The preceding application does nothing other than display a static file in the Electron shell. Let's add some more functionality to the application to get a much more clear idea about what Electron can do and how it works. You can get the source code for this section in example02 folder of the chapter 01 code bundle.
As we discussed earlier, with Electron you can directly access Node.js and its modules directly from your web page. Let's expand our application to use node native file system modules to access operating systems file system. We don't have the readme file inside the application, which is essential for providing some information about the application to other developers. So let's add one readme.md file to the root of the application and add some dummy markdown content. If you are new to markdown, it's a lightweight markup language with plain text formatting syntax. It can be converted to HTML and many other formats.
Create a readme.md file with the sample content. You can get some same dummy content here from this URL: https://raw.githubusercontent.com/electron/electron/master/README.md. We then need to display this markdown into the HTML page by transforming the markdown to HTML.
Create a new JavaScript file named markdown-processor.js in the same directory where your main.js sits. Node.js does not support parsing markdown file natively. But there are lots of third-party libraries available over the Internet. Let's check how to install third-party node modules via npm. Install Node.js markdown parser called marked (https://github.com/chjj/marked) into the application via npm by entering the following command from your project directory:
npm install --save marked
Save the flag that says that this library information should be added to the package.json dependencies section.
Let's add some code to the markdown-processor.js file:
// imports the node js module
const fs = require('fs');
const marked = require('marked');
// read the contents of readme.md file using fs module
const content = fs.readFileSync('readme.md', 'utf-8');
// transform readme.md file's content into html using marked library
const html = marked(content);
// update the DOM with the transformed html. Even if this is a node context
// we have the access to the browser DOM
document.getElementById('viewer').innerHTML = html;
The preceding code is pretty much straightforward. The first two lines use the require function to load the external modules into the current scope. It's like importing some other classes and methods in Java or C#. We use Node's fs module, which gives a wrapper around the standard POSIX function to read the content readme.md file. All the methods inside the fs module have synchronous and asynchronous versions. Usually, when working with Node.js in server environments it's always recommended to use an asynchronous version of fs module methods as multiple users need to access the resources concurrently. But here in case of Electron, you can simply use asynchronous or synchronous as we are not targeting multi user experience on the desktop client system Here we use the readFileSync method to read file content synchronously. It then parses the content and transforms it into HTML using the marked library.
Until now it's just an independent module. Import it into your HTML file either using a standard script tag or using node's require function as follows:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Hello World!</title>
<link rel="stylesheet" type="text/css" href="style.css" />
<link rel="stylesheet" type="text/css" href="github-markdown.css" />
<style type="text/css">
#banner {
font-size: 22px;
font-weight: bold;
font-family: Verdana;
margin: 10px;
}
#shellInfo {
margin: 10px;
line-height: 2;
font-size: 14px;
font-family: verdana;
}
</style>
<script>
document.addEventListener('DOMContentLoaded', () => {
const { node, chrome, electron } = process.versions;
document.querySelector('#nodeVersion').innerHTML = node;
document.querySelector('#chromeVersion).innerHTML = chrome;
document.querySelector('#electronVersion).innerHTML = electron;
});
</script>
</head>
<body>
<div id="banner">Electron is up and running.</div>
<div id="shellInfo">
We are using node <span id="nodeVersion"></span>
Chrome <span id="chromeVersion"></span>, and
Electron <span id="electronVersion"></span>.
</div>
<div id="viewer">
</div>
<script type="text/javascript">
require('./markdown-processor');
</script>
</body>
</html>
Our application is ready to display the content of the markdown file. But before running the application, let's add some style to the application. Create a file called style.css in the same directory with the following content:
body, html {
margin: 0;
padding: 0;
height: 100%;
width: 100%;
background: #F5f5f5;
}
#viewer {
margin: 10px auto 0 auto;
max-width: 968px;
background-color: #FFF;
border: solid 1px #ECECEC;
box-shadow: 0 0 15px 0px #ccc;
padding: 15px;
}
Add the style sheet reference to the HTML file. As we are using the markdown file from the official GitHub Electron repository, let's style it using the official GitHub styles. There is an open source GitHub style sheet available for markdown files at this URL (https://github.com/sindresorhus/github-markdown-css). Let's add this CSS to the application:
<head>
...
<link rel="stylesheet" href="style.css" />
<link rel="stylesheet" href="github-markdown.css" />
</head>
....
Run the application using the following command from the current working directory:
electron .
You should get an output like this:
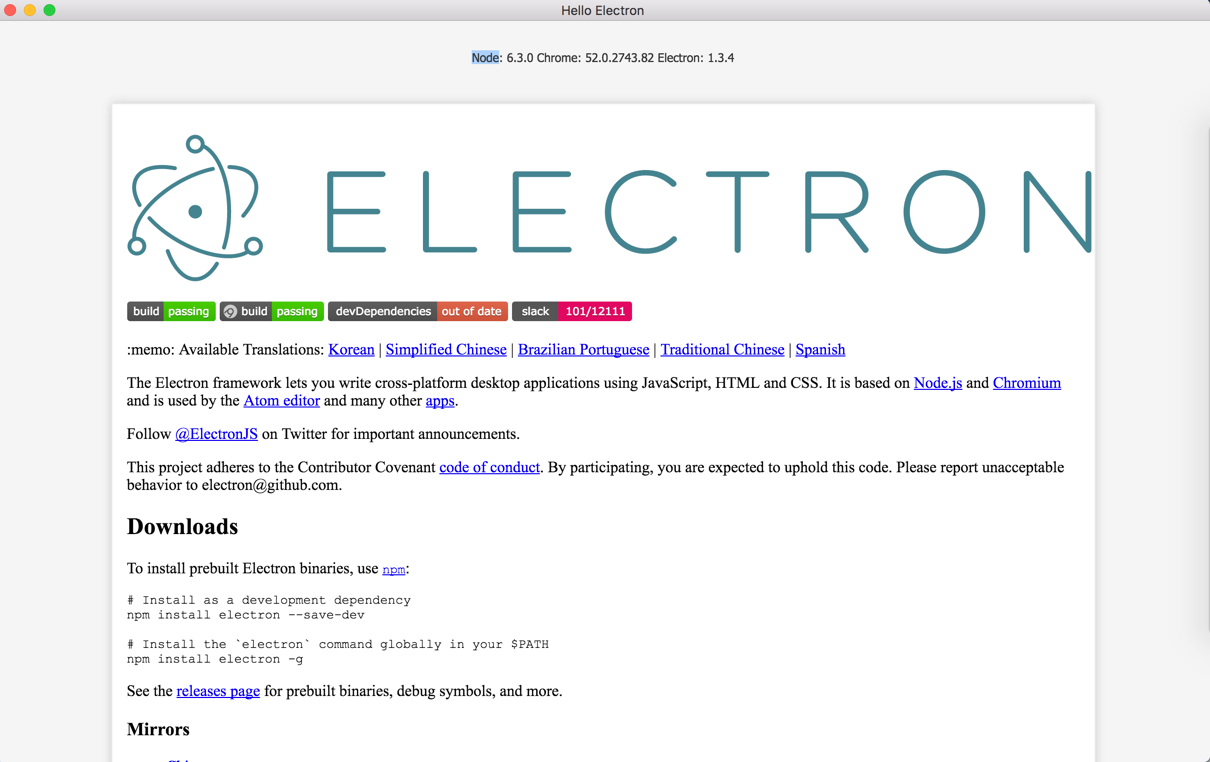
With Electron you can see how to combine the power of node and Chrome together on one page. The power of HTML and CSS allows us to create the amazing user interface. In the preceding example, we could work with native file systems directly from the DOM, which is not possible with traditional web applications. This is why Electron really shines.