-
Create http-server.go, where we will create a simple HTTP server that will render Hello World! browsing http://docker-machine-ip:8080 or executing curl -X GET http://docker-machine-ip:8080 from the command line, as follows:
package main
import
(
"fmt"
"log"
"net/http"
)
const
(
CONN_HOST = "localhost"
CONN_PORT = "8080"
)
func helloWorld(w http.ResponseWriter, r *http.Request)
{
fmt.Fprintf(w, "Hello World!")
}
func main()
{
http.HandleFunc("/", helloWorld)
err := http.ListenAndServe(CONN_HOST+":"+CONN_PORT, nil)
if err != nil
{
log.Fatal("error starting http server : ", err)
return
}
}
-
Create a DockerFile, which is a text file that contains all the commands needed to build an image. We will use golang:1.9.2 as the base, or the parent image, which we have specified using the FROM directive in the Dockerfile, as follows:
FROM golang:1.9.2
ENV SRC_DIR=/go/src/github.com/arpitaggarwal/
ENV GOBIN=/go/bin
WORKDIR $GOBIN
# Add the source code:
ADD . $SRC_DIR
RUN cd /go/src/;
RUN go install github.com/arpitaggarwal/;
ENTRYPOINT ["./arpitaggarwal"]
EXPOSE 8080
With everything in place, the directory structure should look like the following:
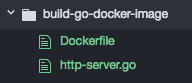
-
Build a Docker image from the Dockerfile executing the docker build command with the image name as golang-image using the -t flag, as follows:
$ docker build --no-cache=true -t golang-image .
Once the preceding command has executed successfully, it will render the following output:
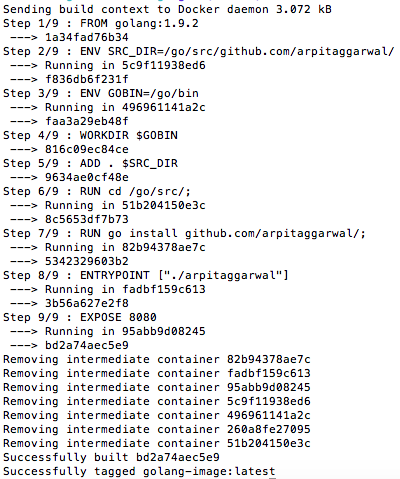
If you are building an image behind a corporate proxy, you will probably have to provide the proxy settings. You can do this by adding environment variables using the ENV statement in the Dockerfile, which we often call as a runtime customization, as follows:
FROM golang:1.9.2
....
ENV http_proxy "http://proxy.corp.com:80"
ENV https_proxy "http://proxy.corp.com:80"
...
We can also pass the proxy settings at build time to the builder using the --build-arg <varname>=<value> flag, which is called as a build time customization, as follows:
$ docker build --no-cache=true --build-arg http_proxy="http://proxy.corp.com:80" -t golang-image.