There will inevitably be tips that don’t fit the mold. Tips that don’t necessarily fit in a chapter and not enough of them were found to create a chapter will be here.
In this chapter, you will find a smorgasbord of tips ranging from string and array best practices, interactive prompting, creating PowerShell script shortcuts, and more.
Write for Cross-Platform
At one time, PowerShell was called Windows PowerShell. PowerShell only existed on Windows. Not anymore. Nowadays, PowerShell is available on just about every platform out there. As a result, scripts are being run on Windows, Linux, macOS, and other platforms every day.
If you’re writing PowerShell for the community or others in your organization, it’s a good idea to ensure those scripts are cross-platform compatible. IT workloads are constantly moving around on-prem and to/from the cloud on different operating systems. A web server can just as easily run on nGinx as it can on IIS. You should write scripts to account for this potential change even though there are no plans to run on another platform.
Even though the Microsoft PowerShell Team has taken every effort to maintain complete parity between Windows PowerShell and PowerShell, there will be differences, especially across different operating systems. If you believe your script may run on both versions, be sure to write and test for both.
Now let’s say you’ve been working hard on this script and want to share it with others. This script doesn’t necessarily need to be run on Windows. There is no dependency on Windows because perhaps you’re querying an API with the Invoke-RestMethod cmdlet that’s available cross-platform.
- 1.
ls is a shell command in macOS. However, on Windows, ls is an alias to the Get-ChildItem cmdlet. You’re running two separate commands expecting both to have the same functionality.
- 2.
The Encrypt() method is not available on macOS. It’s only available in Windows. If you need to encrypt the files, you need to find a cross-platform way to do it.
You don’t have to always write code to be cross-platform but it can never hurt!
Tip Source: https://twitter.com/migreene
Further Learning
Don’t Query the Win32_Product CIM Class
One common use for PowerShell is to find installed software on a Windows machine. Installed software can be found in various places in both WMI, the registry and the file system.
One place that installed software is located is within the Win32_Product CIM class. It may be tempting to use Get-CimInstance to query this class but don’t! This class is special and forces Windows to run external processes behind the scenes. It’s slower to respond and will instruct Windows to run msiexec for each instance when you don’t expect it.
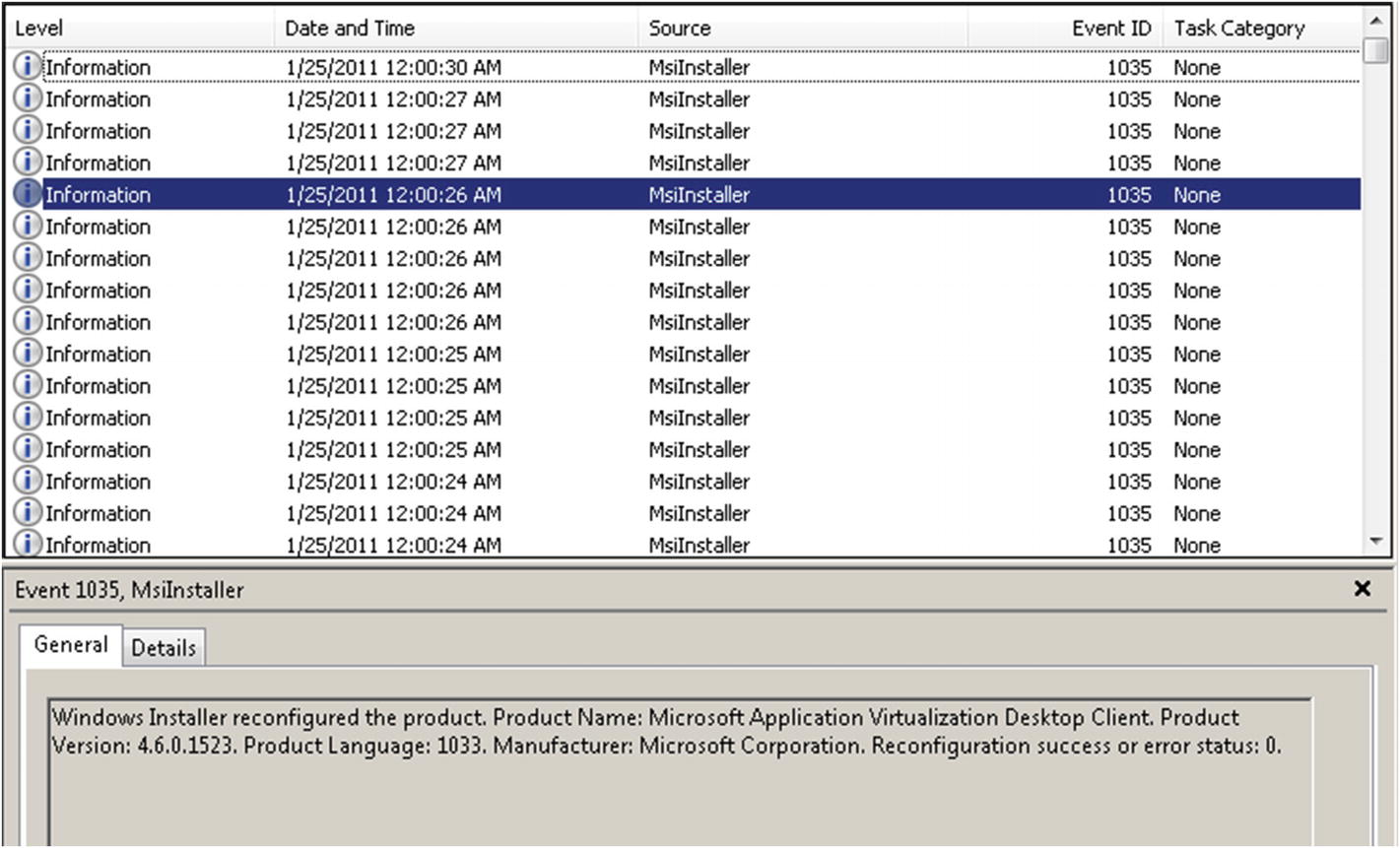
MsiInstaller event log messages
Instead of querying the WMI Win32_Product class, use the registry. You can query the registry yourself, but to save time, check out the PSSoftware community module or any other that queries the registry instead.
Further Learning
Create a Shortcut to Run PowerShell As Administrator
Much of the time, you need to run PowerShell “as administrator” in Windows. Doing so allows you to perform many system tasks you wouldn’t typically have access to.

Manually running PowerShell “as administrator”
Replace Start-Process PowerShell with Start-Process pwsh to launch PowerShell Core “as administrator”
Tip Source: https://www.reddit.com/user/Wugz/
Further Learning
Store “Formattable” Strings for Later Use
You can store “formattable” strings without actually using them until you need to. What’s a “formattable” string? As mentioned in the “Write for the Next Person” chapter, PowerShell allows you to create placeholders in strings to replace via the -f operator.

Replacing placeholders with values in a string
You can use this feature to your advantage by also storing the entire string with placeholders in a variable and then replacing those placeholders with a variable. For example, perhaps you need to store a common SQL query in code but one value in that query will change. You could create the string with placeholders, assign it to a variable, and invoke the code later.
Tip Source: https://www.reddit.com/user/Vortex100/
Further Learning
Use Out-GridView for GUI-Based Sorting and Filtering
Sometimes the command line isn’t enough. Whether you’re feeling mouse-happy or you’re giving a script to a user that needs a GUI, you can easily display objects using a cmdlet called Out-GridView . The Out-GridView cmdlet takes one or more objects as input and then displays them in a window with a grid. You can then filter, sort, and click around the rows as much as you’d like.

Viewing Windows services in a GUI-based grid layout
Not only can you create a nice, grid layout of objects, you can also provide input to other commands via the grid by passing selected objects using the PassThru parameter.
The Out-GridView cmdlet is an easy and simple way to create an interactive, GUI-based grid layout.
Tip Source: https://www.reddit.com/user/alphanimal/
Further Learning
Don’t Make Automation Scripts Interactive
When you’re building scripts to automatically perform tasks, don’t introduce any kind of interactivity. The point of automation is complete, hands-off-the-keyboard behavior. The last thing you want to want to happen is to be woken up in the middle of the night because a mission-critical script didn’t run because it was waiting on you to type something in.
Every time a script prompts for input, it’s paused. The entire script just waits for a human to come along and type some characters on the keyboard. Don’t create this. Instead, think through the input you need to pass at runtime and automatically use that information.
Don’t define a mandatory parameter and not pass a value to that parameter:
The only place to prompt for anything is when someone intends to be in front of that code when it runs.