero, self.power, self.location.country, self.sighted_on) def get_absolute_url(self): from django.urls import reverse return reverse('sighting_details', kwargs={'pk': self.id}) class Meta: unique_together = ("superhero", "power") ordering = ["-sighted_on"] verbose_name = "Sighting & Encounter" verbose_name_plural = "Sightings & Encounters"
Let's take a look at how admin uses all these nonfield attributes:
- __str__(): Without this, the list of superhero entries would look extremely boring. All entries would be shown alike, with the format of < Sighting: Sighting object>. Try to display the object's unique information in its str representation (or Unicode representation, in the case of Python 2.x code), such as its name or version. Anything that helps the admin to recognize the object unambiguously would help.
- get_absolute_url(): This method is handy if you like to switch between the admin site and the object's corresponding detail view on your (nonadmin) website. If this method is defined, then a button labeled View on site will appear in the top right-hand corner of the object's Edit page within the admin.
- ordering: Without this Meta option, your entries can appear in any order as returned from the database. As you can imagine, this is no fun for the admins if you have a large number of objects. The admins usually prefer to see fresh entries first, so sorting by date in the reverse chronological order (hence the minus sign) is common.
- verbose_name: If you omit this attribute, your model's name would be converted from CamelCase into camel case. In this case, it used frivolously to change "Sighting" to "Sighting & Encounter". But sometimes, the automatically generated verbose_name looks awkward, and you can specify how you would like the user-readable name to appear in the admin interface here.
- verbose_name_plural: Again, omitting this option can leave you with funny results. Since Django simply prepends an s to the word, the generated plural would be shown as "Sighting & Encounters" (on the admin front page, no less), so it is better to define it correctly here.
It is recommended that you define the previous Meta attributes and methods not just for the admin interface, but for better representation in the shell, log files, and so on.
However, you can use many more features of the admin by creating a custom ModelAdmin class. In this case, we customize it as follows:
# admin.py class SightingAdmin(admin.ModelAdmin): list_display = ('superhero', 'power', 'location', 'sighted_on') date_hierarchy = 'sighted_on' search_fields = ['superhero'] ordering = ['superhero'] admin.site.register(models.Sighting, SightingAdmin)
Let's take a look at these options more closely:
- list_display: This option shows the model instances in a tabular form. Instead of using the model's __str__ representation, it shows each field mentioned as a separate sortable column. This is ideal if you like to sort by more than one attribute of your model.
- date_hierarchy: Specifying any date-time field of the model as a date hierarchy will present a date drill down (note the clickable years below the Search box).
- search_fields: This option shows a Search box above the list. Any search term entered would be searched against the mentioned fields. Hence, only text fields such as CharField or TextField can be mentioned here.
- ordering: This option takes precedence over your model's default ordering. It is useful if you prefer a different ordering in your admin screen, which is the preference we have adopted here.
We have only mentioned a subset of the most commonly used admin options. Certain kinds of sites use the admin interface heavily. In such cases, it is highly recommended that you go through and understand the admin part of the Django documentation.