In this recipe, we will simulate a simple drone with dronekit-sitl. The simulator API is compatible with the Dronekit API that actually controls the drones. Hence, you may develop once and run in simulation and production very easily, as with our previous recipes on Mininet emulation.
First, run the dronekit-sitl in a Terminal before running 13_4_dronekit_sitl_simulation.py:
$ dronekit-sitl copter-3.3 --home=-45.12,149.22,544.55,343.55 os: linux, apm: copter, release: 3.3 SITL already Downloaded and Extracted. Ready to boot. Execute: /home/pradeeban/.dronekit/sitl/copter-3.3/apm --home=-45.12,149.22,544.55,343.55 --model=quad Started model quad at -45.12,149.22,544.55,343.55 at speed 1.0 bind port 5760 for 0 Starting sketch 'ArduCopter' Serial port 0 on TCP port 5760 Starting SITL input Waiting for connection ....
Listing 13.4 provides a simple simulation of a drone, which can connect to the drone or in our case, a simulation running, through a TCP network connection:
#!/usr/bin/env python # Python Network Programming Cookbook, Second Edition -- Chapter - 13 # This program is optimized for Python 2.7.12. # It may run on any other version with/without modifications. import dronekit_sitl from dronekit import connect, VehicleMode # Connect to the default sitl, if not one running. sitl = dronekit_sitl.start_default() connection_string = sitl.connection_string() # Connect to the Vehicle. print("Connected: %s" % (connection_string)) vehicle = connect(connection_string, wait_ready=True) print ("GPS: %s" % vehicle.gps_0) print ("Battery: %s" % vehicle.battery) print ("Last Heartbeat: %s" % vehicle.last_heartbeat) print ("Is Armable?: %s" % vehicle.is_armable) print ("System status: %s" % vehicle.system_status.state) print ("Mode: %s" % vehicle.mode.name) # Close vehicle object before exiting script vehicle.close() print("Completed")
The following code shows the execution of Dronekit:
$ python 13_4_dronekit_sitl_simulation.py Starting copter simulator (SITL) SITL already Downloaded and Extracted. Ready to boot. Connected: tcp:127.0.0.1:5760 >>> APM:Copter V3.3 (d6053245) >>> Frame: QUAD >>> Calibrating barometer >>> Initialising APM... >>> barometer calibration complete >>> GROUND START GPS: GPSInfo:fix=3,num_sat=10 Battery: Battery:voltage=12.587,current=0.0,level=100 Last Heartbeat: 0.862903219997 Is Armable?: False System status: STANDBY Mode: STABILIZE Completed
The following screenshot shows the execution of both the Dronekit and the simulator:
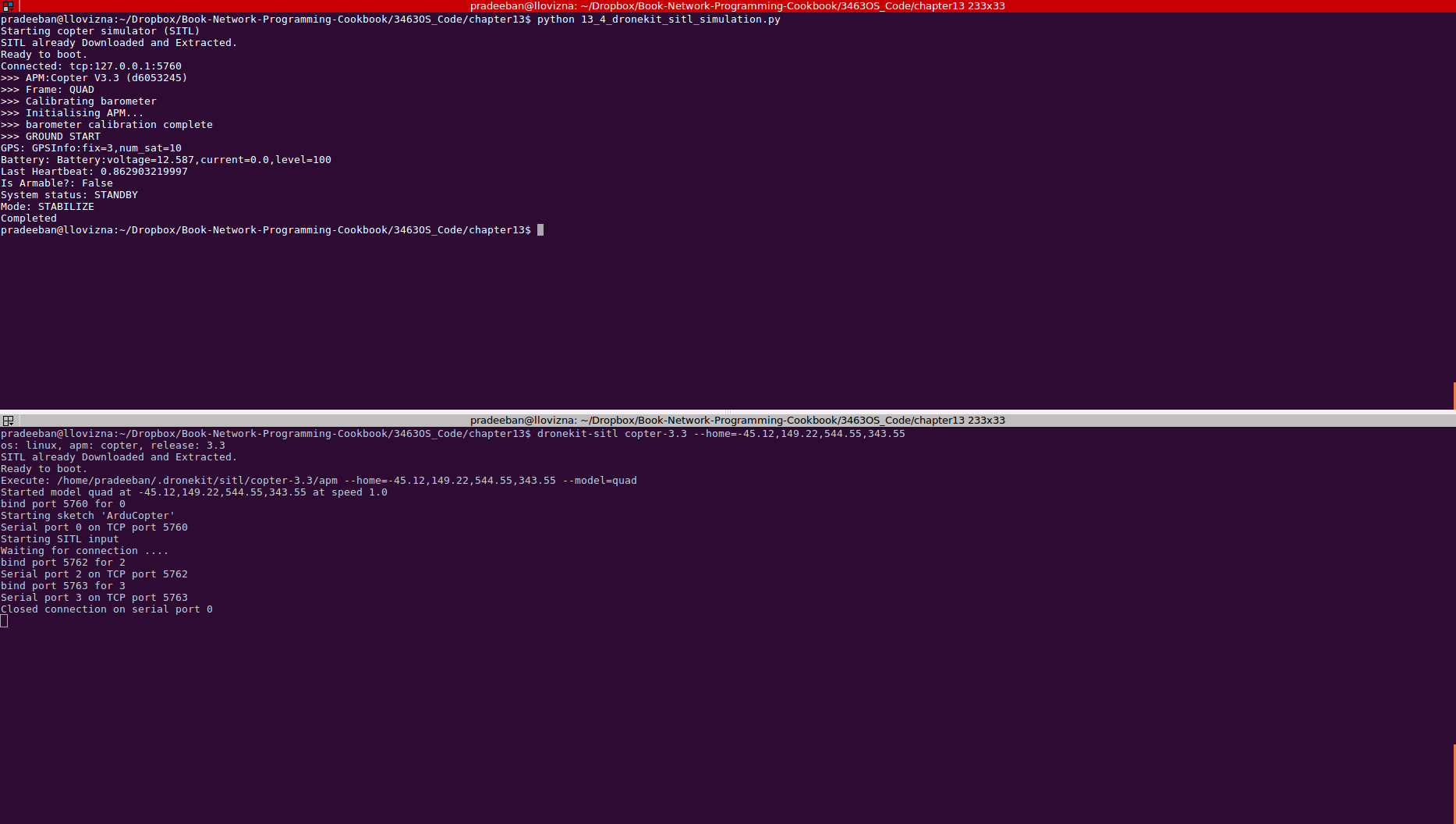
The following lines are printed in the SITL Terminal window, indicating the TCP connection:
bind port 5762 for 2 Serial port 2 on TCP port 5762 bind port 5763 for 3 Serial port 3 on TCP port 5763 Closed connection on serial port 0
This recipe shows the simulated default values and connects to the SITL with the ports, and closes the execution on its own when it completes.