The previous chapter explained how to locate buttons like default, radio, checkbox, and select list. Each button has a functionality associated with it, such as submit, select, or deselect. Button functionalities are performed with a click action from a mouse (see Chapter 3). This chapter discusses locating web elements like frames and textboxes. It also explains handling single and multiple frames on a web page.
The chapter also covers textbox types, relevant Selenium WebDriver commands, and value insertion.
Iframe
An iframe is another web element available in HTML. It is widely used for embedding media-related web elements (videos, images, etc.) on a web page. YouTube videos and advertisements are two of the most popular examples of embedded videos and images the web pages.
A frame can embed any HTML web element. Frames can be nested within one another. Iframes are defined using the <iframe> tag at the start and the </iframe> tag at end.
Iframes are important to test because they often have web elements that are embedded from other web sites or sources.
To test with web elements that are present in an iframe, you need to switch to that particular frame. It is difficult to locate an iframe because it is in a DOM-like structure. When there are multiple iframe elements in a stack structure, switching to the related iframe gives access to the web elements present within it.
An inline frame is another term used to describe an iframe.
In previous versions of HTML, frameset tags contained the frame tags, but an iframe does not require these tags. One major difference is that iframes can have nested iframes in it, which was not allowed in frames. In Selenium, frames and iframes are treated the same; hence, only iframes are tested in this chapter.
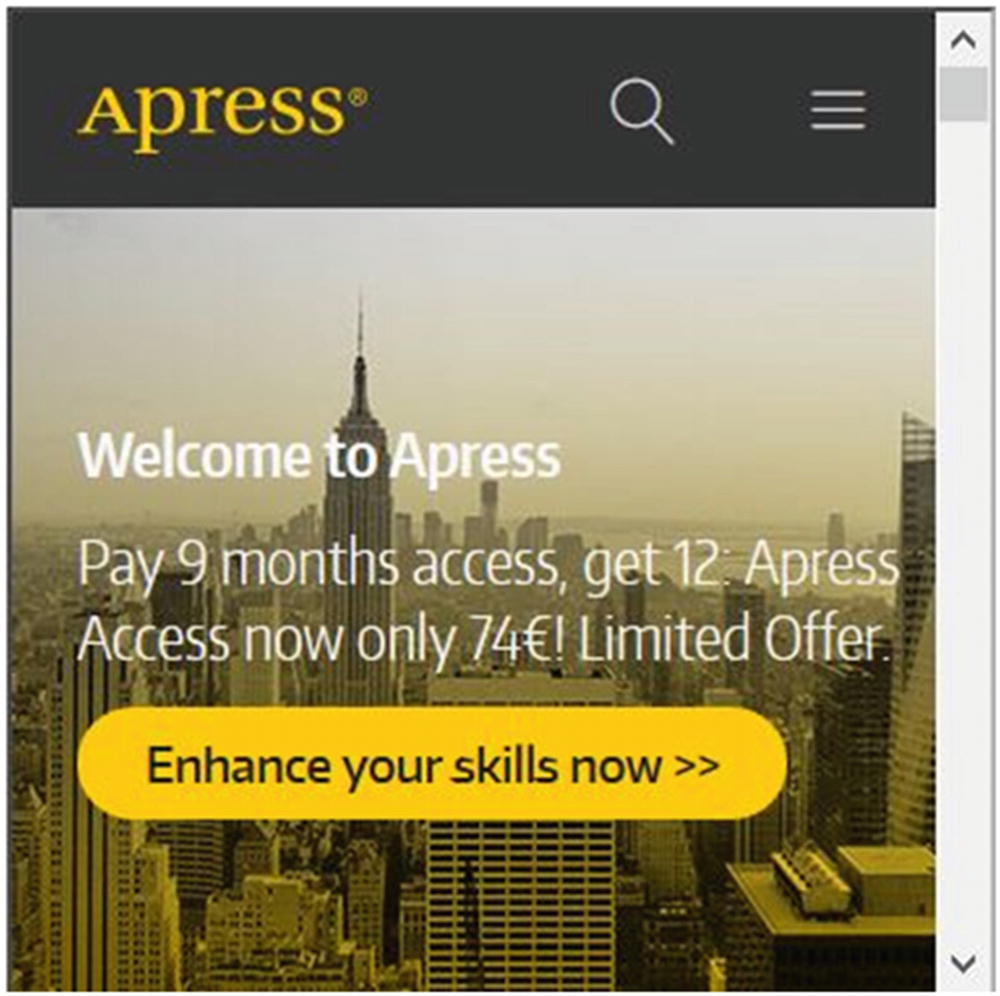
A single iframe
The preceding code displays the Apress web site in an iframe. You can see that the iframe contains the link to the site. The source (src) attribute may have web elements that are within or outside of the web page. The height and width can be in pixels or percentages; here, the pixels are used.
Frame and frameset tags were deprecated in HTML5. Iframes are used instead.
Switching to Iframe
Similar to locating web elements, iframes can be located using the switch function. When a defined iframe is switched, then only tests can be performed on any web element present inside that iframe. Many web pages use iframes because there is no need to reload or refresh the page after interacting with an iframe. The following sections describe ways to switch to an iframe in Python with Selenium.
Switching Using ID
Switching Using Name
Switching Using Index

Multiple iframes
This method is not recommended because there may be changes to a frame’s position when other frames are added or removed from the web page.
Selenium initializes the index value of an iframe on a web page from 0 (zero).
Switching as an Element
When multiple iframes on a web page have the same IDs and names, switching to a specific frame is difficult. In these cases, iframes can be identified by web elements. Web elements like ID and name are for switching to defined iframes.
The iframes have been located using XPath, which is different for every frame.
Since anchor tags are unavailable, link or partial link text is not used to switch an iframe.
Switching to a Main Iframe
There are two ways to switch to the main frame, which are discussed next.
Default Content
Parent Frame
Frames with Waits
Frame ID :
Frame Name :
Frame XPath :
Frame CSS :
Let’s now move on to discuss textboxes.
Textboxes
A textbox accepts user input in the form of text. This text is stored in a database or used by the web page. There are two types of textboxes: single line and multiline.
Single-line Textbox

Single-line textbox
A single-line textbox is commonly used in forms or as a search box on a web page. The Google search engine is the most-used single-line textbox.
Multiline Textbox

Multiline textBox
The textarea can be dragged at the lower-right corner to increase its size. The size of the textarea can be restricted by specifying its corresponding parameters.
A non-editable textbox is used only to display the values associated with it.
Inserting Values
Inserting values in a textbox and textarea are largely the same; the only difference is the lines that incorporate them.
Getting Values from Textbox and Textarea
Summary
This chapter briefly introduced iframes and the ways in which an iframe can be located. There are instances when multiple iframes are present on a web page. The process of switching to a specific iframe was explained with an example.
Textboxes are broadly classified as two types—single line and multiline. A multiline textbox is also known as a textarea. You learned to identify both types of textboxes using locators. You also learned about inserting values in both textbox types and retrieving the value associated with a specified textbox.
The next chapter is about validating web elements.