- Install github.com/gorilla/mux and github.com/gorilla/securecookie using the go get command, as follows:
$ go get github.com/gorilla/mux
$ go get github.com/gorilla/securecookie
- Create home.html inside the templates directory, as follows:
$ mkdir templates && cd templates && touch home.html
- Copy the following content to home.html:
<html>
<head>
<title></title>
</head>
<body>
<h1>Welcome {{.userName}}!</h1>
<form method="post" action="/logout">
<button type="submit">Logout</button>
</form>
</body>
</html>
In the preceding template, we defined a placeholder, {{.userName}}, whose values will be substituted by the template engine at runtime and a Logout button. By clicking the Logout button, the client will make a POST call to a form action, which is /logout in our case.
- Create html-form-login-logout.go, where we will parse the login form, read the username field, and set a session cookie when a user clicks the Login button. We also clear the session once a user clicks the Logout button, as follows:
package main
import
(
"html/template"
"log"
"net/http"
"github.com/gorilla/mux"
"github.com/gorilla/securecookie"
)
const
(
CONN_HOST = "localhost"
CONN_PORT = "8080"
)
var cookieHandler = securecookie.New
(
securecookie.GenerateRandomKey(64),
securecookie.GenerateRandomKey(32)
)
func getUserName(request *http.Request) (userName string)
{
cookie, err := request.Cookie("session")
if err == nil
{
cookieValue := make(map[string]string)
err = cookieHandler.Decode("session", cookie.Value,
&cookieValue)
if err == nil
{
userName = cookieValue["username"]
}
}
return userName
}
func setSession(userName string, response http.ResponseWriter)
{
value := map[string]string
{
"username": userName,
}
encoded, err := cookieHandler.Encode("session", value)
if err == nil
{
cookie := &http.Cookie
{
Name: "session",
Value: encoded,
Path: "/",
}
http.SetCookie(response, cookie)
}
}
func clearSession(response http.ResponseWriter)
{
cookie := &http.Cookie
{
Name: "session",
Value: "",
Path: "/",
MaxAge: -1,
}
http.SetCookie(response, cookie)
}
func login(response http.ResponseWriter, request *http.Request)
{
username := request.FormValue("username")
password := request.FormValue("password")
target := "/"
if username != "" && password != ""
{
setSession(username, response)
target = "/home"
}
http.Redirect(response, request, target, 302)
}
func logout(response http.ResponseWriter, request *http.Request)
{
clearSession(response)
http.Redirect(response, request, "/", 302)
}
func loginPage(w http.ResponseWriter, r *http.Request)
{
parsedTemplate, _ := template.ParseFiles("templates/
login-form.html")
parsedTemplate.Execute(w, nil)
}
func homePage(response http.ResponseWriter, request *http.Request)
{
userName := getUserName(request)
if userName != ""
{
data := map[string]interface{}
{
"userName": userName,
}
parsedTemplate, _ := template.ParseFiles("templates/home.html")
parsedTemplate.Execute(response, data)
}
else
{
http.Redirect(response, request, "/", 302)
}
}
func main()
{
var router = mux.NewRouter()
router.HandleFunc("/", loginPage)
router.HandleFunc("/home", homePage)
router.HandleFunc("/login", login).Methods("POST")
router.HandleFunc("/logout", logout).Methods("POST")
http.Handle("/", router)
err := http.ListenAndServe(CONN_HOST+":"+CONN_PORT, nil)
if err != nil
{
log.Fatal("error starting http server : ", err)
return
}
}
With everything in place, the directory structure should look like the following:
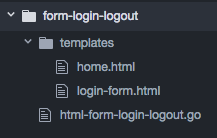
- Run the program with the following command:
$ go run html-form-login-logout.go