In this section, we will discuss another set of transformations that are done by multiplying appropriate matrices (often expressed in homogeneous coordinates) with the image matrix. These transformations change the geometric orientation of an image, hence the name.
Reflecting an image
We can use the transpose() function to reflect an image with regard to the horizontal or vertical axis:
im.transpose(Image.FLIP_LEFT_RIGHT).show() # reflect about the vertical axis
The next figure shows the output image produced by running the previous line of code:

Rotating an image
We can use the rotate() function to rotate an image by an angle (in degrees):
im_45 = im.rotate(45) # rotate the image by 45 degrees
im_45.show() # show the rotated image
The next figure shows the rotated output image produced by running the preceding line of code:
Applying an Affine transformation on an image
A 2-D Affine transformation matrix, T, can be applied on each pixel of an image (in homogeneous coordinates) to undergo an Affine transformation, which is often implemented with inverse mapping (warping). An interested reader is advised to refer to this article (https://sandipanweb.wordpress.com/2018/01/21/recursive-graphics-bilinear-interpolation-and-image-transformation-in-python/) to understand how these transformations can be implemented (from scratch).
The following code shows the output image obtained when the input image is transformed with a shear transform matrix. The data argument in the transform() function is a 6-tuple (a, b, c, d, e, f), which contains the first two rows from an Affine transform matrix. For each pixel (x, y) in the output image, the new value is taken from a position (a x + b y + c, d x + e y + f) in the input image, which is rounded to nearest pixel. The transform() function can be used to scale, translate, rotate, and shear the original image:
im = Image.open("../images/parrot.png")
im.transform((int(1.4*im.width), im.height), Image.AFFINE, data=(1,-0.5,0,0,1,0)).show() # shear
The next figure shows the output image with shear transform, produced by running the previous code:
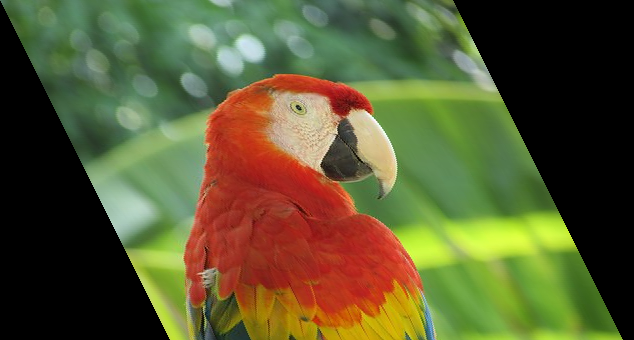
Perspective transformation
We can run a perspective transformation on an image with the transform() function by using the Image.PERSPECTIVE argument, as shown in the next code block:
params = [1, 0.1, 0, -0.1, 0.5, 0, -0.005, -0.001]
im1 = im.transform((im.width//3, im.height), Image.PERSPECTIVE, params, Image.BICUBIC)
im1.show()
The next figure shows the image obtained after the perspective projection, by running the preceding code block:
