Thanks to the extension functions of Kotlin, you can turn any Iterable instance, such as list, to Observable without much effort. We have already used this method in Chapter 1, Kotlin – Data Types, Objects, and Classes, but again take a look at this:
fun main(args: Array<String>) { val observer: Observer<String> = object : Observer<String> { override fun onComplete() { println("Completed") } override fun onNext(item: String) { println("Received-> $item") } override fun onError(e: Throwable) { println("Error Occured => ${e.message}") } override fun onSubscribe(d: Disposable) { println("Subscription") } }//Create Observer val list:List<String> = listOf("Str 1","Str 2","Str 3","Str 4") val observable: Observable<String> = list.toObservable() observable.subscribe(observer) }
The output is as follows:
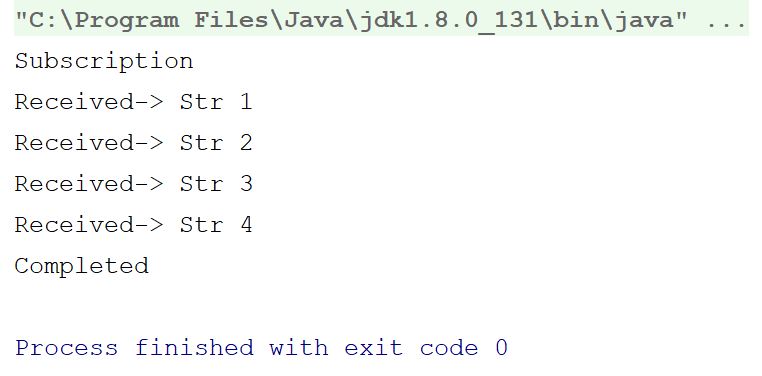
So, aren't you curious to look into the toObservable method? Let's do it. You can find this method inside the observable.kt file provided with the RxKotlin package:
fun <T : Any> Iterator<T>.toObservable(): Observable<T> = toIterable().toObservable() fun <T : Any> Iterable<T>.toObservable(): Observable<T> = Observable.fromIterable(this) fun <T : Any> Sequence<T>.toObservable(): Observable<T> = asIterable().toObservable() fun <T : Any> Iterable<Observable<out T>>.merge(): Observable<T> = Observable.merge(this.toObservable()) fun <T : Any> Iterable<Observable<out T>>.mergeDelayError(): Observable<T> = Observable.mergeDelayError(this.toObservable())
So, it uses the Observable.from method internally, thanks again to the extension functions of Kotlin.