Lua has a simple syntax that is easy to both learn and read. The following is a simple script:
-- This is a sample Lua script -- Single line comments begin with two dashes --[[ This is a multi-line comment. Everything between the double square brackets is part of the comment block. ]] -- Lua is loosely typed var = 1 -- This is a comment var ="alpha" -- Another comment var ="A1" -- You get the idea... -- Lua makes extensive use of tables -- Tables are a hybrid of arrays and associative arrays val1 = 1 val2 = 2 my_table = { key1 = val1, key2 = val2, "index 1", "index 2" } --[[ When the Lua script is called from FreeSWITCH you have a few magic objects. The main one is the 'freeswitch' object: freeswitch.consoleLog("INFO","This is a log line ") If script is executed from dialplan (eg: there is an incoming call to manage) you have the 'session' object which lets you manipulate the call: session:answer() session:hangup() ]] freeswitch.consoleLog("INFO","my_table key1 is '" .. my_table["key1"] .."' ") freeswitch.consoleLog("INFO","my_table index 1 is '" .. my_table[1] .."' ") -- Access arguments passed in arg1 = argv[1] -- First argument arg2 = argv[2] -- Second argument -- Simple if/then if ( var =="A1" ) then freeswitch.consoleLog("INFO","var is 'A1' ") end -- Simple if/then/else if ( arg1 =="ciao" ) then freeswitch.consoleLog("INFO","arg1 is 'ciao' ") else freeswitch.consoleLog("INFO","arg1 is not 'ciao'! ") end -- String concatenation uses .. var ="This" .." and " .. "that" freeswitch.consoleLog("INFO","var contains '" .. var .."' ") freeswitch.consoleCleanLog("This Rocks!!! "); -- The end
Save this script as /usr/local/freeswitch/scripts/test2.lua, and then call it from the FreeSWITCH console, as lua test2.lua ciao cucu:
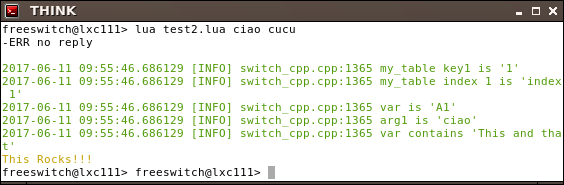