Once we run the program, the HTTP server will start locally listening on port 8080, and accessing http://localhost:8080/, http://localhost:8080/post, and http://localhost:8080/hello/foo from a browser or command line will produce the message defined in the corresponding handler definition. For example, execute http://localhost:8080/ from the command line, as follows:
$ curl -X GET -i http://localhost:8080/
This will give us the following response from the server:

We could also execute http://localhost:8080/hello/foo from the command line, as follows:
$ curl -X GET -i http://localhost:8080/hello/foo
This will give us the following response from the server:
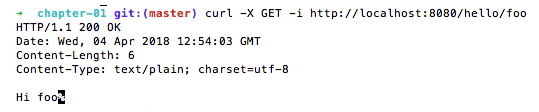
Let's understand the code changes we made in this recipe:
- First, we defined GetRequestHandler and PostRequestHandler, which simply write a message on an HTTP response stream, as follows:
var GetRequestHandler = http.HandlerFunc
(
func(w http.ResponseWriter, r *http.Request)
{
w.Write([]byte("Hello World!"))
}
)
var PostRequestHandler = http.HandlerFunc
(
func(w http.ResponseWriter, r *http.Request)
{
w.Write([]byte("It's a Post Request!"))
}
)
- Next, we defined PathVariableHandler, which extracts request path variables, gets the value, and writes it to an HTTP response stream, as follows:
var PathVariableHandler = http.HandlerFunc
(
func(w http.ResponseWriter, r *http.Request)
{
vars := mux.Vars(r)
name := vars["name"]
w.Write([]byte("Hi " + name))
}
)
- Then, we registered all these handlers with the gorilla/mux router and instantiated it, calling the NewRouter() handler of the mux router, as follows:
func main()
{
router := mux.NewRouter()
router.Handle("/", GetRequestHandler).Methods("GET")
router.Handle("/post", PostCallHandler).Methods("POST")
router.Handle("/hello/{name}", PathVariableHandler).
Methods("GET", "PUT")
http.ListenAndServe(CONN_HOST+":"+CONN_PORT, router)
}