After we finish the TopStoriesComponent to list top stories, we are going to allow users to view the actual web pages of stories. In this chapter, we’ll use Cordova InAppBrowser plugin to view web pages.
A Simple Solution
Use <a> for a story’s URL
When we run the code inside of an emulator or a device, clicking the title opens the platform’s default browser and shows the web page. On Android, the user can use the back button to return to the app; while on the iOS platform, the user can use the back button on the top left of the status bar to return to the app.
In-App Browser
Using <a> elements is a valid solution for opening links in the app, but it has one major disadvantage in that the user needs to leave the app to read the stories in the browser and return to the app after that. This creates a bad user experience for interrupting the user’s normal flow. Another disadvantage is that we cannot customize the behaviors of the opened browser windows. A better solution is to use the Cordova InAppBrowser plugin ( https://cordova.apache.org/docs/en/latest/reference/cordova-plugin-inappbrowser/ ).
To use Cordova plugins in Ionic apps, Ionic creates a library Ionic Native ( https://ionicframework.com/docs/v2/native/ ) as a wrapper for Cordova plugins. The package @ionic-native/core is already installed when the app is created from the starter template.
Installation
To use this plugin, we need to import the module InAppBrowser and make it as a provider in the AppModule. Then we can inject InAppBrowser into components.
Open a URL
_self - If the URL is in the white list, it opens in the WebView, otherwise it opens in the InAppBrowser.
_blank - Opens in the InAppBrowser.
_system - Opens in the system web browser.
The parameter options is a string to configure the on/off status for different features in InAppBrowser. Different platforms have their own supported options. location is the only option that is supported on all platforms. The option location can be set to yes or no to show or hide the browser’s location bar. The default value of options is location=yes. Options for different features are separated by commas, for example, location=no,hidden=yes.
hidden - When set to yes, the browser is created and loads the page, but it’s hidden. We need to use the method InAppBrowser.show() to show it.
clearcache - When set to yes, the browser’s cookie cache is cleared before opening the page.
clearsessioncache - When set to yes, the browser’s session cookie cache is cleared before opening the page.
Open page
OpenPageService
Updated ItemComponent
Event handler of toOpen
Events
loadstart – Fired when the InAppBrowserObject starts to load a URL.
loadstop – Fired when the InAppBrowserObject finishes loading a URL.
loaderror – Fired when an error occurred when the InAppBrowserObject is loading a URL.
exit - Fired when the InAppBrowserObject is closed.
To add listeners on a certain event, we can use the method on(event) of InAppBrowserObject object. The method on() returns an Observable< InAppBrowserEvent> object that emits event objects when subscribed. Unsubscribing the Observable removes the event listener.
With these events, we can have fine-grained control over web page loading in the InAppBrowserObject to improve user experiences. For example, we can make the InAppBrowserObject hidden when it’s loading the page and show it after the loading is completed.
Alerts
We can use components ion-loading-controller and ion-loading to show a message when the page is still loading. The component ion-loading only supports adding spinners and texts, but we need to add a cancel button in the loading indicator to allow users to abort the operation. In this case, we should use Ionic components ion-alert-controller and ion-alert.
The usage of components ion-alert-controller and ion-alert is like ion-loading-controller and ion-loading. The component ion-alert-controller has the same method create() to create ion-alert components, but the options are different. The component ion-alert has the same methods present() and dismiss() to show and hide the alert, respectively.
header – The header of the alert.
subHeader – The subheader of the alert.
message – The message to display in the alert.
cssClass – Space-separated extra CSS classes.
inputs – An array of inputs.
buttons – An array of buttons.
backdropDismiss – Whether the alert should be dismissed when the user taps on the backdrop.
type – The type of the input. Possible values are text, radio, checkbox, tel, number, and other valid HTML5 input types.
name – The name of the input.
placeholder – The placeholder of the input.
label – The label of the input, for radio buttons and checkboxes.
value – The value of the input.
checked – Whether the input is checked or not.
id – The id of the input.
disabled – Whether the input is disabled or not.
handler – The handler function for the input.
min – Minimal value of the input.
max – Maximal value of the input.
text – The text of the button.
handler – The expression to evaluate when the button is pressed.
cssClass – Space-separated extra CSS classes.
role – The role of the button – can be null or cancel. When backdropDismiss is set to true and the user taps on the backdrop to dismiss the alert, then the handler of the button with cancel role is invoked.
A Better Solution
We can now create a better implementation that combines the InAppBrowserObject events and alerts to give users more feedback and control over opening web pages. In Listing 7-6, the options when creating a new InAppBrowserObject instance is location=no,hidden=yes, which means the location bar is hidden and the browser window is initially hidden. We subscribe to all four different kinds of events: loadstart, loadstop, loaderror, and exit. In the handler of the event loadstart, an ion-alert is created and presented with the button to cancel the loading. In the handler of the event loadstop, we hide the alert and use the method show() of InAppBrowserObject to show the browser window. In the handler of the event loaderror, we create a toast notification and show it. In the handler of the event exit, we unsubscribe all subscriptions to the event observables and use the method close() of InAppBrowserObject to close the browser window.
The handler of the cancel button is the method cancel() of OpenPageService, so when the cancel button is tapped, the loading alert is dismissed and the browser window is closed.
Updated OpenPageService
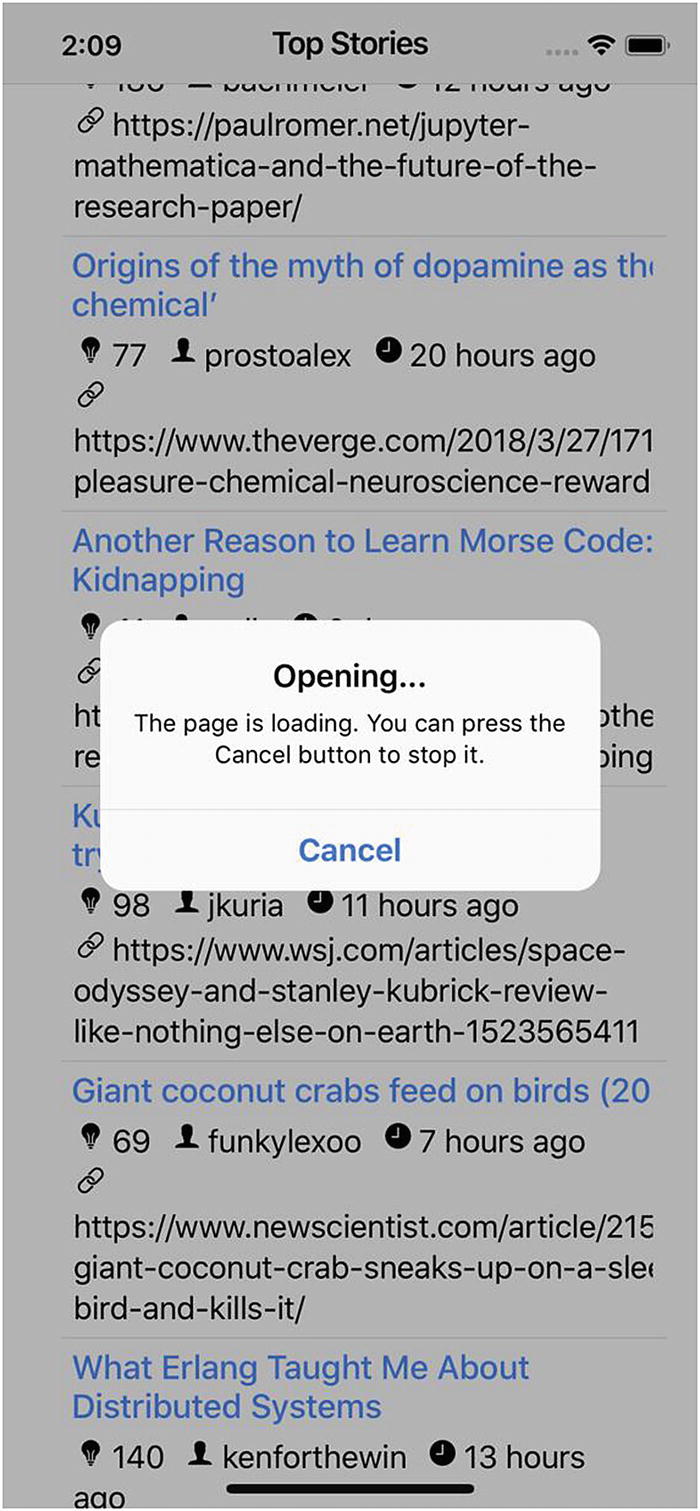
Opening page
Testing
Open page test spec
Summary
In this chapter, we implemented the user story to view stories. We used the plugin InAppBrowser to allow users to view the web pages inside of the app itself. Events of InAppBrowser are used to control its behavior. By using Ionic 4 Alerts, we can create a smooth experience for users to view web pages. In the next chapter, we’ll implement the user story to view comments of stories.