Visual Studio Code is not just another evolved text editor with syntax colorization and automatic indentation. Instead, it is a very powerful code-focused development environment expressly designed to make it easier to write web, mobile, and cloud applications using languages that are available to different development platforms.
With the ambition to provide a powerful, rich development environment, Visual Studio Code integrates a number of editing features that are focused on improving the productivity and quality of your code. This chapter discusses what languages are supported in Visual Studio Code and all the available code editing features, starting from the most basic that are available to all the supported languages to the most advanced productivity tools that are available to specific languages such as C# and TypeScript.
Note
Keyboard shortcuts used in this chapter are based on the default settings in Visual Studio Code.
Language Support
Language Support by Feature
Languages | Editing Features |
---|---|
Batch, C, C#, C++, Clojure, CoffeeScript, Diff, Dockerfile, F#, Go, HLSL, Jade, Java, HandleBars, Ini, Lua, Makefile, Objective-C, Objective-C++, Perl, PowerShell, Properties, Pug, Python, R, Razor, Ruby, Rust, SCSS, ShaderLab, Shell Script, SQL, Visual Basic, XML | Common features (syntax coloring, bracket matching, basic word completion) |
Groovy, Markdown, PHP, Swift | Common features and code snippets |
CSS, HTML, JSON, JSON with Comments, Less, Sass | Common features, code snippets, IntelliSense, Outline |
TypeScript, TypeScript React, JavaScript, JavaScript React | Common features, code snippets, IntelliSense, Outline, parameter hints, refactoring, Find All References, Go To Definition, Peek Definition |
Visual Studio Code can be extended with additional languages produced by the developer community and that can be downloaded from the Visual Studio Marketplace. This is discussed in more detail in Chapter 6, “Installing and Managing Extensions,” but, in the meantime, you can have a look at the available languages.
Working with C# and C++
The C# programming language deserves a more detailed version, because of its popularity and because it is now a cross-platform language that you can use not only on Windows but also on macOS and Linux. As you can see from Table 3-1, the editing experience that Visual Studio Code offers out of the box for C# is limited to common features.
However, full and rich support for the coding experience with C# is offered via the Microsoft C# free extension ( https://marketplace.visualstudio.com/items?itemName=ms-vscode.csharp ). This provides an optimized experience for .NET Core development and includes all the support and tools you need to build apps with C#, including the necessary support for the .NET Core debugger. With this extension, you will basically get the same experience available to TypeScript, including advanced editing capabilities based on the .NET Compiler Platform (also known as Roslyn) that makes it easier to fix code issues as you type. If you plan to work with C#, I definitely recommend you to install this extension, especially because this chapter discusses some editing features that are available only through the extension. Extensibility is explained in more detail in Chapter 6, "Installing and Managing Extensions," so the easiest way to get the extension installed without further information is opening any C# code file (.cs) and following the instructions shown by Visual Studio Code when it detects that a proper extension is available for that file type.
Similarly, you might want to install the Microsoft C/C++ extension that adds enhanced editing features to the C and C++ languages, plus debugging support for Windows (PDB, MinGW, Cygwin), macOS, and Linux. The extension is available at https://marketplace.visualstudio.com/items?itemName=ms-vscode.cpptools , and you can follow the same steps described before about the C# extension for easy installation, of course opening a .c, .h, or .cpp file.
Basic Code Editing Features
Visual Studio Code provides many of the features you would expect from a powerful code editor. This section describes what editing features make your coding experience amazing with this new tool. If you are familiar with Microsoft Visual Studio 2017, you will also see how some features have been inherited from this IDE. It is worth mentioning that Visual Studio Code provides keyboard shortcuts for almost all the editing features, giving you an option to edit code faster. For this reason, the keyboard shortcut is also mentioned for many of the described features.
Note
Features described in this section apply to all the supported languages described in Table 3-1, except where expressly specified.
Working with Text
As you would expect, the code editor in VS Code offers commands for text manipulation and text selection. The Edit menu provides the Undo, Redo, Copy, Cut, Paste, Find, Replace, Find in Files, and Replace in Files commands. These commands are available in every text editor and do not require any further explanation, except for the find and replace tools that were described in the previous chapter.
The Edit menu also provides two commands to work with code snippets, Emmet: Expand Abbreviation and Emmet…. The first command is the menu representation of keyboard shortcuts offered by code editor to add a code snippet, whereas the second command opens the Command Palette and shows the list of code snippets that you can add. Code snippets are discussed in more detail in the “Reusable Code Snippets” section in this chapter.
The Selection menu not only provides commands for text selection but also commands that make it easier to move or duplicate lines of code above and below the current line. The Add Cursor Above, Add Cursor Below, and Add Cursors To Line Ends commands allow working with multicursors, described in the “Multicursors” section in this chapter.
If you click an identifier, reserved word, or type name in the editor, you can use the Add Next Occurrence, Add Previous Occurrence, and Select All Occurrences commands that allow to quickly select occurrences of the selected word, and occurrences will be highlighted in a different color, which differs depending on the current theme.
Syntax Colorization
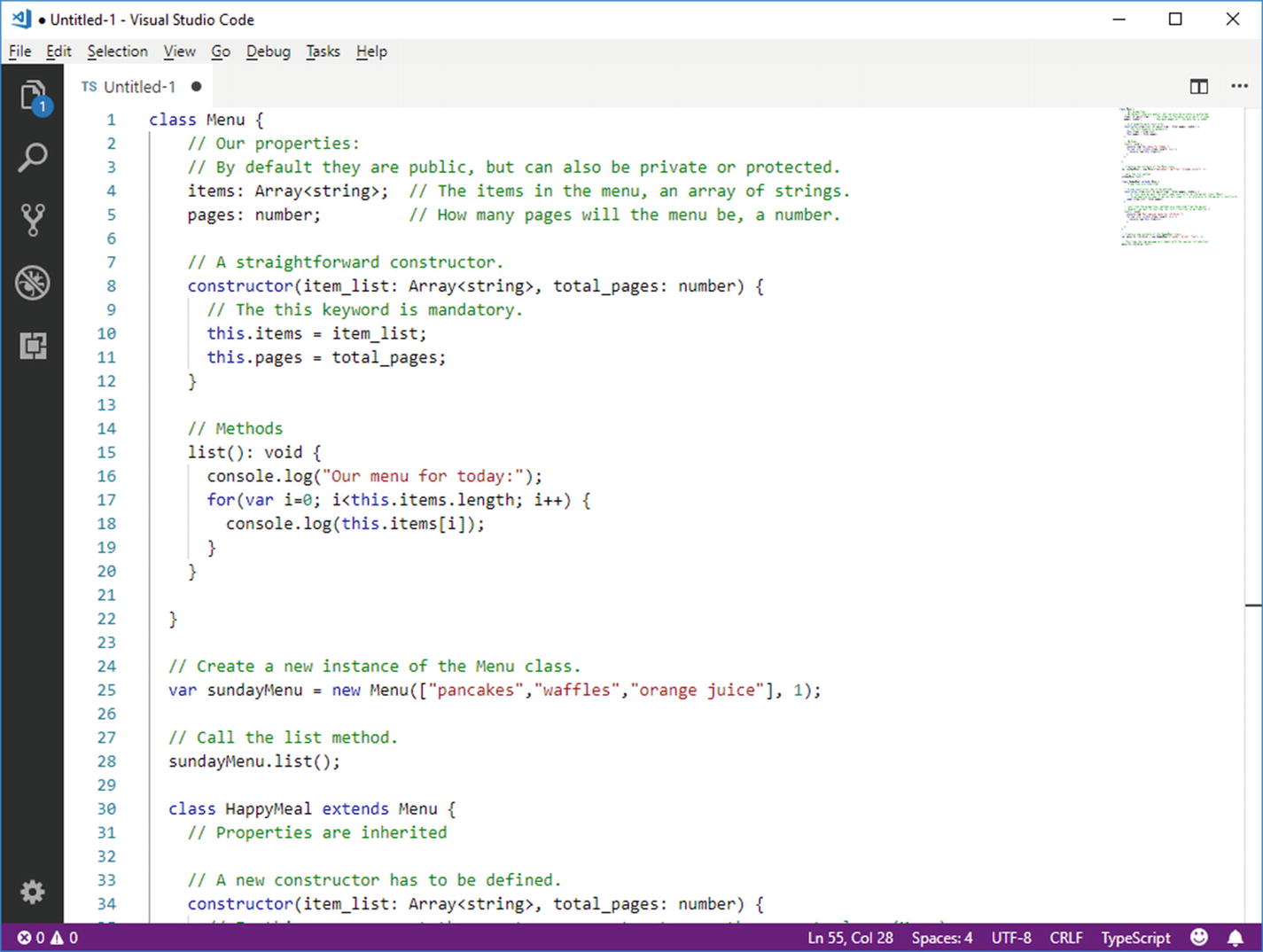
Syntax colorization
Syntax colorization is available for other languages via extensibility. If you need to work with a language that is not included out of the box, you can check the Visual Studio Marketplace and see if an extension is available to support such a language. See Chapter 6, "Installing and Managing Extensions," for information about extensibility. As a side note, syntax colorization is the minimum that an extension must provide to add support for a new language.
Delimiter Matching and Text Selection

Delimiter matching
This feature is also very useful when you need to visually delimit nested blocks and with complex and long expressions. It is worth mentioning that you can press Ctrl+D to fast select a word or identifier at the right of the cursor and that you can also easily expand (Shift+Alt+Right) and shrink (Shift+Alt+Left) text selection within enclosing delimiters of a code block.
Code Block Folding
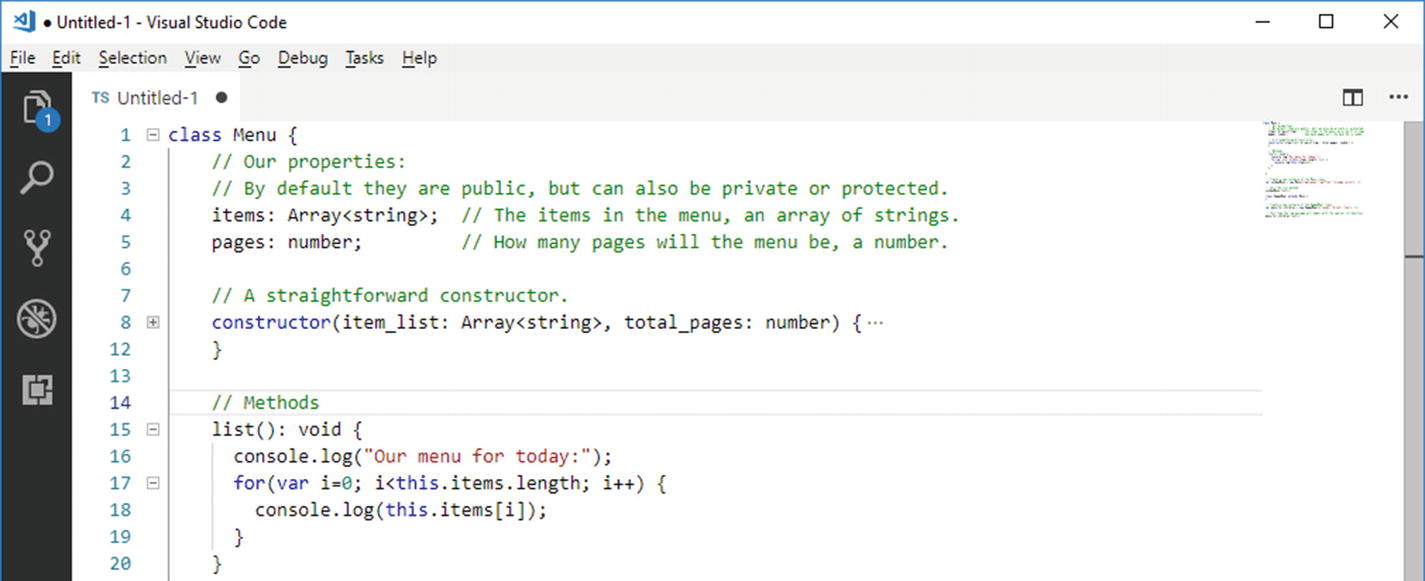
Code block folding
Multicursors
The code editor supports multicursors . Each cursor operates independently, and you can add secondary cursors by pressing Alt-Click at the desired position. You will see that secondary cursors will be rendered thinner. The most typical situation in which you want to use multicursors is when you want to add (or replace) the same text in different positions of a code file.
Reusable Code Snippets
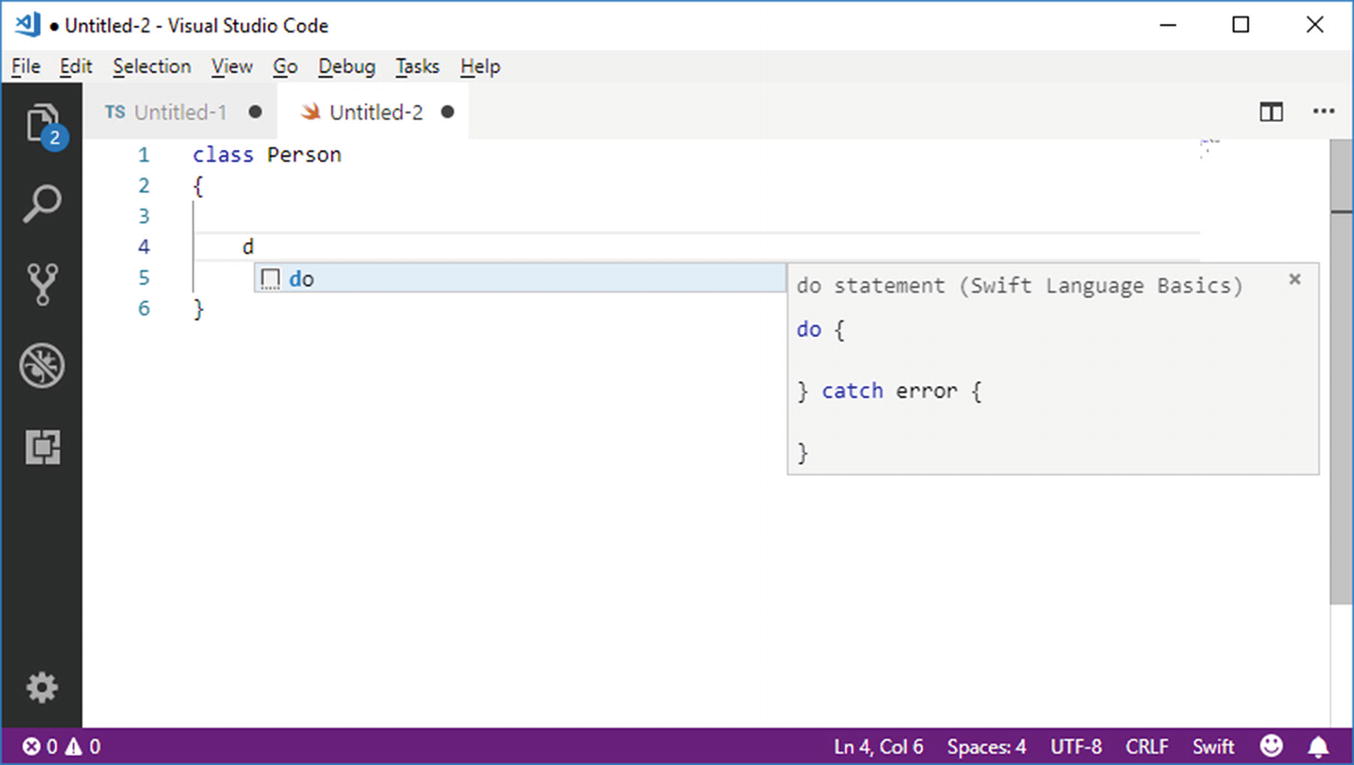
Adding code snippets
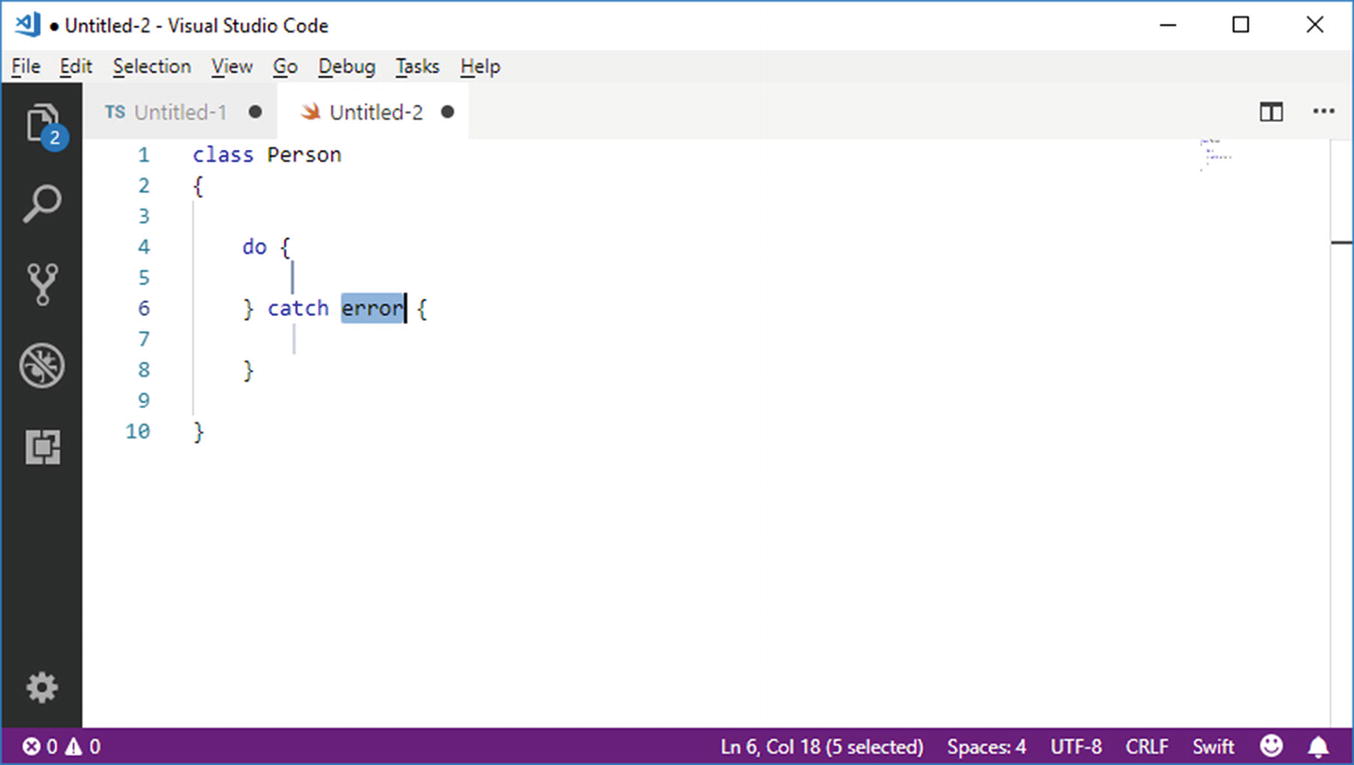
A newly added code snippet with a variable name highlighted
Notice that if the code snippet contains variable names or identifiers, these might be highlighted suggesting that you should give them a different name (like for the error identifier in Figure 3-5). When you rename a highlighted identifier, all occurrences will be also renamed.
Visual Studio Code is not limited to built-in code snippets. You can download code snippets produced by other developers for many languages from the Visual Studio Marketplace. Actually, most of the extensions that introduce or extend support for programming languages also include a number of code snippets .
Word Completion
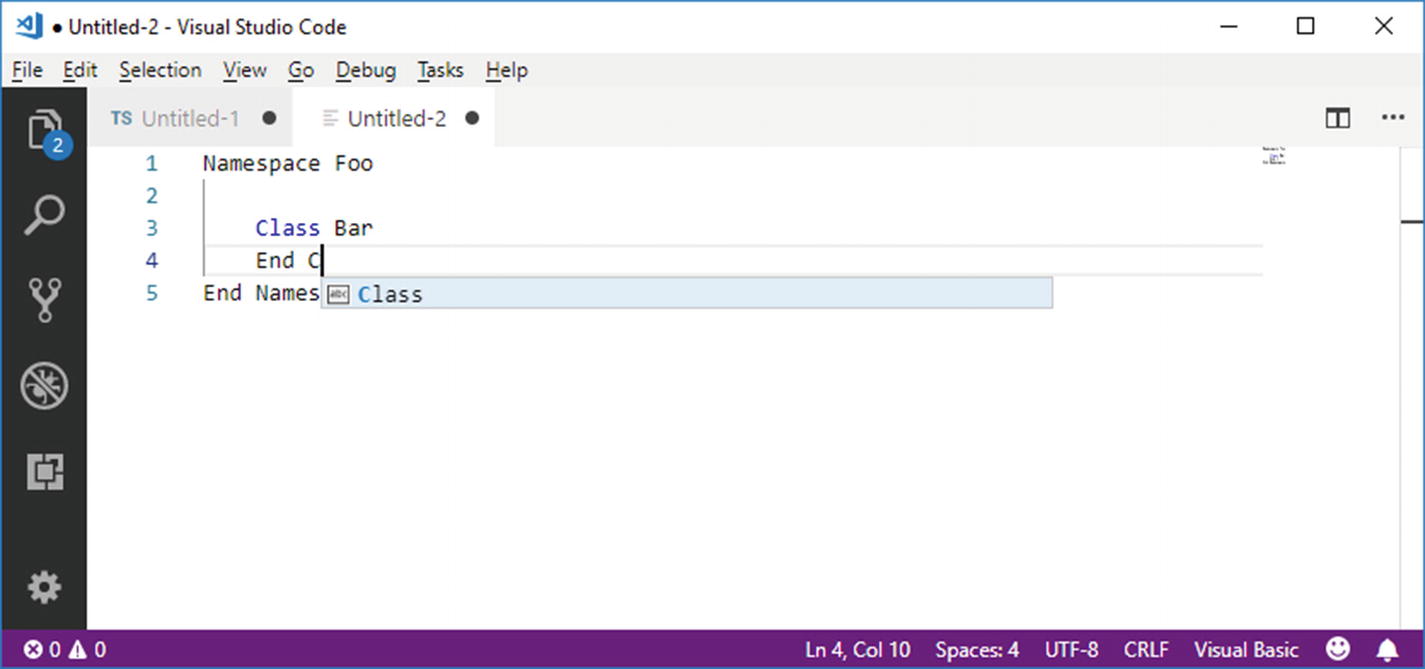
Completing a statement with word completion
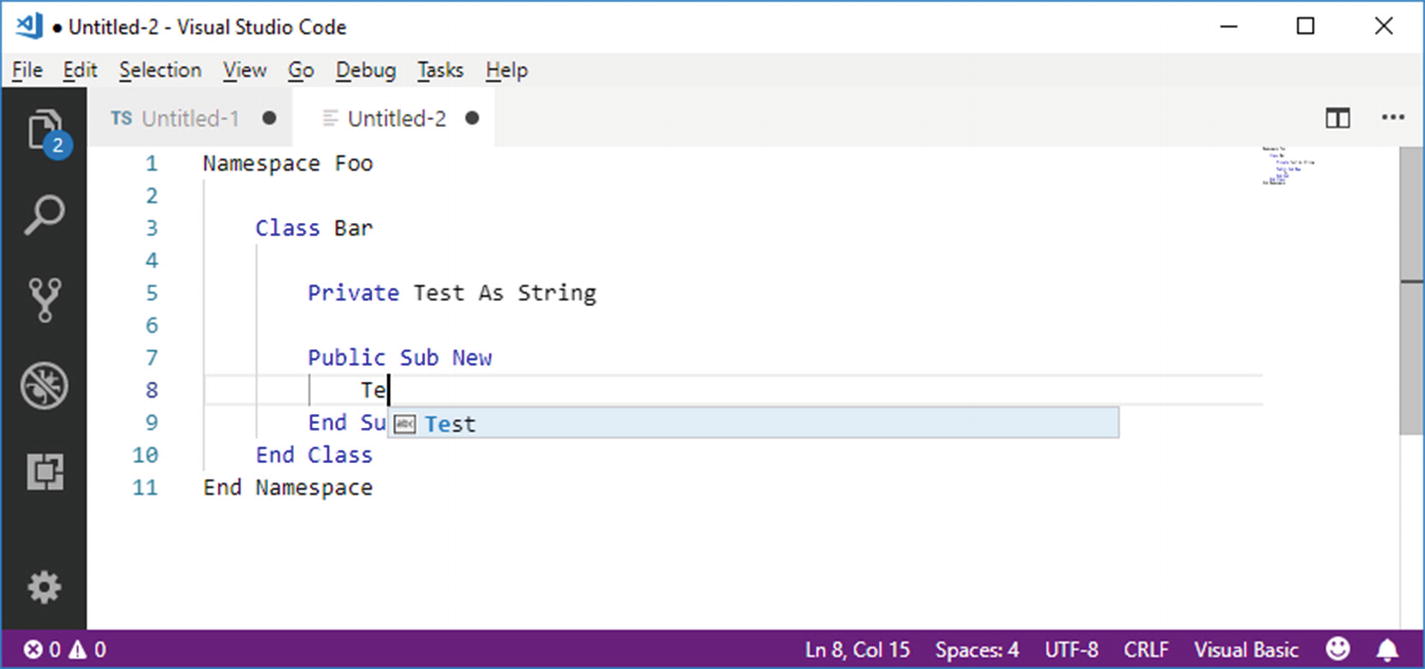
The code editor can suggest identifiers declared in the code
Minimap Mode

The Minimap allows for previewing source code on the scrollbar
If you click the Minimap, the portion of source code that is visible in the code editor is highlighted in the scrollbar, so that you can have a better perception of the current position of the cursors. The Minimap can be disabled and enabled using the View ➤ Toggle Minimap command.
Whitespace Rendering and Breadcrumbs
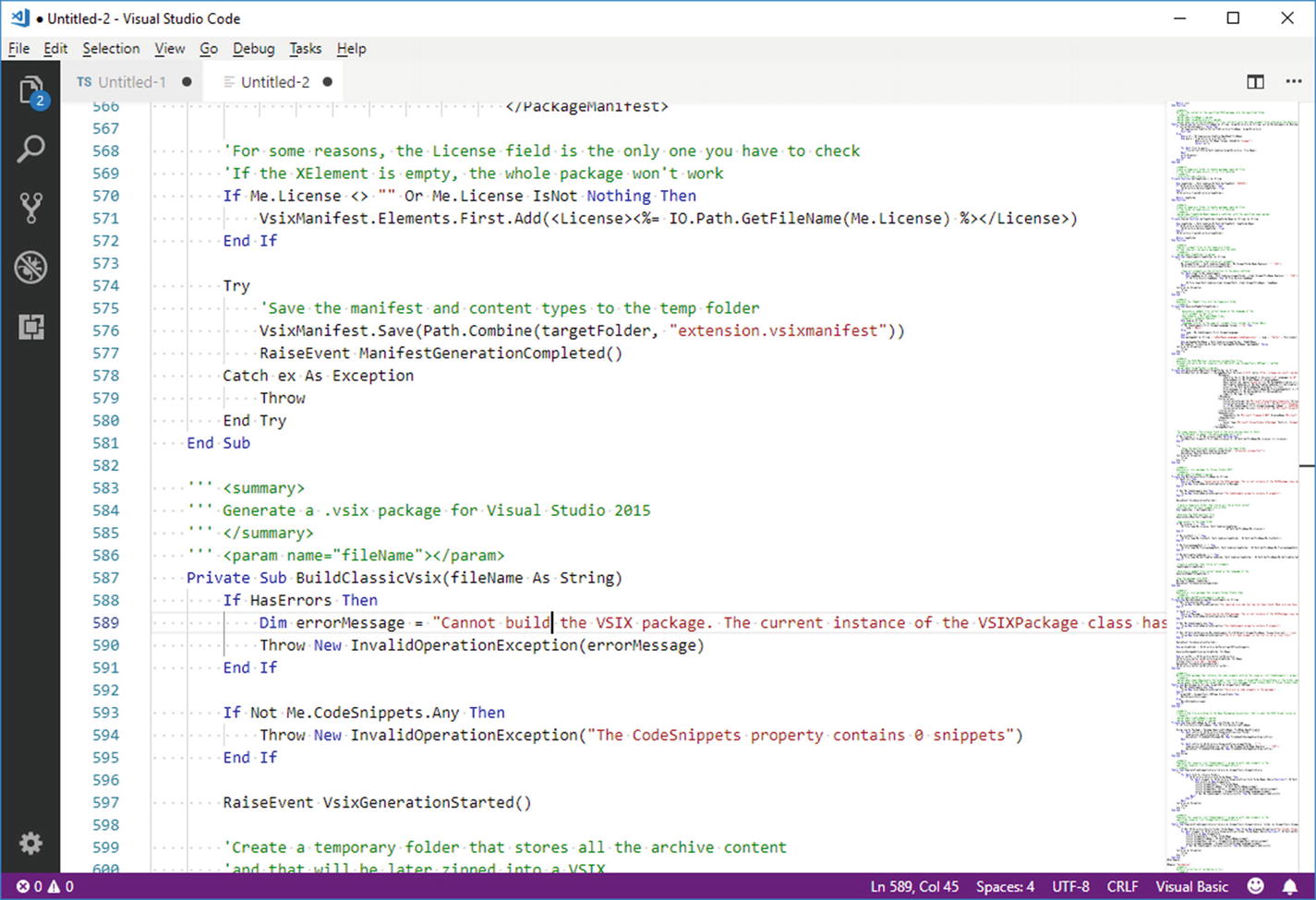
Rendering indentation spaces with dots
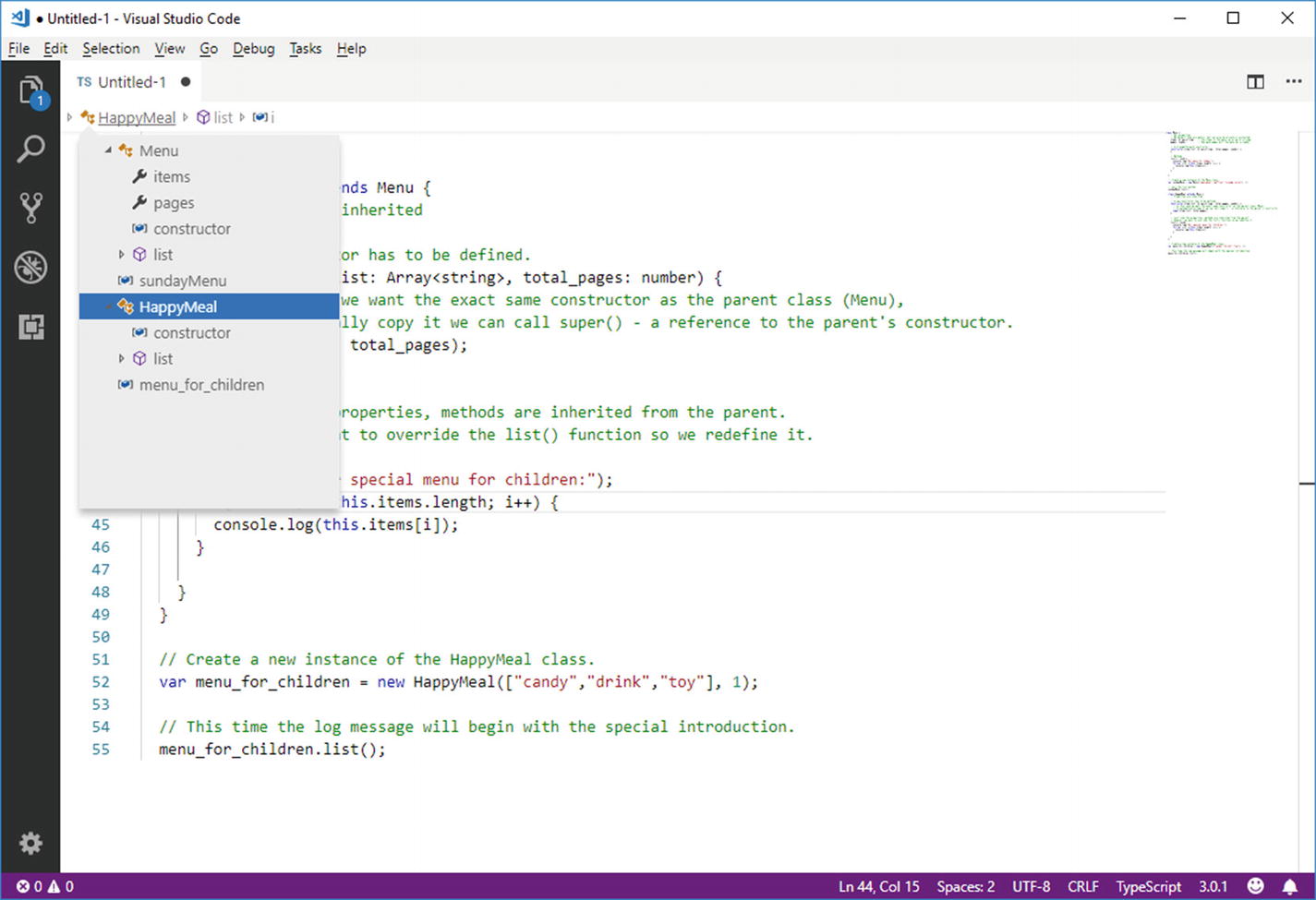
Navigating between types and members with breadcrumbs
By clicking a type or member name, the cursor will be moved to its definition, making code navigation much easier.
Markdown Preview

Integrated Markdown preview
This feature is very useful because it allows to preview your documents without the need of an external program such as a web browser.
Evolved Code Editing
Visual Studio Code is an extremely powerful code editing tool and brings to a cross-platform and multilanguage environment many features that have been available in Microsoft Visual Studio since many years, providing what is called evolved code editing. This section explains all the advanced code editing features that are available, out of the box, to languages such as TypeScript and JavaScript and to languages like C#, C++, and Python with the appropriate extensions installed.
Working with IntelliSense

IntelliSense showing suggestions as you type and advanced word completion
As you can see in Figure 3-12, IntelliSense shows the list of available members as you write, for the given type (in this case Console). When you select a word from the completion list, Visual Studio Code shows the member documentation.
Note
The documentation for a type or member will only be available if it has been supplied by the developers. For example, in C# the documentation for types and members must be provided with XML comments. This will enable IntelliSense to display it in a tooltip, like in Figure 3-12.

Suggestion filtering in IntelliSense
IntelliSense also provides the foundation for other advanced features in the code editor that depend on it, described in the next subsections.
Parameter Hints

IntelliSense shows parameter hints
For languages such as C# and TypeScript or, more generally, with languages that allow for function overloads, parameter hints show the description for the parameters of each overload. You can also scroll the list of overloads with the up and down arrow keys to select a different overload .
Inline Documentation with Tooltips
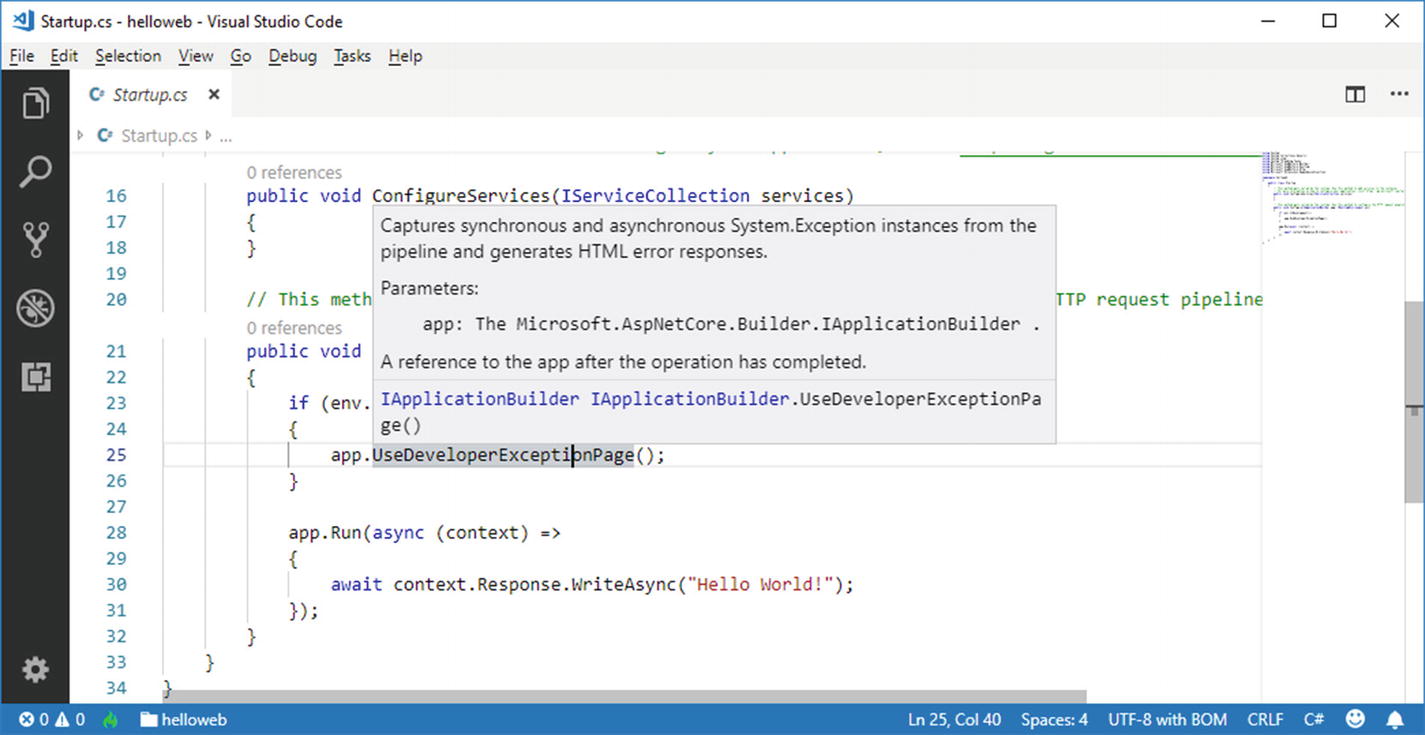
Tooltips provide quick, inline documentation
Like parameter hints, this feature is available only if the documentation has been implemented. If you instead hover a variable name, the tooltip will only show the type for the variable.
Go To Definition
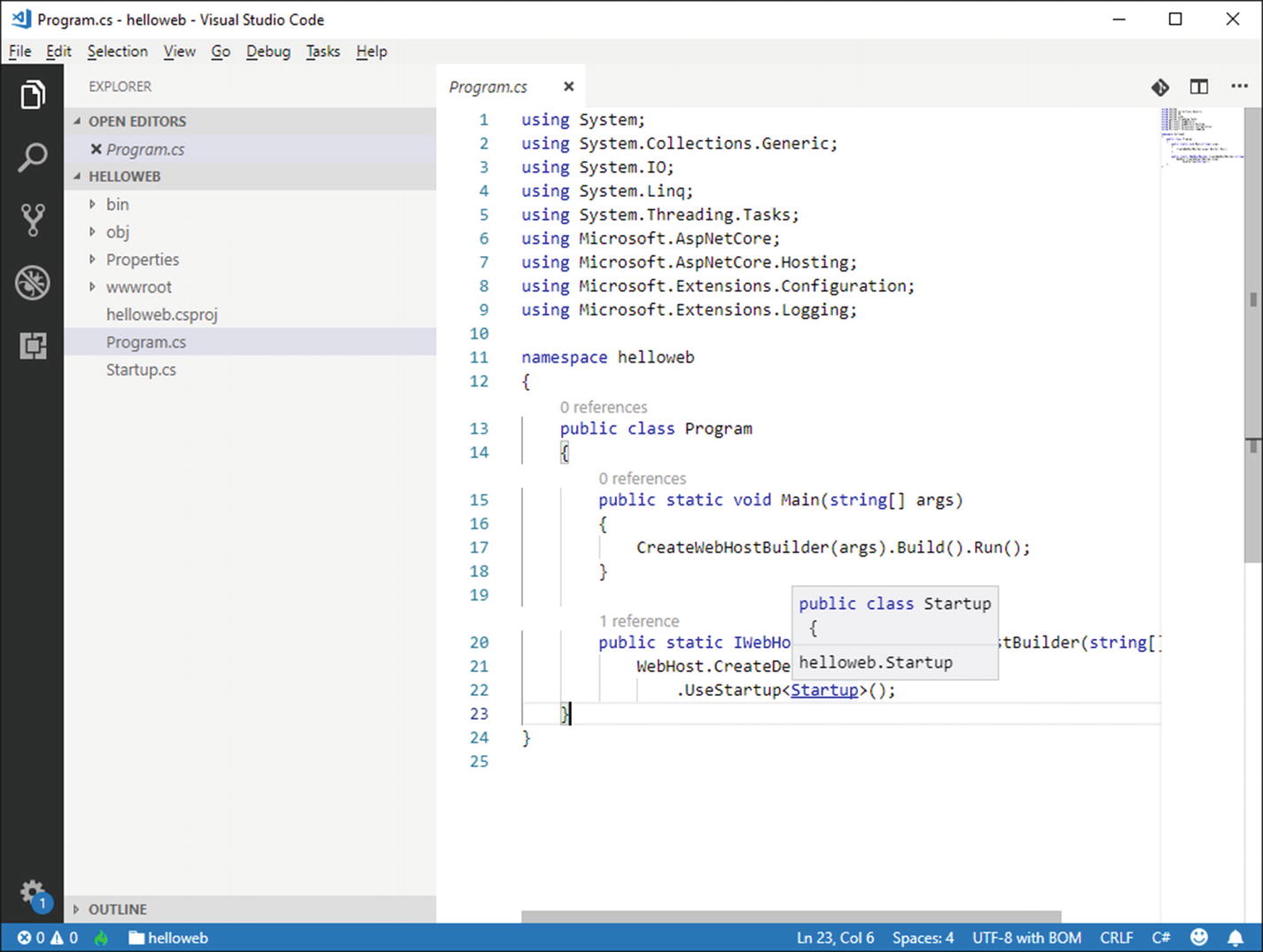
Ctrl + hovering over a type enables Go To Definition
The same tool is available if you select a type name and press F12 or if you right-click a type name and then select Go To Definition from the context menu. This is an extremely useful feature that lets you quickly browse between type definitions that are in different code files.
Note
For C#, Go To Definition can also open the definition of a type exposed by the .NET Core libraries, not just your code.
Find All References

Finding all references of types and members
If you click an occurrence in the list on the right, the code editor brings up a popup containing the code where that occurrence has been found. It is very important noting that this popup is interactive, which means that you can edit the code directly, without the need of opening the containing code file separately. This allows keeping your focus on the code, saving time. Also, notice that the interactive popup shows, at the top, the file name that contains the selected reference .
Peek Definition
Suppose you have dozens of code files and that you want to see or edit the definition of a type you are currently using. With other editors, you should search among the code files, which not only can be annoying but that would also move your focus away from the original code. Visual Studio Code brilliantly solves this problem with a feature called Peek Definition .
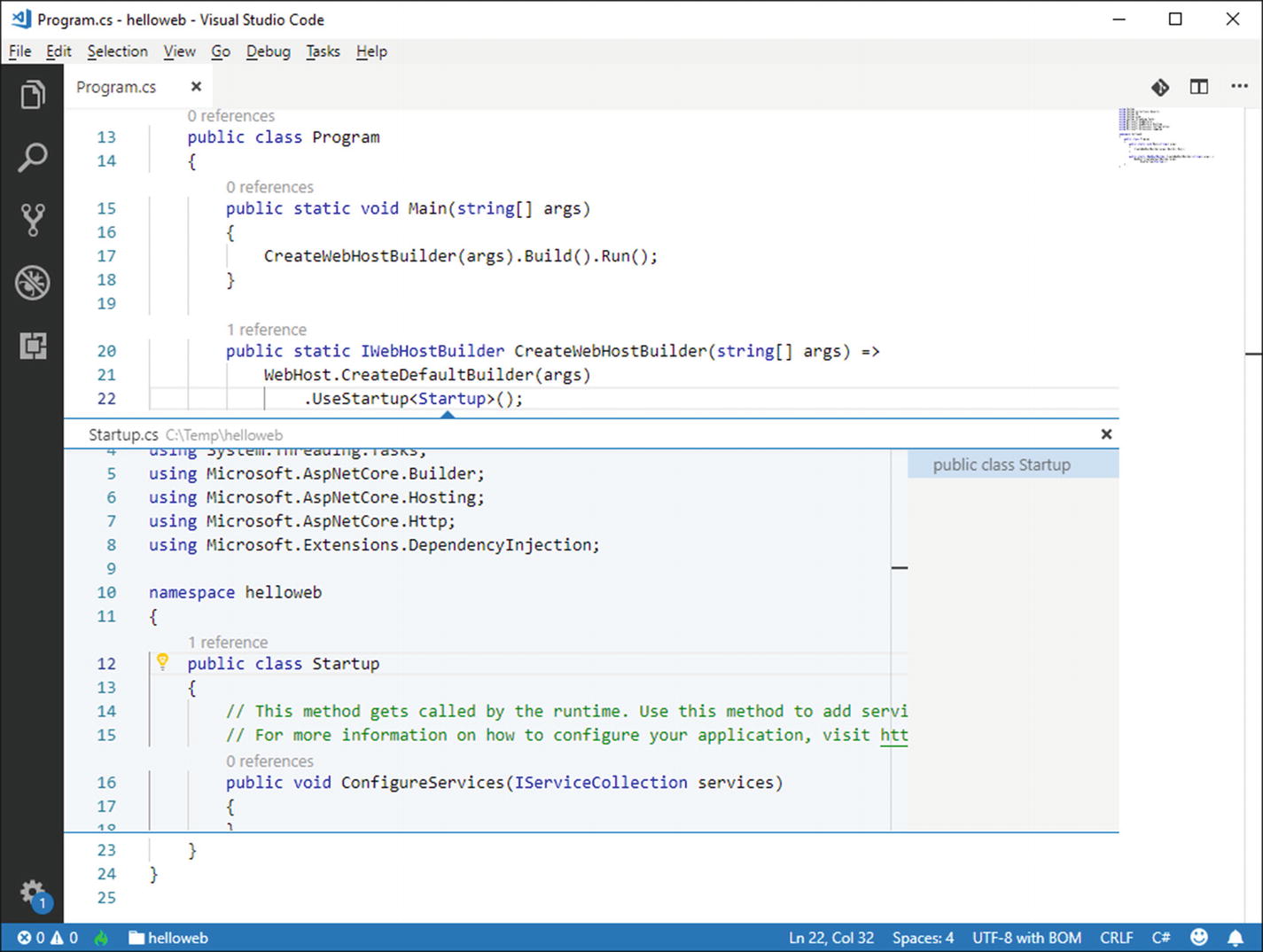
Working on a type defined in another file with Peek Definition
As you can see, the peek window is very similar to the Find All References feature, and it still shows the file name that defines the type at its top. Simply click the file name to open the code file in a separate editor.
Renaming Symbols and Identifiers

Renaming symbols
All references of that symbol will be renamed accordingly. Additionally, you can rename all the occurrences of an identifier. You simply right-click the identifier, then select Change All Occurrences (or press Ctrl+F2 on Windows/Linux and ⌘+F2 on macOS); all the occurrences will be highlighted and updated with the new name as you type.
Live Code Analysis
With C#, TypeScript, and with languages whose support can be enhanced via extensions like Python, Visual Studio Code can detect code issues as you type, suggesting fixes and offering code refactorings. This is one of the most powerful features in this tool, which is something that you will not find in most other code editors. The next examples are based on the C# programming language, since (together with TypeScript) this supports the richest experience possible in Visual Studio Code, and therefore it is a good choice to discuss the powerful coding features available. Of course, everything discussed here applies to all other languages that support the same enhanced features.
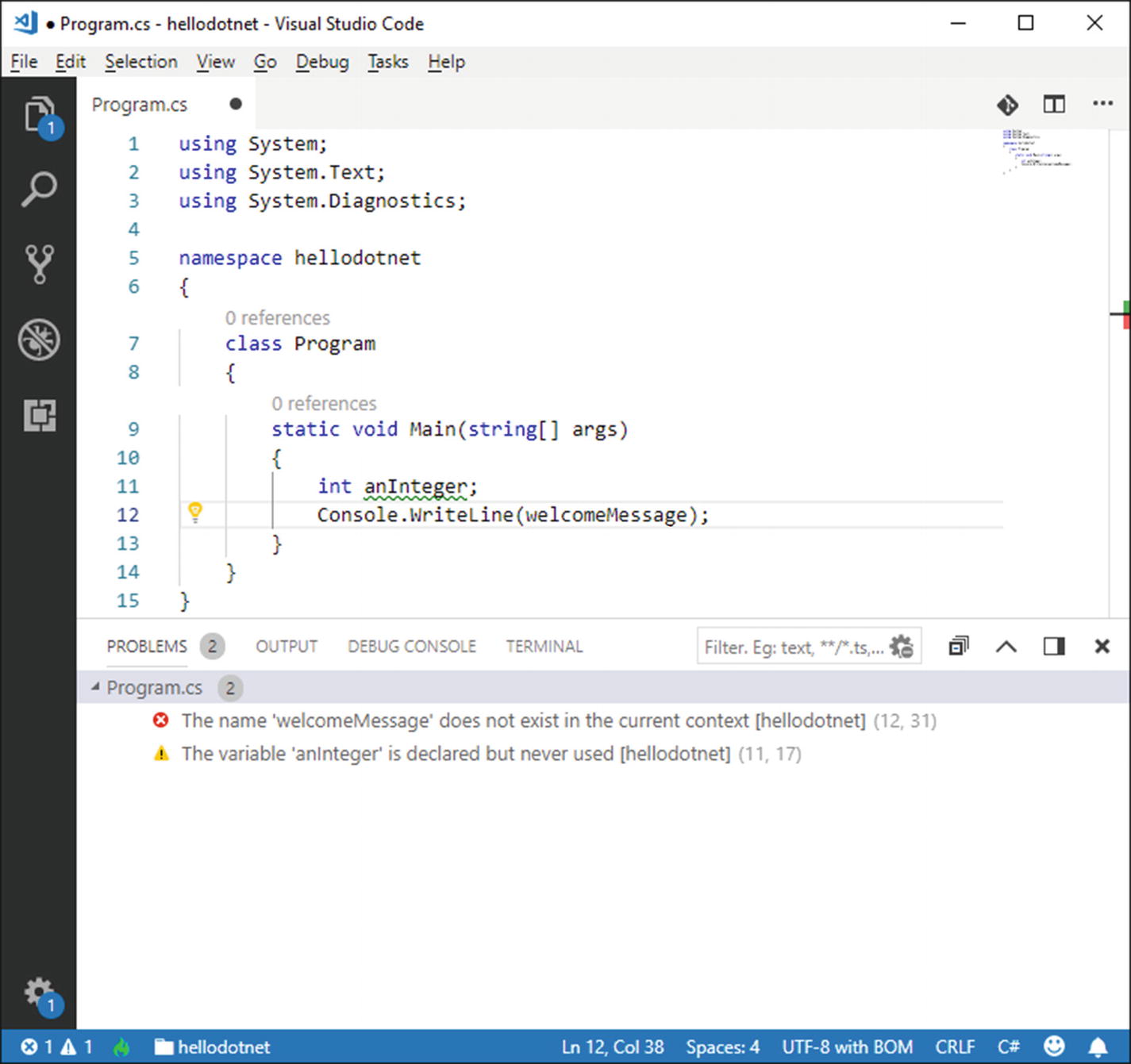
Code issue detection as you type

Potential fixes suggested by the Light Bulb
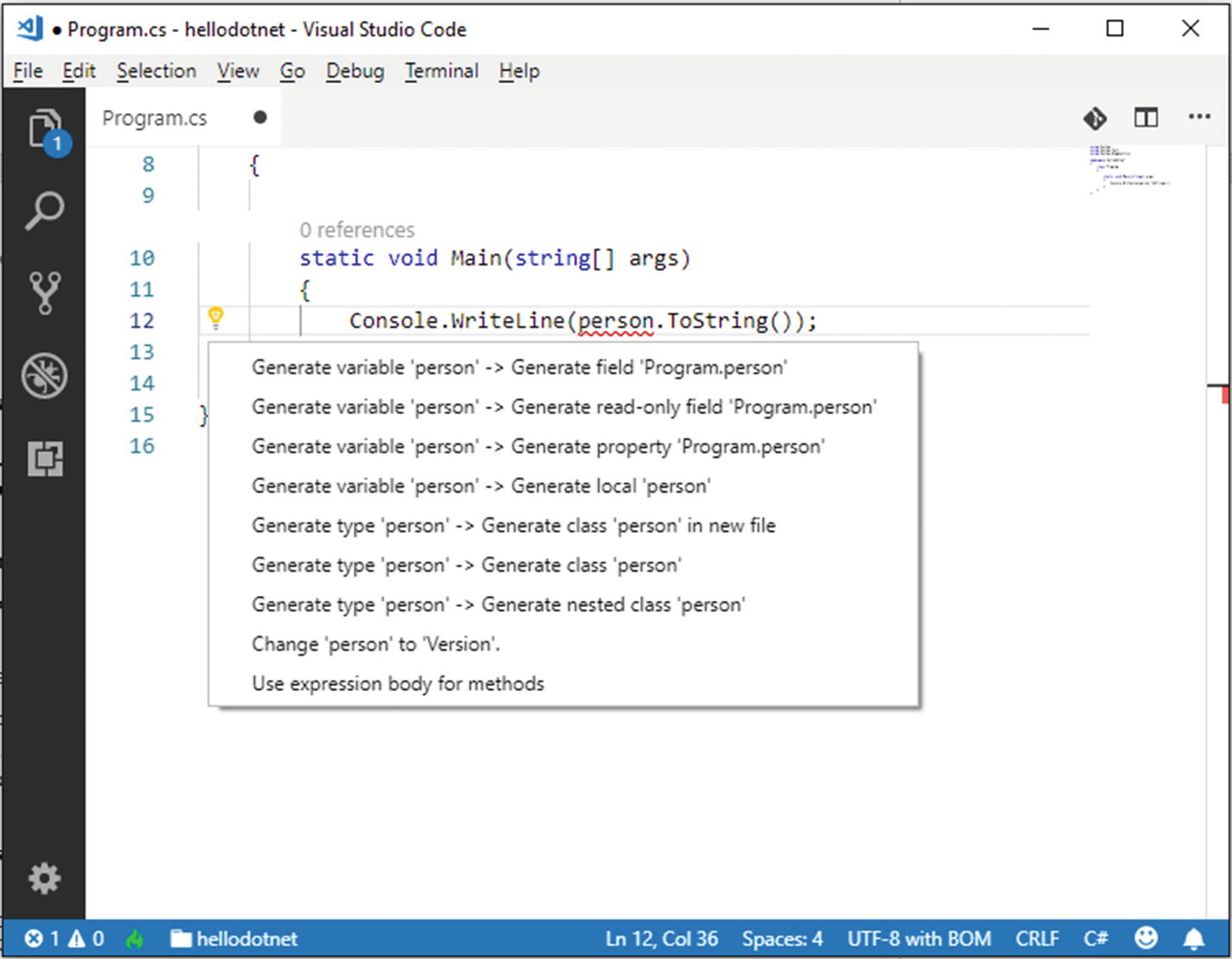
Generating types on the fly

Code refactoring made easy

Adding missing directives

The Light Bulb provides suggestions based on the current context
You would get a similar result, but with different implementation, if the choice was one of the other possible code fixes. Though it is not possible to show examples for all the code fixes that Code can apply, what you have to keep in mind is that suggestions and code fixes are based on the context for the code issue, which is a very powerful feature that makes Visual Studio Code a unique editor.
Summary
Visual Studio Code is a code-centric tool that supports out of the box a wide variety of languages, offering coding features such as syntax colorization, delimiter matching, code block folding, multicursors, code snippets, and code completion that are common to all the supported languages.
In addition, languages such as TypeScript and C# provide the so-called evolved code editing experience via integrated tools such as IntelliSense, Go To Definition and Peek Definition, Find All References, and the extremely powerful Light Bulb that detects code issues as you type and that suggests potential fixes based on the context. Now that you have knowledge of the powerful coding features that Visual Studio Code offers, it is time to see how to use them with individual source code files and structured folders.