The program in Python on Selenium that tests a specified element on a web application or web page is called a test case. Selenium with Python is a popular choice for testing a web application or web page because there is a lower learning curve to implement it. Python is an easy-to-understand language. Testing requires you to generate simple, understandable test reports for a web application, which can be done with Python and Selenium combined.
This chapter discusses the various test cases used to take a screenshot. In the previous chapter, you learned how POM creates a test case.
Let’s now study the most widely used framework supported by Python in Selenium. The framework implementation as a test case for a web page is also discussed. First, let’s quickly review the test outcomes.
Test Outcomes
The test code has three outcomes: pass, fail, or error. These outcomes define the changes that need to be provided in a test case. The following offers a quick description.
Pass/OK
Pass/OK is the outcome that a developer usually expects. It means that the application is ideal and errorless in terms of functionality and client satisfaction. It is achieved by rigorously testing the web page or application. When a test passes, the application does not need any changes or bug fixes.
Fail
A test fails when a test condition is not satisfied in the corresponding web elements or its behavior. When a test case fails, an AssertionError exception is raised by the system. This error is stated to the developer to make the necessary changes, which are also tested.
Error
An error occurs when an exception is raised. The error may be due to logic, syntax, or semantics and can be traced by the raised exception. This exception is not an AssertionError.
Let’s now look at a few test cases in which the same goal is achieved using multiple approaches.
Test Case 1: Taking a Screenshot
This test case takes a picture (i.e., screenshot) of the web page. The size of the screenshot is determined by specifying the height and width. This is useful in bug analyses reports, for seeing the test case flow, and in recovering failed test cases.
Three methods can be used to capture screen images in Selenium. Each one is described next.
save_screenshot (‘name_of_file’)
get_screenshot_as_file (‘name_of_file’)
get_screenshot_as_png( )
The Python Imaging Library (PIL) Library is used for converting a binary image. It is available in Python. The size of the image is specified before implementing this function.
In Python on Selenium, the save_screenshot ( ) and get_screenshot_as_file ( ) methods can only store the image in .png format, whereas the get_screenshot_as_png ( ) method can store the image in multiple formats.
Now we’ll look at the testing frameworks and the most popular testing framework used in Selenium implementation.
Testing Frameworks
A test case is governed by a set of rules and regulations, which is done by a framework. The framework is used for testing and hence called a testing framework. A testing framework makes a test case more efficient and robust. The framework includes features like handling test data, test standards, test repositories, page objects, and so forth.
Several Python frameworks can be used with Selenium. One of the most commonly used frameworks is unittest. The unittest framework, including its modules and functions, is described next.
The Unittest Framework
The unittest framework is a Python testing framework that is based on the XUnit framework design pattern developed by Kent Beck and Erich Gamma. It is also known as PyUnit. The unittest framework is commonly used to generate reports based on automated test cases.
In this framework, a test case is tested in small individual units (like functions, methods, class, etc.) that determine the correctness of the web element or web page tests. These tests are carried out in small chunks or units by defining classes as methods and using assert functions.
A test fixture executes one or more test cases and the related cleanup actions. Creating proxy or temporary databases, starting a process on a server, or creating new directories are some examples of a text fixture.
In a test case module, small individual units are tested. These units are related to the web elements that are to be tested. The unittest framework contains a base class and the test cases that are used for creating new test cases.
A test suite aggregates multiple test cases. It can also be a combination of test suites. The execution of a test suite is the same as a test case.
A test runner helps to run all the test cases and their associated results. The runner can be integrated with interfaces, such as a graph, text, or a special value indicating return values from the test case.
A test report is the generated result or output of a test case (i.e., whether it is passed or failed). The test case’s execution time is arranged in a systematic way to generate the report. These details are summarized in a report for the client.
Test Case 2: Unittest
First, the unittest library needs to be downloaded using pip in Python. The unittest framework code has several instances that can be incorporated in a test case.
setUp( )
The setUp() method is beneficial when there are multiple test cases for the same web application or web page. This reduces the initial configuration or setup that is required before a test.
tearDown( )
This method contains actions such as closing the web driver or browser, and logout or any closure action related to the test case. The tearDown() method is executed once the setUp() method is executed regardless of the test case results (i.e., pass/fail).
A test case can contain both the setUp( ) and tearDown( ) methods in the same test class in unittest.
setUpClass
The setUp() function is not mandatory in setUpClass. If this class fails to execute, it raises an exception, and the test cases or tearDown() function in it do not execute.
tearDownClass
You can use this method regardless of the setUpClass method.
An example of all the classes and functions is shown in the following simple test case.
setUpModuleandtearDownModule
In the program, the print statement in setUpModule() is printed first followed by setUpClass(), setUp(), test_apress(), tearDown(), tearDownClass(), and finally, tearDownModule() is printed.
Test Execution Order
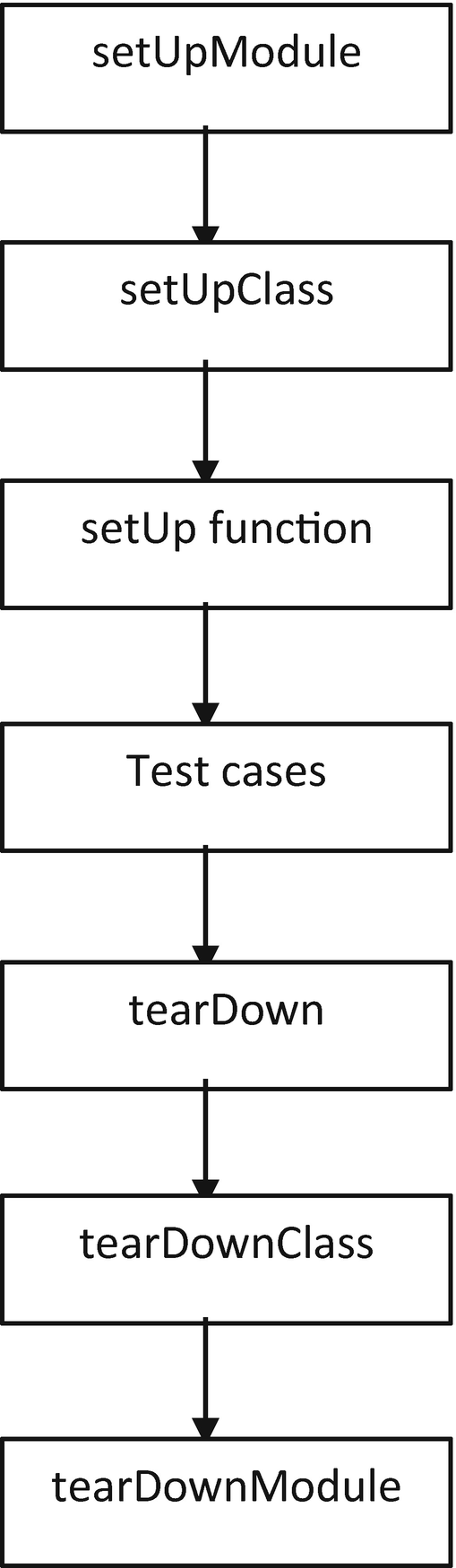
Order of execution in unittest framework
The order of execution for test cases is in alphabetical order by default in unittest. To prioritize, you can define the same name to all the test cases followed by a sequential number for each; for example, test1, test2, test3, test4, and so on.
If there are multiple test cases, then they are executed in alphabetical order.
Testing Tool Comparisons
Testing Tools in Python
Testing Tool | Category | License Type | Description |
---|---|---|---|
DocTest | Unit Testing | Freeware | Generates test cases based on the output of a Python interpreter |
Nose2 | Unit Testing | Freeware | The successor of the nose testing framework Extends features more than the nose framework; very easy to understand |
Pytest | Unit Testing | Freeware | A beginner-friendly tool that supports integration with unittest and nose Easy to build small tests that can later scale to complex functionality testing |
Robot | Acceptance Testing | Freeware | A generic framework for acceptance test-driven development (ATDD) and robotic process automation (RPA) Offers a simple syntax; easy to implement with other frameworks |
Testify | Unit Testing | Freeware | Python-based testing plugin providing more functionality and modules related to reporting Offers features beyond unittest and nose |
Summary
This final chapter explained the test cases intended to get the same results using different approaches. The unitttest framework is also used by Google to automate test cases. The framework is elaborated with basic terminologies and modules. Each module was examined with a testing example associated with it. When all the modules are combined in a single test case, the order of each module execution forms a flowing pattern. We wrapped up the chapter with a comparison of different testing tools supported by Python Selenium.