In this chapter, you will apply some of the techniques developed in previous chapters to produce some interesting images. These images should give you some idea of the things that can be accomplished with Python graphics.
10.1 Saturn
Saturn is famous for its rings. While Jupiter, Saturn, Uranus, and Neptune also have rings, Saturn’s are the largest, brightest, and most well known in our solar system. They consist of particles as small as dust up to boulder-sized objects. These objects are composed mostly of ice and are thought to have originated when a comet or large asteroid collided with one of Saturn’s moons, shattering both into small pieces. Saturn has been known from ancient times but in 1610 Galileo was the first to observe it with a telescope. The planet is named after Saturn, the Roman god of agriculture, as is our sixth day, Saturday.
Listing 10-1 builds on an earlier program, Listing 7-2 from Chapter 7. That program is left mostly intact here except for the introduction of algorithms that construct Saturn’s rings and the shadow of the planet on the rings.
Figures 10-1 through 10-5 show images produced by Listing 10-1. They are at different angles of orientation, which are listed in the captions. Also listed are the unit vector components of the incoming light rays. For example, lx=+.707, ly=+.707, lz=0 indicates a source in the upper left quadrant; lx=-1, ly=0, lz=0 indicates a light source coming from the right. In the images, please notice the shadow cast by the planet on the rings, especially in Figure 10-5, which shows the curvature of the planet.

Saturn with rings and shadow 1: Rx=-20, Ry=0, Rz=-10, lx=1, ly=0, lz=0 (produced by Listing 10-1)
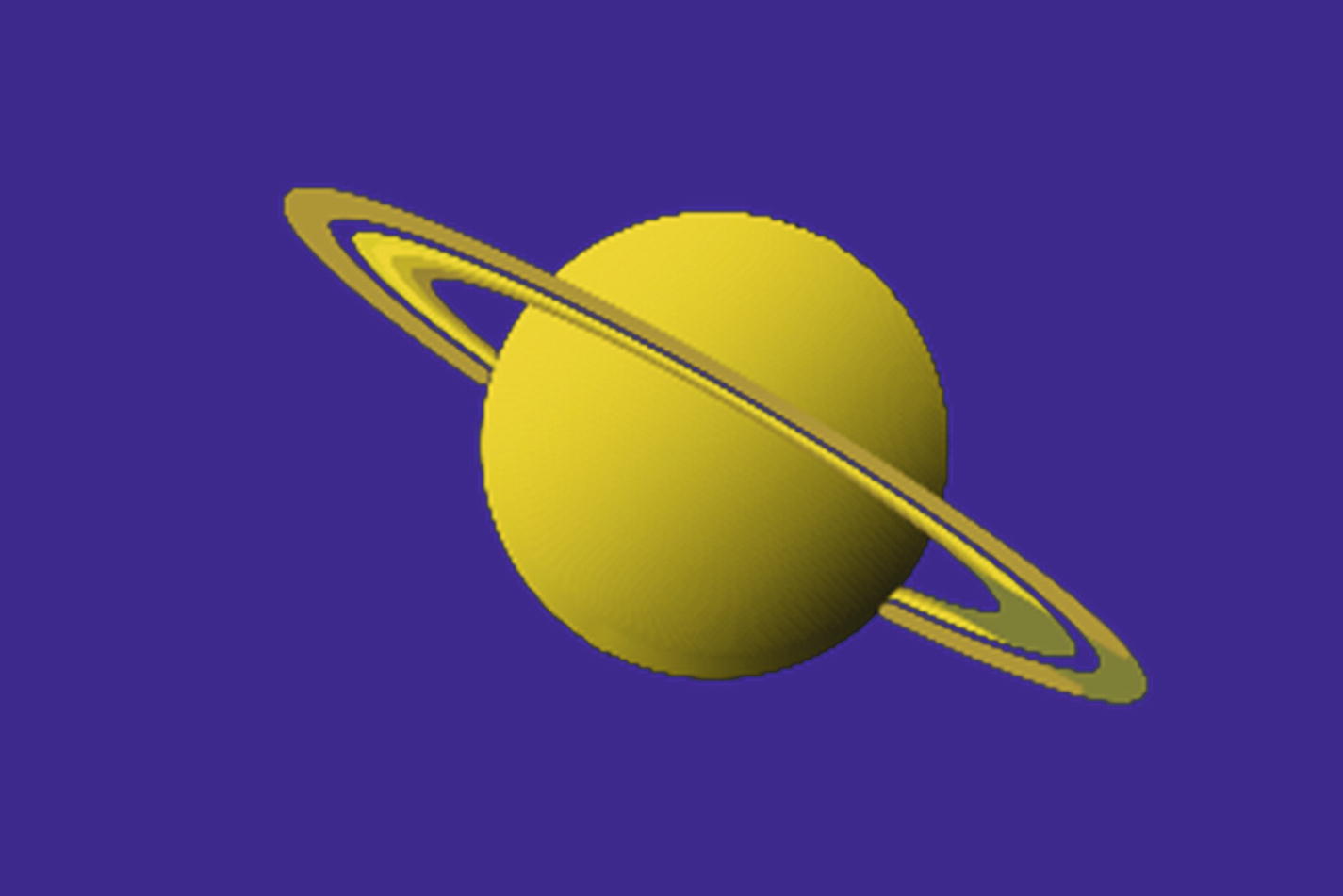
Saturn with rings and shadow 2: Rx=-8, Ry=0, Rz=30, lx=.707, ly=.707, lz=0 (produced by Listing 10-1)
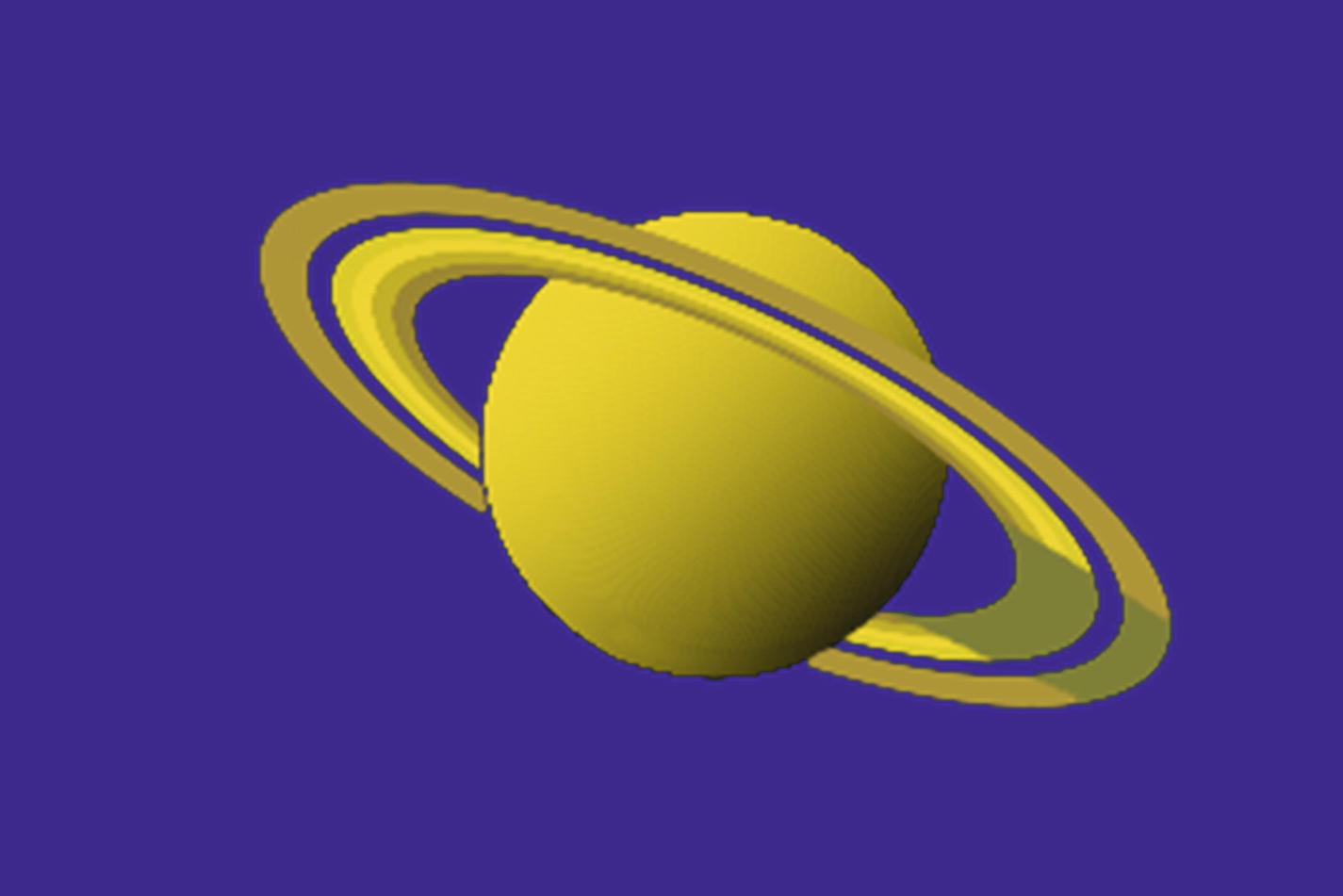
Saturn with rings and shadow 3, Rx=-20, Ry=0, Rz=25, lx=.707, ly=.707, lz=0 (produced by Listing 10-1)
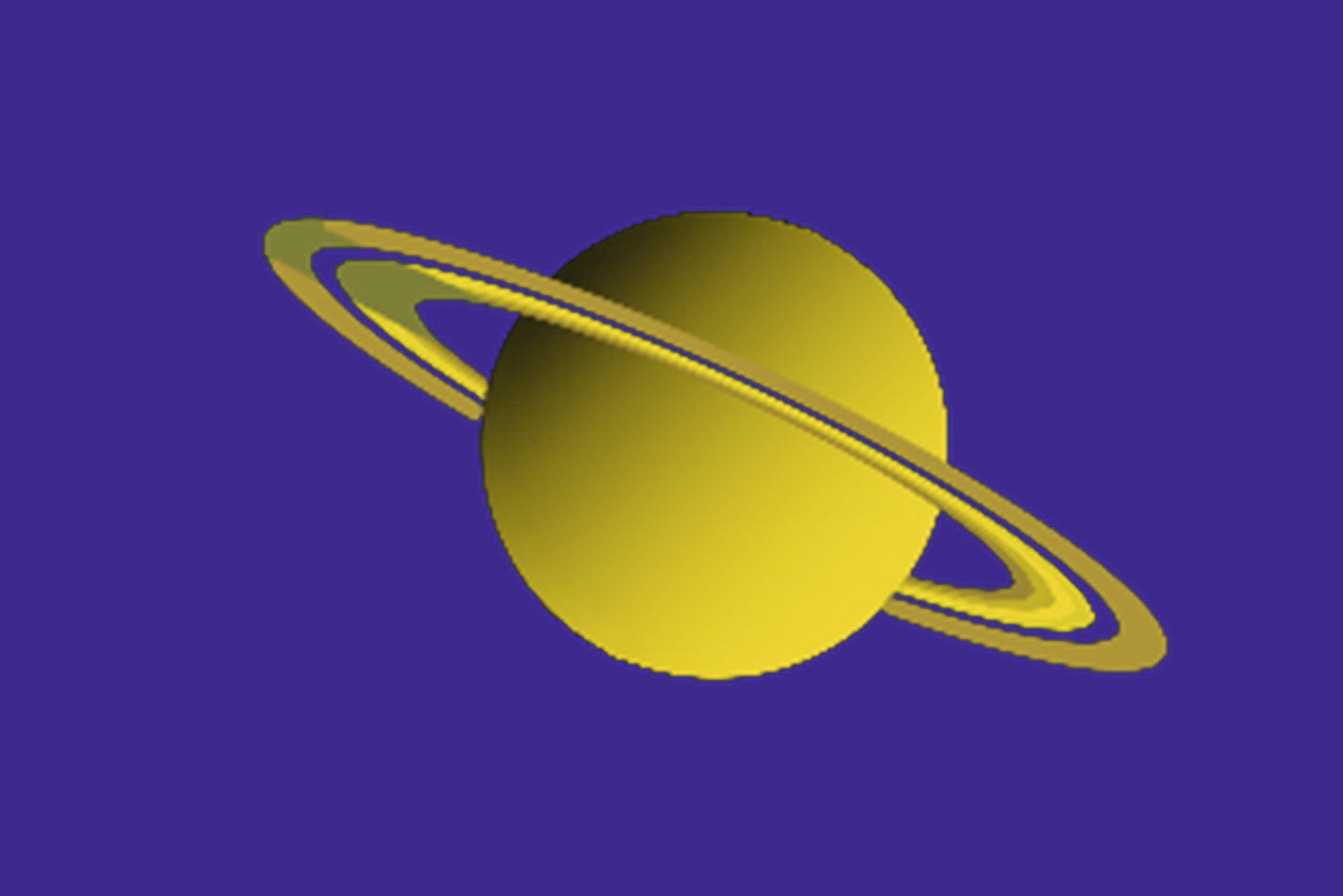
Saturn with rings and shadow 4: Rx=-10, ry=0, Rz=25, lx=-.707, ly=-.707, lz=0 (produced by Listing 10-1)
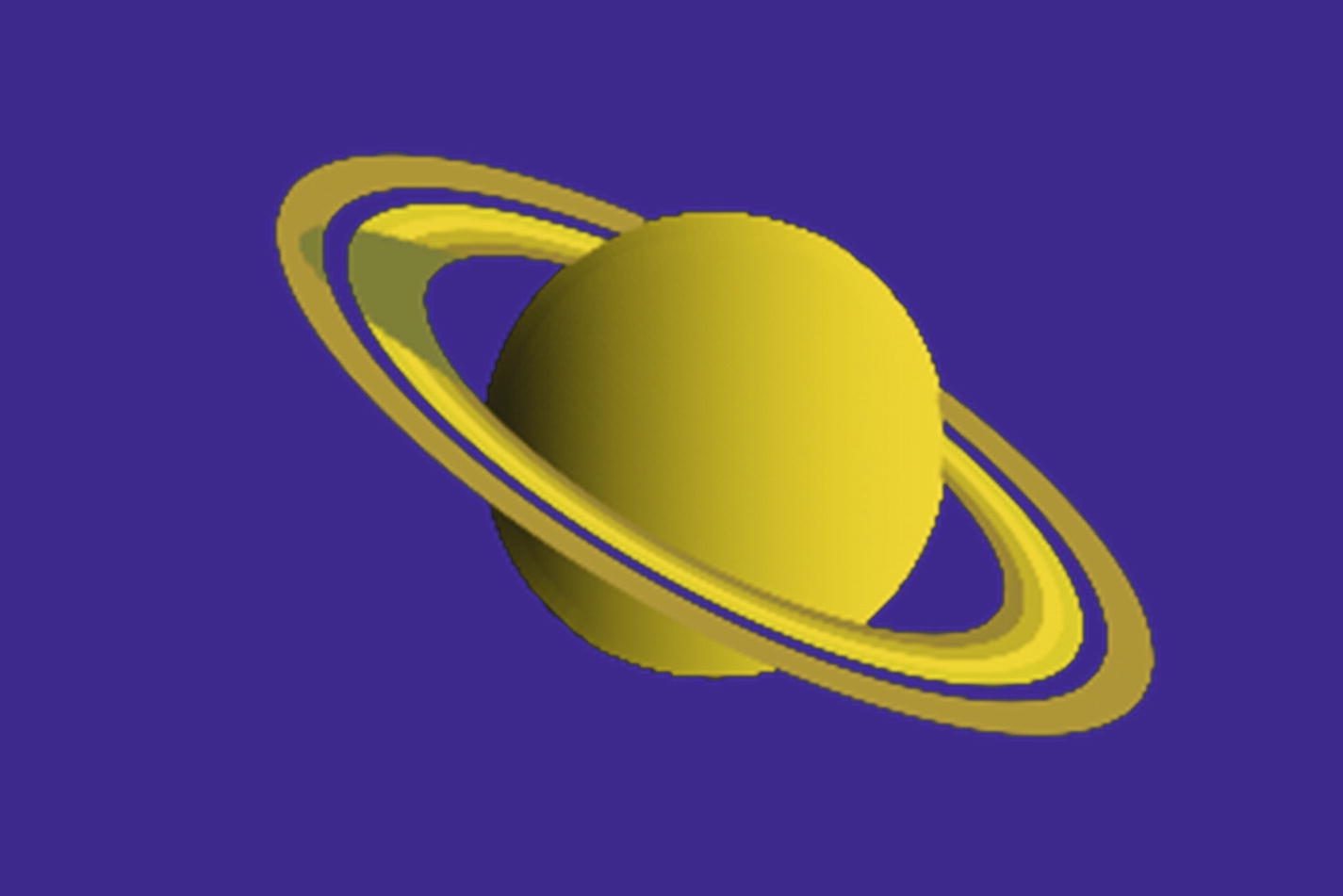
Saturn with rings and shadow 5: Rx=20, Ry=0, Rz=30, lx=-1, ly=0, lz=0 (produced by Listing 10-1)
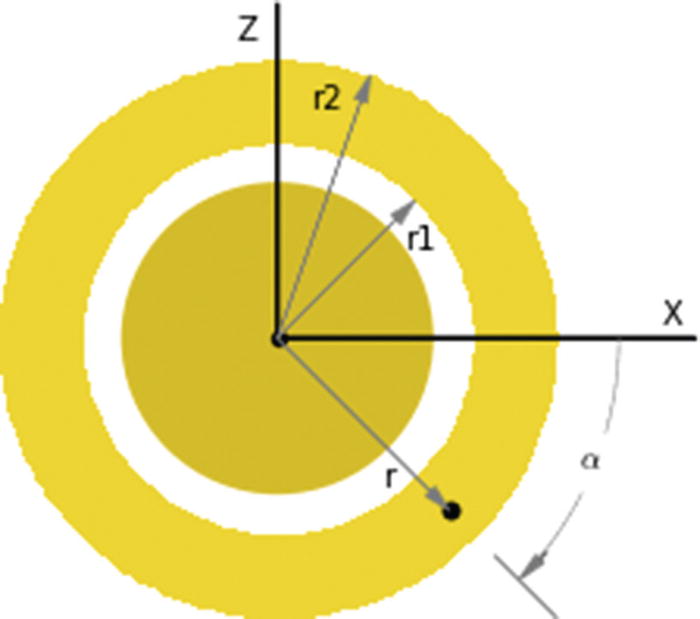
Rings model: top view of planet and rings looking down on the x,z plane with Rx=0, Ry=0, Rz=0
The band is drawn as a series of adjacent concentric circles, each of which is composed of short line segments. Referring to Figure 10-6 and Listing 10-1, program lines 42 and 43 set the inner and outer radii of the rings. Line 44 sets the distance between circles. The rings are divided into seven annular bands (not shown in Figure 10-6) to accommodate different colors; their width is deltar in line 45.
Each line segment is rotated and plotted separately. Line 48 starts a radial direction loop from r1 to r2 plotting the circle segments. Line 49 starts a loop plotting in the circumferential direction. Lines 50-61 do the rotating producing global plotting coordinates xpg and ypg in lines 62 and 63. The rotation functions are the same as in previous programs.
Next, you set the colors of the segments. The rings are arranged in bands of different colors, which are a result of their physical composition as seen in the NASA image. This is done in lines 66-75. The first band, which goes from r=r1 to r1+deltar, has color clr=(.63,.54,.18) and so on for the remaining bands. You omit the fifth band, which is empty; the background color shows through. The sixth band is twice as wide as the others. This provides the colors for the seven bands.
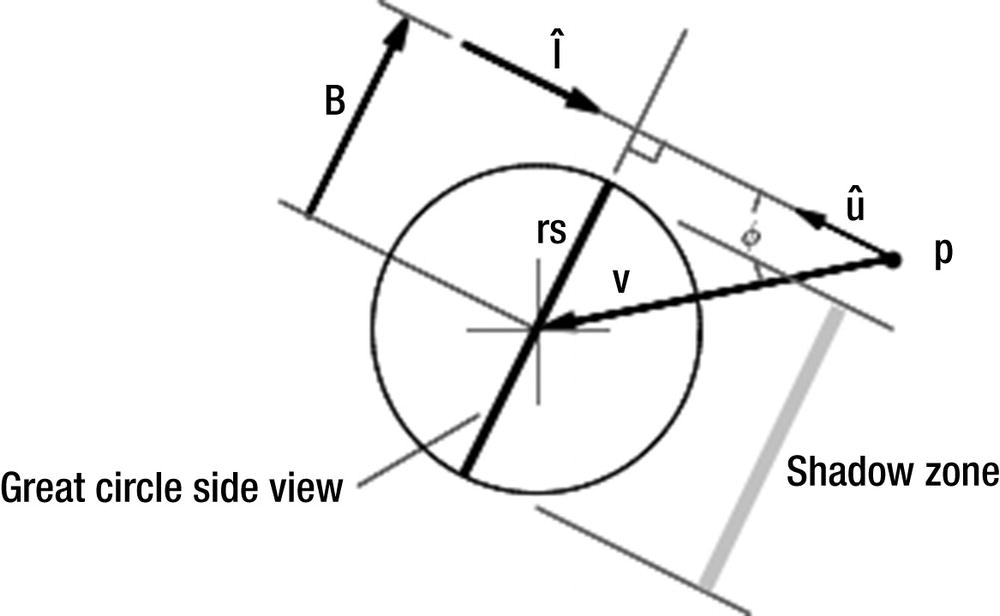
Shadow model

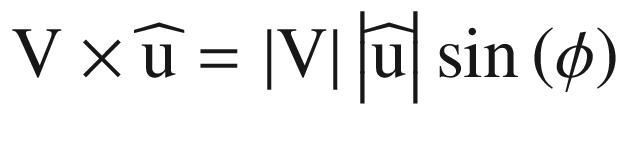


In Listing 10-1, line 78 establishes the length of the incident light vector, ˆl. This should equal 1, but it may not if the components entered in lines 23-25 do not compute to 1 (i.e. ). Lines 79-81 then reestablish the components if necessary. Lines 82-84 establish the components of vector V. Lines 85-87 compute components of B. Line 88 gives its magnitude magB=|B|. Line 89 determines if p lies within the shadow zone. If it does, line 90 is executed. This is the dot product of V with ˆl. It determines whether p lies on the side of the planet that is toward the light source, in which case it is opposite the dark side of the planet and not in the shadow zone. This is necessary since the shadow algorithm in lines 78-89 does not make this distinction. If p does lie on the dark side within the shadow zone, the color is set to a medium grey in line 91.
You will notice in the images above that there is a dark band within the rings. This is because Saturn’s rings have a void in that band: there are no particles there to reflect light; what you see is the background color, 'midnightblue', showing through. This creates a problem since the shadow color will overplot the background color in that void. Lines 93 and 94 reestablish it as 'midnightblue'.
Now that the band colors have been established, you can plot the rings. This is done by plotting short line segments. Lines 97-100 compute the starting location of the first segment. Referring to Figure 10-6, lines 100-101 determine if the segment is in front of the planet, in which case it is plotted. Lines 103-108 determine if it is behind the planet, in which case it is not plotted. This is done by calculating the distance c of the point’s global coordinates from the planet’s center. Line 107 says, if c is greater than the sphere’s radius times 1.075, then plot the segment. The factor of 1.075 is included to prevent the line segments from nibbling into the sphere’s edges. It is necessary to go through this logic; otherwise the front visible segments, which are within the radius of the sphere, won’t be plotted.
Two things can be noted regarding the above images produced by Listing 10-1. First is the color. The NASA photographic image shows a greyish hue, almost devoid of color. But many observers of Saturn have described it as having a golden hue, hence my choice of colors. As any photographer knows, capturing an object’s true colors in a photographic image is difficult; so much depends on the color of the incident light and the image-capturing medium. Perhaps it is best to rely on the observations of stargazers. If you do not agree with the colors in the images produced by Listing 10-1, you can tinker with them by altering the clr definitions in the program. The second thing to notice is the curvature of the shadow that follows the planet’s curvature in Figure 10-5. It shows that the shading algorithm works as expected.
Regarding use of the program, you can change the direction of the incident light in lines 24-26 and the angles of rotation in lines 32-34. Listing 10-1 takes a while to run so be patient.
Program SATURN
10.2 Solar Radiation
This section illustrates a typical scenario: using Python to plot and label curves that represent mathematical functions. Here you plot Max Planck’s spectrum of radiation. You use it to represents the energy spectrum emitted by the Sun, calculate the Sun’s total power output, and the amount that reaches Earth, which is called the solar constant . The scientific aspects of this section are quite interesting, as is the history of their development. The major benefit from a Python programming aspect is seeing how the programs perform numerical integration, set up the plots, and display numerical data.
10.2.1 Photons and the Sun
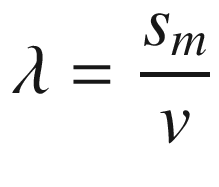
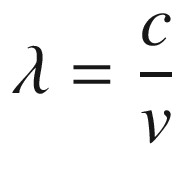
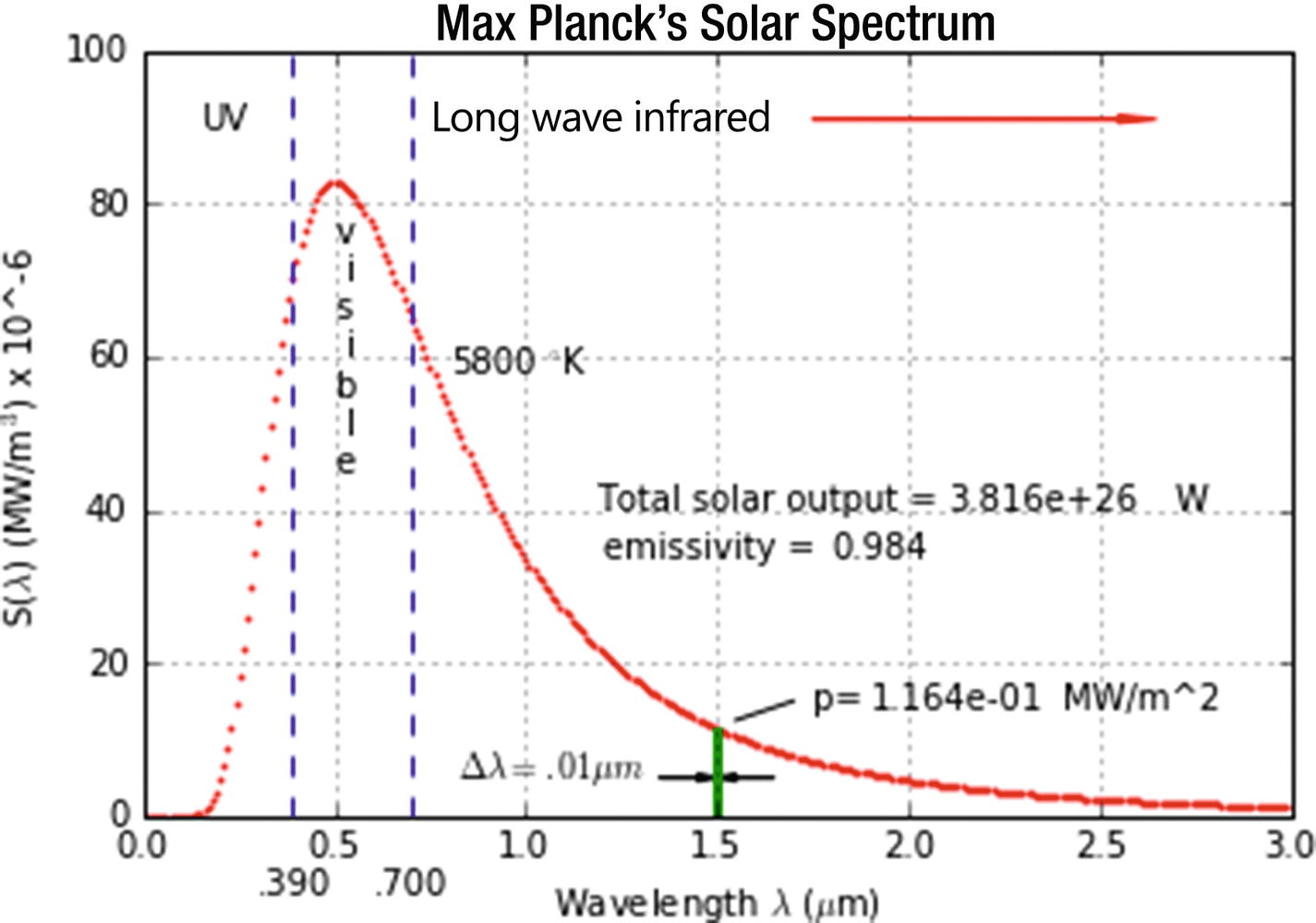
Max Planck’s Solar Spectrum
Most of the frequencies emitted by the Sun, which range from high frequency, short wavelength ultraviolet to low frequency, long wavelength infrared, are invisible to our human eyes. We are able to see only a small range of the spectrum which, fortunately for us, lies near the peak of the Sun’s emitted power spectrum. This must have pleased our hunter-gatherer ancestors since it enabled them to hunt and gather earlier and later in the day. While we can thank the shape of the Sun’s power spectrum for this, it is also a characteristic of our eyes’ biology which, if we believe Charles Darwin, probably evolved to be optimized at the frequencies near the Sun’s maximum power output.
10.2.2 Max Planck’s Black Body Radiation
As mentioned, light is photons. But what is a photon? We know photons are quantized forms of electromagnetic energy. But in the late 19th century, that was still a mystery. There were many attempts to explain the light spectrum that was emitted from heated materials. For example, when we heat up an iron poker, at first we don’t see any change in color but then, after a certain temperature is reached, we visually observe it glowing through a progression of colors: dull red, brighter red, orange, yellow, white, blue, and then violet. These colors correspond to different frequencies of the electromagnetic radiation emitted by the object. Early attempts to explain this phenomenon were based on the classical theory at that time called Maxwell’s Equations. These equations describe an electromagnetic field where the electromagnetic energy is assumed to be a smooth continuum. Despite many attempts, this approach failed to explain what was being observed.
Many scientists at the time struggled with this problem. Then in 1900, Max Planck, a German physicist, sent a postcard to a colleague. On the back he had written an equation that accurately described the spectrum. Plank’s breakthrough was to assume, contrary to the prevailing theories at the time, that the electromagnetic field was not a continuum of energy. Rather, he guessed that electromagnetic energy exists in discrete packets and not as a continuous field, as was assumed by Maxwell’s equations. This led to his breakthrough formulation, shown in Equation 10-7. He presented his idea to the German Physical Society on December 14, 1900, a date that has become known as the birth of quantum mechanics. His equation is known as the blackbody radiation formula . You will see more of it later.
In 1901, Planck published his results in an article in Annalen der Physik in which he hypothesized that electromagnetic energy could only be released by a source, such as the Sun, in the form of discrete packets of energy rather than as continuous waves. Since it was known that light exhibits wave characteristics, in 1905 Albert Einstein extended this idea by suggesting Planck’s discrete “packets” could only exist as discrete “wave-packets.” He called such a packet a Lichtquant or “light quantum.” Later, in 1928, Arthur Compton used the term “photon” which derives from Phos, the Greek word for light.
Planck also assumed that the source of the wave packets are thermally excited charges, each emitting a packet of electromagnetic energy, a photon, at a particular frequency. The more charges emitting photons at the same frequency, the greater the power of the emitted light at that frequency. He further theorized that the energy of a wave packet could only occur at specific fixed energy levels or states.
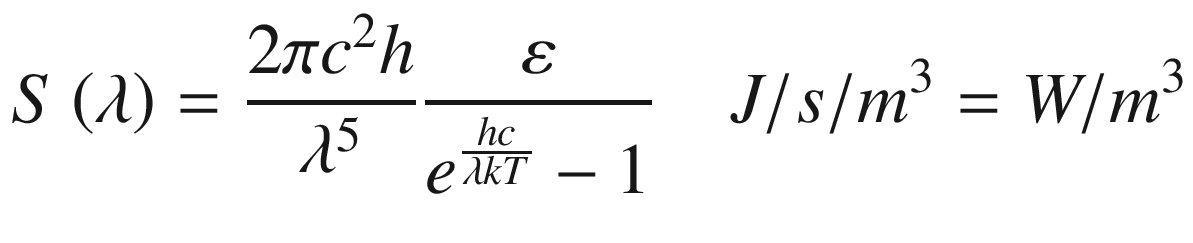
Over the past 100+ years this relation has withstood the test of time and gives very accurate results. Displayed in Figure 10-8, it is often referred to as Planck’s black body radiation formula . It applies equally well to all radiating bodies as well as the Sun. Even though the Sun certainly doesn’t look like a black body, as far as its radiation characteristics are concerned it behaves like one—a very hot one. As an analogy, you might think of the Sun as being a very hot (about 5800°K) black stove glowing very brightly.
Figure 10-8 shows the solar output spectrum (red curve) of the Sun as predicted by Equation 10-7. This is called a power spectral density, or simply a power spectrum. Each point on the curve gives the power density S(λ) at a corresponding wavelength λ. The green band shown in the figure will be explained later.
10.2.3 The Sun’s Total Power Output
The quantity S(λ) displayed in Figure 10-8 is a power density . What is a power density and how does it differ from a simple power? Notice in Equation 10-7 that the units of S(λ) are power per cubic volume. These are the units of a density. You might think of this “density” as analogous to mass density that has units of mass per cubic volume. In the case of S(λ) you are dealing with a power density.


Equation 10-9 gives the power emitted by the Sun over the bandwidth λ1 to λ2. It equals the integral of S(λ) times the infinitesimally small bandwidth dλ. In other words, if you pick a point along the S(λ) curve, as shown in Figure 10-9, and multiply it by dλ and then sum all those values from λ1 to λ2, you would get the total electromagnetic power emitted by the wavelengths in the waveband λ1 to λ2. This is the area under the S(λ) curve from λ1 to λ2.

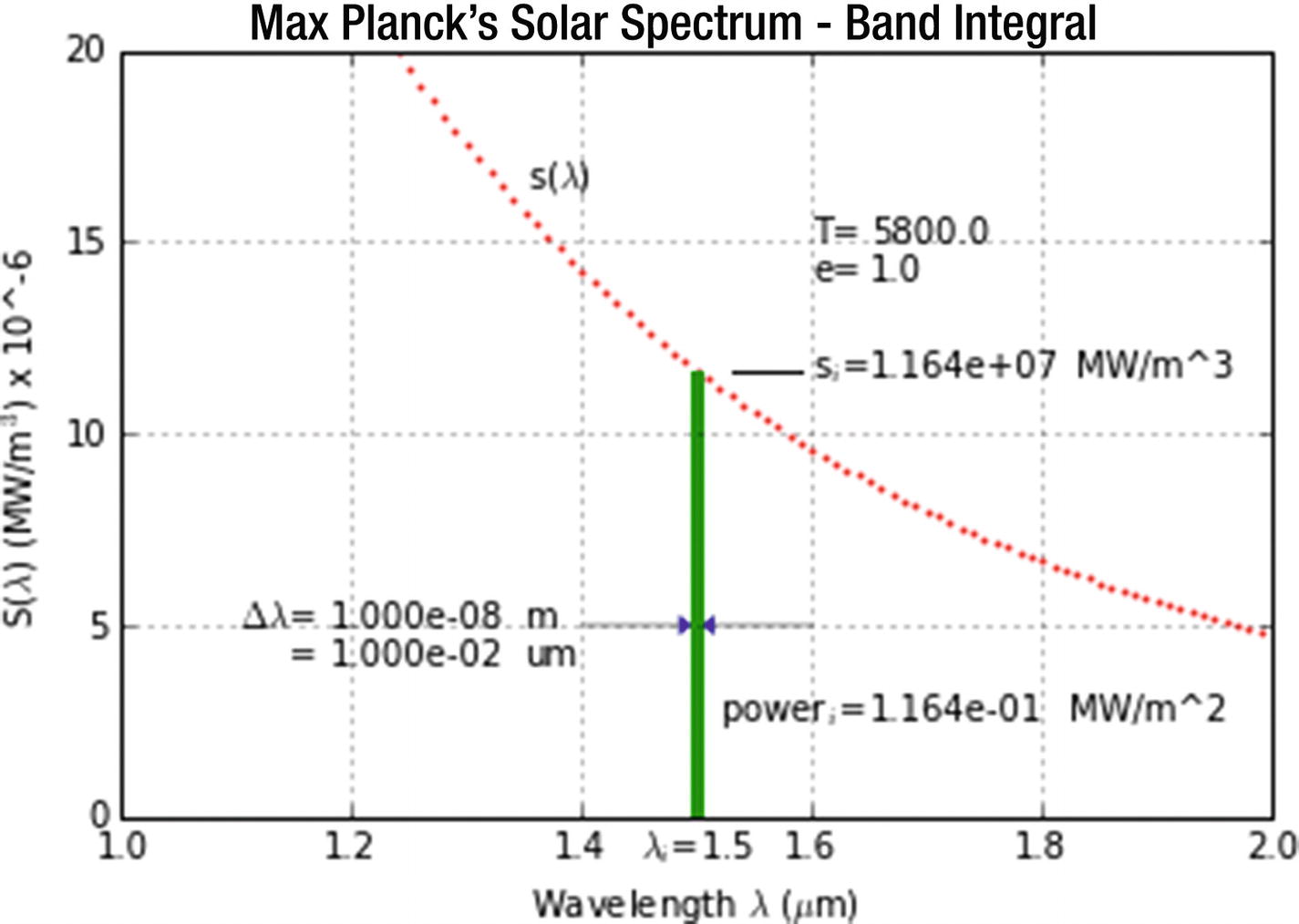
Numerical integration of power S(λ)dλ emitted by spectrum bandi across a .01 μm bandwidth at λi=1.5 (produced by Listing 10-2)
Equation 10-10 is an approximation to Equation 10-9 because it assumes the value of S(λ) is constant across the width of each band ∆λ. However, if ∆λ is chosen small enough, the curve S(λ i − ∆λ/2) → S(λ i + ∆λ/2) can be approximated by the constant value S(λ i ) across the bandwidth ∆λ, in which case the results can be quite accurate. With this simple integration scheme, the power in the band equals the band’s rectangular area. While there are more sophisticated integration schemes you could use, this one is simple, easy to program, and adequate for your purposes.

In Listing 10-2, which plots Figure 10-9, the area of the band is calculated according to Equation 10-11. The magnitude of ∆λ is arbitrary. In the program, it is the parameter dla , which is set to .01x10−6 meters or .01 μm. Whether ∆λ is large or small, the power it emits will be the power radiated by the wavelengths across that bandwidth. Wider bandwidths will generate proportionally more power, narrower ones less. Later, when you do a numerical integration of the area under the entire S(λ) curve to get the total power radiated by the Sun across its entire spectrum, choosing a small value of ∆λ will lead to more accurate results.
In Figure 10-9, the band is shown at λ=1.5 um. The corresponding value of S, as calculated by the program, is 1.164x107 MW/m3. With a bandwidth of .01 μm, which equals 1.0x10−8 meters, the power generated by this band is (1.164x107)x(1x10−8)=.1164 MW/m2, about what a small power plant produces.
Note that the units of S(λ) will be consistent with those of the input parameters: speed of light, Planck’s Constant, Boltzman’s Constant, and wavelength λ. The units of these parameters should be consistent with one another. To avoid confusion, in this work you will keep all of these quantities in the spatial dimension of meters when evaluating Equation 10-7. S(λ) will then have the units (J/s)/m3, which is the same as W/m3. If output is needed in another power dimension, such as kW or MW, the conversion can be done after S(λ) has been evaluated by multiplying S(λ) in watts by 10−3 to get kilowatts or 10−6 to get megawatts. When calculating power emitted across a waveband, the width of that band ∆λ should also be in meters. For example, 1.5μm should be specified as 1.5x10−6m. Conversion from meters back to micrometers μm for display or other purposes later can be done by multiplying λ meters by 10+6. This is shown in Listing 10-2 in the section plot s curve in line lag=la*10**6.
In the figure, S i is shown with a value of 1.164x107 MW/m3. The S(λ) axis indicates a value of 11.64 MW/m3x10−6, which indicates that the value of 11.64 has been multiplied by 10−6 for display purposes. This would make its actual value 11.64x10+6, which equals the value calculated by the program. This is displayed on the plot as 1.164x10+7 MW/m3.
In Listing 10-2, which created Figure 10-9, the section plot S curve solves Equation 10-7 for values of wavelength la, which go from la=lamin to lamax in increments dla . The comments within the code trace the evolution of the units of S. As given by Equation 10-7, when the parameters are as indicated in section establish parameters, the units of s start off as Joules/second per cubic meter (remember s(λ) is a density). Since one Joule per second defines the watt, the units are watts per cubic meter. These are converted to megawatts per cubic meter and then scaled to be plotted against the vertical axis in the units (MW/m3)x10−6 as the variable sg. The 10−6 factor indicates the actual values have been multiplied by that amount. Next, the green band is plotted and the values of temperature and emissivity are displayed.
The value of S(λ) at λ=1.5 is calculated using Equation 10-7, converted to MW/m3, and then multiplied by the bandwidth dl=.01x10−6 to get pl MW/m2, the power within that bandwidth. The remainder of the program displays the data and cleans up the plot.
Program BANDINTEGRAL
Next, let’s look at Max Planck’s entire black body spectrum as shown in Figure 10-8. It’s titled as “Max Planck’s Solar Spectrum” since the temperature used is that of the Sun, approximately 5800 ° K.
The program that produced this plot, Listing 10-3, follows the logic in the preceding program, Listing 10-2, but here you sum the individual band powers from λ=.01x10−6 to 10.x10−6 meters (.01μm to 10.μm) to get the area under the entire (almost) S(λ) curve. The band you looked at in Listing 10-2 is shown at λ=1.5 um.
The process used here is to simply advance along wavelengths, calculate the value of S(λ) at each wavelength, multiply it by ∆λ to get the power within that band, and then sum the power generated by each band in accordance with Equation 10-10. This will give you the total power emitted by all wavelengths.
You extend the range of integration to 10x10−6 meters in order to get a more accurate measure of the total power under the S(λ) curve. This will be the total spectral power emitted by each square meter of the Sun’s surface. Then you multiply that by the Sun’s spherical surface area to get the total power emitted by the Sun, which is known as the solar luminosity . In Figure 10-8, it is called the “total solar output.” As shown on the plot, its value as calculated by the program is 3.816x1026 watts. This is in close agreement with published values.
Many researchers use e=1.0 for emissivity, which is an idealization that assumes the Sun is a perfect radiator (you can assume it isn’t). Here you use an emissivity of e=.984. When you use Planck’s spectrum to calculate the solar constant, which has been measured by satellite (next section), you must either reduce the temperature of the Sun in your calculations or lower its emissivity to less than 1.0 in order to get the results to agree with measured values. If you choose to stay with a Sun temperature of 5800°K, then you must lower the emissivity to .984 in order to obtain agreement. Another option, as you will see, is to keep e=1.0 and lower the Sun’s temperature to 5777 ° K.
Program PLANCKSSOLARSPECTRUM
10.3 Earth’s Irradiance
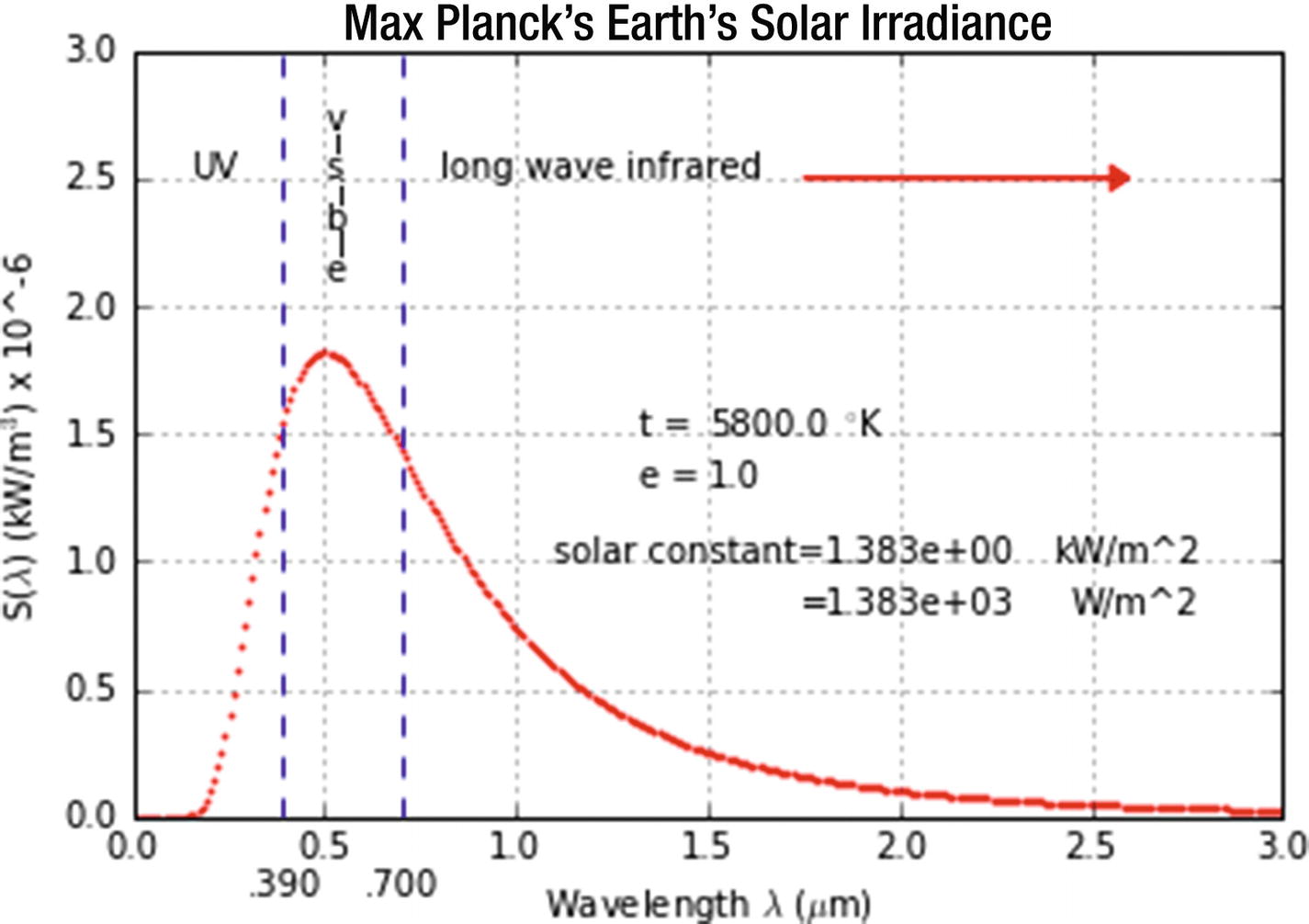
Svpectrum of solar power reaching Earth, the Earth’s solar irradiance, produced by Listing 10-3, which has been modified by inclusion of the inverse square law
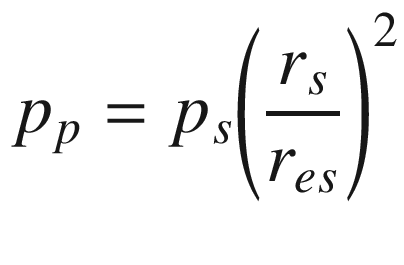

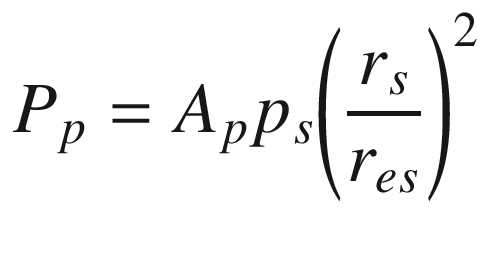
When Equation 10-13 is included in Listing 10-3, the spectrum reduces to Figure 10-10. Again, notice how much lower the values are than in Figure 10-8 as a result of the inverse square law.
P p , which is the solar power reaching the top of the Earth’s atmosphere, is called the solar constant . Its value as measured by satellite is about 1361 W/m2. About 30% of this is reflected off the Earth’s surface and atmosphere by albedo effects such as snow, ice, clouds, water, etc. The remainder is absorbed by the Earth. Much of that is reradiated back into space, allowing the planet to reach a thermal equilibrium. The Earth is also a hot (warm) body and it exhibits its own thermal radiation out into space. But some of what should be reradiated is blocked by greenhouse gasses including CO2, contributing to global warming. All this is, as we know, being actively investigated by climate researchers.
10.3.1 The Earth Sun Model

The Earth-Sun Model produced by Listing 10-4
Program EARTHSUN
10.4 Summary
In this chapter you have seen some typical applications of Python graphics programming. In the first section you saw how relatively easy it is the create the image of Saturn. The planet’s body utilized the sphere and shading algorithms developed in earlier chapters; the bands were developed by constructing concentric rings flat in the x,z plane. The shadow algorithm required a bit of original geometry. The whole thing was then rotated in space about the x,y,z axes. In the section on solar radiation you learned about the physics of solar radiation, especially Max Planck’s black body radiation formula, and Python’s ability to construct technical illustrations. Of special note are the techniques used to scale variables for plotting. Then you learned how to build images such as Figure 10-8 which displays the model used to understand Earth’s solar irradiance.