Believe it or not, your PowerShell script isn’t going to work right all the time. It will fail and fail hard sometimes. A novice developer typically doesn’t worry much about error handling. Error handling is one of those topics that separates the novices from the professionals.
To create a production-ready, robust PowerShell solution, you must ensure all the ways your scripts can fail are properly handled. Inevitably, they will throw an error when you least expect it and it’s important to catch that error to either fix the problem mid-run or exit gracefully.
Force Hard-Terminating Errors
Unlike many other languages, PowerShell has two types of exceptions/errors – terminating and non-terminating errors. When encountered, a non-terminating error does not stop script execution. It does not terminate code execution. A hard-terminating error, on the other hand, does.
You should not rely on non-terminating errors under most circumstances. Why? Because you have much more control with hard-terminating errors. Non-terminating errors are essentially just red text on the screen. Just like using the Write-Host cmdlet , you don’t have much, if any, control over those errors. Always use hard-terminating errors.
There are a couple of different ways to force a hard-terminating error, either through using the common ErrorAction parameter or using the global $ErrorActionPreference automatic variable.
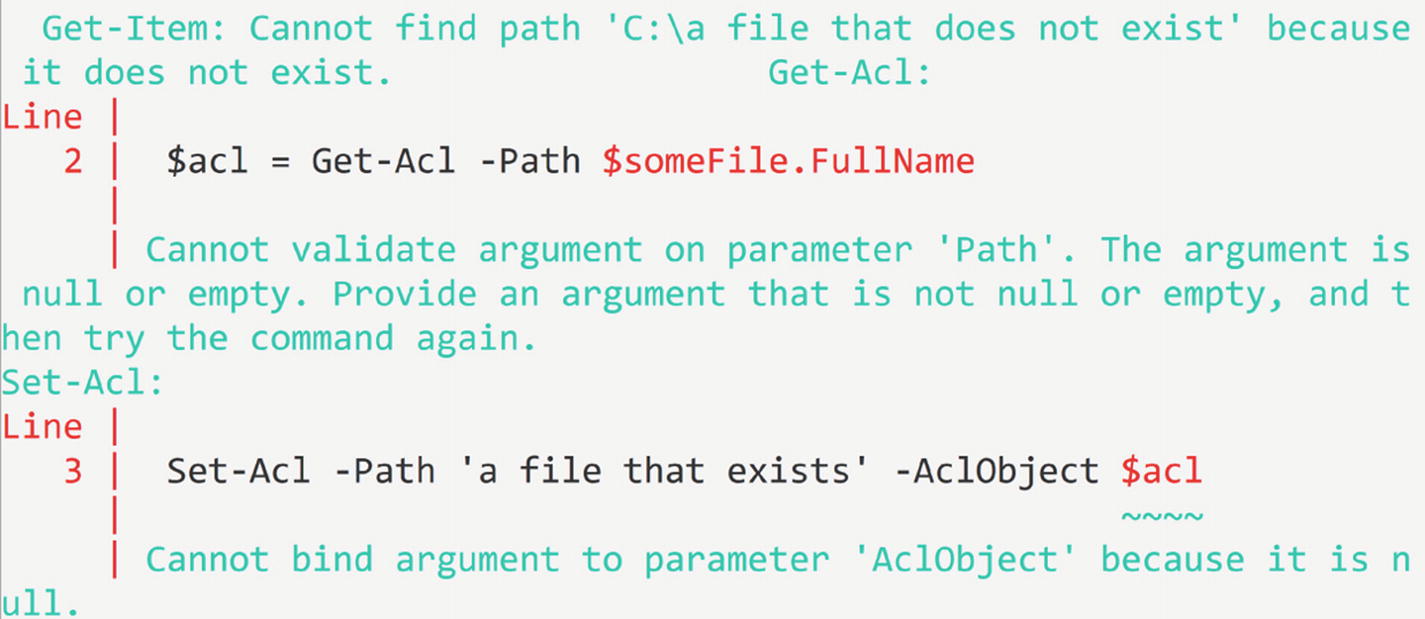
Three non-terminating errors
When you run the script now, you receive a nice warning. PowerShell threw the exception that Get-Item created and the catch block caught it and ran the code inside.
Use $ErrorActionPreference and the ErrorAction parameter as much as possible to ensure you can control those exceptions!
Further Learning
Avoid Using $?
It’s important to write code as simple as possible. Convoluted, complex code does no good to anyone regardless if you think you look smart doing it or if you think it’s saving you a few characters of typing. You should always focus on writing code that others can read and understand.
PowerShell has a default variable called $? which some are tempted to use. Just looking at this variable, what do you think it does? You undoubtedly have no idea. There’s no name or indication of what this variable’s purpose is. The only way to find out is to read some documentation. This is not the type of code you want to write.
To save you some time scouring through the documentation, this variable indicates if the last command executed was successful or not by returning True or False. Not only is this variable’s name unintuitive, but it also just returns a single Boolean value if an error occurred. It provides no other information.
At the end of the day, the preceding code is accomplishing the same goal as the earlier try/catch example with a hard-terminating error. But, by using the much less known $? variable, the script isn’t near as readable.