We’ve learned the basics of the most important windows in the Unity engine, and we know how to create simple objects and view their components through the Inspector. Now let’s get familiar with moving, rotating, and sizing GameObjects in our scene.
Transform Tools
The section of the Unity editor just beneath the title bar and the title bar buttons (like File, Edit, Assets, etc.) is called the toolbar . It’s a bar stretching across the width of the screen, with a handful of different buttons on it. This includes the Layout dropdown menu we learned about earlier, which is the rightmost button in the toolbar.

Buttons corresponding to the transform tools. The second button is currently selected, giving it a darker background than the rest
These are the transform tools. You just learned that the Transform component is position, rotation, and size, so you can probably guess that the transform tools are primarily used to move, rotate, and size GameObjects in the scene.
There are six buttons for six different kinds of tools. You may also see a seventh button on the right side that deals with custom editor tools, which we don’t need to concern ourselves with right now anyway. If you don’t see it, don’t worry about that.
Each of these buttons can be clicked to switch to a different tool. Only one tool is ever active at a time, and they all serve different purposes.
From left to right, you can use the hotkeys Q, W, E, R, T, and Y to toggle between these tools, which is often faster than clicking.
The first tool, with the hotkey Q, is the hand tool, which lets you left-click and drag on the screen in the Scene view to drag your scene camera around. It doesn’t edit the scene. It just helps you navigate it.
The other tools will allow you to edit the GameObjects you are selecting. In the Scene window, the transform tool you have selected will provide little “gizmos” on or around your selected GameObjects. These gizmos are simple tools that we click and drag to use the transform tool to interact with the GameObject. You will notice, if you select a GameObject and toggle between these tools, that the gizmo drawn around the object changes as the selected tool changes.
W is the position tool. While active, it shows arrow gizmos on your selected GameObject. You can drag the object in specific directions by clicking and dragging the arrows. You can also drag it along two directions at once by clicking the square shapes between arrows. Holding the Ctrl key while dragging will only move in increments of 1 unit at a time.
E is the rotation tool. It shows circle gizmos on the selected GameObject. Clicking and dragging the circles will spin the object, and each circle turns it along different directions. You can also click between the circles to turn the object in multiple directions at once.
R is the scale tool. It shows gizmos like the arrows, but with cube-shaped ends. Click and drag these boxy arrows to change an object’s width (red), length (blue), or height (green). Click the cube in the center of the gizmo and drag to scale the entire object at once – that is, raising or lowering the width, length, and height evenly at once.
T is the rect tool (“rect” being short for rectangle). It is most applicable to 2D projects but can have its uses in 3D as well. The gizmo shows a rectangle around the selected object, with circles at the corners. The edges or corners can be clicked and dragged to expand or shrink the object as a rectangle, affecting both the position and scale at once. This can be useful to make an object larger or smaller on one side only, since the scale tool will affect the scale on both sides.
There’s also a circle at the center of the gizmo which can be clicked and dragged to reposition the object along the two axes that the rect is aligned with. You’ll notice that the rectangle gizmo operates on two axes at any time. Attempting to move your camera over to the side of the rectangle will cause it to flip around and face the camera again.
The Y tool combines the W, E, and R tools, showing the arrows for moving, the circles for rotating, and the cube at the center for scaling, all at once.
Positions and Axes
So how does positioning work in 3D space? It might take a little getting used to, but a position in 3D space is defined by three number values, referred to as X, Y, and Z.
The X position is right and left.
The Y position is up and down.
The Z position is forward and back.
These positions are often written as (X, Y, Z). For example, (15, 20, 25) would be an X value of 15, a Y value of 20, and a Z value of 25.
If you have a position of (0, 0, 0), you are at the “world origin,” so to speak – the center of the universe, or at least the center of the scene.
Add 5 to your X position, and you’ve moved 5 units to the right.
Subtract 5 from your X position, and you’ve moved 5 units to the left.
It works similarly for the Y and Z values: adding moves in one direction, subtracting moves in the opposite.
Adding to the Y will take you up, and decreasing it will take you down.
Adding to the Z will take you forward, and decreasing it will take you backward.
It is the combination of these three values which defines where something is in the world. Each of these is called an axis (plural “axes”). So you might hear people say “the X axis” or “the Y axis” or “the X and Z axes.”
The scale and rotation work much the same way: they have the same three axes, each one determining a different direction.
The X scale is the width – left and right.
The Y scale is the height – up and down.
The Z scale is the length – forward and back.
I’m sure you can imagine how rotation works pretty much the same way. The object’s orientation is defined by three angle values between 0 and 360, which determine how it is turned on each axis.
You’ll notice that the tools we use to position, rotate, and scale objects (W, E, and R, respectively) are all color-coded.
The X axis is always red, the Y axis is always green, and the Z axis is always blue.
This is pretty much universally accepted. Get into making 3D models, and you’ll see the same thing – although some programs consider the Y axis to be forward and back and the Z axis to be up and down, which is opposite to how Unity does it.
Making a Floor
Let’s use what we’ve learned to make some cubes, position them, and scale them. But first, let’s make a floor.
Create a Plane, using the same method we made the cube with earlier: GameObject ➤ 3D Object ➤ Plane.
A plane is like one surface of a cube – a paper-thin, flat surface that has no thickness. They’re one-sided: you can’t see them at all if you look at them from the backside. Try navigating your camera beneath the plane and looking up at it. You won’t see anything, as if it never existed. Still, it’ll serve fine for our floor, because we don’t expect to be looking at it from below.
Because we know exactly where we want our floor to be, we can set it up using the Inspector. With the new Plane selected, look to its Transform component in the Inspector.
As stated before, the Transform has a position (where it is), rotation (how it’s turned about), and scale (how big or small it is).
Remember, the Inspector’s primary purpose is to interact with components, not just to view their data. So it exposes the actual values of the position, rotation, and scale of the Transform to us. We can edit the individual axes to our liking, simply by clicking these fields and typing in the numbers we want.
This is a useful way to set things up if you know exactly how you want to set them up, because getting precise values for positions and rotations using the transform tools can be very tedious. We want our plane to be at the world origin (the center of the scene), so use the Inspector to change its position to 0 on all three axes, if it isn’t already. As for the rotation, it should be (0, 0, 0) already, so leave it as is.
Scale and Unit Measurements
Now for the scale. “What is a unit of space?” you might be asking. What does it actually mean when we change a GameObject’s position from 0 to 1? How much space is that?
This is a slightly confusing concept for some. You probably expect a straight answer. The Unity developers decided what it is, right? It’s a foot, or maybe a meter – perhaps a yard.
But that’s not how it works. Don’t worry, though; it’s still quite as simple. It’s just that we must decide what a unit is ourselves. Let’s say we decide that 1 unit is 1 foot. So be it. As long as we follow this in every measurement we make, then that’s what 1 unit means. We make our people between 5 and 6 units tall, roughly. If we want something to be just one inch, we make it a twelfth of a unit (roughly .083). If we want something to be a yard, we make it 3 units.
But one more thing we need to note about the scale of a Transform is the scale is not “how many units wide, long, and tall something is.” It’s actually a multiplier . It multiplies the size of the mesh (the 3D model).
The mesh itself has its own size, and then the scale value of the Transform just multiplies that size.
This is fine for a cube. The cube mesh is 1 unit wide, tall, and long. So if we set its scale to 5, it’s going to be 5 times 1 on each axis, so it’s still just 5 units large.
But a plane is trickier. The mesh itself is 10 units wide and long (and it’s paper-thin, so it really has no height). So when we have a scale of (1, 1, 1) with a plane mesh, it’s actually 10 units wide and long already.

A Plane and a Cube with scale (1, 1, 1) positioned in the exact same spot
This is because the size of their actual meshes is not the same. Even if their scale is the same, the cube mesh is 1 unit wide and long, while the plane is 10 units wide and long. Since their scale is just a multiplier of the mesh size, not a depiction of the actual size of the object, the scale of (1, 1, 1) is not affecting the mesh size at all. It’s multiplying by 1, so of course, it’s leaving them as is.
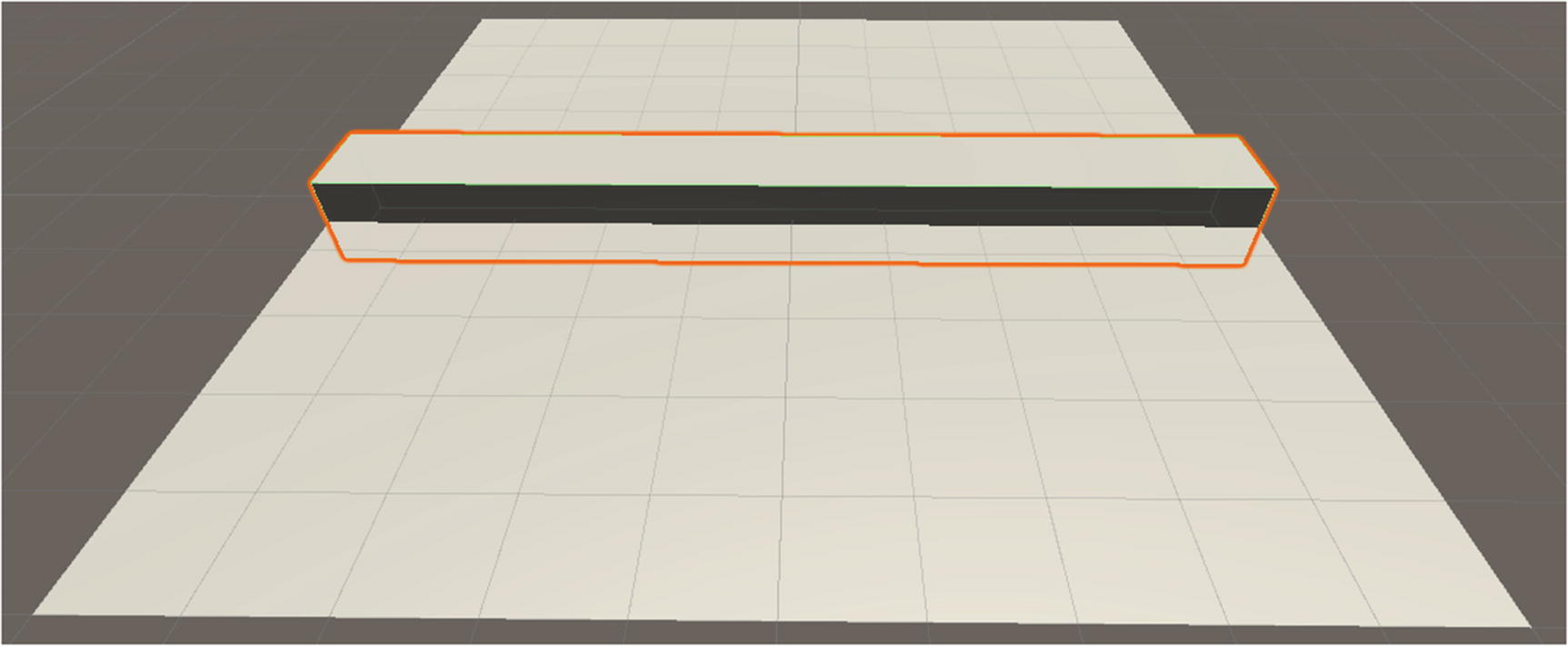
A Plane with scale (1, 1, 1) at the same position as a cube with scale (10, 1, 1). The cube is exactly as wide as the plane
To sum it up, just remember this: the mesh has its own size, and the scale is merely a multiplier for the mesh size. It is not a flat value depicting “how big the mesh is.”
All that aside, let’s press on. We want a large floor so we don’t have to worry about making it bigger every time we want to put some more stuff on it. Let’s make it 10 and 10 on the X and Z scales – which, mind you, is 100 units wide and 100 units long. Of course, it’ll be easier to just set the X and Z scale values to 10 in the Inspector rather than using the scaling tool.
Summary
How to manipulate the position, rotation, and scale of GameObjects using the transform tools (hotkeys W, E, and R).
Positions are resembled as an X, Y, and Z value. Adding to a value moves in one direction, while subtracting from it moves in the opposite direction. X is right (positive) and left (negative), Y is up (positive) and down (negative), and Z is forward (positive) and back (negative). By combining all three values, we can define a 3D point in space.
Scale is a multiplier for the size of the actual mesh that the GameObject is rendering. It’s not the number of units wide, tall, and long a GameObject is. Rather, the X, Y, and Z scale of the Transform multiplies the size of the mesh that’s being rendered.
A single unit doesn’t correspond to a particular number of feet or inches or meters by default in Unity. We have to decide what a unit means ourselves, and as long as we stay consistent with that decision, our objects will end up properly sized proportionate to each other.